Binary Search - Leetcode 704 - Python Solution
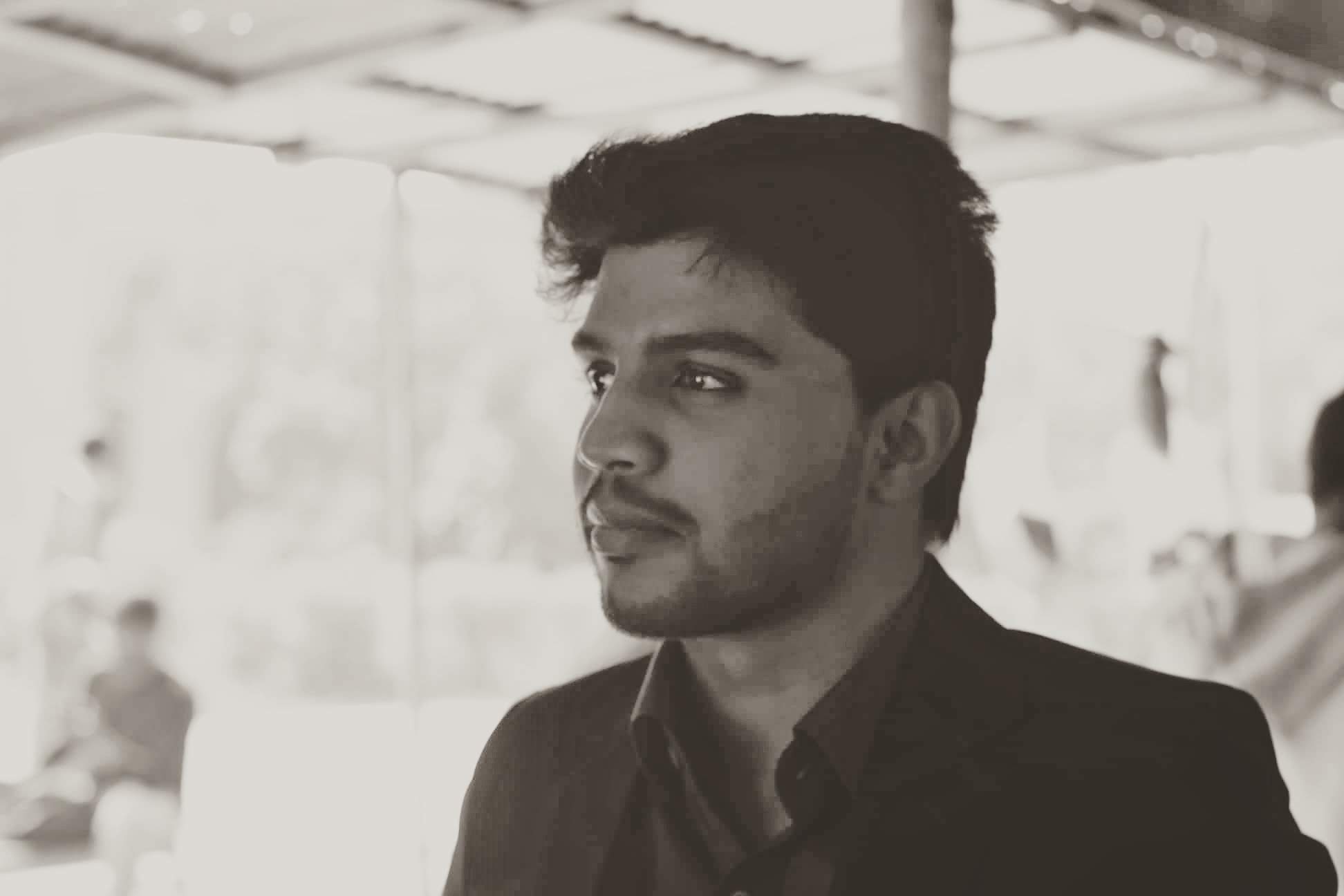
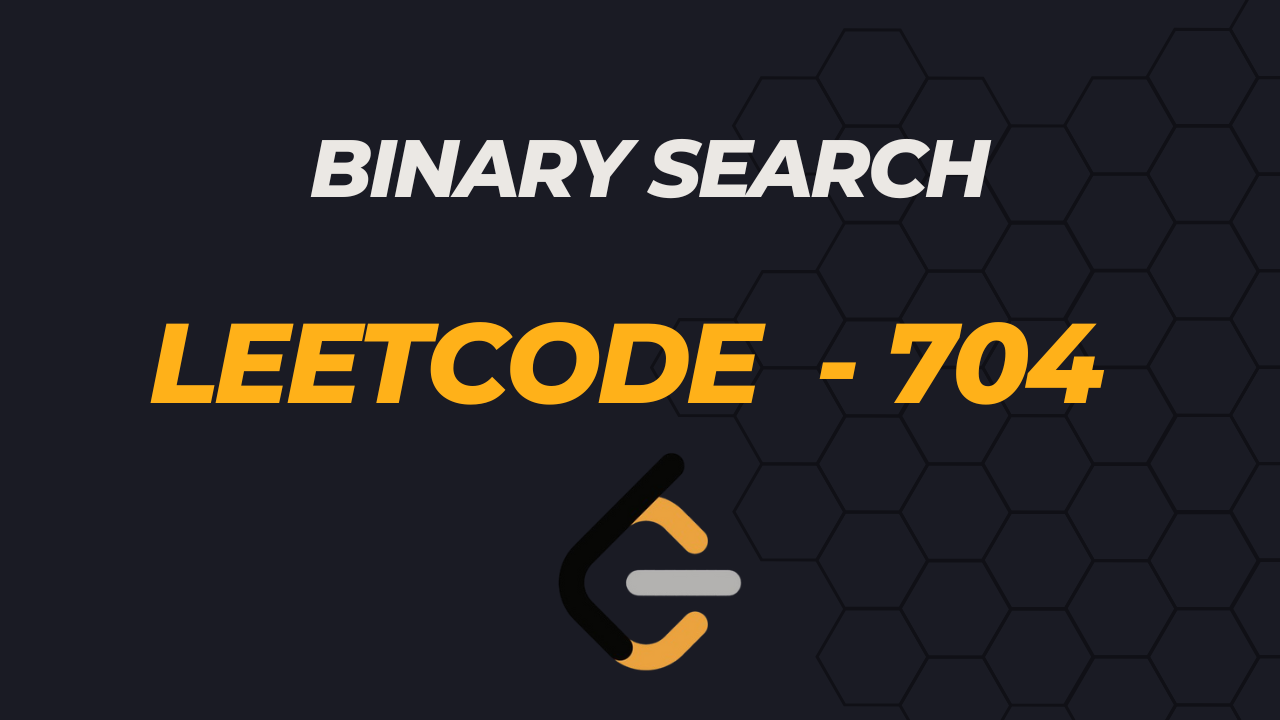
Hey Everyone, today we will solve how to find a number in a sorted array according to its target
using Binary Search.
Step-1
Initialize the start
pointer at the beginning of the array and the end
pointer at the end of the array.
def binary_search(nums, target):
start = 0
end = len(nums) - 1
Step - 2
Traverse the array and Calculate the mid
point of the array.
while start <= end:
mid = (start + end) // 2
Step-3
If the
target
is equal to, return the mid index.If the
target
is greater than, we'll need to search in the right portion by increasing thestart
pointer.If the
target
is less thannums[mid]
, we'll search in the left portion by decreasing theend
pointer.
if target == nums[mid]:
return mid
elif target < nums[mid]:
end = mid - 1
else:
start = mid + 1
return -1 # Target not found
Full Code
def binary_search(nums, target):
start = 0
end = len(nums) - 1
while start <= end:
mid = (start + end) // 2
if target == nums[mid]:
return mid
elif target < nums[mid]:
end = mid - 1
else:
start = mid + 1
return -1 # Target not found
Video Explanation
Thanks, Everyone hanging in there till the last ❤️ and I'll see you in the next one!!
