21 Days of Solidity Smart Contract Security Research: Day - 4
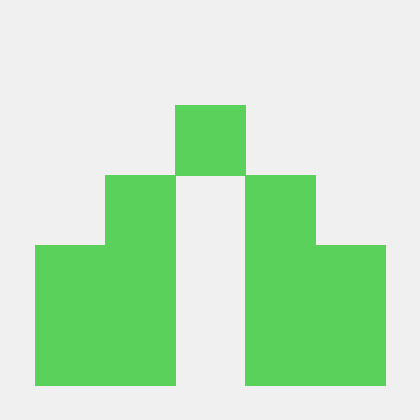
We successfully crossed Day 1, Day 2, and Day 3 about Solidity Smart Contract Security Research. Now, we’re on Day 4 to learn about Decentralized Finance and the important terms where smart contracts play a vital role.
Decentralized Finance (DeFi) and Smart Contracts: A Comprehensive Guide for Beginners
Introduction to DeFi and Smart Contracts
Decentralized Finance, or DeFi, represents a paradigm shift in the world of finance. It aims to recreate and improve upon traditional financial systems using blockchain technology, primarily built on platforms like Ethereum. At the heart of DeFi lie smart contracts - self-executing pieces of code that automate financial transactions and agreements without the need for intermediaries.
Smart contracts are the building blocks of DeFi applications. They enable trustless, transparent, and efficient financial operations, from simple token exchanges to complex lending and borrowing protocols. By removing intermediaries and automating processes, smart contracts reduce costs, increase speed, and enhance accessibility to financial services.
Before we dive into specific DeFi concepts, it's crucial to understand that smart contracts play a central role in each of these areas. They define the rules, manage the processes, and ensure the security and integrity of DeFi protocols.
Key DeFi Concepts and the Role of Smart Contracts
1. Decentralized Exchange (DEX)
Definition: A DEX is a platform where users can trade cryptocurrencies without a central authority.
Role of Smart Contracts: DEX smart contracts manage the entire trading process, from order matching to trade execution and liquidity provision.
Example:
contract SimpleSwap {
mapping(address => mapping(address => uint)) public liquidity;
function swap(address fromToken, address toToken, uint amountIn) external returns (uint amountOut) {
require(liquidity[fromToken][toToken] > 0, "Insufficient liquidity");
amountOut = (amountIn * liquidity[toToken][fromToken]) / liquidity[fromToken][toToken];
// Transfer tokens
IERC20(fromToken).transferFrom(msg.sender, address(this), amountIn);
IERC20(toToken).transfer(msg.sender, amountOut);
// Update liquidity
liquidity[fromToken][toToken] += amountIn;
liquidity[toToken][fromToken] -= amountOut;
return amountOut;
}
}
This simplified DEX contract allows users to swap tokens based on the available liquidity.
2. Liquidity Pool
Definition: Liquidity pools are smart contracts holding reserves of two or more tokens, enabling trading on DEXs and other DeFi protocols.
Role of Smart Contracts: These contracts manage token deposits, withdrawals, and the exchange of tokens within the pool.
Example:
contract LiquidityPool {
mapping(address => uint) public balances;
uint public totalLiquidity;
function addLiquidity(uint amount) external {
IERC20(tokenAddress).transferFrom(msg.sender, address(this), amount);
balances[msg.sender] += amount;
totalLiquidity += amount;
}
function removeLiquidity(uint amount) external {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
totalLiquidity -= amount;
IERC20(tokenAddress).transfer(msg.sender, amount);
}
}
This basic liquidity pool contract allows users to add and remove liquidity.
3. Yield Farming
Definition: Yield farming involves lending or staking cryptocurrency assets to generate returns.
Role of Smart Contracts: These contracts automate the process of depositing assets, calculating rewards, and distributing them to participants.
Example:
contract YieldFarm {
mapping(address => uint) public stakedBalance;
mapping(address => uint) public lastUpdateTime;
uint public rewardRate = 1; // 1 token per second per staked token
function stake(uint amount) external {
updateReward(msg.sender);
IERC20(stakingToken).transferFrom(msg.sender, address(this), amount);
stakedBalance[msg.sender] += amount;
lastUpdateTime[msg.sender] = block.timestamp;
}
function getReward() external {
updateReward(msg.sender);
uint reward = calculateReward(msg.sender);
IERC20(rewardToken).transfer(msg.sender, reward);
}
function updateReward(address account) internal {
uint reward = calculateReward(account);
stakedBalance[account] += reward;
lastUpdateTime[account] = block.timestamp;
}
function calculateReward(address account) internal view returns (uint) {
return (block.timestamp - lastUpdateTime[account]) * stakedBalance[account] * rewardRate;
}
}
This yield farming contract allows users to stake tokens and earn rewards over time.
4. Gas Fees
Definition: Gas fees are the costs associated with performing transactions or executing smart contracts on the Ethereum network.
Role of Smart Contracts: While not directly managed by smart contracts, efficient contract design can significantly impact gas costs for users.
Example: Optimizing a function to use less gas:
// Expensive
function expensiveFunction() public {
for (uint i = 0; i < array.length; i++) {
// Do something
}
}
// Optimized
function optimizedFunction() public {
uint length = array.length;
for (uint i = 0; i < length; i++) {
// Do something
}
}
By storing the array length in a variable, we reduce the number of storage reads, thus saving gas.
5. Staking
Definition: Staking involves locking up cryptocurrency to support network operations and earn rewards.
