Mastering Arrays in Java : The Key to Efficient Data Management .

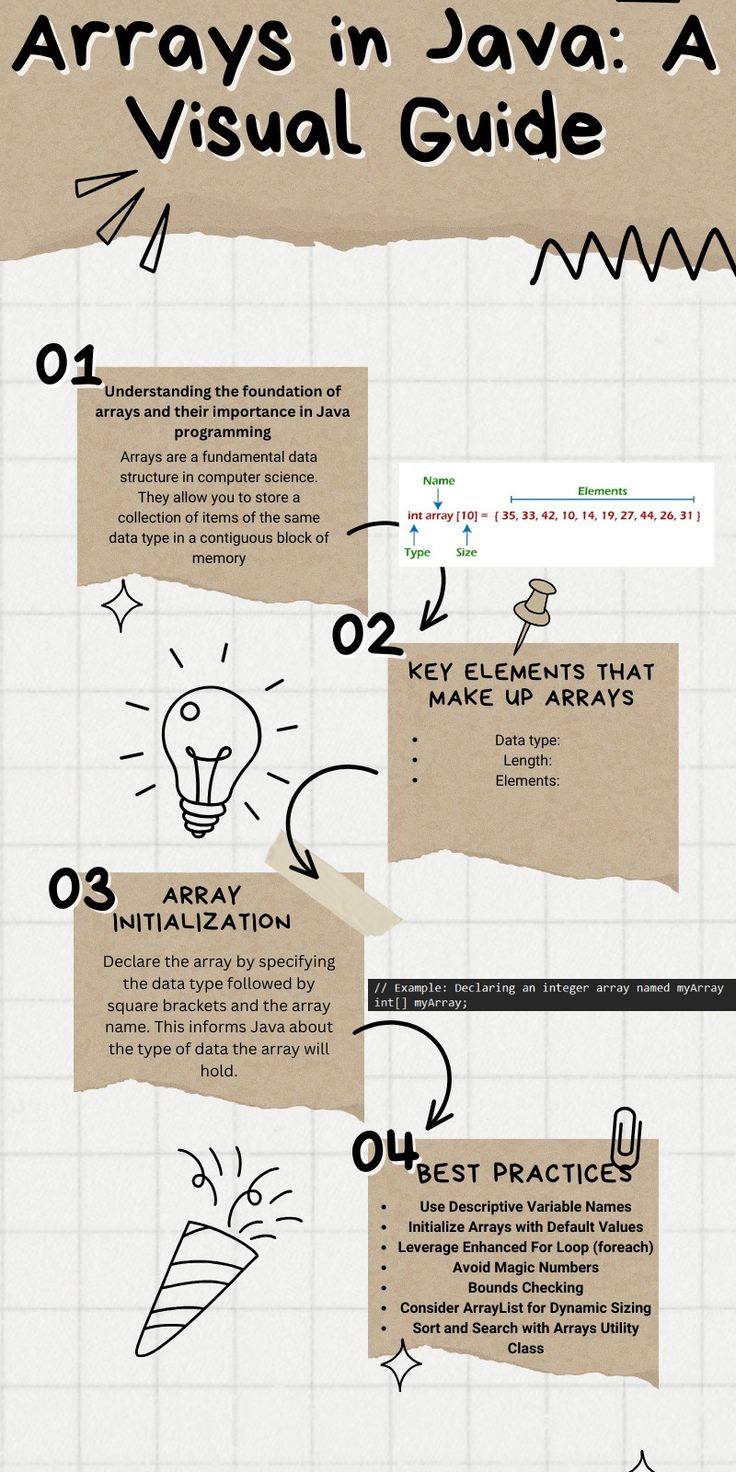
Why Array ?
Before understanding what an array is, let’s look at the problems we face in data management. When you first learn programming, you often store data using individual variables of primitive types like int, float, char, etc. But what happens if you need to store and manage a large amount of similar data, such as the scores of 100 students?
Creating 100 separate variables (score1, score2, ..., score100) is not only impractical but also makes the code hard to manage and inefficient. This is where arrays come in. So let’s understand what an array is in depth.
What is an Array ?
An array is a data structure that lets you store multiple values of the same type in a single variable. Instead of declaring a new variable for each value, you can use one array to store them all and easily access each value using its index. Let's discuss it in more detail:
Imagine a library with many books, where you want to store books of the same genre, like "science fiction." If you stored each science fiction book in separate boxes scattered across the library (like individual variables), finding a specific book would be difficult and take up a lot of space.
Instead, you can use a single shelf (like an array) with designated slots (indices) to store all the science fiction books together. The books are placed in a neat, organized manner, side by side, and you can quickly locate any book by knowing its position on the shelf (its index).
Definition :
An array is a collection of elements, all of the same type, stored at contiguous memory locations. Each element can be accessed via an index number.
Key Features of Array :
Fixed Size: Arrays have a predefined size, meaning they cannot dynamically resize.
