How to Fix the SqlExceptionHelper Null Return in Hibernate with Spring Boot: A Step-by-Step Guide
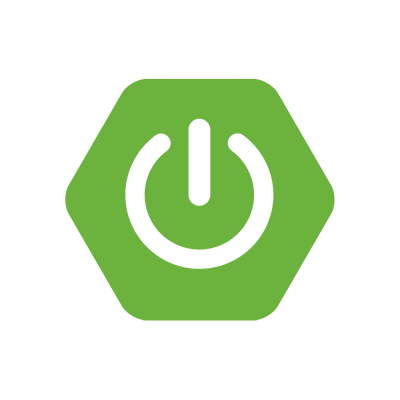
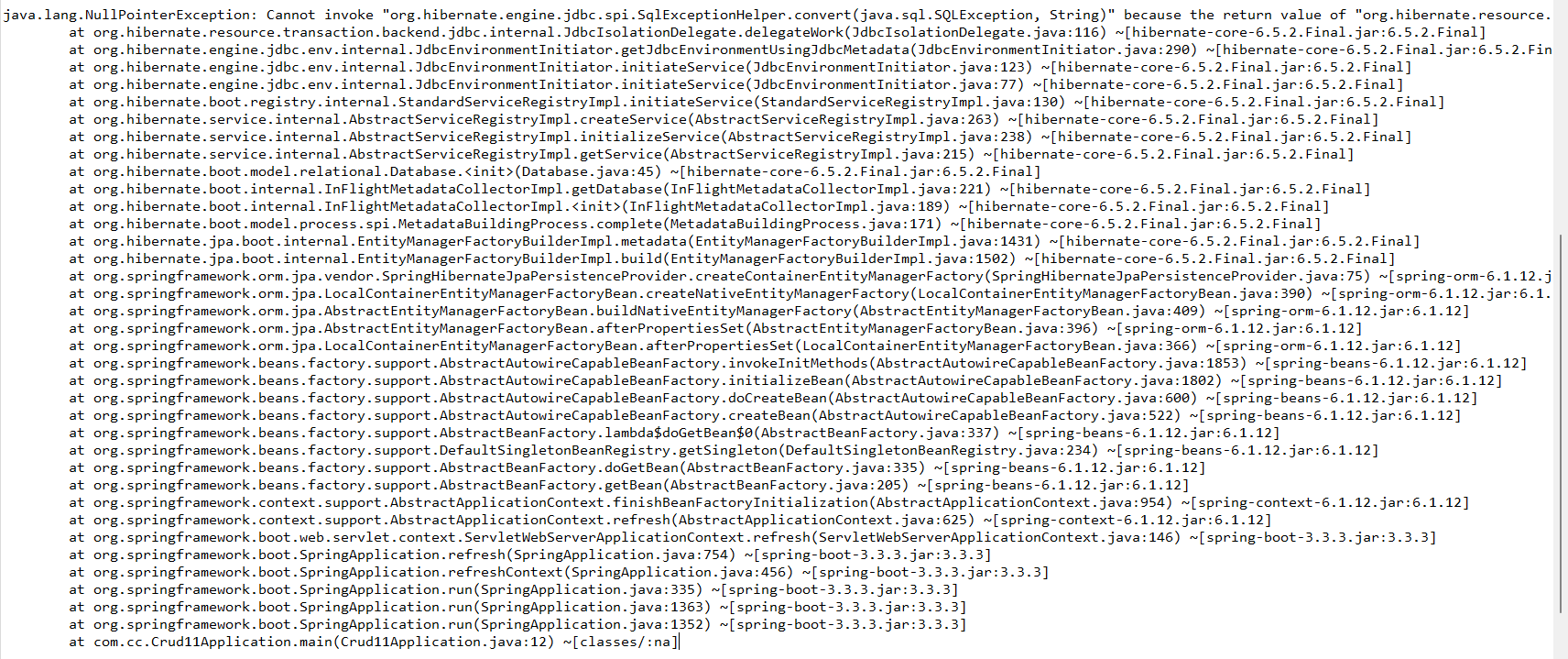
If you’ve encountered the error message:
Cannot invoke "org.hibernate.engine.jdbc.spi.SqlExceptionHelper.convert(java.sql.SQLException, String)" because the return value of "org.hibernate.resource.transaction.backend.jdbc.internal.JdbcIsolationDelegate.sqlExceptionHelper()" is null
while working with Hibernate and Spring Boot, you’re not alone. This error occurs when Hibernate’s JdbcIsolationDelegate
fails to properly initialize, often due to configuration issues related to database connectivity or transaction management.
In this article, we’ll explore the common causes of this error and provide detailed solutions to help you resolve it. By the end of this guide, you’ll be able to troubleshoot and fix this issue in your Spring Boot application.
Table of Contents
Understanding the Error
The error occurs because Hibernate’s sqlExceptionHelper()
method is returning null
. This usually points to an incomplete or incorrect setup of the database environment. The issue often lies in Hibernate's inability to establish a proper connection with the database, or a misconfigured transaction handling mechanism in Spring Boot.
