Can I Build a Micro-SaaS Platform to Collect User Feedback?
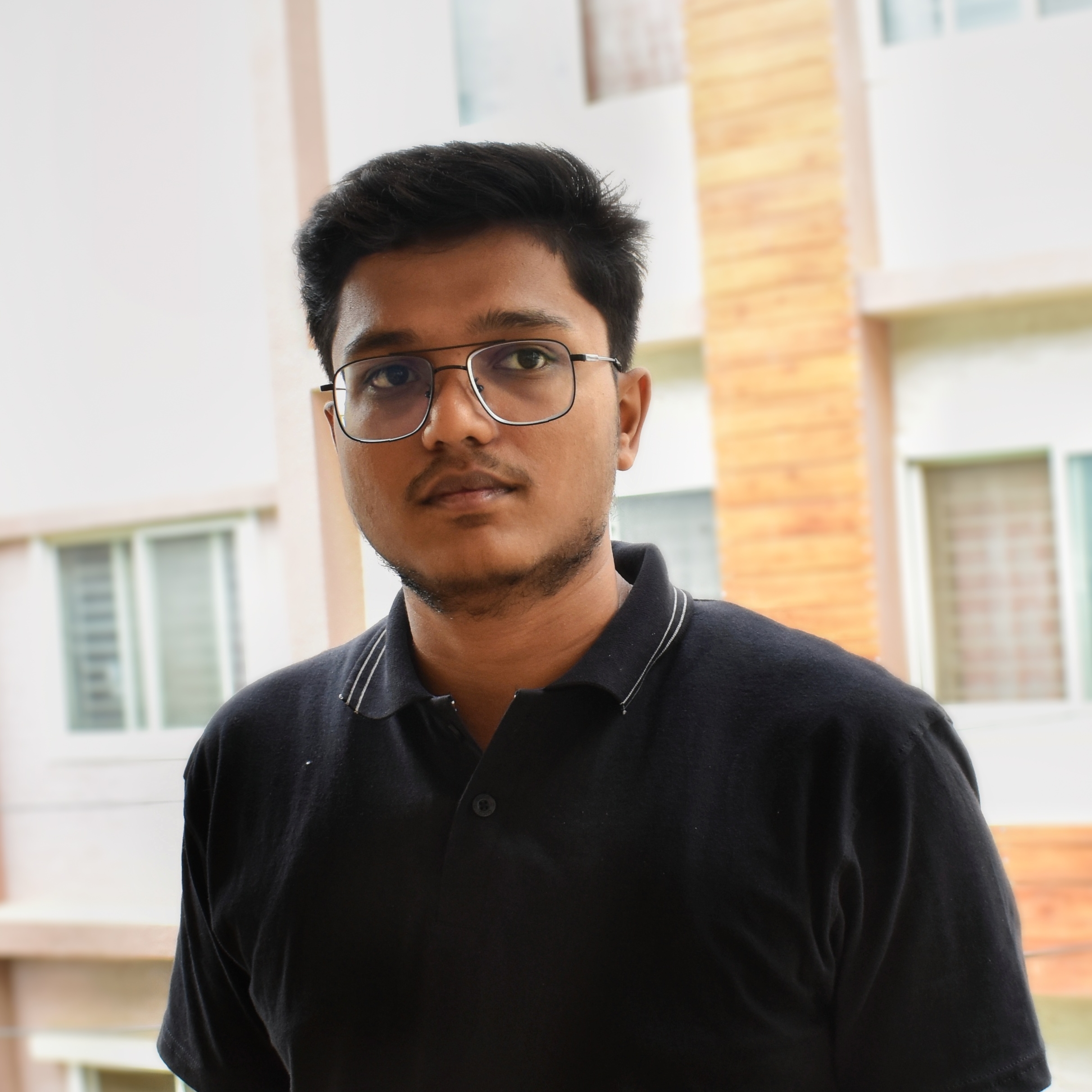
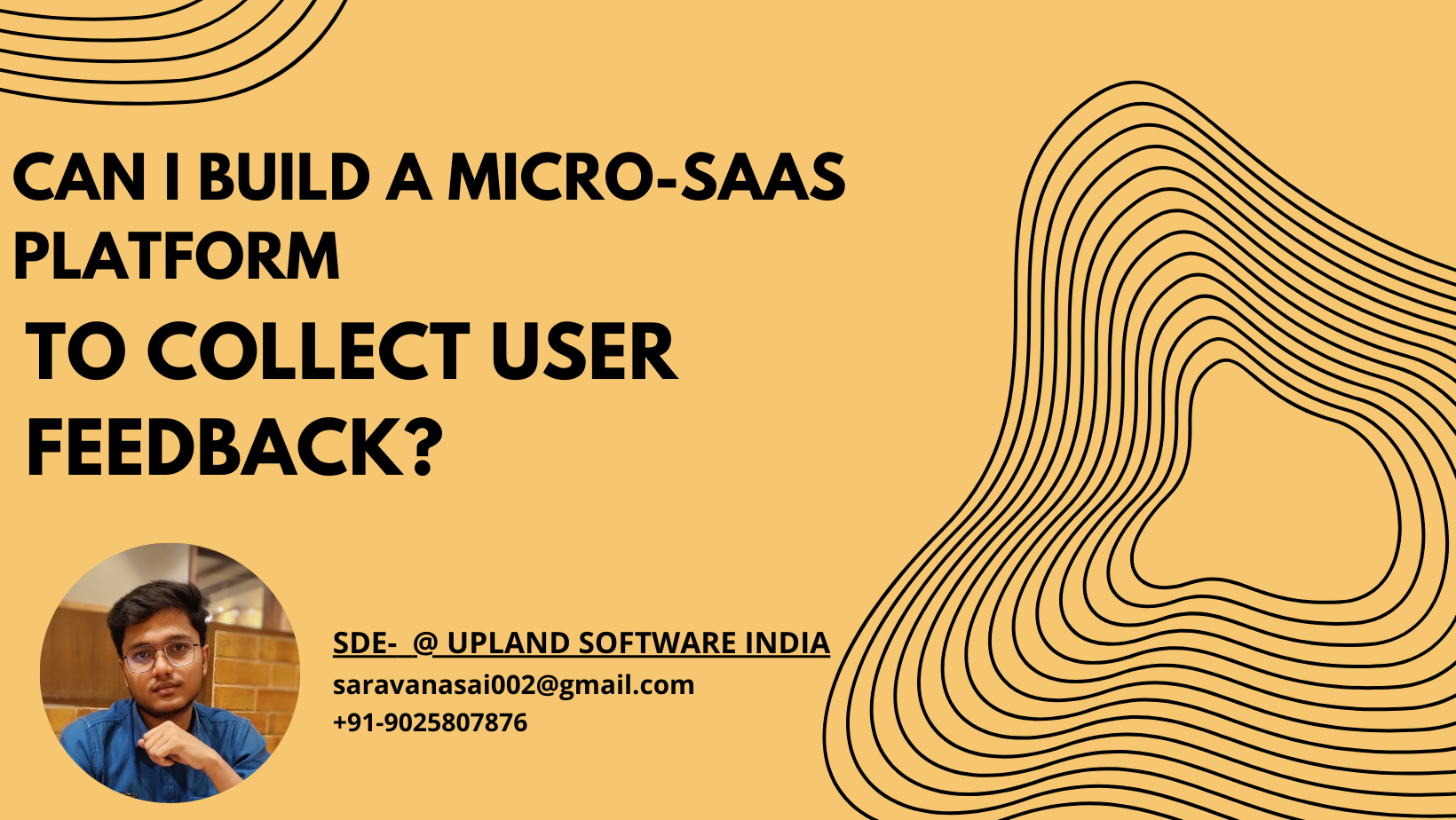
Introduction
Hi Folks, In this article, we'll take a deep dive into the architecture of user feedback popups, exploring the frontend and backend components that work together to make them tick. We'll examine the technologies and techniques used to create these popups, from the client-side rendering of the popup itself to the server-side processing of user input.
Back story
As a curious engineer, I've always been on the lookout for innovative micro SaaS ideas to kickstart my side hustle. One day, while browsing online, I stumbled upon a fascinating platform that caught my attention. It allowed website owners to create a feedback form, integrate it with their site using a simple JavaScript script, and receive user feedback via email. The elegance of this solution sparked my curiosity - what magic happens behind the scenes to make this seamless experience possible?
