Building a Robust Web Scraper with Python and BeautifulSoup
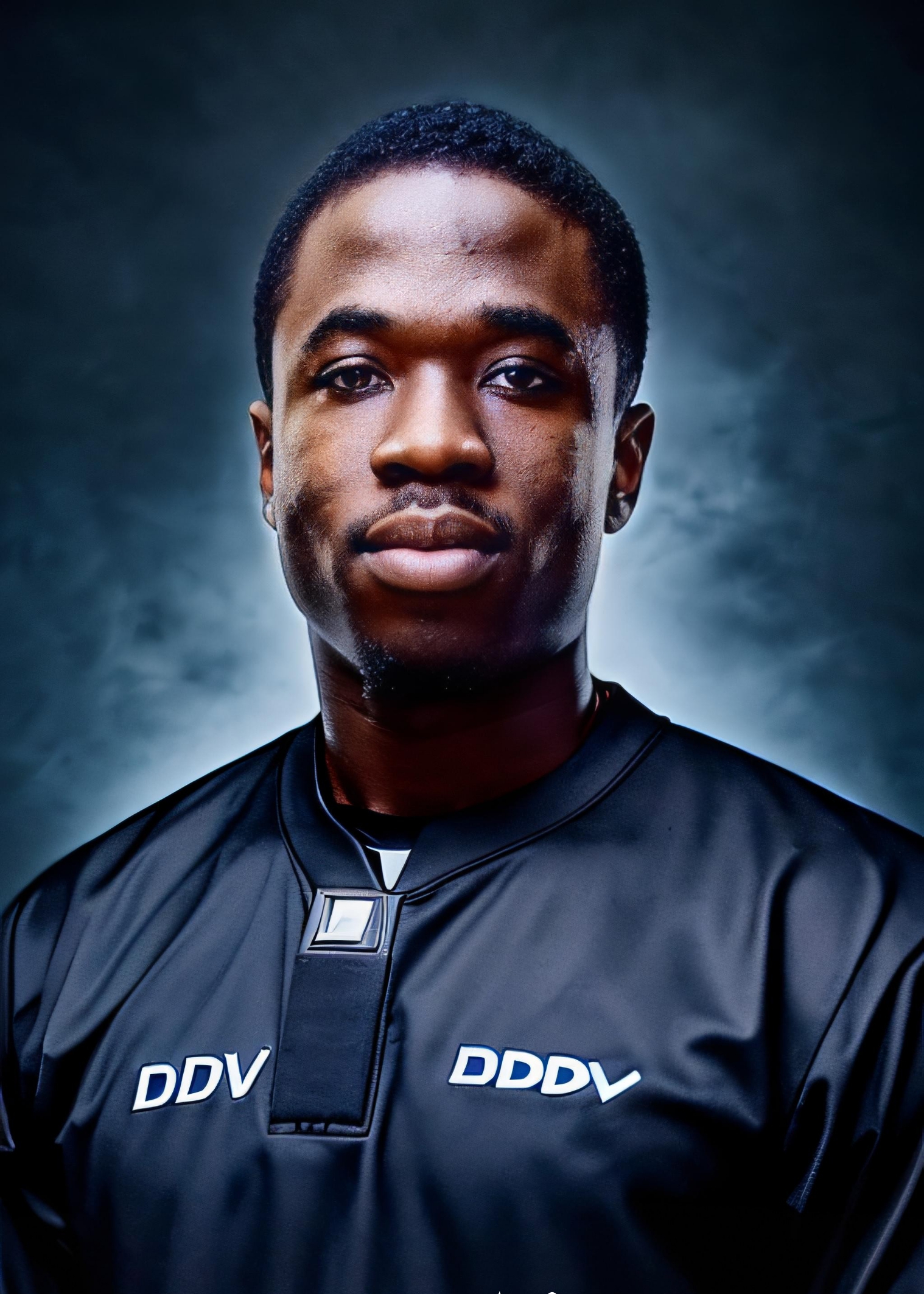
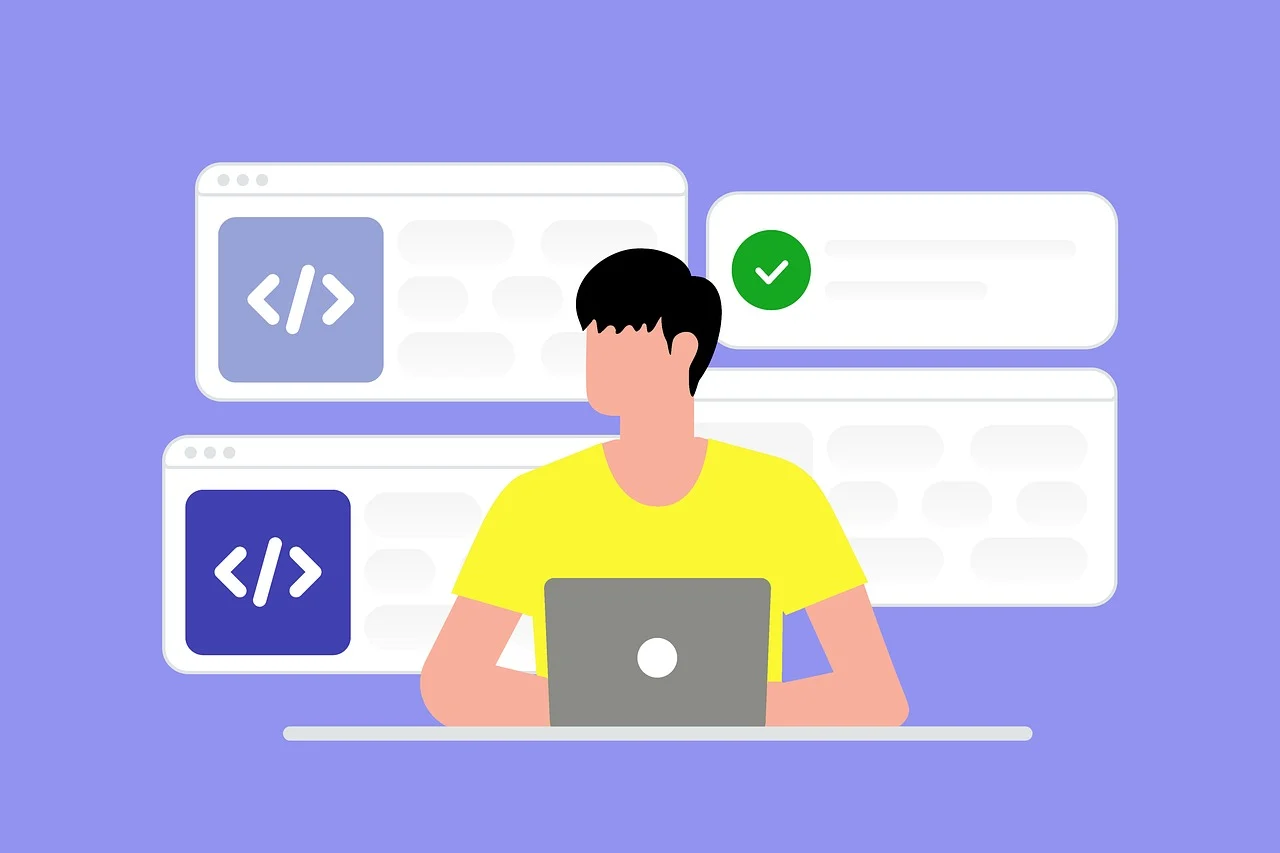
Introduction
Web scraping has become an essential tool for extracting useful information from websites. Whether it's gathering data for research, tracking product prices, or scraping blog posts, web scraping automates the process of fetching data that would otherwise require manual copying.
Python, with its clean syntax and an extensive set of libraries, has become a popular choice for building web scrapers. Among these libraries, BeautifulSoup stands out for its simplicity in parsing HTML and extracting data. This article walks you through the process of building a robust web scraper using Python and BeautifulSoup, while ensuring it can handle real-world challenges like errors, dynamic content, and ethical considerations.
Prerequisites
Before diving into the details, there are a few prerequisites to keep in mind:
Basic Python Knowledge: You should have a solid understanding of Python syntax, functions, and control structures.
Python Environment Setup: Ensure that Python is installed (preferably version 3.x). You will also need to install additional libraries like BeautifulSoup and Requests.
HTML and CSS: Familiarity with the basic structure of HTML and how CSS selectors work will be beneficial.
