Boost Node.js Security: A Guide to Two-Factor Authentication

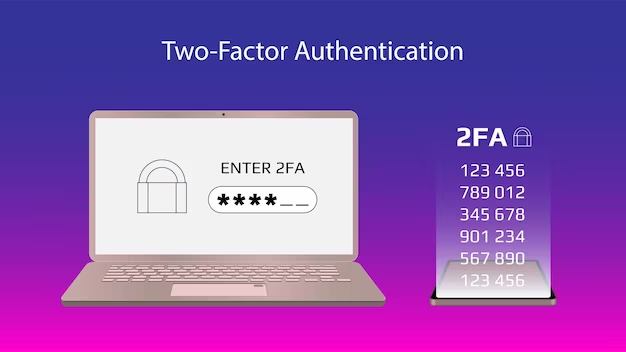
Introduction
Explanation of Two-Factor Authentication (2FA)
Two-Factor Authentication (2FA) is a security process that enhances the protection of your online accounts by requiring two different forms of identification before granting access. This method adds an extra layer of security beyond just a username and password.
When you enable 2FA, you will typically be asked to provide something you know (like your password) and something you have (such as a smartphone or a hardware token). For instance, after entering your password, you might receive a code on your phone that you need to input to complete the login process.
There are various methods of 2FA, including:
SMS-based 2FA: A code is sent to your mobile phone via text message.
Authenticator Apps: Apps like Google Authenticator or Authy generate time-based codes.
Hardware Tokens: Physical devices like YubiKey that generate or store authentication codes.
Biometric Verification: Using fingerprints or facial recognition as the second factor.
By requiring two forms of verification, 2FA significantly reduces the risk of unauthorized access, even if your password is compromised. This makes it much harder for attackers to gain access to your accounts, thereby providing a more secure online experience.
Importance and Benefits of 2FA
Increased Security: Even if your password is stolen, 2FA makes it much harder for attackers to access your account.
Multiple Verification Methods: You can use SMS codes, authenticator apps, hardware tokens, or biometric verification to secure your account.
Reduced Risk of Unauthorized Access: With two forms of verification, the chances of someone else gaining access to your account are significantly lower.
Enabling 2FA is a simple yet effective way to ensure a more secure online experience.
Overview of Implementing 2FA with Node.js
Implementing Two-Factor Authentication (2FA) in a Node.js application is a crucial step towards enhancing the security of your user accounts. By adding an extra layer of security, you can protect your users from unauthorized access, even if their passwords are compromised. Here, we will explore the steps and considerations involved in integrating 2FA into your Node.js application.
Step 1: Choose Your 2FA Method
First, decide which type of 2FA you want to implement. Common methods include:
SMS-based 2FA: Send a verification code to the user's mobile phone via SMS.
Authenticator Apps: Use apps like Google Authenticator or Authy to generate time-based one-time passwords (TOTPs).
Hardware Tokens: Utilize physical devices like YubiKey that generate or store authentication codes.
Biometric Verification: Implement fingerprint or facial recognition as the second factor.
Step 2: Set Up Your Node.js Environment
Ensure your Node.js environment is set up and ready for development. You will need to install necessary packages and dependencies. For example, if you are using an authenticator app, you might need the speakeasy
and qrcode
packages:
npm install speakeasy qrcode
Step 3: Generate and Store 2FA Secrets
For methods like authenticator apps, you will need to generate a secret key for each user. This key will be used to generate the time-based codes. Here’s an example using the speakeasy
package:
const speakeasy = require('speakeasy');
// Generate a secret key
const secret = speakeasy.generateSecret({ length: 20 });
console.log(secret.base32); // Save this secret in your database
Step 4: Verify 2FA Codes
When the user logs in, you will need to verify the 2FA code they provide. This can be done using the speakeasy
package as well:
const verified = speakeasy.totp.verify({
secret: user.secret, // Retrieve the user's secret from the database
encoding: 'base32',
token: userToken // The token provided by the user
});
if (verified) {
// Grant access
} else {
// Deny access
}
Step 5: Integrate with Your Authentication Flow
Integrate the 2FA verification step into your existing authentication flow. After the user enters their username and password, prompt them for the 2FA code. Only grant access if both the password and the 2FA code are correct.