Intrigued, I began to dig deeper, researching the high-level architecture of such platforms. How do they enable website owners to create customizable feedback forms? How do these forms communicate with the website, and what triggers the popup to appear? What about the back-end infrastructure that processes and forwards the user feedback to the website owner?
In the following sections, I'll take you through the high-level architecture of a user feedback platform, explaining how the popup communicates with the website and the magic that happens behind the scenes to deliver a seamless user experience.
High-level design
Backend Service and User Interface
To build a user feedback platform, we need a robust backend service to store and manage feedback forms and responses. A simple database design can include two tables: Users and Forms. The Users table stores information about the website owners who create accounts on our platform, while the Forms table stores the feedback forms and responses.
Onboarding and Creating Feedback Forms
The user interface is straightforward. When a website owner signs up for our platform, we onboard them and ask them to create a question for their feedback form. We also provide a simple UI for them to customize the feedback form's appearance and settings.
Loading the Feedback Form on the User's Website
Once the feedback form is created, we need to figure out a way to load it on the user's website. Here's where things get interesting. Our approach is to create a user-specific endpoint or route that loads the user's feedback form in HTML. This endpoint will serve as a bridge between our platform and the user's website.
Loading the Feedback Form using a Script
To load the feedback form on the user's website, we provide a script file that loads the form in an iframe and attaches it to the website's body. The form is already equipped with an API that submits the user's response to our server. This way, the feedback form is seamlessly integrated into the user's website, and responses are sent to our server for processing.
This approach allows us to decouple the feedback form from the user's website, making it easy to manage and update the form without affecting the website's codebase.
Simple Implementation
As a backend engineer, I've had my fair share of experience building robust backend services for user onboarding and storing forms and responses. However, things get interesting when it comes to loading a popup on a client's website. In this article, I'll dive deep into the magic of making this happen.
The Challenge
My goal was to load a feedback popup on a client's website, which seemed like a daunting task at first. I had an HTML file that loaded the form on an HTML page, but I needed to inject that into the client's website. To achieve this, I came up with a solution that involved hosting a script file that injects an iframe into the user's website. The user needs to pass a token to initiate the feedback form with the token.
The Solution
I used Vue.js for the front end and Laravel 10 for the back end. I created an HTML file that loads the form on an HTML page and then injected it into the client's website using an iframe. Here's the code snippet that creates the iframe. I have hosted it on js deliver.
let iframe = document.createElement("iframe");
function prepareFrame() {
let container = document.getElementById("popup");
iframe.setAttribute("src", "http://localhost:5173/");
iframe.setAttribute("allowfullscreen", true);
iframe.setAttribute("allow", "fullscreen");
iframe.setAttribute("allowtransparency", true);
iframe.setAttribute("scrolling", "no");
iframe.style.width = "420px";
iframe.style.height = "580px";
container.appendChild(iframe);
}
function toggleFrame() {
let action = message;
iframe.contentWindow.postMessage({ action }, "*");
}
window.addEventListener("message", (event) => {
if (event.data.type == "message") {
alert(event.data.message);
}
});
prepareFrame();
When the script loads. I can load the feedback into the iframe. The above example is super simplified to keep the code simple. This enabled me to load the popup on the user's site.
Making the Form Interactive
The next challenge was to make the form interactive. I wanted to show the popup only when the user clicks the feedback button and closes it when they press the close button. After some trial and error, I discovered the magic of JavaScript's Window.parent.postMessage
. This allowed me to pass messages to the iframe and communicate with the parent window.
Working Example
This code sets up a reference to the isOpen
variable, which tracks the state of the popup. It also defines a toggle
function that toggles the isOpen
state. The sendMessageToSite
function sends a message to the parent window using Window.parent.postMessage
. The onMounted
hook sets up an event listener for messages from the parent window, which updates the isOpen
state accordingly.
Conclusion
In conclusion, loading a feedback popup on a client's website requires some creative problem-solving and a good understanding of JavaScript's Window.parent.postMessage
API. By hosting the script file on a CDN and using the postMessage
API, I was able to load the popup on the user's site and make it interactive. I hope this article has provided valuable insights into the magic of loading a feedback popup on a client's website.
