CI/CD Pipeline with Jenkins for a Simple Web Application.

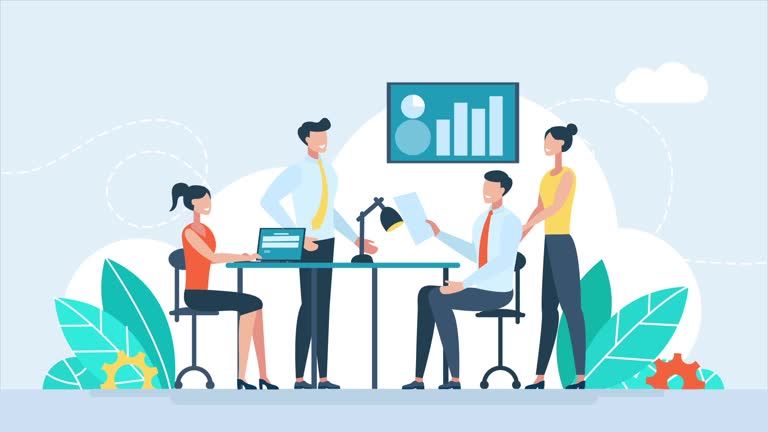
Step 1 : Install Jenkins and npm
Calculator app
const express = require('express');
const app = express();
const port = 3000;
app.use(express.urlencoded({ extended: true }));
app.get('/', (req, res) => {
res.send(`
<h1>Simple Calculator</h1>
<form action="/calculate" method="post">
<input type="number" name="num1" placeholder="Enter first number" required>
<select name="operation">
<option value="add">+</option>
<option value="subtract">-</option>
<option value="multiply">*</option>
<option value="divide">/</option>
</select>
<input type="number" name="num2" placeholder="Enter second number" required>
<button type="submit">Calculate</button>
</form>
`);
});
app.post('/calculate', (req, res) => {
const num1 = parseFloat(req.body.num1);
const num2 = parseFloat(req.body.num2);
const operation = req.body.operation;
let result;
switch (operation) {
case 'add':
result = num1 + num2;
break;
case 'subtract':
result = num1 - num2;
break;
case 'multiply':
result = num1 * num2;
break;
case 'divide':
result = num1 / num2;
break;
default:
result = 'Invalid operation';
}
res.send(`
<h1>Result: ${result}</h1>
<a href="/">Go back</a>
`);
});
app.listen(port, () => {
console.log(`Calculator app listening at http://localhost:${port}`);
});
Add Git and store the file
Jenkins :
Go to manage jenkins
Install the following plugins:
Git Plugin
NodeJS Plugin
Pipeline Plugin
Create a New Jenkins Pipeline Job:
Go to
New Item
>Pipeline
and name your job.In the pipeline section, choose
Pipeline script from SCM
and selectGit
.Enter the repository URL and branch to build.
Create a Jenkinsfile
in your project repository:
pipeline {agent any stages { stage('Build') { steps { script { // Install dependencies sh 'npm install' } } } stage('Test') { steps { script { // Run tests (add your tests here) sh 'echo "Running tests..."' } } } stage('Deploy') { steps { script { // Deploy to a server (simple example: run the app) sh 'nohup node index.js &' } } } } }
Modified jenkinfiles and practice
pipeline { agent any stages { stage('Build') { steps { script { // Install dependencies sh 'npm install' } } } stage('Lint') { steps { script { // Run linting (replace with your actual lint command) sh 'npm run lint || echo "Linting failed, but continuing..."' } } } stage('Test') { steps { script { // Run tests (replace with your actual test command) sh 'npm test || echo "Tests failed, but continuing..."' } } } stage('Package') { steps { script { // Package the application (replace with your actual package command) sh 'npm run build || echo "Packaging failed, but continuing..."' } } } stage('Deploy') { steps { script { // Deploy to a server (simple example: run the app) sh 'nohup node index.js &' } } } stage('Cleanup') { steps { script { // Cleanup steps (optional) sh 'rm -rf node_modules' } } } } }
Subscribe to my newsletter
Read articles from Shreya Rewale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
