Important String Methods in Javascript
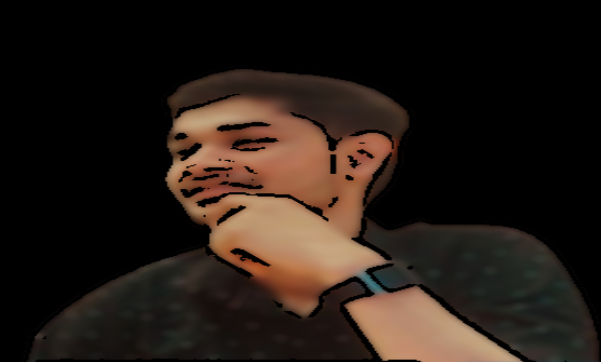
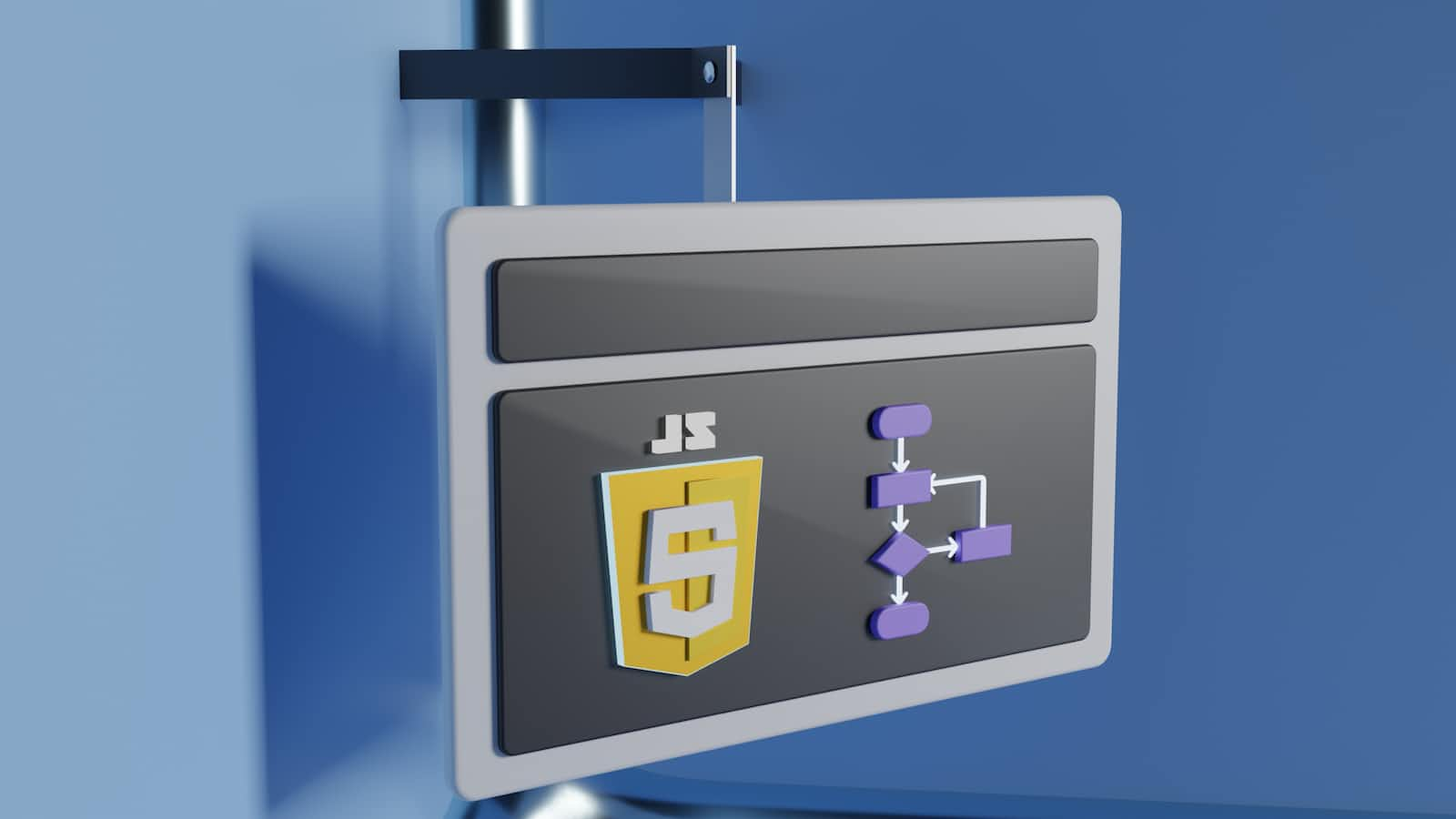
So let us start by creating a string, there are various ways to do this but here i am using Object.
let val = new String("string-metHODs-in-js ");
The way we are going to learn this method is first I will describe what particular method is used for, then provide the code with an example.
length()
This is one of the most commonly used methods. It provides the length/size of the string.
console.log(val.length);
// output
// 20
substring()
The substring()
method in JavaScript is used to extract a portion of a string from a given starting index to a specified ending index and returns a new string containing that extracted portion. Remember that the end index is not included.
