The Ultimate Guide to Mastering Laravel Macros for Developers
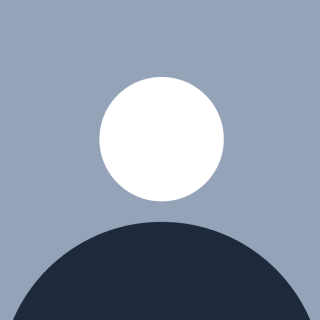
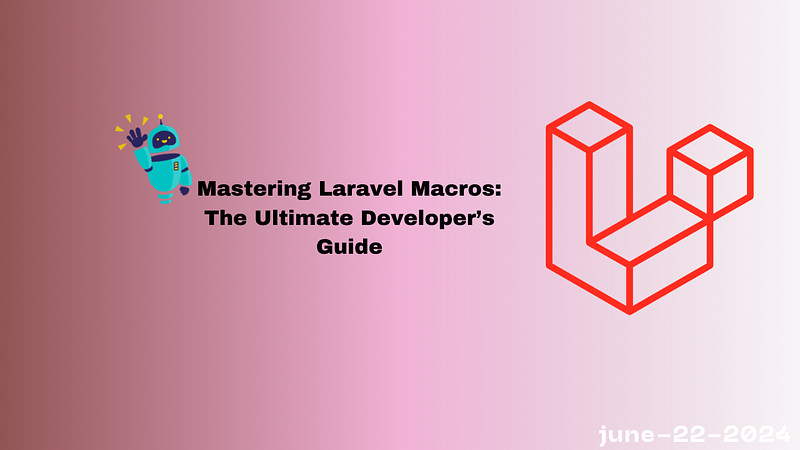
Introduction
Laravel macros are a hidden gem in the Laravel framework that can dramatically improve your development experience. What precisely are they, and why should you care? Let’s look at Laravel macros and how they can help your development process run more smoothly and efficiently.
What are Laravel Macros?
Laravel macros are essentially a technique to extend the functionality of existing Laravel classes. Consider them minor magic tricks that enhance the capabilities of Laravel’s core components without altering the source code.
Importance of Laravel Macros in Development
Macros are crucial because they allow developers to reuse code and create custom methods that can be applied globally across the application. This means less repetition and more streamlined, readable code.
Understanding the Macroable Trait
The Macroable trait is the backbone of Laravel Macros. It allows any class to register custom methods at runtime. This is done using the macro method
Basic Example of a Laravel Macro
Let’s dive into the concept of macros by using a practical example.
Overview of Macroable Classes in Laravel
Here are some of the key classes in Laravel that use the Macroable
trait:
Collection (
Illuminate\Support\Collection
)Request (
Illuminate\Http\Request
)Eloquent Builder (
Illuminate\Database\Eloquent\Builder
)Response (
Illuminate\Http\Response
)Route (
Illuminate\Support\Facades\Route
)Validator (
Illuminate\Support\Facades\Validator
)Cache (
Illuminate\Support\Facades\Cache
)Blade (
Illuminate\Support\Facades\Blade
)
Step-by-Step Guide
- Create the MacrosServiceProvider
First, create a service provider dedicated to macros:
php artisan make: provider MacrosServiceProvider
This Command will generate a new file named MacrosServiceProvider.php
in the app/Providers
directory.
Structure of the Generated Service Provider
<?php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
class MacrosServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
//
}
}
Namespace: The namespace declaration. App\Providers
specifies that this class is part of the Providers namespace, which is consistent with Laravel’s directory structure.
Class Definition: The MacrosServiceProvider
class inherits methods and attributes from Laravel’s ServiceProvider class.
Methods:
register(): This method is used to bind things to the service container. You can use this method to set up any services or bindings that your application requires.
Boot(): This function is used to start any application services. It is called after all other service providers have been registered, implying that you have access to all other services registered using the register method.
