Creating Interactive Dashboards with Python and Plotly Dash
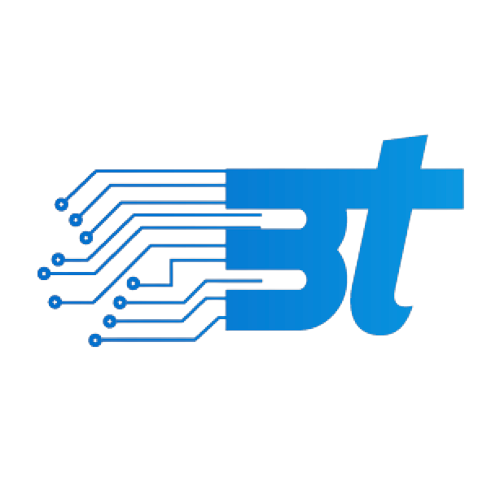
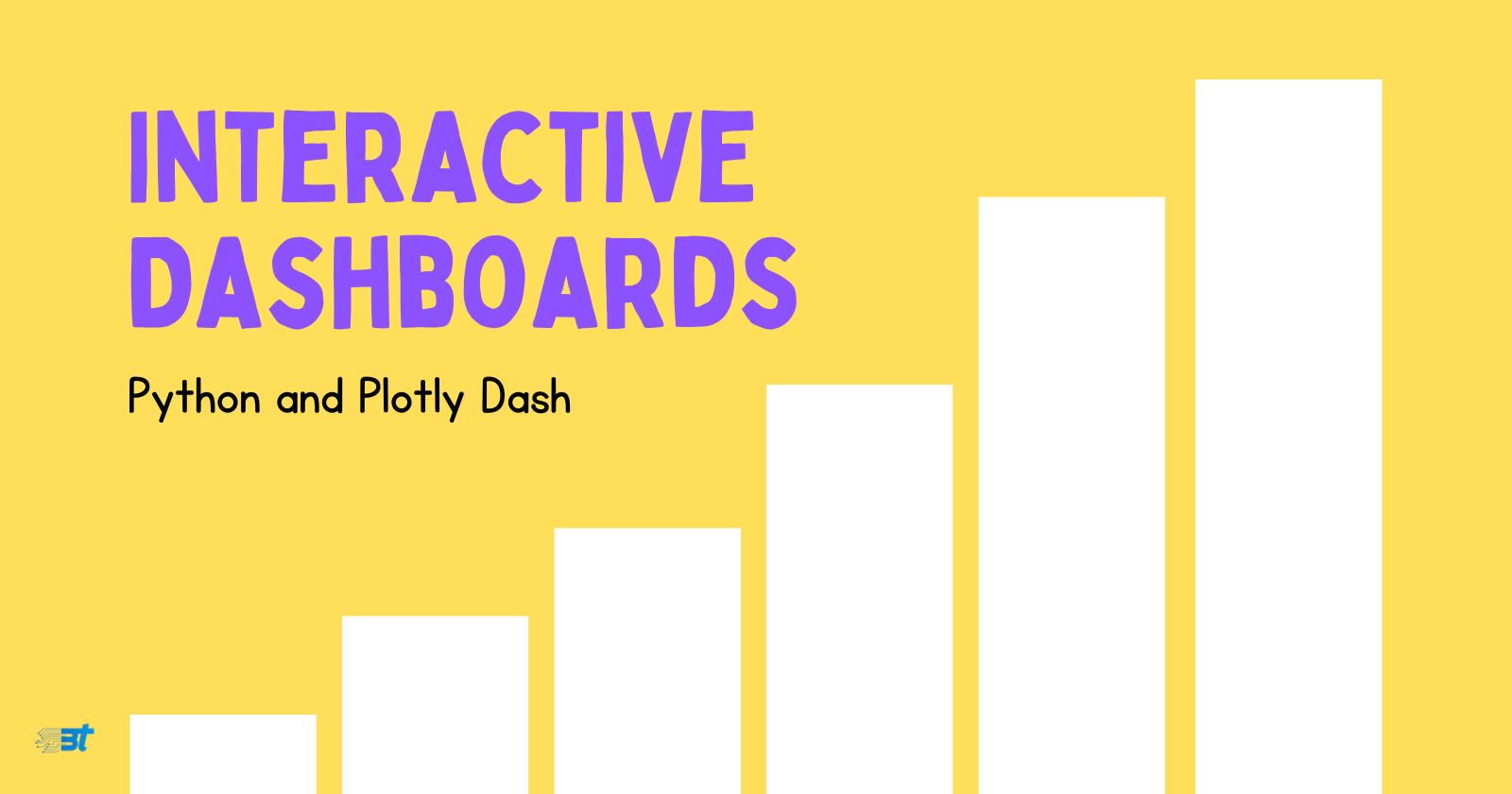
In today's data-driven world, visualizing data in a dynamic and interactive way is crucial for better understanding and decision-making. Python, combined with Plotly Dash, provides a powerful way to create interactive, responsive, and visually appealing dashboards. Plotly Dash is an open-source framework that allows you to build web-based analytical applications using Python without requiring expertise in web development.
In this blog, we'll explore how you can use Python and Plotly Dash to create interactive dashboards, integrate various components, and deploy the final product.
Why Plotly Dash?
Plotly Dash is ideal for developing interactive web applications that provide real-time data insights. Some of the benefits of using Plotly Dash include:
Python-Powered: Dash applications are written entirely in Python. It integrates seamlessly with the Python data science ecosystem.
Rich Ecosystem: It provides built-in support for graphs, tables, and HTML components with high customization options.
