String methods in JavaScript
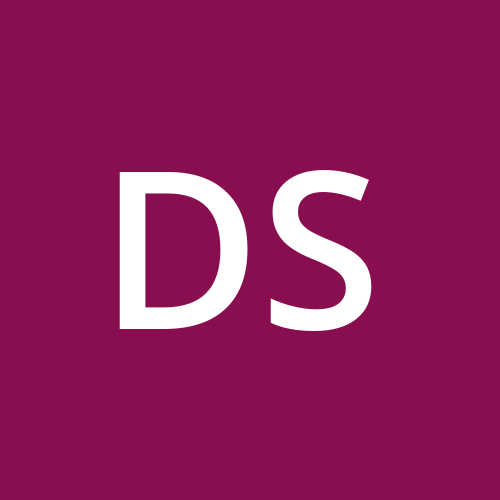
anchor(),big(),blink(),bold(),fixed(),fontcolor(),fontsize(),italics(),link() method is deprecated in JavaScript.
The at() returns an indexed character from a string.It says whatever string at that particular index it is the answer.
let text = "I am rich";
console.log(text.at(0));
Code will display “I” because it is at 0 index.
Next method is charAt().It says whatever character at the particular index it is the answer.If we don’t give any index, charAt() will return the first character of the string. If you provide any index which is not in range it will give empty string
let text = "I told you I am rich";
let firstChar = text.charAt(0); // Returns 'I'
charCodeAt() returns unicode of the character. charPointAt() also return the unicode. but only charPointAt() can return the full value of a Unicode.
concat() joins the 2 or more string without manipulating the original.
let greeting = "Hello";
let name = "World";
let message = greeting.concat(", ", name, "!");
console.log(message); // "Hello, World!"
constructor() gives the function that created the String prototype.
let message = "Hello World!";
let text = message.constructor;
endsWith() returns a boolean value. It says if your particular string ends with string I provide then, it is true .Otherwise you are wrong as always.
let paisa="I am richie rich"
console.log(paisa.endsWith("rich"))//returns true
includes() returns boolean value according to given string.It says if the string you give is included in the string then it is ture.
let paisa="I am richie rich"
console.log(paisa.includes("rich"))//returns true
indexOf() says I will give you the index of the portion of string you provide to me.but the portion you give it to me is not present in the main string then I will give you “-1“.
const isRich="yes divyansh is rich"
console.log(isRich.indexOf("rich"))//returns 16
isWellFormed() is returns trues if the lone surrogate is not present.
const str1 = "hello";
console.log(str1.isWellFormed());//returns true
The lastIndexOf() method returns the index (position) of the last occurrence of a specified value in a string.
let paisa="Today I make relatively lesser money,But sooner I will make money more than anyone."
console.log(paisa.lastIndexOf("money"))//returns 60 because it is the last occurrence
lastIndexOf() returns -1 if sorted before,1 if sorted after,0 if equal.
let text1 = "A";
let text2 = "a";
let result = text1.localeCompare(text2);//returns 1
The match() matches a string against a regular expression.The match() returns an array with the matches.
let text = "The rain in SPAIN stays mainly in the plain";
let result = text.match(/ain/gi);
matchAll() creates an iterator object. It populates the newly created iterator with all the patterns that match the method’s regular expression argument.
const string = "Tuesday";
// Assign a regular expression to a variable:
const regExp = /day/g;
// Match the regular expression's pattern against the string and assign the result to a variable:
const iteratorResult = string.matchAll(regExp);
// Convert the iterator object to an array:
const arrayResult = Array.from(iteratorResult);
// Print the arrayResult variable's content on the console:
console.log(arrayResult);
// The invocation above will return:
[['day', index: 4, input: 'Tuesday', groups: undefined]]
String.prototype.normalize() returns the Unicode Normalized Form of the string. This is because in Unicode, some characters can have different representations.
const name1 = '\u0041\u006d\u00e9\u006c\u0069\u0065';
const name2 = '\u0041\u006d\u0065\u0301\u006c\u0069\u0065';
console.log(`${name1}, ${name2}`);
// expected output: "Amélie, Amélie"
console.log(name1 === name2);
// expected output: false
console.log(name1.length === name2.length);
// expected output: false
const name1NFC = name1.normalize('NFC');
const name2NFC = name2.normalize('NFC');
console.log(`${name1NFC}, ${name2NFC}`);
// expected output: "Amélie, Amélie"
console.log(name1NFC === name2NFC);
// expected output: true
console.log(name1NFC.length === name2NFC.length);
// expected output: true
Subscribe to my newsletter
Read articles from Divyansh Savita directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
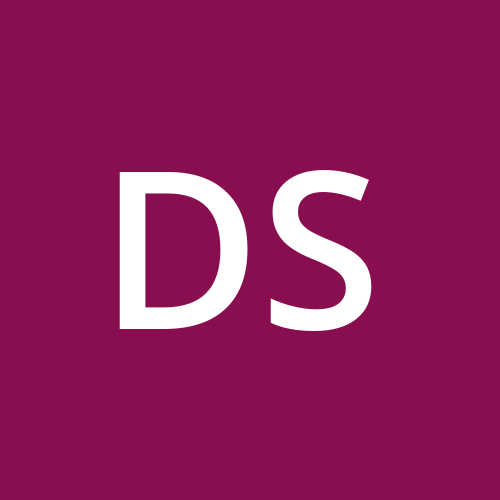