Channels in Go
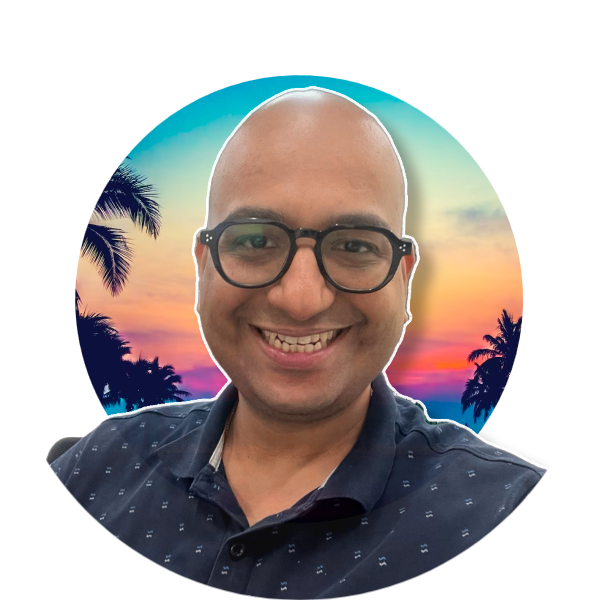
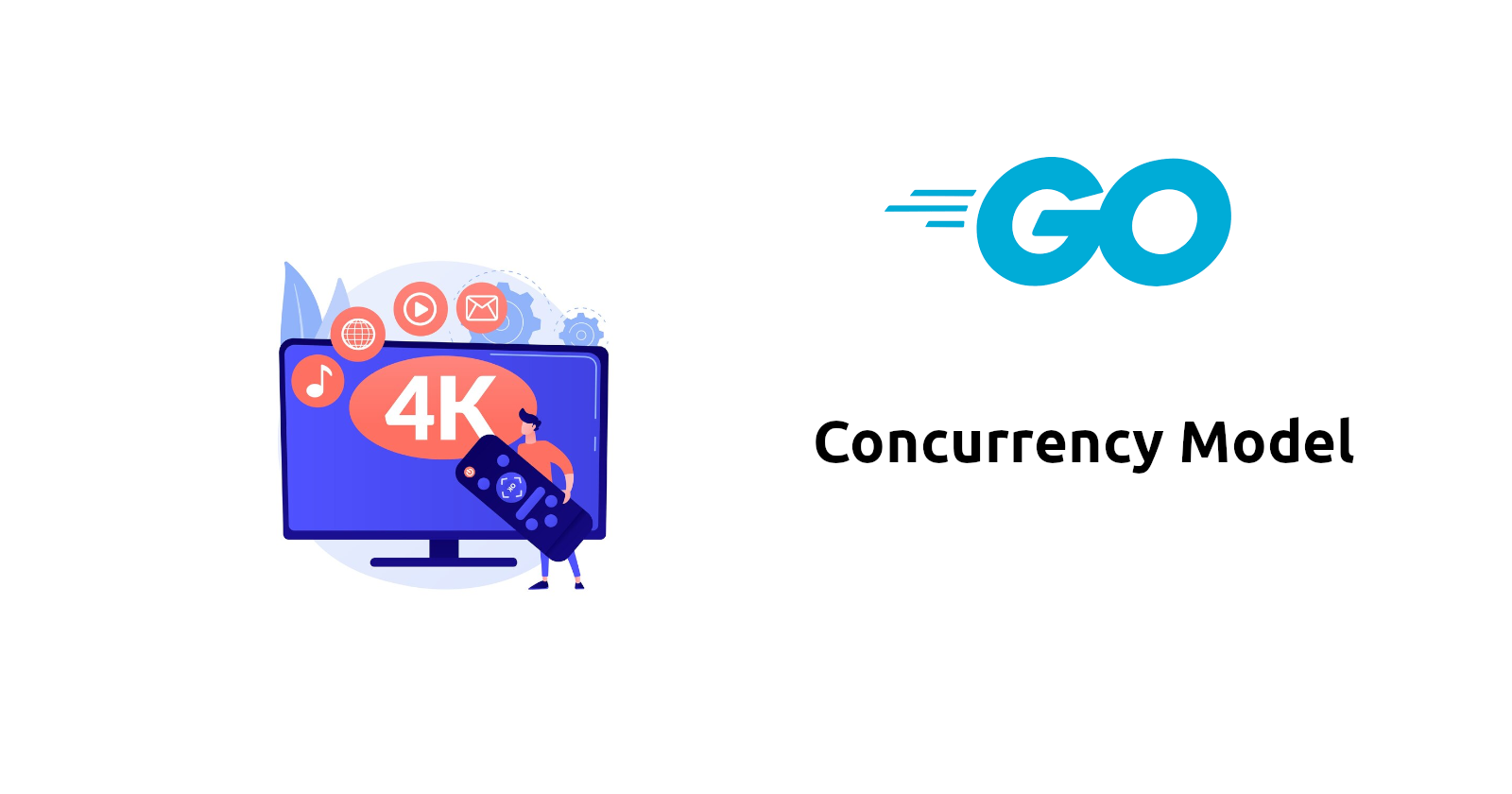
Introduction
The Channels Model is another approach to handling concurrency, commonly associated with the Communicating Sequential Processes (CSP) paradigm. It is prominently used in languages like Go and Clojure (with core.async). In this model, channels are the primary means of communication between concurrent entities (like goroutines in Go).
Key Concepts of the Channels Model
Goroutines (in Go):
Goroutines are lightweight threads managed by the Go runtime.
They run concurrently and can execute functions independently of each other.
Channels:
Channels are conduits through which goroutines can communicate by sending and receiving messages.
