Solving a Data Management Challenge with Django Proxy Models

Table of contents
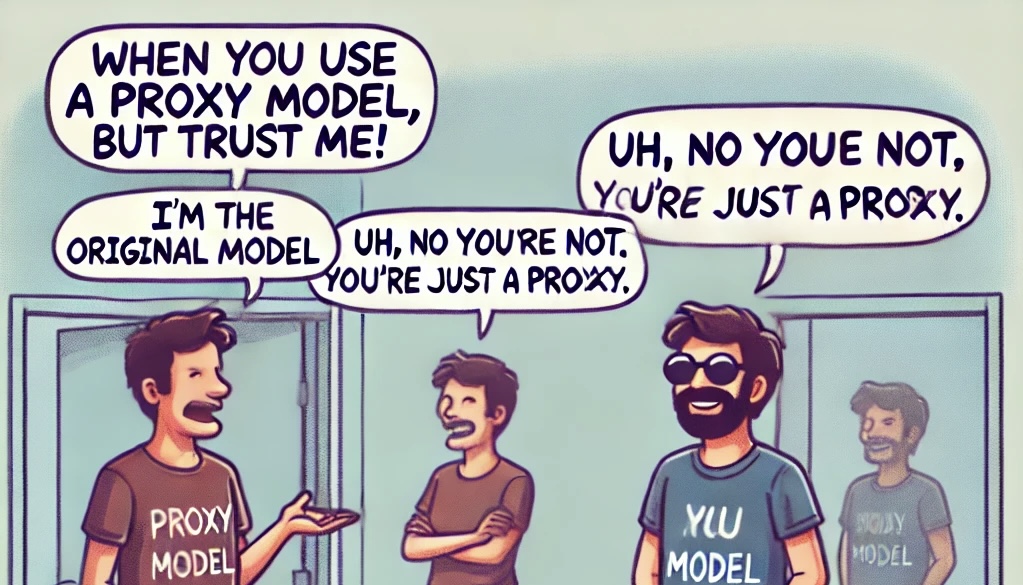
Today, I ran into an intriguing problem in my Django project. I had a table model that was storing combined data from two different models in a JSON field - a bit of a data mess! My goal was to separate and display this combined data into two distinct models in the Django Admin interface, without having to create a new database table for these models. After weighing my options, I decided to use Django's proxy models to tackle this challenge. It was the perfect solution to tidy up my data without disrupting the underlying database structure.
The Challenge
I was working with a model called InsuranceData, which had a field called combined_details. This field was a bit of a catch-all, storing data from both employee-related and company-related models. The problem was, I needed to display and manage these two sets of data separately in the Django admin interface. Easy enough, right? Well, not quite. Django's admin system is super flexible, but this particular problem required some creative thinking. I didn't want to create new database tables just to separate the data - that would've added a whole new layer of complexity and redundancy. I needed a solution that would let me reuse the existing data structure, without making a mess of my database.
The Solution
Since the goal was to separate this data into two neat tables in the Django admin interface without creating any new database tables just to split the data.
Enter Proxy Models - My Savior!
So, what's a proxy model? Think of it like a subclass that inherits from the original model but uses the same database table. It's like a costume change - same underlying structure, different behavior. Unlike abstract models, proxy models can't add new fields, but they can change how the model behaves, especially in the admin interface.
A quick go through:
· Abstract Model: A blueprint for shared fields among subclasses (no database table needed)
· Proxy Model: A subclass that shares the same database table but can alter its behavior (no new fields allowed)
Thanks to proxy models, I created two separate models - one for company insurance and one for employee insurance - without creating new database tables! I could then customize the admin interface to display each model's data in its own table, making data management a breeze.
How I Implemented It
Here’s how I created proxy models to split the combined data into two parts in the Django admin interface.
1.Define the Base Model
My base model, InsuranceData, has a combined_details JSON field.
class InsuranceData(models.Model):
employee = models.ForeignKey(Employee, on_delete=models.SET_NULL, null=True)
company = models.ForeignKey(Company, on_delete=models.SET_NULL, null= True)
combined_details = JSONField()
2.Create Proxy Models
I created two proxy models, one for handling employee_data and the other for company_data. These models inherit from InsuranceData but don't create any new database tables.
class InsuranceDataEmployee(InsuranceData):
class Meta:
proxy = True
verbose_name = 'Employee Insurance Data'
verbose_name_plural = 'Insurance Data (Employee)'
class InsuranceDataCompany(InsuranceData):
class Meta:
proxy = True
verbose_name = 'Company Insurance Data'
verbose_name_plural = 'Insurance Data (Company)'
3. Register Proxy Models in Admin
Then, I customized the Django admin interface for both proxy models. This allowed me to control how the employee_data and company_data from combined_details are displayed
class EmployeeDataAdmin(admin.ModelAdmin):
list_display = ('id', 'employee_data_pretty')
def employee_data_pretty(self, obj):
return obj.combined_details.get('employee_data', {})
class CompanyDataAdmin(admin.ModelAdmin):
list_display = ('id', 'company_data_pretty')
def company_data_pretty(self, obj):
return obj.combined_details.get('company_data', {})
admin.site.register(InsuranceDataEmployee, InsuranceDataEmployeeAdmin)
admin.site.register(InsuranceDataCompany, InsuranceDataCompanyAdmin)
Using proxy models, I successfully split and displayed the employee and company data from the combined_details JSON field in the Django admin interface. This approach was a game-changer, allowing me to present the data in a more organized and intuitive way without messing with the database structure or creating new tables.
The beauty of proxy models lies in their flexibility. I could customize the behavior of the model in Django admin to fit my specific needs without compromising the integrity of the underlying data.
So, why did proxy models work so well for me?
No New Table:
I avoided creating a new database table, which was a top priority. I didn't want to add redundancy and unnecessary complexity to my database.
Flexibility in Admin:
I could tailor the data display and handling in the admin interface to my liking, without altering the core InsuranceData model.
No Additional Fields:
Proxy models don't allow new fields, which was perfect for my requirements.
The separate wrapper table for employee and company containing data bifurcated between them in a single json from other model
In short, proxy models offered the perfect solution - flexibility without compromise. I could customize the admin interface to my heart's content without messing with the underlying data structure. Win-win!"
Conclusion
Django's proxy models are a total lifesaver when it comes to modifying existing models without disrupting the database. They offer a flexible and lightweight solution to customize the admin interface, making it a must-know tool for any Django developer. So, the next time you're faced with a data display dilemma, remember: proxy models are your friend!"
Subscribe to my newsletter
Read articles from Ateeb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