We also have a similar method substr()
but the difference is that it takes two arguments one is startindex
and the second is the length
of the portion you want to extract.
NOTE: If we put a negative value in startIndex it will start from 0 only.
console.log(val.substring(2, 8));
// output
// ring-m
console.log(val.substr(2,8));
// output
// ring-met
slice()
The slice()
method in JavaScript is used to extract a portion of a string or an array. It can take one or two arguments that define the range of elements to be extracted. While similar to the substring()
method, slice()
introduces the ability to use negative values as indices.
When a negative value is used, slice()
calculates the index from the end of the string or array. However, it's important to note that the approach for negative indices in slice()
differs from regular indexing.
Here's an example for better clarity:
console.log(val.slice(2,8));
// output
// ring-m
console.log(val.slice(-8));
// output
// Ds-in-js
toLowerCase()/toUpperCase()
These methods are especially useful for tasks like comparing strings in a case-insensitive manner or ensuring consistent formatting of user input or data. Keep in mind that the original string remains unchanged, and the result is a new string with the requested case (lowercase or uppercase).
console.log(val.toLowerCase());
// output
// string-metHODs-in-js
console.log(val.toLowerCase());
// output
// STRING-METHODS-IN-JS
charAt()
The charAt()
method is used to retrieve the character at a specified index within a string. It takes a single parameter, which is the index of the character you want to retrieve.
console.log(val.charAt(3));
// output
// i
indexOf()
The indexOf()
method returns the index of the first occurrence of a specified value or substring. It returns -1
if the value is not found.
console.log(val.indexOf("i"));
// output
// 3
console.log(val.indexOf("-in-"));
// output
// 14
console.log(val.indexOf("park"));
// output
// -1
replace()/replaceAll()
The replace()
method in JavaScript is used to replace a specified substring or pattern in a string with another substring or value. This method operates on a string and returns a new string with the replacements made. It doesn't modify the original string.
To replace all the occurrences of particular substring in the string we use replaceAll()
method.
console.log(val.replace("-", "_"));
// output
// string_metHODs-in-js
console.log(val.replaceAll("-", "_"));
// output
// string_metHODs_in_js
split()
The split()
method in JavaScript is used to split a string into an array of substrings based on a specified separator. This separator can be anything. The method does not modify the original string but instead returns a new array containing the substrings.
console.log(val.split("-"));
// output
// ['string', 'metHODs', 'in', 'js ']
trim()
The trim()
method is used to remove whitespace characters from both ends of a string. Whitespace characters include spaces, tabs, and newline characters. The method returns a new string with the leading and trailing whitespace removed, without modifying the original string, we can also use trimEnd(), trimStart()
for more controlled trimming.
console.log(val.trim());
// output
// string-metHODs-in-js
search()
The search()
method searches for a specified pattern or regular expression within a string and returns the index of the first match or -1
if no match is found. The search()
method is similar to indexOf()
, but it allows the use of regular expressions for more flexible pattern-matching.
It's important to note that the search()
method searches for the first occurrence only. If you want to find all occurrences, you might want to use the match()
method with the global (g
) flag on a regular expression.
console.log(val.search("i"));
// output
// 3
console.log(val.match(/i/g));
// output
// ['i', 'i']
includes()
The includes()
method checks if a string contains a specified substring and returns true
or false
.. The includes()
method is case-sensitive, meaning it distinguishes between uppercase and lowercase characters.
console.log(val.includes("i"));
// output
// true
JavaScript strings come with a rich set of methods that allow for powerful and flexible manipulation of text. From extracting substrings to transforming cases and searching patterns, these methods are essential tools for any JavaScript developer. These are some of the most commonly used methods. For more detailed information, consider exploring the MDN documentation.
Subscribe to my newsletter
Read articles from Sahil Saini directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
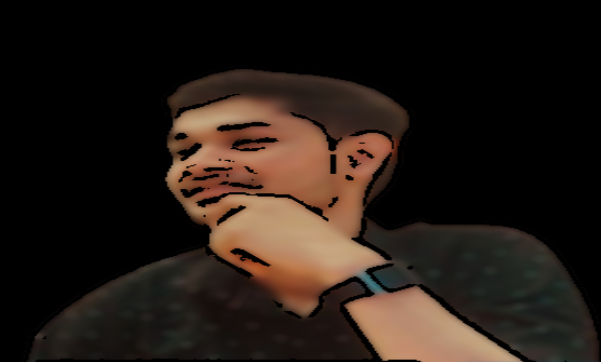
Sahil Saini
Sahil Saini
I'm Sahil Saini, a Full Stack Developer, Technical Writer, and a passionate learner. I am pursuing B.Tech in Chemical Engineering, IIT (BHU) Varanasi, India. I have worked on a wide range of technologies and have worked on projects ranging from small to large scale. I am a self-taught and self-motivated individual who is always looking for new challenges and opportunities. I love contributing to open-source projects.