Here’s how you can systematically address the issue.
Step-by-Step Solutions
1. Verify Hibernate Configuration
One of the most common causes of this error is incorrect Hibernate configuration. Double-check your application.properties
or application.yml
file to ensure all database-related properties are correctly defined.
Example:
spring.datasource.url=jdbc:mysql://localhost:3306/yourdb
spring.datasource.username=yourusername
spring.datasource.password=yourpassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.hibernate.ddl-auto=update
spring.jpa.database-platform=org.hibernate.dialect.MySQLDialect
Key Points:
Make sure your
datasource.url
,username
, andpassword
values are correct.Use the correct dialect for your database. For example, if you are using MySQL, the dialect should be
org.hibernate.dialect.MySQLDialect
.
2. Ensure Proper Transaction Management
Improper transaction management could also lead to the error. Spring Boot uses declarative transaction management through @Transactional
. Ensure you have enabled transaction management in your Spring Boot application.
Solution:
In your main configuration class, ensure the
@EnableTransactionManagement
annotation is added.@EnableTransactionManagement @Configuration public class AppConfig { // configuration }
If using JPA, ensure that your transaction manager bean is correctly configured.
@Bean public PlatformTransactionManager transactionManager(EntityManagerFactory emf) { return new JpaTransactionManager(emf); }
3. Check Hibernate and Spring Boot Version Compatibility
Another common issue arises from version incompatibility between Hibernate and Spring Boot. In your case, you’re using Hibernate 6.5.2.Final
and Spring Boot 3.3.3
. Make sure that these versions are compatible.
Steps to Resolve:
Update your Hibernate and Spring Boot versions to the latest stable releases.
You can check the official documentation or issue trackers for known compatibility issues.
4. Review Connection Pool Configuration
If you’re using a connection pool (like HikariCP), ensure that it’s properly configured. Incorrect connection pool settings can cause Hibernate to fail when trying to connect to the database.
Sample Configuration:
spring.datasource.hikari.connection-timeout=20000
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.idle-timeout=30000
Check for the connection pool library in your pom.xml
or build.gradle
file and ensure the version matches your Spring Boot configuration.
5. Fixing the Null Reference Issue
The error message specifically mentions that sqlExceptionHelper()
is returning null
. This indicates that either Hibernate’s JdbcIsolationDelegate
or JdbcEnvironmentInitiator
is not being initialized properly.
Key Considerations:
If you’ve overridden any of Hibernate’s internals, such as the
JdbcIsolationDelegate
orJdbcEnvironmentInitiator
, verify that these components are correctly initialized.Look into any custom transaction handling logic you may have added, as it might interfere with Hibernate's default behavior.
6. Test Database Connectivity
If Hibernate cannot establish a connection to the database, it may lead to this error. Ensure that the database is up and running, and that your application has access to it.
Steps to Check:
Test your database connection from the command line or database client (e.g., MySQL Workbench, pgAdmin).
Check the firewall settings or network configurations that may be blocking database access.
7. Enable Hibernate Logging for More Insights
To gain deeper insights into the issue, enable Hibernate logging. This will provide you with more detailed logs on what happens before the error occurs.
Configuration:
logging.level.org.hibernate.SQL=DEBUG
logging.level.org.hibernate.type.descriptor.sql.BasicBinder=TRACE
With SQL and Binder logging enabled, you’ll be able to see the SQL queries Hibernate is generating and binding, which can help in pinpointing the issue.
8. Ensure Dependencies Are Complete
Finally, ensure that all necessary Hibernate and Spring ORM dependencies are included in your project. Missing dependencies can result in null references or incomplete configuration setups.
Check your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.5.2.Final</version>
</dependency>
Ensure you’re not missing any transitive dependencies required by Hibernate and Spring.
Conclusion
The SqlExceptionHelper
null return issue in Hibernate with Spring Boot can be frustrating, but by systematically addressing the common causes—such as misconfigurations, version compatibility, and transaction management—you can resolve the issue.
Make sure your Hibernate configurations are correct, check your transaction management setup, and ensure that your application can connect to the database. Debugging Hibernate logs and reviewing your connection pool configuration can also provide valuable insights into what’s going wrong.
By following the steps outlined in this article, you should be able to fix the error and restore normal operation to your Spring Boot application.
Subscribe to my newsletter
Read articles from Nikhil Soman Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
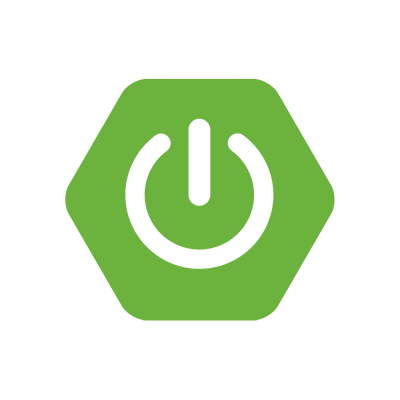
Nikhil Soman Sahu
Nikhil Soman Sahu
Software Developer