Interactive Visuals: Create highly interactive charts and visualizations with Plotly’s powerful graphing capabilities.
Extensible and Scalable: Dash allows for easy integration with web-based frameworks and can be scaled for production applications.
Now, let’s dive into how to create a basic interactive dashboard.
Step 1: Installing Dependencies
First, you’ll need to install Dash and Plotly. If you don’t have them installed, you can easily add them using pip
.
pip install dash plotly
Step 2: Understanding the Basics of Dash
Dash applications consist of two parts:
Layout: Defines the structure of the dashboard (what it looks like).
Callbacks: Adds interactivity to the dashboard (how it behaves).
The basic structure of a Dash app looks like this:
import dash
from dash import dcc, html
from dash.dependencies import Input, Output
app = dash.Dash(__name__)
app.layout = html.Div([
html.H1("Interactive Dashboard with Plotly Dash"),
dcc.Dropdown(
id='dropdown',
options=[
{'label': 'Option 1', 'value': '1'},
{'label': 'Option 2', 'value': '2'}
],
value='1'
),
html.Div(id='output-container')
])
@app.callback(
Output('output-container', 'children'),
Input('dropdown', 'value')
)
def update_output(value):
return f'You have selected {value}'
if __name__ == '__main__':
app.run_server(debug=True)
In this basic structure:
We define a
layout
usinghtml.Div
to structure the dashboard.dcc.Dropdown
is used to create a dropdown component.A callback connects the dropdown to a display
Div
to show the selected value.
Step 3: Adding Interactive Graphs
To make things more interesting, let’s add a graph to the dashboard that updates based on the dropdown selection. We’ll use Plotly’s graphing capabilities to create this graph.
import plotly.express as px
# Sample Data
df = px.data.iris()
app.layout = html.Div([
dcc.Dropdown(
id='dropdown',
options=[{'label': col, 'value': col} for col in df.columns if col != 'species'],
value='sepal_width'
),
dcc.Graph(id='graph')
])
@app.callback(
Output('graph', 'figure'),
Input('dropdown', 'value')
)
def update_graph(selected_column):
fig = px.histogram(df, x=selected_column, color="species", barmode="overlay")
return fig
In this code:
Plotly Express (
px
) is used to create a histogram plot.The graph updates dynamically based on the column selected in the dropdown.
dcc.Graph is used to embed the Plotly chart within the layout.
Step 4: Adding Multiple Components
Dash applications can support multiple components and callbacks. Let’s extend our dashboard by adding more controls such as sliders, checkboxes, or date pickers to refine the data being plotted.
app.layout = html.Div([
html.H1("Iris Dataset Analysis"),
dcc.Dropdown(
id='dropdown',
options=[{'label': col, 'value': col} for col in df.columns if col != 'species'],
value='sepal_width'
),
dcc.Slider(
id='slider',
min=1,
max=10,
value=5,
marks={i: str(i) for i in range(1, 11)}
),
dcc.Graph(id='graph')
])
@app.callback(
Output('graph', 'figure'),
[Input('dropdown', 'value'), Input('slider', 'value')]
)
def update_graph(selected_column, slider_value):
filtered_df = df[df[selected_column] < slider_value]
fig = px.scatter(filtered_df, x=selected_column, y='sepal_length', color='species')
return fig
Here:
We introduce a
dcc.Slider
to filter the data before plotting.The
update_graph
function now takes two inputs:dropdown
andslider
, making the graph interactive with multiple controls.
Step 5: Styling Your Dashboard
Dash allows you to easily style your applications using external CSS stylesheets. You can import a CSS file or define inline styles using the style
attribute.
app.layout = html.Div([
html.H1("Styled Dashboard", style={'textAlign': 'center', 'color': '#7FDBFF'}),
dcc.Graph(id='graph', style={'border': '1px solid #000'})
])
You can use popular CSS frameworks such as Bootstrap or custom CSS files to create professional-looking dashboards.
Step 6: Deploying Your Dash App
Once your dashboard is ready, deploying it to a cloud platform like Heroku is straightforward. Here’s a basic process:
Create a
Procfile
: This file specifies how the app will run.web: gunicorn app:server
Install Gunicorn:
pip install gunicorn
Push to Heroku:
Follow Heroku’s instructions to push your code to their servers and start your Dash app.
Examples of Use Cases
Stock Market Dashboard: Create interactive dashboards to analyze stock performance over time using sliders for date ranges and dropdowns to select different stocks or financial indicators.
Customer Analytics Dashboard: Visualize customer data, track metrics like sales growth, customer retention, and demographic breakdowns, and provide the ability to filter by regions, products, and timeframes.
COVID-19 Data Tracker: Build a dashboard to display live COVID-19 case data by region, vaccination rates, and other relevant statistics. Users can interact with maps and charts to explore trends.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
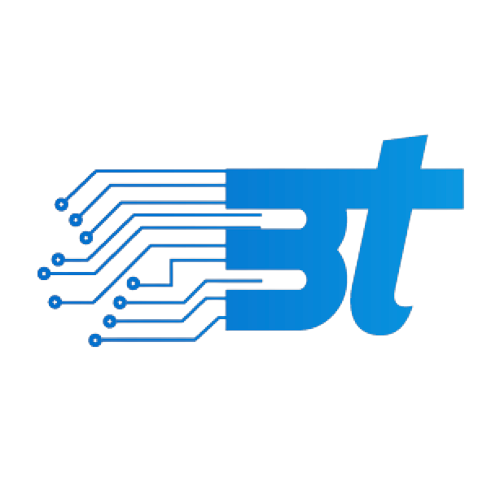
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.