Browser Automation Techniques with Selenium WebDriver in Python
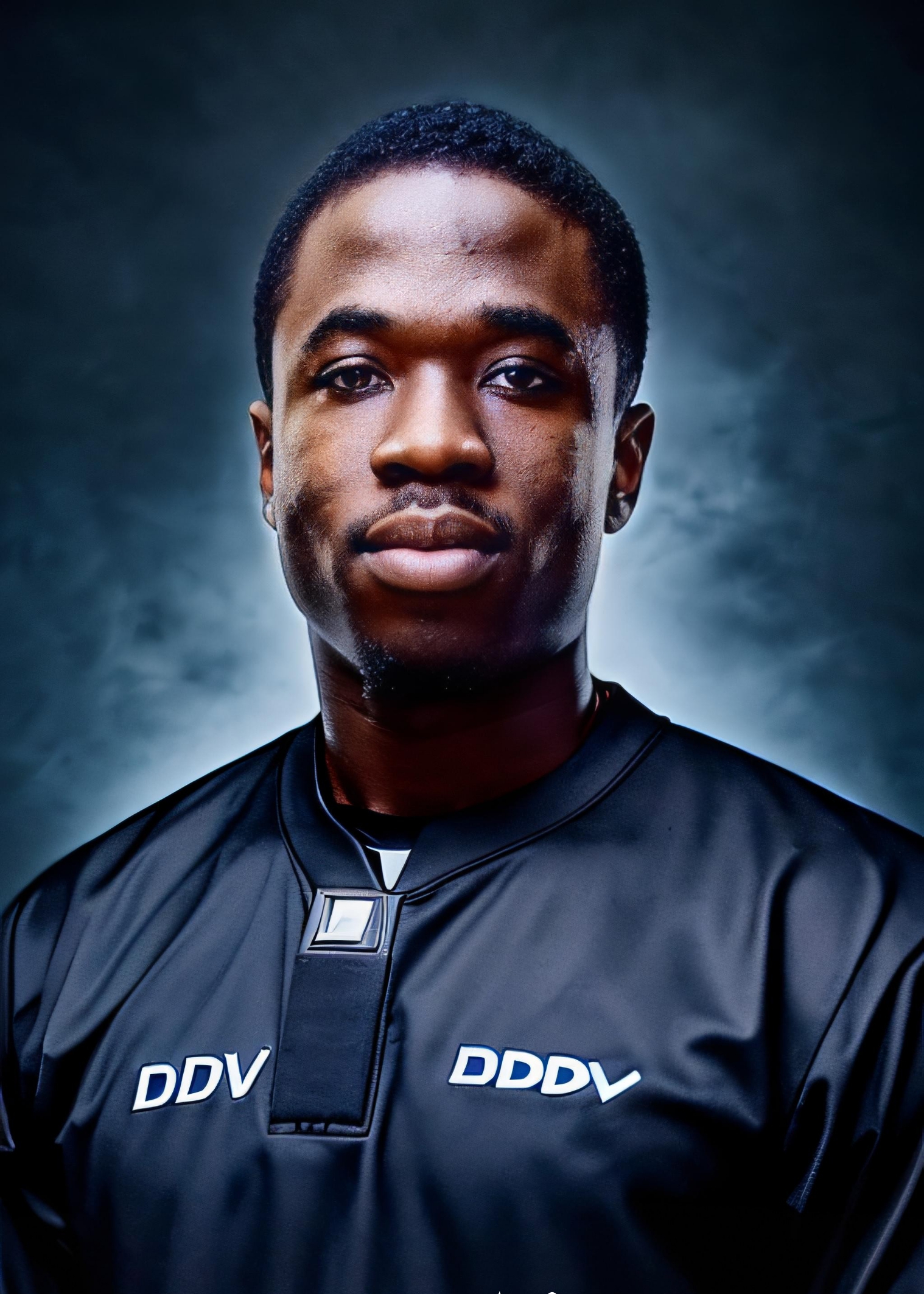
Table of contents
- Introduction
- Prerequisites
- Setting Up Selenium WebDriver
- Basic Browser Automation Techniques
- Advanced Browser Automation Techniques
- Working with WebDriver Waits
- Automating Complex Workflows
- Handling Exceptions and Debugging
- Running Selenium Tests Headlessly
- Best Practices for Browser Automation
- Conclusion
- References
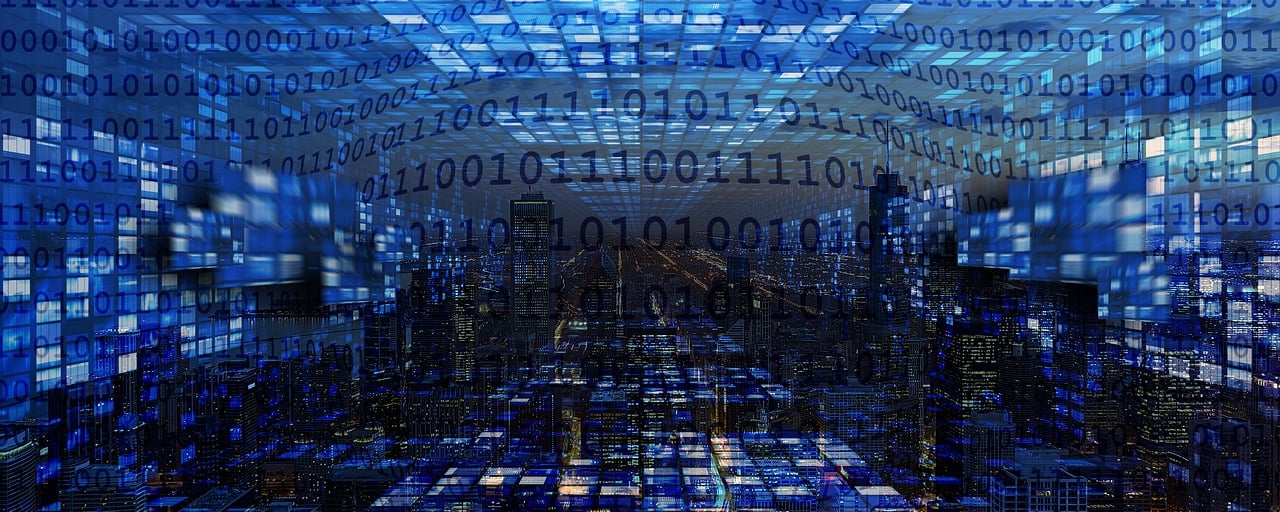
Introduction
Browser automation is an essential part of web development and testing, enabling developers and testers to automate repetitive browser-based tasks. Selenium WebDriver is one of the most widely-used tools for this purpose. It provides a suite of functionalities to simulate browser actions, interact with web elements, and automate testing workflows. Python, with its simple syntax and powerful libraries, is an excellent language to implement Selenium-based browser automation.
In this article, we will dive deep into various techniques for browser automation using Selenium WebDriver in Python. By the end, you will have a clear understanding of how to automate different tasks in a web browser using Python and Selenium.
Prerequisites
Before diving into the techniques, it is essential to ensure that you have the following prerequisites:
Basic knowledge of Python programming: Familiarity with Python syntax and concepts such as variables, loops, and functions is necessary.
Understanding of HTML and web technologies: You need to know how web pages work, specifically the Document Object Model (DOM), CSS selectors, and HTML attributes.
Python environment setup: Ensure you have Python (version 3.x) installed on your machine. You can verify the installation by running the following command in the terminal:
python --version
Installing Selenium: Use the following command to install Selenium via
pip
:pip install selenium
WebDriver: Selenium requires a specific WebDriver for the browser you intend to use (e.g., ChromeDriver for Chrome, GeckoDriver for Firefox). Download the correct WebDriver and ensure it's in your system’s PATH.
For example, download ChromeDriver for Chrome or GeckoDriver for Firefox.
Setting Up Selenium WebDriver
After installing Selenium and downloading the WebDriver, it's time to set up a simple browser automation script.