Web Scraping Legalities: Always check the legal terms of the website you're scraping, and ensure you comply with its terms of service. Respect
robots.txt
files and ethical guidelines.
Installing Required Libraries
To get started, you'll need to install a few Python packages. Run the following commands in your terminal:
pip install beautifulsoup4 requests lxml
BeautifulSoup4
: For parsing and navigating HTML.Requests
: For making HTTP requests to websites.lxml
: An optional library that speeds up HTML parsing.
Step-by-Step Guide to Building the Scraper
Step 1: Setting Up the Python Environment
After installing Python and the required libraries, we can start by writing a simple script to fetch and display a web page.
import requests
from bs4 import BeautifulSoup
# Fetch the webpage content
url = 'https://example.com'
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
print("Web page fetched successfully!")
else:
print(f"Failed to fetch page. Status code: {response.status_code}")
# Parse the HTML content
soup = BeautifulSoup(response.content, 'lxml')
print(soup.prettify())
This code sends an HTTP request to a webpage and parses the returned HTML content using BeautifulSoup. The prettify()
method prints the HTML in a readable format, making it easier to inspect the structure.
Step 2: Understanding the HTML Structure of the Target Website
To scrape meaningful data, you need to inspect the HTML structure of the target site. Use your browser’s developer tools (right-click on a webpage and select “Inspect”) to locate the HTML tags, classes, and IDs that contain the data you want to extract.
For example, if you're scraping a blog, look for the <h1>
or <h2>
tags that hold the article titles.
Step 3: Fetching and Parsing the Web Page
Once you've identified the elements to scrape, use BeautifulSoup to extract them:
# Find all the headings in the page
headings = soup.find_all('h1')
for heading in headings:
print(heading.text)
The find_all()
method returns all the matching elements based on the tag specified. You can refine this by targeting specific classes or IDs using the class_
or id
parameters.
Step 4: Extracting Data Efficiently
For more complex websites, you may need to deal with tables, links, or images. BeautifulSoup allows you to efficiently navigate and extract such data.
# Extract all links from the page
links = soup.find_all('a')
for link in links:
print(link.get('href'))
This code extracts all <a>
tags (which represent links) and prints the href
attribute, which contains the link URL.
Making the Scraper Robust
Handling Errors and Exceptions
Not all requests succeed, and websites can be unreliable. Your scraper should handle errors gracefully:
try:
response = requests.get(url, timeout=10)
response.raise_for_status() # Raises HTTPError for bad responses
except requests.exceptions.HTTPError as e:
print(f"HTTP error: {e}")
except requests.exceptions.RequestException as e:
print(f"Error fetching data: {e}")
This code uses try-except
blocks to catch errors such as connection timeouts or HTTP errors, ensuring your scraper doesn't crash unexpectedly.
Avoiding Scraping Blocks
Some websites block requests from scripts. To avoid this, you can mimic a real browser by modifying the headers of your requests:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
This sets a User-Agent
header that mimics a legitimate browser, reducing the chances of being blocked.
Dealing with Dynamic Content
If a website uses JavaScript to load content dynamically, BeautifulSoup alone won’t suffice. In such cases, you can use Selenium to render the page before scraping:
pip install selenium
Selenium controls a web browser and can interact with pages, simulating a real user.
Saving and Storing the Scraped Data
Once the data is extracted, you’ll want to store it in a usable format. You can write the data to a CSV, JSON, or database:
import csv
# Save data to a CSV file
with open('data.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Heading'])
for heading in headings:
writer.writerow([heading.text])
This stores the scraped data in a CSV file, which can be opened in spreadsheet applications like Excel.
Ethical Considerations and Best Practices
Web scraping should be done responsibly. Here are some guidelines:
Respect
robots.txt
: Many websites userobots.txt
to specify which parts of the site should not be scraped.Avoid Overloading Servers: Implement delays between requests to avoid overwhelming the server.
import time
time.sleep(2) # Pause for 2 seconds between requests
- Comply with Terms of Service: Some websites explicitly forbid scraping in their terms of service. Always review these before proceeding.
Conclusion
Building a robust web scraper with Python and BeautifulSoup is straightforward, but ensuring it can handle real-world challenges like dynamic content, errors, and ethical scraping is key. With the steps outlined above, you're ready to create a web scraper tailored to your needs. Experiment with additional features like scraping multiple pages or storing data in databases, and enjoy the efficiency that automated data extraction brings!