Subscribe to my newsletter
Read articles from Saravana Sai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
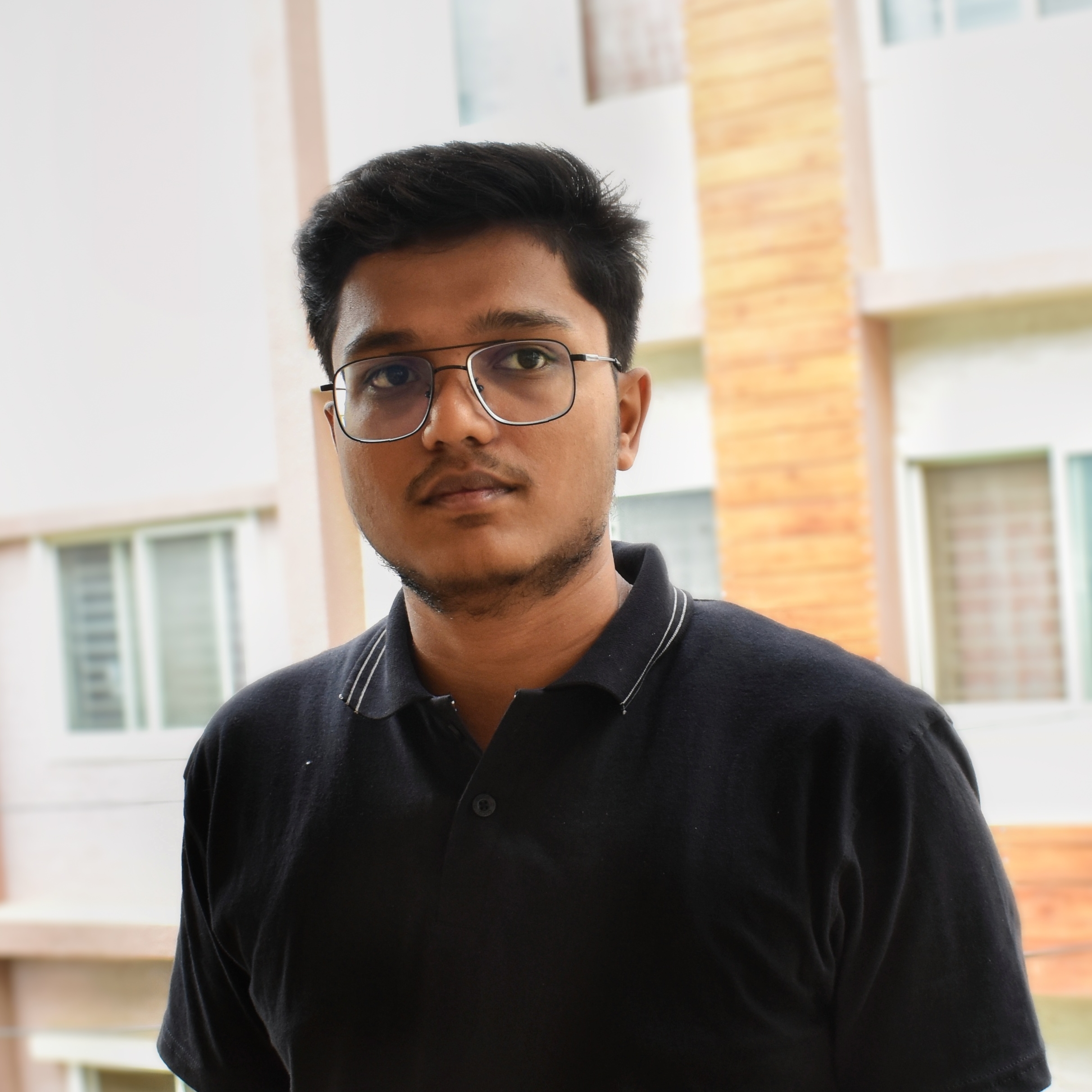
Saravana Sai
Saravana Sai
I am a self-taught web developer interested in building something that makes people's life awesome. Writing code for humans not for dump machine