Handling Dynamic Content and Complex Interactions with Playwright 🎭
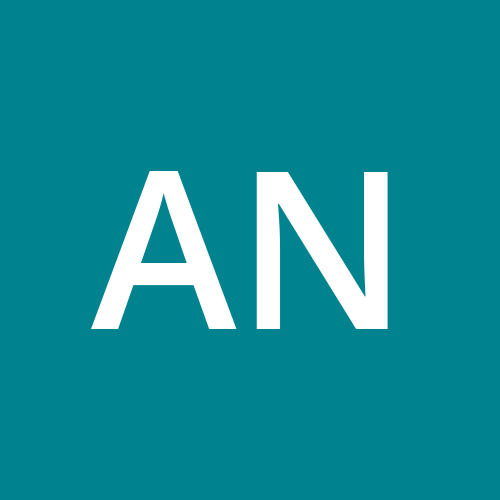
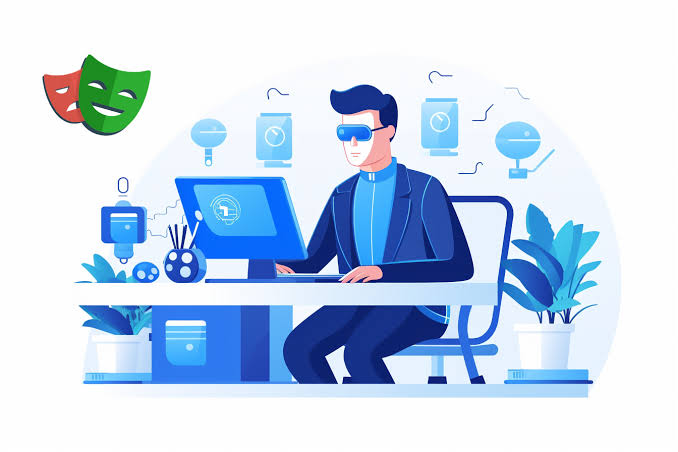
When working with web automation, dynamic content and complex interactions often pose challenges ⏳. Web elements may load late, or interactions like drag-and-drop, hover, or complex form submissions require careful handling. Playwright is a fantastic tool that provides solutions to these challenges while ensuring stable and fast automation. 🎯
In this blog, we'll explore strategies to handle dynamic content, complex interactions, and the various locator strategies Playwright offers 🚀.
1️⃣ Locating Elements Using getByTestId
🆔
Using data-testid
attributes to identify elements is a common practice when writing UI tests. Playwright provides a getByTestId
locator method to make this easier:
// Locate element using test ID
const submitButton = await page.getByTestId('submit-button');
await submitButton.click();
This approach makes your tests more reliable by avoiding brittle locators that can change often, like class names. 👍
2️⃣ Locating Elements by Role with getByRole
🎭
Elements can be located by their ARIA roles to ensure your tests are accessible-friendly. getByRole
is a great way to interact with buttons, links, or other ARIA-compliant elements:
// Locate a button by its role
const loginButton = await page.getByRole('button', { name: 'Log In' });
await loginButton.click();
This method ensures your automation interacts with elements as a user would perceive them 👀.
3️⃣ Waiting for Elements to be Visible 🕵️♂️
Dynamic content often appears after some delay ⏱️. You can use Playwright’s built-in waiting mechanism to ensure that your test waits for the element to be visible before interacting with it.
// Wait for the button to become visible and then click it
await page.waitForSelector('button#submit', { state: 'visible' });
await page.click('button#submit');
Playwright automatically waits for the element to appear and be interactable before clicking. No more race conditions! 🎉
4️⃣ Locating Elements with Text Using getByText
📜
Sometimes, the simplest way to locate an element is by its text content 📝. Playwright's getByText
makes it easy to find elements with specific text:
// Locate a button by its text
const nextButton = await page.getByText('Next');
await nextButton.click();
This is particularly useful for interacting with elements that don’t have unique IDs or easily accessible attributes. 🔍
5️⃣ Handling Complex Interactions 🧩
Certain user interactions, like drag-and-drop or hover, can be tricky to automate 💡. Playwright handles these with built-in methods that simplify such actions.
Let’s look at a drag-and-drop example:
// Drag an element from source to target
await page.dragAndDrop('#source', '#target');
That’s it! 🎯 The action is seamless and mimics how a real user would perform the task.
6️⃣ Handling Asynchronous Content Updates ⏳
Sometimes the content on a page updates asynchronously after the initial page load 🔄. For instance, a search result might populate after you enter a query. You can use waitForResponse
or waitForFunction
to handle such cases.
// Wait for the API call to return search results
await page.waitForResponse(response =>
response.url().includes('search') && response.status() === 200
);
With this strategy, you ensure that your test waits until the dynamic data is fully loaded before proceeding. ✅
7️⃣ Retries for Flaky Elements 🔁
Even with robust waiting mechanisms, there might be times when an element isn’t consistently interactable due to network or server issues. Here’s a retry pattern you can implement:
async function retryClick(selector: string, retries = 3) {
for (let i = 0; i < retries; i++) {
try {
await page.click(selector);
break; // Success! Exit loop
} catch (e) {
if (i === retries - 1) throw e; // If out of retries, throw error
}
}
}
await retryClick('button#retry');
This ensures that intermittent failures don’t break your tests, adding a layer of reliability 🛡️.
8️⃣ Managing Timeouts Efficiently ⏲️
Handling long waits or ensuring that certain tasks complete within a reasonable time is essential. Setting timeouts for specific actions can keep your tests from hanging too long ⌛:
// Click with a custom timeout
await page.click('button#confirm', { timeout: 5000 }); // 5 seconds timeout
Efficient use of timeouts ensures that your tests run smoothly even when the application is slow 🏃♂️.
9️⃣ Simulating Keyboard Input 🎹
Complex interactions like form-filling often require simulating keyboard input. Playwright makes this straightforward, too:
// Type into a search box with a delay between keystrokes
await page.type('#search', 'Playwright Automation', { delay: 100 }); // 100ms delay between each keystroke
Adding a delay mimics real typing, making your test more realistic 👩💻.
🎯 Handling dynamic content and complex interactions in web automation may seem daunting at first 🤔, but Playwright offers powerful features and a variety of locator strategies like getByTestId
, getByRole
, and getByText
to make this process much simpler 🔨. From handling asynchronous loading to simulating complex user interactions, Playwright is your best friend in creating robust and reliable automated tests. 🎉
Stay tuned for more Playwright tips and tricks! 🚀
Subscribe to my newsletter
Read articles from Anandkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
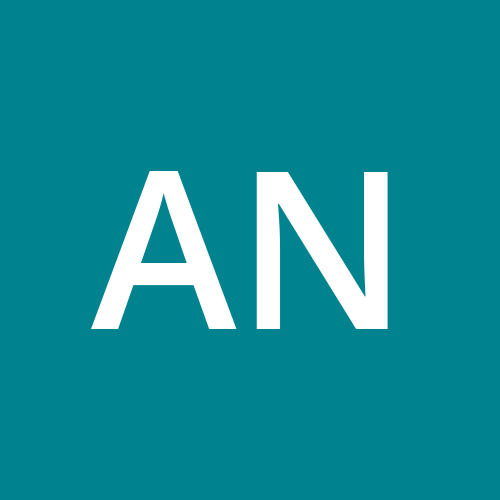
Anandkumar
Anandkumar
"Passionate playwright dedicated to refining scripts through innovative methods. Embracing automated testing to enhance creativity and efficiency in crafting compelling narratives. Let's revolutionize storytelling together!"