Indexing: Elements in an array are accessed via indices, starting from 0.
Contiguous Memory: Elements are stored in adjacent memory locations, allowing for fast access and iteration.
Homogeneous Data: All elements in an array must be of the same type (e.g., integers, characters).
Let’s discuss how can we define an array in many ways :
Syntax :
Declaration :
dataType[] arrayName;
Declaration: dataType[] arrayName;
Instantiation (Allocating memory): arrayName = new dataType[size];
Declaration and Instantiation together: java dataType[] arrayName = new dataType[size];
Initialization (Assigning values): arrayName[index] = value;
Example :
// Declaration and instantiation
int[] numbers = new int[5];
// Initialization
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
numbers[3] = 40;
numbers[4] = 50;
You can also declare, instantiate, and initialize an array in one line:
java int[] numbers = {10, 20, 30, 40, 50};
Types Of Array :
Single-Dimensional Arrays:
A simple list of elements (like a row of values).
Example: int[] numbers = {1, 2, 3, 4};
Multi-Dimensional Arrays:
Arrays with more than one row or column (like a table of values).
Example: int[][] matrix = {{1, 2}, {3, 4}}; (2D array)
Advantages of Using Arrays :
Efficient Memory Management: Arrays help store multiple elements in contiguous memory, making it easier and faster to access the data.
Easy Data Access: You can access any element in the array using its index, like arrayName[index].
Better Code Management: Using arrays, you can write cleaner, more readable, and organized code instead of managing hundreds of variables.
Data Traversal: Arrays allow you to efficiently traverse through data using loops, which makes processing large datasets easier.
Every thing has it’s advantages and it’s disadvantages .We have discussed the advantages of array and now let’s discuss about it’s disadvantages .
Disadvantages of Array :
Fixed Size: Once an array is created, its size is fixed. You can't change the number of elements later.
Inefficient Insertion/Deletion: Inserting or deleting elements in an array can be inefficient since elements may need to be shifted to maintain order.
Homogeneous Data: Arrays can only store elements of the same type, so you can't mix different types of data in one array.
Let’s Discuss more about array with the some example :
This example shows how to create an array, access its elements, modify it, and print the values.
public class ArrayExample {
public static void main(String[] args) {
// 1. Declaration and Initialization
int[] numbers = new int[5]; // An array of size 5
// 2. Inserting values into the array
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
numbers[3] = 40;
numbers[4] = 50;
// 3. Accessing elements from the array
System.out.println("First element: " + numbers[0]); // Output: 10
System.out.println("Last element: " + numbers[4]); // Output: 50
// 4. Modifying an element
numbers[2] = 35; // Changing the third element from 30 to 35
System.out.println("Modified third element: " + numbers[2]); // Output: 35
// 5. Iterating through the array
System.out.println("Array elements:");
for (int i = 0; i < numbers.length; i++) {
System.out.print(numbers[i] + " "); // Output: 10 20 35 40 50
}
}
}
Let’s understand how we can store the daily temperatures of a week and calculate the average!
We will create an array to store the daily temperatures and then calculate the average by fetching all the recorded temperatures of the week.
First, we need to decide what type of array to create.
Since the temperature values are floating-point numbers, we need an array that can store floating-point types.
Next, we need to create an array and decide its size. Since we are storing the daily temperatures of a week, we need an array of size 7.
Then, we will fetch all the elements of the array, add all the temperatures, and divide by 7 to calculate the average.
So let’s code it step by step:
public class TemperatureTracker {
public static void main(String[] args) {
// An array to store temperatures for 7 days
double[] temperatures = {72.5, 75.0, 71.3, 69.8, 68.5, 70.0, 74.6};
// Calculate the average temperature of the week
double totalTemperature = 0;
for (int i = 0; i < temperatures.length; i++) {
totalTemperature += temperatures[i];
}
double averageTemperature = totalTemperature / temperatures.length;
// Display the result
System.out.println("Average Temperature for the week: " + averageTemperature);
}
}
Conclusion:
In this blog, we've explored the fundamental concept of arrays, understanding what they are, how to create and use them, and their various types and features. Arrays serve as a foundational data structure in programming due to their simplicity and efficiency in handling multiple elements of the same type. While they offer quick access to elements via indexing and are easy to implement, they also have limitations like fixed size and lack of dynamic resizing.
We've also touched on some practical applications of arrays by solving problems like the temperature tracker, which demonstrates the utility of arrays in real-world scenarios.
Final Message:
Arrays are a basic but essential part of any programmer's toolkit. Mastering them will provide a solid foundation for learning more complex data structures and algorithms. Keep practicing and applying these concepts to real-world problems. The more you experiment with different use cases, the better you will become at handling arrays and other data structures. Remember, programming is a journey, and every problem you solve brings you one step closer to mastery!
Keep exploring facts about arrays and become a better coder. Happy coding!
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.