Install Selenium:
pip install selenium
Download WebDriver: If you’re using Chrome, download ChromeDriver.
Sample Script: A basic Selenium script to launch Chrome and navigate to a website.
from selenium import webdriver # Specify the path to the ChromeDriver executable driver = webdriver.Chrome(executable_path='/path/to/chromedriver') # Open a website driver.get("https://www.example.com") # Close the browser driver.quit()
Basic Browser Automation Techniques
Now that you’ve set up Selenium, let’s explore how to automate basic browser tasks.
Opening a browser window: You can open a new browser window by instantiating a
webdriver
object.driver = webdriver.Chrome() # For Chrome
Navigating to a URL: To load a website, use the
get
method.driver.get("https://www.example.com")
Locating Elements: Use methods such as
find_element_by_id
,find_element_by_name
,find_element_by_xpath
, etc., to interact with specific elements.element = driver.find_element_by_id('element_id')
Interacting with Elements: Interactions such as clicking buttons, typing text, and submitting forms can be easily handled.
Clicking a button:
button = driver.find_element_by_id('submit_button') button.click()
Typing text into a form field:
input_field = driver.find_element_by_name('username') input_field.send_keys('my_username')
Submitting a form:
form = driver.find_element_by_tag_name('form') form.submit()
Advanced Browser Automation Techniques
Selenium also provides capabilities to handle more advanced scenarios.
Handling pop-ups and alerts: You can switch to alerts and either accept or dismiss them.
alert = driver.switch_to.alert alert.accept() # Or alert.dismiss()
Switching between tabs or windows: Selenium allows you to manage multiple browser tabs or windows.
driver.switch_to.window(driver.window_handles[1]) # Switch to the second tab
Taking screenshots: Capture screenshots of the current state of the browser.
driver.save_screenshot('screenshot.png')
Executing JavaScript: You can run JavaScript directly within the browser’s context.
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
Working with WebDriver Waits
Waits are critical for ensuring that the elements you want to interact with are available. There are two types of waits in Selenium:
Implicit Waits: Instruct WebDriver to wait for a specific amount of time before throwing an exception.
driver.implicitly_wait(10) # Waits for 10 seconds
Explicit Waits: More flexible, allowing you to wait for a specific condition to be met.
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, 'element_id')) )
Automating Complex Workflows
Automating login: Selenium can be used to automate login by entering credentials and clicking the login button.
driver.find_element_by_name('username').send_keys('your_username') driver.find_element_by_name('password').send_keys('your_password') driver.find_element_by_name('login').click()
Scraping data: Use Selenium to extract and scrape data from a web page.
data = driver.find_element_by_xpath('//div[@class="data"]').text
Handling Exceptions and Debugging
Common exceptions: Selenium throws various exceptions like
NoSuchElementException
if an element isn’t found. Handle these using try-except blocks.from selenium.common.exceptions import NoSuchElementException try: element = driver.find_element_by_id('non_existent_id') except NoSuchElementException: print("Element not found")
Running Selenium Tests Headlessly
Headless mode allows Selenium to run browser automation without a visible UI, which is useful for running tests on servers or CI pipelines.
Setting up headless mode in Chrome:
options = webdriver.ChromeOptions() options.add_argument('--headless') driver = webdriver.Chrome(options=options)
Best Practices for Browser Automation
Keep WebDriver and browser versions in sync to avoid compatibility issues.
Use waits properly to ensure stability.
Write maintainable scripts: Use functions and modular approaches to make scripts reusable.
Handle errors gracefully: Implement proper error handling to avoid abrupt failures.
Conclusion
In this article, we covered a wide range of browser automation techniques using Selenium WebDriver in Python. We explored basic automation tasks such as opening a browser, interacting with web elements, and filling forms. We also went into advanced techniques such as handling pop-ups, switching between tabs, and automating complex workflows. Finally, we discussed best practices to make your scripts more reliable and maintainable.
For further learning, you can explore integrating Selenium with testing frameworks like PyTest for more comprehensive testing automation.