2. Define Macro Classes
Create a directory named Macros
inside the app
folder, so it will be app\Macros
. Then, create individual classes for each type of macro within this directory. For example:
- CollectionMacros
Create a file app/Macros/CollectionMacros.php
:
namespace App\Macros;
use Illuminate\Support\Collection;
class CollectionMacros
{
public static function register()
{
// Macro to convert all items in a collection to uppercase
Collection::macro('toUpper', function () {
return $this->map(function ($value) {
return strtoupper($value);
});
});
// Macro to calculate the average length of strings in a collection
Collection::macro('averageLength', function () {
return $this->map(function ($item) {
return strlen($item);
})->avg();
});
}
}
- RequestMacros
Create a file app/Macros/RequestMacros.php
:
namespace App\Macros;
use Illuminate\Http\Request;
class RequestMacros
{
public static function register()
{
// Macro to check if the authenticated user is an admin
Request::macro('isAdmin', function () {
return $this->user() && $this->user()->isAdmin();
});
// Macro to check if the request is an AJAX request
Request::macro('isAjax', function () {
return $this->ajax();
});
}
}
- BuilderMacros:
Create a file app/Macros/BuilderMacros.php
:
namespace App\Macros;
use Illuminate\Database\Eloquent\Builder;
class BuilderMacros
{
public static function register()
{
// Macro to scope query to only active records
Builder::macro('active', function () {
return $this->where('status', 'active');
});
// Macro to scope query to only recent records created in the last 30 days
Builder::macro('recent', function () {
return $this->where('created_at', '>=', now()->subDays(30));
});
}
}
- ResponseMacros
Create a file app/Macros/ResponseMacros.php
:
namespace App\Macros;
use Illuminate\Http\Response;
class ResponseMacros
{
public static function register()
{
// Macro to return a standardized JSON response for API
Response::macro('api', function ($data, $status = 200) {
return response()->json([
'data' => $data,
'status' => $status,
], $status);
});
}
}
- RouteMacros
Create a file app/Macros/RouteMacros.php
:
namespace App\Macros;
use Illuminate\Support\Facades\Route;
class RouteMacros
{
public static function register()
{
// Macro to define standard CRUD routes for a resource
Route::macro('crud', function ($name, $controller) {
Route::get("$name", [$controller, 'index'])->name("$name.index");
Route::get("$name/create", [$controller, 'create'])->name("$name.create");
Route::post("$name", [$controller, 'store'])->name("$name.store");
Route::get("$name/{id}", [$controller, 'show'])->name("$name.show");
Route::get("$name/{id}/edit", [$controller, 'edit'])->name("$name.edit");
Route::put("$name/{id}", [$controller, 'update'])->name("$name.update");
Route::delete("$name/{id}", [$controller, 'destroy'])->name("$name.destroy");
});
}
}
- ValidatorMacros
Create a file app/Macros/ValidatorMacros.php
:
namespace App\Macros;
use Illuminate\Support\Facades\Validator;
class ValidatorMacros
{
public static function register()
{
// Macro to add a UUID validation rule
Validator::extend('uuid', function ($attribute, $value, $parameters, $validator) {
return preg_match('/^\{?[0-9a-fA-F]{8}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{12}\}?$/', $value);
});
}
}
- CacheMacros
Create a file app/Macros/CacheMacros.php
:
namespace App\Macros;
use Illuminate\Support\Facades\Cache;
class CacheMacros
{
public static function register()
{
// Macro to remember a cache value once and return it
Cache::macro('rememberOnce', function ($key, $ttl, $callback) {
if (Cache::has($key)) {
return Cache::get($key);
}
$value = $callback();
Cache::put($key, $value, $ttl);
return $value;
});
}
}
- BladeMacros
Create a file app/Macros/BladeMacros.php
:
namespace App\Macros;
use Illuminate\Support\Facades\Blade;
class BladeMacros
{
public static function register()
{
// Macro to render a Blade directive to show the user's name
Blade::directive('username', function ($expression) {
return "<?php echo auth()->user()->name; ?>";
});
// Macro to render a Blade directive to check if the user has a specific role
Blade::if('role', function ($role) {
return auth()->check() && auth()->user()->hasRole($role);
});
// Add more Blade macros as needed
}
}
These are the several types of uses of Macros.
3. Register Macros in MacrosServiceProvider
In app/Providers/MacrosServiceProvider.php
, register the macro classes:
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use App\Macros\CollectionMacros;
use App\Macros\RequestMacros;
use App\Macros\BuilderMacros;
use App\Macros\ResponseMacros;
use App\Macros\RouteMacros;
use App\Macros\ValidatorMacros;
use App\Macros\CacheMacros;
class MacrosServiceProvider extends ServiceProvider
{
public function boot()
{
// Registering all macros
CollectionMacros::register();
RequestMacros::register();
BuilderMacros::register();
ResponseMacros::register();
RouteMacros::register();
ValidatorMacros::register();
CacheMacros::register();
}
public function register()
{
//
}
}
4. Register the MacrosServiceProvider
In config/app.php
, add the MacrosServiceProvider
to the providers
array:
'providers' => [
// Other service providers...
