Day 26 Task: Jenkins Declarative Pipeline

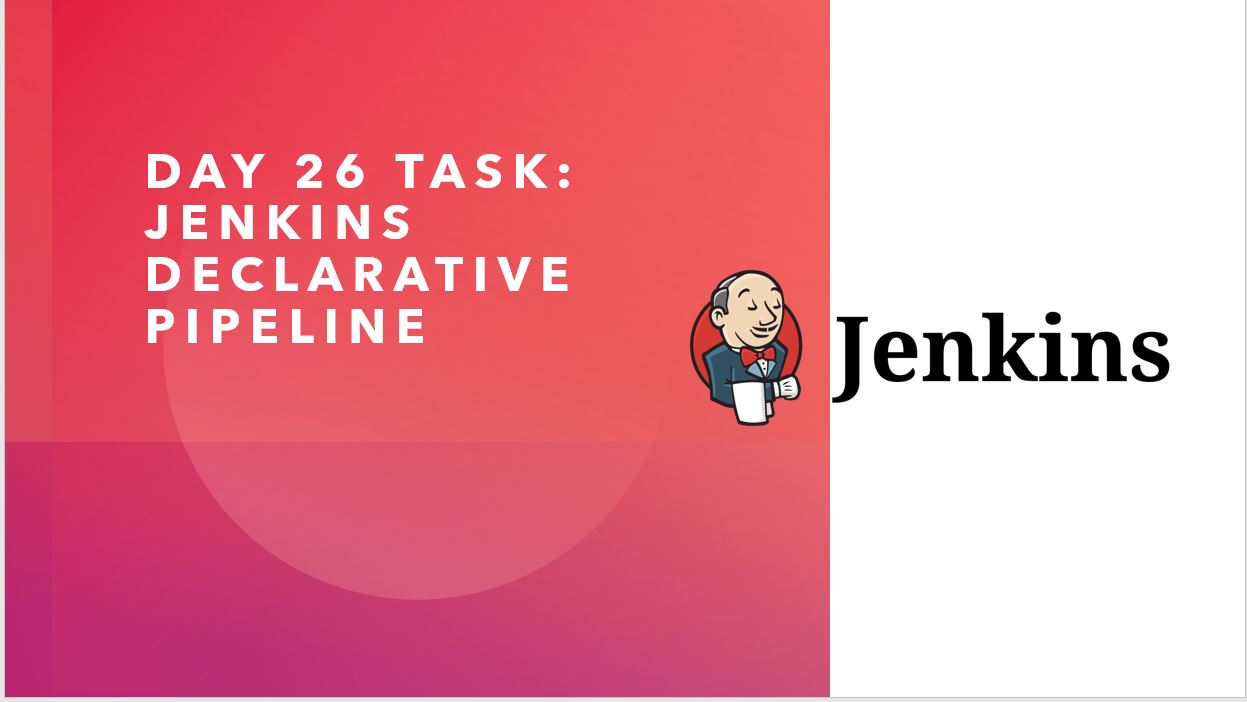
What is Pipeline
A pipeline is a collection of steps or jobs interlinked in a sequence.
Declarative:
Declarative is a more recent and advanced implementation of a pipeline as a code.
Scripted:
Scripted was the first and most traditional implementation of the pipeline as a code in Jenkins. It was designed as a general-purpose DSL (Domain Specific Language) built with Groovy.
Why you should have a Pipeline
The definition of a Jenkins Pipeline is written into a text file (called a Jenkinsfile
) which in turn can be committed to a project’s source control repository.
This is the foundation of "Pipeline-as-code"; treating the CD pipeline as a part of the application to be versioned and reviewed like any other code.
Creating a Jenkinsfile
and committing it to source control provides a number of immediate benefits:
Automatically creates a Pipeline build process for all branches and pull requests.
Code review/iteration on the Pipeline (along with the remaining source code).
Pipeline syntax
pipeline {
agent any
stages {
stage('Build') {
steps {
//
}
}
stage('Test') {
steps {
//
}
}
stage('Deploy') {
steps {
//
}
}
}
}
Task-01
Create a New Job, this time select Pipeline instead of Freestyle Project.
Follow the Official Jenkins Hello world example
Complete the example using the Declarative pipeline
pipeline {
agent {
node {
label 'dev-server'
}
}
stages {
stage('Hello') {
steps {
echo 'Hello World'
}
}
}
}
Output -
Console Output -
Stages Output :
Declarative vs Scripted Pipeline – Key differences
Declarative Pipeline:
The Declarative Pipeline syntax is designed to provide a more structured and easier-to-read approach, with predefined blocks and limited flexibility.
Syntax: Declarative pipelines have a predefined, structured syntax, making it simpler and easier to read.
Error handling: Built-in mechanisms for handling errors and failures (e.g.,
post
blocks).Pipeline structure: Defined inside a
pipeline
block. It enforces a well-defined structure, such asstages
,steps
,post
, etc.Use case: Preferred for simple to moderately complex pipelines where readability and simplicity are prioritized.
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
}
}
stage('Test') {
steps {
echo 'Testing...'
}
}
stage('Deploy') {
steps {
echo 'Deploying...'
}
}
}
post {
always {
echo 'This will run after every stage.'
}
}
}
Scripted Pipeline:
The Scripted Pipeline is more flexible and powerful, as it allows the full use of Groovy syntax. However, it is more complex to write and maintain.
Syntax: Uses Groovy scripting, which provides maximum flexibility, but can be harder to read and maintain.
Error handling: Manual error handling, requiring custom code for error scenarios.
Pipeline structure: More flexible structure, not bound by strict blocks. You can define custom logic, loops, and conditions freely.
Use case: Best for complex pipelines where flexibility is essential and you need more control over the execution flow.
node {
try {
stage('Build') {
echo 'Building...'
}
stage('Test') {
echo 'Testing...'
}
stage('Deploy') {
echo 'Deploying...'
}
} catch (Exception e) {
echo 'Pipeline failed: ' + e.toString()
} finally {
echo 'This will always run after the pipeline stages.'
}
}
Follow for more updates:)
Subscribe to my newsletter
Read articles from Vibhuti Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vibhuti Jain
Vibhuti Jain
Hi, I am Vibhuti Jain. A Devops Tools enthusiastic who keens on learning Devops tools and want to contribute my knowledge to build a community and collaborate with them. I have a acquired a knowledge of Linux, Shell Scripting, Python, Git, GitHub, AWS Cloud and ready to learn a lot more new Devops skills which help me build some real life project.