Further Enhancements
Scraping Multiple Pages: Implement pagination to scrape data across multiple pages.
Using APIs: Where available, using APIs can be more efficient and legally safer than scraping.
Advanced Scraping: Combine BeautifulSoup with tools like Selenium or Scrapy for larger-scale scraping projects.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
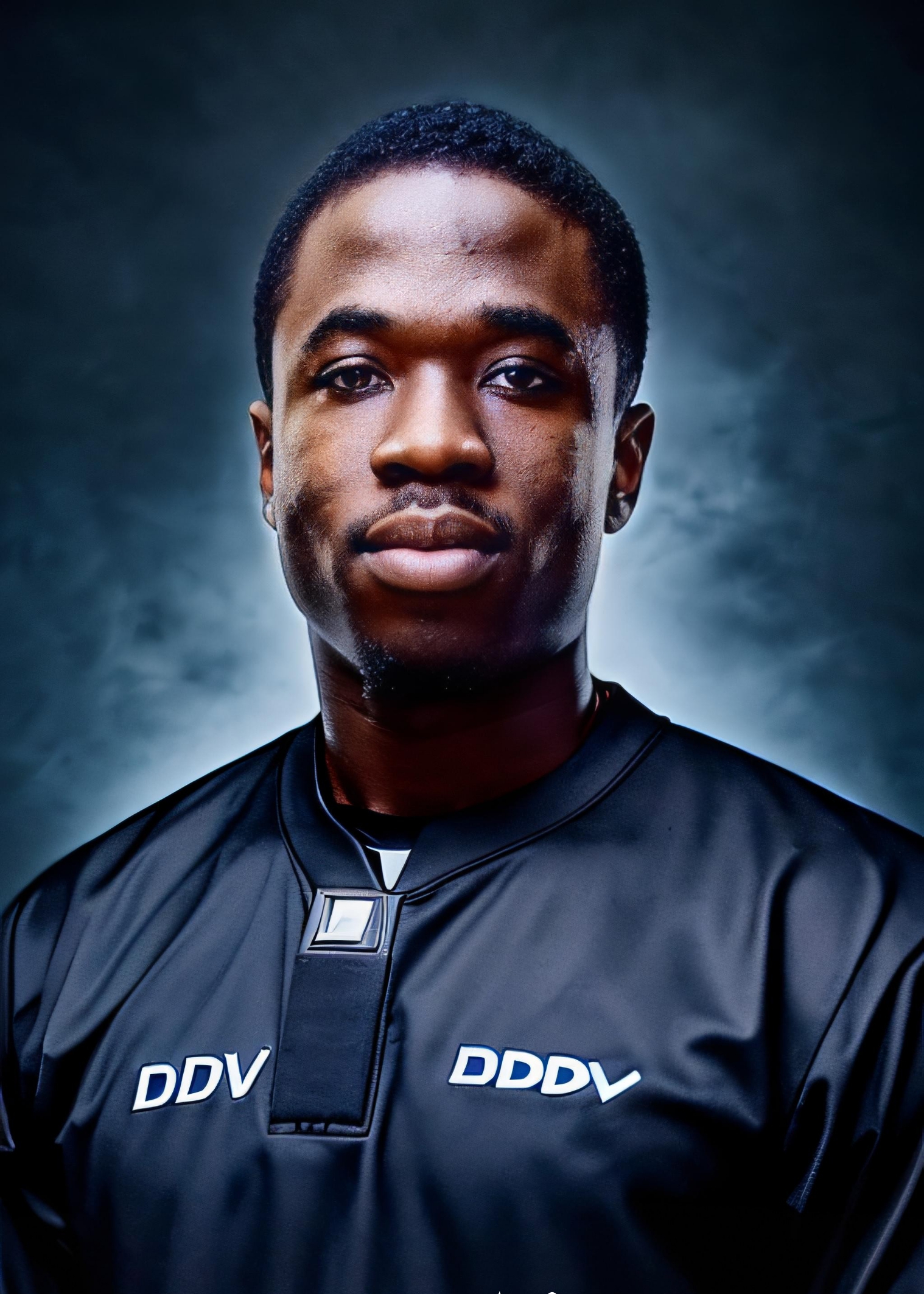
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.