References
This article provides a solid foundation for automating web tasks using Selenium in Python. With practice, you can extend these concepts to build more complex automation scripts tailored to your specific needs.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
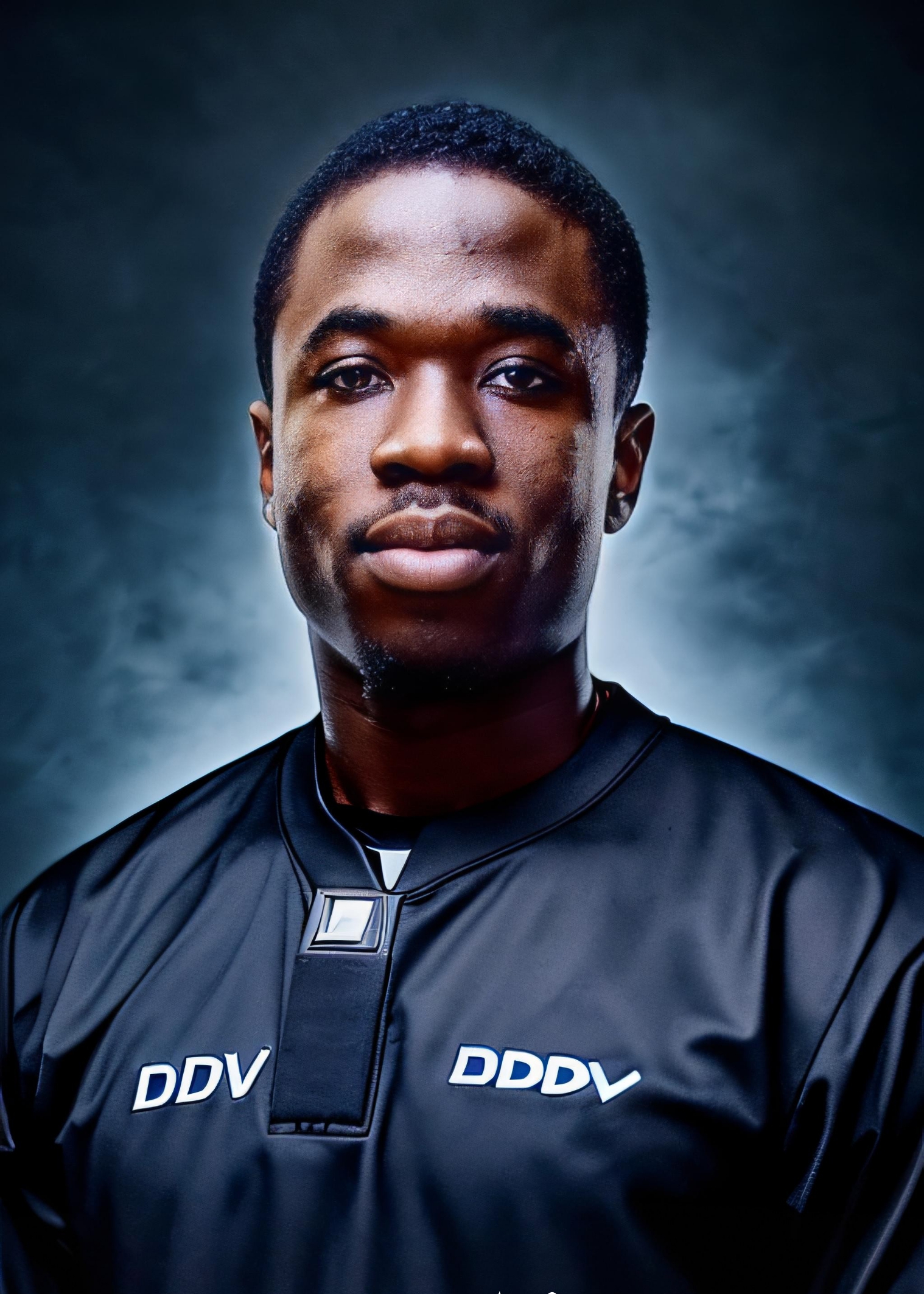
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.