App\Providers\MacrosServiceProvider::class,
],
5. Using the Macros
Now, you can use the macros in your application as follows:
Collection Macros
$collection = collect(['hello', 'world', 'Laravel']);
$averageLength = $collection->averageLength(); // 6
$uppercased = $collection->toUpper(); // ['HELLO', 'WORLD', 'LARAVEL']
// averageLength: Calculates the average length of strings in the collection
// toUpper: Converts all items in the collection to uppercase
Request Macros
if ($request->isAdmin()) {
// isAdmin: Checks if the authenticated user is an admin
// Do something for admin user
}
if ($request->isAjax()) {
// isAjax: Checks if the request is an AJAX request
// Handle AJAX request
}
Builder Macros
$activeUsers = User::active()->get(); // active: Scopes the query to only active users
$recentPosts = Post::recent()->get(); // recent: Scopes the query to only posts created in the last 30 days
Response Macros
return response()->api(['message' => 'Success'], 200); // api: Returns a standardized JSON response for API
Route Macros
Route::crud('posts', 'PostController'); // crud: Defines standard CRUD routes for the 'posts' resource using PostController
Validator Macros
$validator = Validator::make($data, [
'uuid' => 'required|uuid', // uuid: Adds a validation rule to check for valid UUIDs
]);
Cache Macros
$value = Cache::rememberOnce('key', 3600, function () {
return 'some expensive query result';
}); // rememberOnce: Remembers a cache value once and returns it
Blade Macros
{{-- Blade directive to display the current user's name --}}
<p>Welcome, @username</p>
{{-- Blade directive to check if the user has a specific role --}}
@role('admin')
<p>You have admin privileges.</p>
@else
<p>You do not have admin privileges.</p>
@endrole
Commonly Overlooked Information
Macro Conflicts: Ensure that the macro names you choose do not conflict with existing methods in the class.
Testing Macros: Always write tests for your macros to ensure they behave as expected.
Performance Considerations: While macros are convenient, overusing them can lead to performance issues. Use them judiciously.
Advanced Interview Questions for Laravel Macros
1. Can macros be used to modify existing methods in Laravel classes?
No, macros in Laravel are used to add additional methods to classes without changing the core code. They cannot directly alter current methods. However, you can alter existing methods by extending classes and using macros.
2. How can macros be shared across multiple Laravel projects?
Macros can be shared across projects by packaging them into reusable Composer packages. Create a package containing your macro classes and the MacrosServiceProvider. Publish this package to a repository like Packagist and then include it in your Laravel projects via Composer.
3. What are some best practices for naming macros?
Descriptive Names
Namespace Consistency
4. Is there a limit to the number of macros that can be defined for a class?
There is no hard limit on the amount of macros that can be written for a class in Laravel. However, to ensure maintainability, macros should be ordered and logically categorized.
5. Can I use closures or anonymous functions in macros?
Yes, macros can have robust and flexible custom functionality because they are usually constructed using closures or anonymous functions.
If you love the content and want to support more awesome articles, consider buying me a coffee! ☕️🥳 Your support means the world to me and helps keep the knowledge flowing. You can do that right here: 👉 Buy Me a Coffee
Check out this awesome article that breaks it down in a super-duper easy-to-understand way. 📖✨ You can find it right here: 👉Introduction to Laravel: Master the Foundation of Modern Web Development
And don’t forget to share your thoughts and feedback! 🤜💬 Let’s learn and grow together! 😊💡 #Laravel #Authentication #LearnAndGrow 🌟
Oh, and don’t forget to follow me for more exciting updates and articles! 🚀 You can find me here: Follow me
I hope you enjoy the article! 🤓
Subscribe to my newsletter
Read articles from Aman jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Aman jain
Aman jain
I'm a developer who shares advanced insights and expertise through technology-related articles. Passionate about creating innovative solutions and staying up-to-date with the latest tech trends. Let's connect and explore the world of technology together!