Step-by-Step Guide to Using Playwright for Page Interactions and Assertions 🌠
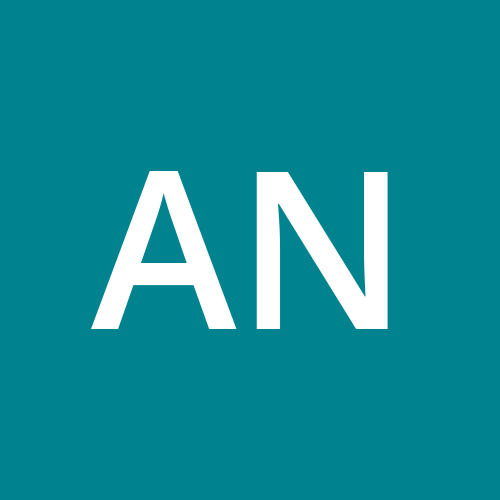
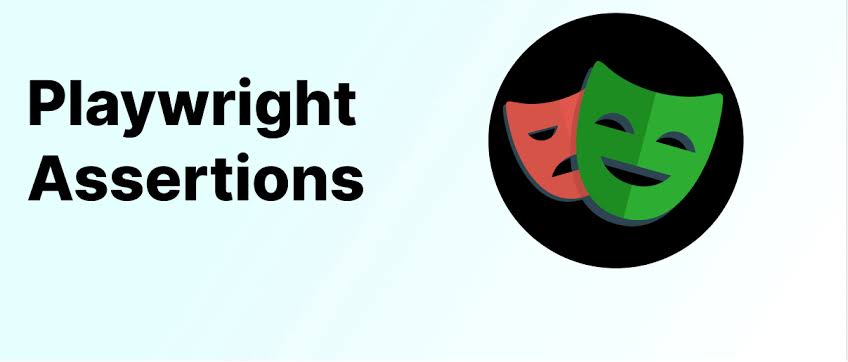
When automating web testing, it's not just about finding elements on a page — it's about interacting with them and verifying that they behave as expected. In this blog, we’ll explore how Playwright helps you handle user interactions and assert conditions with confidence, making your tests more robust and reliable.
🖱️ 1. Basic Page Interactions
Page interactions are fundamental to any web automation. Whether you’re clicking a button, typing into a form, or selecting an option from a dropdown, Playwright has simple, intuitive APIs for these actions.
Example: Typing into a Form
Let's say you’re testing a login form. The typical interaction would involve filling in a username and password and clicking the submit button.
await page.goto('https://example.com/login');
await page.fill('#username', 'testuser'); // Fill username field
await page.fill('#password', 'testpassword'); // Fill password field
await page.click('button[type="submit"]'); // Click submit button
💡 Real-Time Scenario: Testing a login form with different user roles (admin, guest, etc.) would involve multiple test cases to validate input handling and form submission behavior.
📄 2. Selecting Dropdowns and Options
Dropdowns are common in forms, and selecting options dynamically is crucial. Playwright offers simple methods to interact with select
elements.
Example: Selecting from a Dropdown
await page.selectOption('#country', 'US'); // Select the United States from a dropdown
💡 Real-Time Scenario: When automating the user registration process, selecting different countries or states from a dropdown is crucial for validating that all options are working correctly.
✅ 3. Verifying Page Navigation
Often, actions such as form submissions or button clicks navigate to new pages or trigger redirects. Playwright provides an easy way to ensure that navigation happens as expected.
Example: Waiting for Page Navigation
await page.click('text=Submit');
await page.waitForNavigation(); // Wait for navigation to complete
💡 Real-Time Scenario: After clicking "Submit" on a registration form, the user should be redirected to a success page. You can assert that the URL has changed to the success page’s URL.
🔍 4. Assertions to Validate Conditions
Assertions are used to verify that the webpage behaves as expected. In Playwright, you can assert various conditions, such as element visibility, text content, or URL changes. These assertions ensure your tests pass only when the right conditions are met.
Example: Asserting Element Visibility
await expect(page.locator('text=Welcome, User')).toBeVisible();
💡 Real-Time Scenario: After logging in, a welcome message should appear. By asserting that the "Welcome, User" text is visible, you ensure that the login process worked as intended.
Example: Asserting URL
await expect(page).toHaveURL('https://example.com/dashboard');
💡 Real-Time Scenario: After logging in, the user should be redirected to the dashboard page. This URL assertion verifies the user lands on the correct page.
⏲️ 5. Waiting for Elements and Conditions
In real-world scenarios, elements on a webpage may take some time to load. Playwright provides different waiting mechanisms to ensure actions and assertions are executed only when the elements are ready.
