Building a Simple Cat Photo App with HTML:

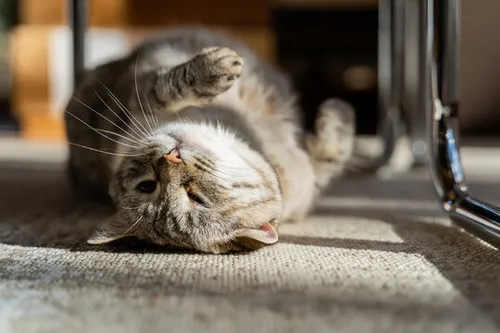
Introduction
As a developer, sometimes you need a fun project to break the monotony of complex coding tasks. That’s where the Cat Photo App comes in! Today, I'll walk you through how I created a simple yet adorable cat photo application using pure HTML.
In this guide, I'll cover the main features of the Cat Photo App and show you the importance of structuring your code with clean comments to make it understandable and reusable.
Why Build a Cat App?
First things first—why cats? Well, why not! The internet loves cats, and a Cat Photo App is a perfect way to practice HTML, especially when starting as a web developer. You get to focus on the essentials of page structure, forms, and media, all while working on a fun project that can be expanded upon later.
Setting Up the Basic Structure
For this project, I used pure HTML. Here’s the breakdown of what we’re building:
A simple homepage with a title and a main header.
A section showcasing cat photos.
Lists displaying what cats love and hate (spoiler: they hate other cats).
A form where users can submit details about their own cats.
Let’s start with the structure of our HTML document:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>CatPhotoApp</title>
</head>
<body>
<main>
<h1>CatPhotoApp</h1>
<!-- Rest of the code goes here -->
</main>
<footer>
<p>No Copyright - <a href="https://www.freecodecamp.org">freeCodeCamp.org</a></p>
</footer>
</body>
</html>
<!--This simple structure contains the essential elements for the app.
The DOCTYPE declaration defines the HTML version,
the <meta> tag specifies character encoding, and the <title> tag defines the name
that appears in the browser tab.-->
Adding Cat Photos
The heart of the Cat Photo App is, of course, the photos. Here’s how I added a section to display them:
<section> <h2>Cat Photos</h2> <p>See more <a target="_blank" href="
https://freecatphotoapp.com">cat
photos</a> in our gallery.</p> <a href="
https://freecatphotoapp.com
"> <img src="
https://cdn.freecodecamp.org/curriculum/cat-photo-app/relaxing-cat.jpg
" alt="A cute orange cat lying on its back."> </a> </section>
By using an <a>
tag, I linked to a gallery of cat photos and displayed a cute image using the <img>
tag. The alt
attribute ensures accessibility, providing a description of the image for those using screen readers.
Displaying What Cats Love and Hate
To add some personality to the app, I included two lists: one for things cats love, and one for things they hate. Here’s the HTML:
htmlCopy code<section>
<h2>Cat Lists</h2>
<h3>Things cats love:</h3>
<ul>
<li>cat nip</li>
<li>laser pointers</li>
<li>lasagna</li>
</ul>
<h3>Top 3 things cats hate:</h3>
<ol>
<li>flea treatment</li>
<li>thunder</li>
<li>other cats</li>
</ol>
</section>
Notice the use of both <ul>
for unordered lists and <ol>
for ordered lists. This makes it easy to distinguish between loves and hates. To add a bit of fun, I included a photo of lasagna and made a light-hearted comment about how much cats love it!
Adding User Interaction with Forms
Finally, I added a form where users can submit their cat's details. This form uses two <fieldset>
elements for grouping related fields, making it more organized and accessible:
htmlCopy code<section>
<h2>Cat Form</h2>
<form action="https://freecatphotoapp.com/submit-cat-photo">
<fieldset>
<legend>Is your cat an indoor or outdoor cat?</legend>
<label><input type="radio" name="indoor-outdoor" value="indoor" checked> Indoor</label>
<label><input type="radio" name="indoor-outdoor" value="outdoor"> Outdoor</label>
</fieldset>
<fieldset>
<legend>What's your cat's personality?</legend>
<input type="checkbox" name="personality" value="loving" checked> Loving
<input type="checkbox" name="personality" value="lazy"> Lazy
<input type="checkbox" name="personality" value="energetic"> Energetic
</fieldset>
<input type="text" name="catphotourl" placeholder="cat photo URL" required>
<button type="submit">Submit</button>
</form>
</section>
The form allows users to select whether their cat is an indoor or outdoor cat and its personality traits. Additionally, they can submit a URL to a photo of their cat. The form action links to a fictional service that would handle the form submission.
Conclusion
The Cat Photo App is a great way to learn HTML basics while having fun. This project teaches you how to structure a web page with headings, lists, images, and forms, all of which are key elements in creating user-friendly websites.
If you want to expand the project, consider adding JavaScript to make the form interactive or CSS to style the app. The possibilities are endless!
Feel free to check out the source code and let me know if you have any suggestions!
http://127.0.0.1:3000/d:/complete web devlopment/THECATAPP.html
Subscribe to my newsletter
Read articles from Arpit Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arpit Verma
Arpit Verma
B.Tech Computer Science Student | Tech Blogger | Aspiring Full-Stack Developer Currently pursuing B.Tech in Computer Science at BBD University, I'm passionate about learning different programming languages and sharing my journey through tech blogs. From coding tutorials to small full-stack projects, I document everything as I grow in the field. Always eager to explore new technologies and help others learn along the way.