Role of Smart Contracts: Staking contracts manage the locking of assets, validation of network activities, and distribution of rewards.
Example:
contract Staking {
mapping(address => uint) public stakedBalance;
mapping(address => uint) public stakingTime;
function stake(uint amount) external {
require(amount > 0, "Cannot stake 0");
IERC20(stakingToken).transferFrom(msg.sender, address(this), amount);
stakedBalance[msg.sender] += amount;
stakingTime[msg.sender] = block.timestamp;
}
function unstake() external {
require(stakedBalance[msg.sender] > 0, "No tokens staked");
uint reward = calculateReward(msg.sender);
uint amount = stakedBalance[msg.sender];
stakedBalance[msg.sender] = 0;
IERC20(stakingToken).transfer(msg.sender, amount);
IERC20(rewardToken).transfer(msg.sender, reward);
}
function calculateReward(address user) internal view returns (uint) {
return (block.timestamp - stakingTime[user]) * stakedBalance[user] * rewardRate;
}
}
This staking contract allows users to stake tokens and earn rewards based on the duration of staking.
6. Oracle
Definition: Oracles provide external data to smart contracts, crucial for many DeFi applications.
Role of Smart Contracts: Oracle contracts act as a bridge between blockchain and off-chain data sources, ensuring DeFi protocols have accurate, up-to-date information.
Example:
contract PriceOracle {
address public admin;
mapping(address => uint) public prices;
constructor() {
admin = msg.sender;
}
function updatePrice(address token, uint price) external {
require(msg.sender == admin, "Only admin can update prices");
prices[token] = price;
}
function getPrice(address token) external view returns (uint) {
return prices[token];
}
}
This simple Oracle contract allows an admin to update prices and users to fetch the latest price for a token.
7. Liquidity Mining
Definition: Liquidity mining is a subset of yield farming where users provide liquidity to a protocol and earn rewards in return.
Role of Smart Contracts: These contracts manage the distribution of rewards to liquidity providers based on their contribution to the pool.
Example:
contract LiquidityMining {
mapping(address => uint) public userLiquidity;
uint public totalLiquidity;
uint public rewardPerBlock = 1e18; // 1 token per block
function provideLiquidity(uint amount) external {
IERC20(lpToken).transferFrom(msg.sender, address(this), amount);
userLiquidity[msg.sender] += amount;
totalLiquidity += amount;
}
function withdrawLiquidity(uint amount) external {
require(userLiquidity[msg.sender] >= amount, "Insufficient liquidity");
userLiquidity[msg.sender] -= amount;
totalLiquidity -= amount;
IERC20(lpToken).transfer(msg.sender, amount);
}
function claimRewards() external {
uint reward = (userLiquidity[msg.sender] * rewardPerBlock) / totalLiquidity;
IERC20(rewardToken).transfer(msg.sender, reward);
}
}
This liquidity mining contract allows users to provide liquidity and earn rewards proportional to their share of the pool.
8. Token Swap
Definition: Token swaps allow users to exchange one cryptocurrency for another directly.
Role of Smart Contracts: Swap contracts manage the exchange process, determining exchange rates and executing the transfer of tokens.
Example:
contract TokenSwap {
function swap(address fromToken, address toToken, uint amount) external {
require(IERC20(fromToken).transferFrom(msg.sender, address(this), amount), "Transfer failed");
uint swapAmount = calculateSwapAmount(fromToken, toToken, amount);
require(IERC20(toToken).transfer(msg.sender, swapAmount), "Swap failed");
}
function calculateSwapAmount(address fromToken, address toToken, uint amount) internal view returns (uint) {
// In a real implementation, this would use an oracle or liquidity pool to determine the exchange rate
return amount; // Simplified 1:1 swap for this example
}
}
This basic token swap contract allows users to swap one token for another.
9. Gas Limit
Definition: The gas limit is the maximum amount of gas you're willing to spend on a transaction.
Role of Smart Contracts: While not directly set by smart contracts, understanding gas limits is crucial for interacting with DeFi protocols efficiently.
Example:
contract GasAware {
uint public constant MAX_GAS_LIMIT = 300000;
function gasIntensiveOperation() external {
require(gasleft() >= MAX_GAS_LIMIT, "Insufficient gas");
// Perform gas-intensive operation
}
}
This contract ensures that a function is only called with sufficient gas to complete its operation.
Conclusion
Smart contracts are the cornerstone of DeFi, enabling trustless, automated financial operations. As a beginner, understanding these concepts and their relationship to smart contracts is crucial for navigating the DeFi landscape. While DeFi offers exciting opportunities, it's important to approach it with caution, understand the risks involved, and always do thorough research before participating in any DeFi protocol.
Remember, the examples provided here are simplified for illustrative purposes. Real-world DeFi smart contracts are much more complex and require rigorous security measures to protect users' funds.
Subscribe to my newsletter
Read articles from Manojkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
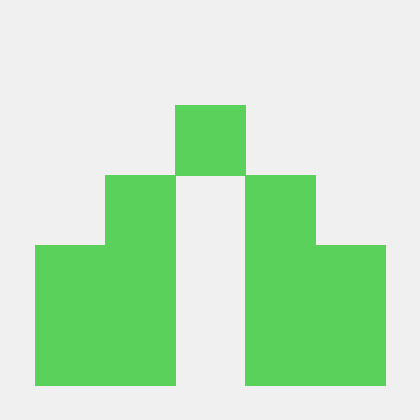