Example: Waiting for an Element to Appear
await page.waitForSelector('#loading-spinner', { state: 'hidden' });
💡 Real-Time Scenario: If there’s a loading spinner that appears during data fetching, you’d want to wait until the spinner disappears before interacting with the page.
🔄 6. Handling Dynamic Content
Modern web applications often load dynamic content asynchronously (e.g., via API calls). Playwright allows you to interact with dynamic elements and verify their appearance or disappearance as needed.
Example: Waiting for Dynamic Content
await page.waitForSelector('.dynamic-data', { state: 'visible' });
💡 Real-Time Scenario: In a dashboard application, data might load after a brief delay. You can ensure the data is visible before running any assertions or interactions.
🔗 7. Handling Multiple Tabs or Windows
Web apps often open new tabs or pop-up windows when interacting with specific elements, like clicking external links or opening help documents. Playwright can handle these multi-tab or multi-window scenarios seamlessly.
Example: Working with New Tabs
const [newPage] = await Promise.all([
page.waitForEvent('popup'), // Wait for the new tab
page.click('a[target="_blank"]') // Click the link that opens in a new tab
]);
await newPage.waitForLoadState();
await expect(newPage).toHaveURL('https://example.com/new-tab-page');
💡 Real-Time Scenario: Clicking a "Help" link on a form may open a new tab with a support page. You can ensure the new tab loads properly and asserts the expected URL.
📅 8. File Uploads and Downloads
Handling file uploads and downloads is a common scenario in web automation. Playwright makes it easy to interact with file inputs and download buttons.
Example: File Upload
const filePath = 'path/to/file.pdf';
await page.setInputFiles('input[type="file"]', filePath);
💡 Real-Time Scenario: Testing a form that allows users to upload their resume or profile picture. You can automate the file selection process and ensure it’s handled correctly.
Example: File Download
const [download] = await Promise.all([
page.waitForEvent('download'),
page.click('a#download-link')
]);
const filePath = await download.path();
💡 Real-Time Scenario: After clicking a "Download Report" button, you want to verify the report is downloaded successfully and check its contents.
💬 9. Handling Alerts and Popups
Sometimes, interacting with a page may trigger alerts, confirmation dialogs, or other pop-ups. Playwright allows you to handle these seamlessly.
Example: Handling a Popup
page.once('dialog', dialog => {
console.log(`Dialog message: ${dialog.message()}`);
dialog.accept(); // Accept the popup
});
await page.click('button#delete-account');
💡 Real-Time Scenario: When a user tries to delete their account, a confirmation dialog appears. You can handle this pop-up in your automation and verify it was properly managed.
🔁 10. Retry Logic for Unstable Interactions
In some cases, actions or conditions might not always succeed on the first attempt, especially in unstable environments. Playwright’s retries and timeouts help manage these situations.
Example: Retrying an Action
await page.waitForSelector('#retry-button', { timeout: 5000 });
await page.click('#retry-button');
💡 Real-Time Scenario: On slow networks, an action may fail to trigger due to timeouts. You can add retry logic to ensure the action eventually succeeds.
🚀 Mastering page interactions and assertions is key to writing reliable end-to-end tests with Playwright. From basic form interactions to handling multiple tabs, file uploads, and dynamic content, Playwright offers an intuitive API to make your automation smooth and robust. Assertions help verify that each interaction behaves as expected, ensuring your web application performs consistently across different scenarios.
Playwright’s versatility allows you to handle real-world challenges like asynchronous content, multi-tab navigation, file handling, and more, making it a powerful tool for modern web automation.
🎭 Take control of your test automation and make every interaction count with Playwright!
#Playwright #AutomationTesting #SoftwareTesting #WebAutomation #JavaScript #EndToEndTesting #TechBlog #FrontendTesting #TestAutomation
Subscribe to my newsletter
Read articles from Anandkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
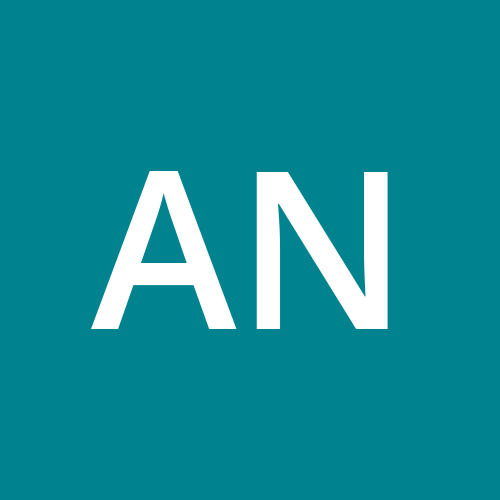
Anandkumar
Anandkumar
"Passionate playwright dedicated to refining scripts through innovative methods. Embracing automated testing to enhance creativity and efficiency in crafting compelling narratives. Let's revolutionize storytelling together!"