Understanding Java Operators with Real-World Examples :

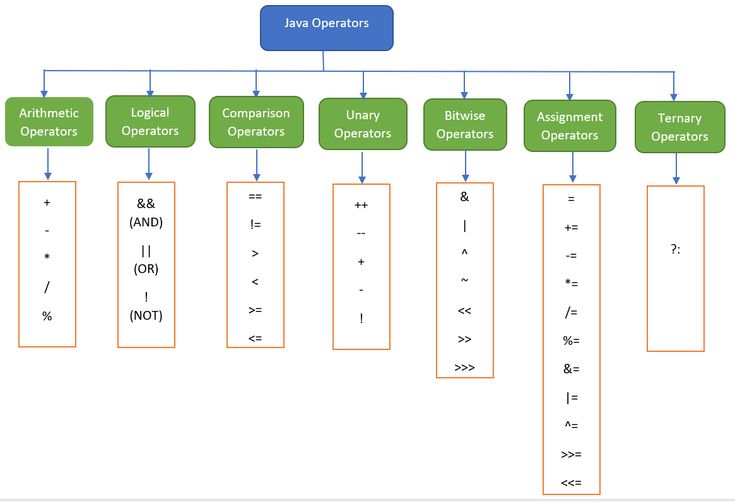
Just like in life, in Java, the right operator at the right time makes all the difference.
Operators in Java are one of the fundamental building blocks for any program. They allow us to perform various operations on variables and values, helping us manipulate data, make decisions, and more. Let’s dive into the different types of operators in Java, with relatable, real-world examples to help solidify our understanding.
1.Arithmetic Operators :
Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication, and division.
Operators:
+ (Addition)
- (Subtraction)
* (Multiplication)
/ (Division)
% (Modulus)
Real-World Example: Imagine you are shopping online, and you want to calculate the total cost of your items.
int item1 = 100; // Cost of first item
int item2 = 150; // Cost of second item
int item3 = 200; // Cost of third item
// Total cost
int totalCost = item1 + item2 + item3;
System.out.println("Total Cost: " + totalCost);
If you apply a discount, you can use the - operator, and if you want to find out the cost per item in a pack of 5, you could use the / operator:
int totalItems = 5;
int costPerItem = totalCost / totalItems;
System.out.println("Cost per item: " + costPerItem);
2. Assignment Operators :
Assignment operators are used to assign values to variables.
Operators:
\= (Simple assignment)
+= (Add and assign)
-= (Subtract and assign)
*= (Multiply and assign)
/= (Divide and assign)
Real-World Example:
Let’s say you’re managing a points system in a game. Players can earn points with each action.
int points = 0; // Initial points
// Player earns 10 points
points += 10;
System.out.println("Points after earning: " + points);
// Player uses 5 points to unlock an item
points -= 5;
System.out.println("Points after spending: " + points);
This way, you can easily update the player’s points balance.
- Relational (Comparison) Operators*
These operators are used to compare two values. They return a boolean (true or false).
Operators:
\== (Equal to)
!= (Not equal to)
\> (Greater than)
< (Less than)
\>= (Greater than or equal to)
<= (Less than or equal to)
Real-World Example:
Imagine you are building a system to check eligibility for a discount based on a customer’s age.
int customerAge = 18;
int discountAge = 18;
if (customerAge >= discountAge) {
System.out.println("Eligible for a discount!");
} else {
System.out.println("Not eligible for a discount.");
}
Here, you use the >= operator to check if the customer is old enough to get a discount.
- Logical Operator :
Logical operators are used to combine multiple conditions.
Operators:
- && (Logical AND) :
If Both the Condition are true then the && operator will return true. Let’s consider 1 is true and 0 is false . “&& “ operator will return the value according to the below table.
A | B | A && B |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
|| (Logical OR) :
If any one condition is true then ‘ || ‘ (OR) operator will return true./
OR Table :
A | B | A | B | |
0 | 0 | 0 | ||
0 | 1 | 1 | ||
1 | 0 | 1 | ||
1 | 1 | 1 |
! (Logical NOT) :
It will return the opposite value of the operand.
A | !A |
0 | 1 |
1 | 0 |
Real-World Example:
Imagine you’re designing an online voting system, where a user can vote if they’re both a registered voter and their age is 18 or above.
boolean isRegisteredVoter = true;
int voterAge = 20;
if (isRegisteredVoter && voterAge >= 18) {
System.out.println("You are eligible to vote.");
} else {
System.out.println("You are not eligible to vote.");
}
Here, both conditions must be true for the voter to be eligible.
