Understanding String Concatenation in Java
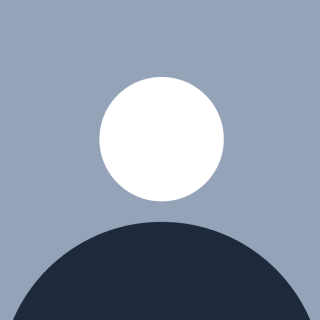
This is super basic, and you might be thinking, "Why does this article even exist? Who doesn’t know string concatenation, right?" But let me tell you something: you might know string concatenation, but as a beginner, when you're writing code, working on large codebases, and solving real-world problems, you won't realize how crucial it is to use these methods effectively. Knowing when to use what can make a huge difference in optimizing your code, saving memory, and cutting down runtime.
And that’s exactly what we’re diving into today.
P.S. – I’ll keep things straightforward and to the point. No fancy language, just simple explanations to make it easy for you to follow along.
How will this make a difference? It’s all about performance and efficiency.
Let’s get started:
String Concatenation Using
+
:
Suppose you have to concatenate one string 6 times and store that concatenated string for further use. You might write the following code:
String str = "u";
String concatenatedStr = "";
for(int i = 0; i < 6; i++){
concatenatedStr += str;
}
How does the above code work?
Every time you use +=
to add str
to concatenatedStr
, a new string is created since strings are immutable in Java (i.e., once created, they cannot be changed). So in the above code, for each iteration of the loop, a new string object is created, and the contents of both the original string and the new part are copied into it.
How's it going to impact performance?
The repeated creation of new string objects in each iteration is inefficient, especially when it comes to longer strings or larger loops. This results in increased time complexity due to the repeated copying of strings.
String Concatenation Using
StringBuilder
:
Let’s rewrite above same code using StringBuilder
class:
String str = "u";
StringBuilder concatenatedStr = new StringBuilder();
for(int i = 0; i < 6 ; i++){
concatenatedStr.append(str);
}
How does the above code work?
StringBuilder
is a mutable sequence of characters. So, instead of creating a new string each time a concatenation is performed, it appends the new string to the existing one, hence modifying it in place.
How's it going to impact performance?
This approach is much more efficient than +
ones, as it doesn’t create a new string object everytime it appends. So it grows dynamically as needed without the overhead of copying strings multiple times.
Summary:
We are almost at end, let’s quickly summarise and compare performance impact, the main thing we want to see and talk about:
Using +
operator: Every string concatenation creates a new string, which isn’t efficient and may impact performance & runtime. It consumes more memory and can lead to more frequent Garbage Collection.
Using StringBuilder
: This approach handles strings more efficiently, resulting in better performance and runtime. It uses memory more efficiently by avoiding creation of numerous intermediate string objects and modifying string in place.
Hence, using StringBuilder
is more optimised for time and space, especially when dealing with larger strings or too many concatenations. It reduces overhead associated with Inefficient String Concatenation in Java, making your code more efficient overall.
So you learned how to handle strings better. Try solving this problem now and handle strings better:
LeetCode Problem: Repeated Substring Pattern
I hope this helps you! See you soon with another one!!
Subscribe to my newsletter
Read articles from Isha Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Isha Gupta
Isha Gupta
Wanna learn together? Let's catch up on Twitter (X)!