Channels are typed, meaning that a channel can only transmit data of a specific type (e.g.,
chan int
for integers).Communication over channels can be synchronous or buffered:
Synchronous channels block the sender until the receiver is ready to receive.
Buffered channels allow a limited amount of data to be sent without blocking, until the buffer is full.
Communication via Channels:
Goroutines communicate by passing messages through channels. This allows for coordination and synchronization without explicit locking mechanisms.
The sending and receiving of messages over channels form the basis for synchronization, making it easier to reason about shared data without the risk of race conditions.
Select Statement:
Go provides a
select
statement that allows a goroutine to wait on multiple channel operations, handling whichever one is ready first.This is akin to a switch statement for channels and is a powerful feature for managing complex concurrency scenarios.
Example: Simple Channels in Go
Here's a basic example in Go:
package main
import (
"fmt"
)
func main() {
messages := make(chan string)
go func() {
messages <- "Hello, Channel!"
}()
msg := <-messages
fmt.Println(msg)
}
In this example:
A channel
messages
is created.A goroutine is spawned that sends
"Hello, Channel!"
through themessages
channel.The main function receives this message and prints it.
Comparison with the Actor Model
Both the Actor Model and Channels Model are powerful paradigms for handling concurrency, but they differ significantly in how they approach communication, state management, and error handling.
Feature | Actor Model | Channels Model |
Concurrency Approach | Actor-based: Independent actors communicate via messages. | CSP-based: Goroutines communicate via channels. |
State Management | Encapsulated within actors; no shared state. | State can be shared via channels, but typically encapsulated within goroutines. |
Communication | Asynchronous message passing between actors. | Synchronous or buffered communication via channels. |
Error Handling | Often uses supervisors to monitor and recover from actor failures. | Typically managed by the programmer, with no built-in supervision model. |
Fault Tolerance | High, due to actor isolation and supervisor strategies. | Depends on implementation; not inherently fault-tolerant. |
Concurrency Granularity | Fine-grained, each actor is a separate unit of concurrency. | Coarse-grained, with channels coordinating goroutines. |
Scalability | High, especially in distributed systems (Erlang, Elixir). | High, with efficient lightweight threads (goroutines) in Go. |
Use Cases | Distributed systems, fault-tolerant applications, telecoms. | Concurrent applications, parallel processing, cloud services. |
Key Differences
State and Isolation:
In the Actor Model, each actor maintains its own private state, and there is no shared memory between actors. This strong isolation simplifies reasoning about concurrency and makes the system more resilient to faults.
In the Channels Model, goroutines may share state, but channels provide a structured way to communicate and synchronize changes to that state. This model requires careful handling to avoid race conditions but offers more flexibility.
Communication:
The Actor Model uses asynchronous message passing, where each actor can decide how to handle messages (e.g., by changing state, sending new messages, or creating new actors).
The Channels Model uses synchronous or buffered communication, where one goroutine sends data into a channel and another goroutine receives it. This model can be simpler to reason about in some scenarios but may require explicit coordination to manage state and handle complex concurrency patterns.
Error Handling and Fault Tolerance:
The Actor Model often includes built-in mechanisms for fault tolerance, such as supervisors that can restart failed actors. This makes it particularly well-suited for building highly reliable systems.
The Channels Model does not inherently include fault tolerance mechanisms. Error handling is typically the responsibility of the programmer, and recovery strategies must be implemented manually.
Scalability and Performance:
Both models are highly scalable, but they shine in different areas. The Actor Model is particularly effective in distributed systems where the isolation of actors and fault tolerance are crucial.
The Channels Model excels in scenarios where lightweight concurrency (like thousands of goroutines) is needed, with Go's runtime efficiently managing these goroutines.
When to Use Which Model?
Actor Model:
Best for systems requiring high fault tolerance and reliability, such as telecom systems, real-time messaging platforms, and distributed systems.
Ideal when you want strong guarantees about isolation and the ability to recover from failures automatically.
Channels Model:
Best for systems requiring fine-grained concurrency and parallelism, such as web servers, parallel processing tasks, or any system where lightweight concurrency primitives (like goroutines) are advantageous.
Ideal when you need a simple, structured way to communicate between concurrent tasks without needing the full isolation and fault tolerance provided by the Actor Model.
In summary, both models offer robust approaches to concurrency, but they are suited to different types of problems. The Actor Model is more focused on isolation, fault tolerance, and message-driven design, while the Channels Model offers a straightforward way to handle communication and synchronization between lightweight concurrent tasks.
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
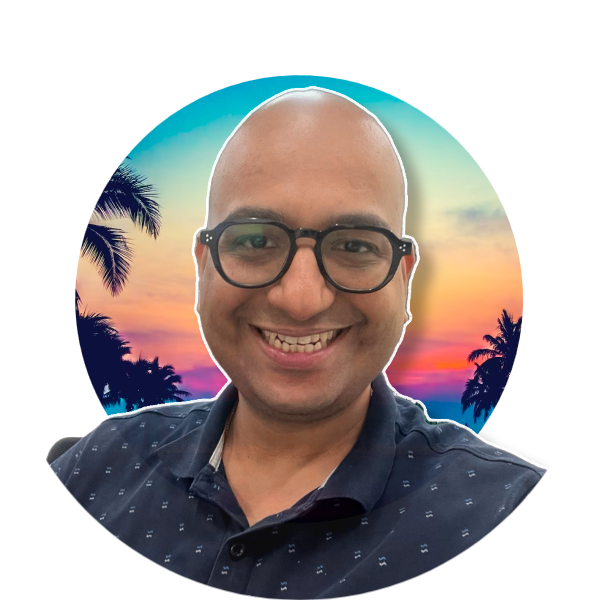
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.