Subscribe to my newsletter
Read articles from Shojib directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
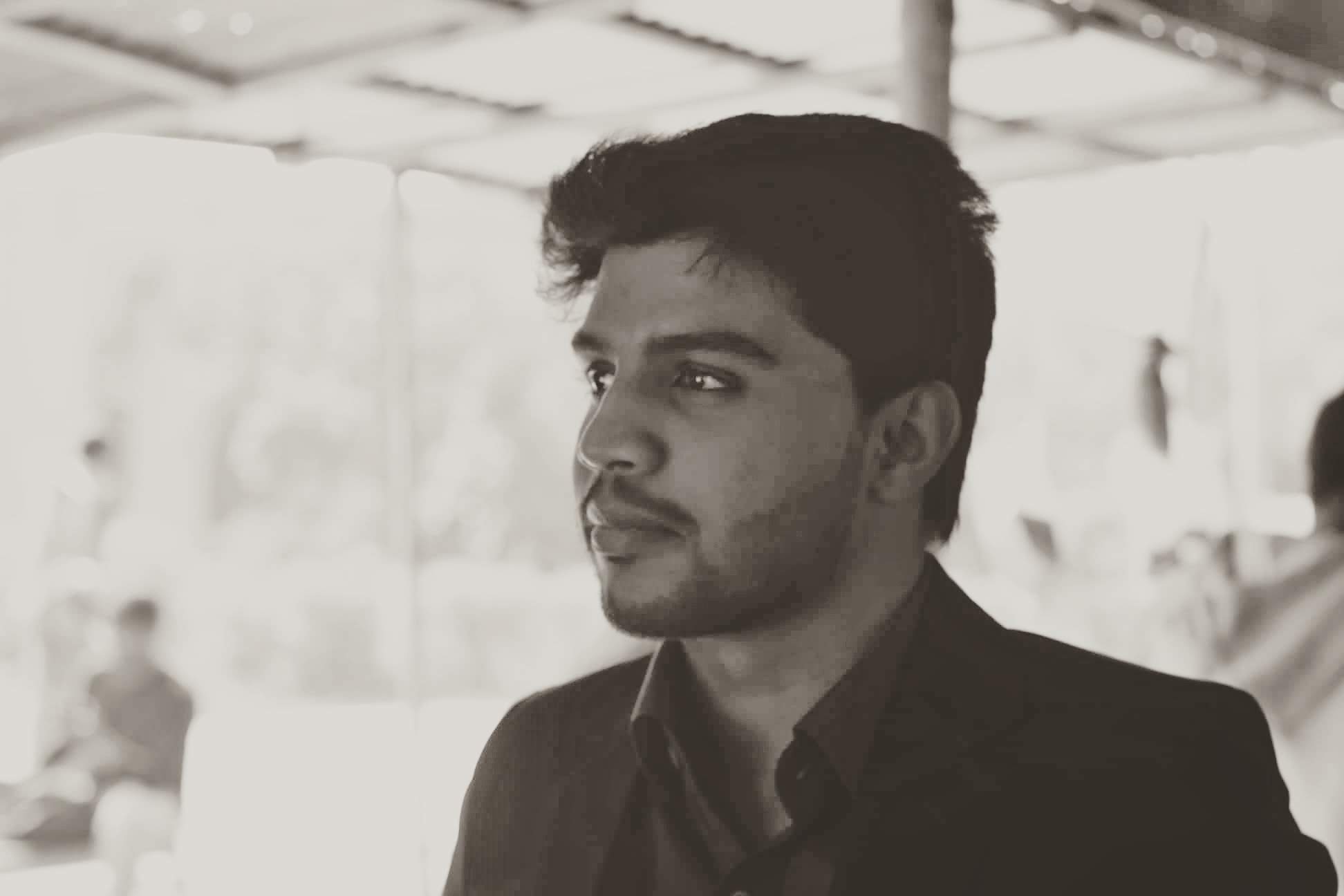
Shojib
Shojib
Hey, I'm Kamruzaman Shojib and I'm a front-end developer. I love to talk about tech mostly I'll be writing about JavaScript and trying to help the community.