- Increment and Decrement Operators*
These operators are used to increase or decrease the value of a variable by 1.
Operators:
++ (Increment)
-- (Decrement)
Real-World Example:
Think about a counter in a turn-based game. Each time a player makes a move, the turn counter should increment.
int turnCounter = 1;
System.out.println("Current Turn: " + turnCounter);
turnCounter++; // Next turn
System.out.println("Next Turn: " + turnCounter);
- Bitwise Operators*
Bitwise operators perform operations on binary representations of numbers.
Operators:
& (Bitwise AND)
| (Bitwise OR)
^ (Bitwise XOR)
~ (Bitwise NOT)
<< (Left Shift)
\>>(Right Shift)
Real-World Example:
Bitwise operations are often used in systems that require efficient storage, like compression algorithms or graphics rendering. Imagine a scenario where you want to turn on specific bits in a control system.
int controlBits = 0b0001; // Initial control (only one bit is ON)
// Enable another feature by using bitwise OR
controlBits = controlBits | 0b0010;
System.out.println("Control bits after enabling feature: " + Integer.toBinaryString(controlBits));
- Ternary Operator*
The ternary operator is a shorthand for if-else statements.
Operator:
- ?: (Condition ? True : False)
Real-World Example:
Let’s say you want to check if a customer’s order qualifies for free shipping.
int orderAmount = 550;
int freeShippingThreshold = 500;
String shippingStatus = (orderAmount >= freeShippingThreshold) ? "Free Shipping" : "Shipping Charge Applied";
System.out.println("Order Status: " + shippingStatus);
Simple Calculator Using Java Operators :
We all know about calculator since our childhood and also used it in so many situations whether it is required to calculate the total amount of money that has been collected by each student of class for the picnic or some school events like festivals.
Sometimes we often uses calculator to calculate our expenses during shopping and in many cases we have used calculator . But have you ever wonder how it is working internally ?? Let’s make out own calculator using java operators which will help us calculate the simple operations .
To create a calculator we can use the Scanner class to take input from user .Don’t be worry about the Scanner class we will discuss more about it in future blogs. For now just know that it is used to take values from the user in command line.
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args) {
// Create a scanner object for user input
Scanner scanner = new Scanner(System.in);
// Ask the user for two numbers
System.out.println("Enter the first number: ");
double num1 = scanner.nextDouble();
System.out.println("Enter the second number: ");
double num2 = scanner.nextDouble();
// Ask the user to select an operator
System.out.println("Choose an operation (+, -, *, /): ");
char operator = scanner.next().charAt(0);
// Variable to store the result
double result = 0;
// Perform the operation based on the operator
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
// Check if the second number is not zero to avoid division by zero
if (num2 != 0) {
result = num1 / num2;
} else {
System.out.println("Error: Division by zero is not allowed.");
return; // Exit the program
}
break;
default:
System.out.println("Invalid operator! Please use one of +, -, *, /.");
return; // Exit the program
}
// Display the result
System.out.println("The result is: " + result);
}
}
When we run the above code it will work like a calculator to perform operations like “ +,-,*,/,% “ .After running this code it will ask to enter the first number and then it will ask to enter the operation which you want to perform and then the second number and after that it will calculate the result .
With this simple calculator you now have a basic understanding about how arithmetic operators work and now you can extend the features of the calculator.
So try exploring the things .The more you explore the more you will know.
Conclusion :
Operators are the backbone of logic in Java, enabling us to perform computations, make decisions, and manage data effectively. Whether you’re adding up items in a shopping cart, evaluating a user’s age for a discount, or managing game points, operators play a critical role in how applications function.
By understanding and mastering these operators, you will have the tools you need to solve everyday programming challenges efficiently.
I hope this blog helped you understand the basics of operators in Java and how to build a simple calculator. Remember, learning is a journey, and there’s always something new to explore. Don’t be afraid to dive deeper, experiment with more complex problems, and push your limits.
If you have any doubts or questions, feel free to reach out—I’d be happy to help! I’d also love to hear your suggestions or feedback, so don’t hesitate to leave a comment below.
And if you enjoyed this post, make sure to share it with others who might find it useful, and subscribe to the newsletter to stay updated on the latest posts and learn more exciting topics.
Happy coding, and keep exploring!
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.