Step 6: Provide Backup Options
Consider providing backup options for users who may lose access to their 2FA method. This could include backup codes, email verification, or support for multiple 2FA methods.
Prerequisites
Basic understanding of Node.js and JavaScript
Node.js and npm installed
Additional tools and libraries (e.g., Express, Speakeasy, etc.)
Setting Up the Node.js Project
Initializing a New Node.js Project
To get started with setting up your Node.js project, follow these detailed steps:
Create a New Directory: First, create a new directory for your project. This will help keep all your project files organized in one place. Open your terminal and run the following command:
mkdir my-2fa-project cd my-2fa-project
Initialize the Project: Next, initialize a new Node.js project within this directory. This will create a
package.json
file, which will keep track of your project's dependencies and scripts. Run:npm init -y
The
-y
flag automatically answers "yes" to all prompts, creating apackage.json
with default settings. You can manually edit this file later if needed.Install Required Dependencies: Now, install the necessary packages for your project. For this example, you'll need Express for setting up a web server and Speakeasy for handling 2FA. Run:
npm install express speakeasy
This command will download and add these packages to your project, updating your
package.json
file accordingly.Create Basic Project Structure: To keep your project organized, create a basic folder structure. At a minimum, you should have separate folders for your routes and any middleware. Run:
mkdir routes touch routes/index.js
Set Up Express Server: In your project root, create a file named
server.js
to set up your Express server. Add the following code to this file:const express = require('express'); const app = express(); const port = 3000; app.use(express.json()); app.get('/', (req, res) => { res.send('Welcome to the 2FA project!'); }); app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Configure Routes: Open the
routes/index.js
file and set up a basic route. This is where you'll later add your 2FA logic. For now, just add a simple route:const express = require('express'); const router = express.Router(); router.get('/', (req, res) => { res.send('This is the main route.'); }); module.exports = router;
Link Routes to Server: Modify your
server.js
file to use the routes you just created. Update it as follows:const express = require('express'); const app = express(); const port = 3000; const indexRouter = require('./routes/index'); app.use(express.json()); app.use('/', indexRouter); app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Run the Server: Finally, start your server to ensure everything is set up correctly. In your terminal, run:
node server.js
You should see a message indicating that the server is running. Open your browser and navigate to
http://localhost:3000
to see the welcome message.
By following these steps, you have successfully initialized a new Node.js project and set up a basic Express server. You are now ready to integrate 2FA functionality into your authentication flow.
Installing necessary packages
Speakeasy
Now that npm is initialized, you need to install the necessary packages for your project. For this example, you'll need Express for your server and Speakeasy for handling two-factor authentication (2FA). Run the following command to install these packages:
npm install speakeasy
Speakeasy is a library that provides easy-to-use methods for generating and verifying one-time passwords (OTPs) for 2FA. It supports various algorithms and is compatible with popular authenticator apps like Google Authenticator.
In addition to Speakeasy, you might need other relevant libraries depending on your project's requirements. For example, you might want to use dotenv
to manage environment variables, mongoose
for MongoDB integration, or jsonwebtoken
for handling JSON Web Tokens (JWTs). Install any additional libraries you need by running:
npm install dotenv mongoose jsonwebtoken
With your project directory set up and the necessary packages installed, you are now ready to start building your authentication flow with 2FA. The next steps involve creating routes, linking them to your server, and integrating 2FA logic using Speakeasy.
Creating the 2FA System
Setting Up User Authentication (Optional brief overview or link to existing setup)
Generating Secret Keys for Users
To implement two-factor authentication (2FA) in your application, the first step is to generate a secret key for each user. This secret key will be used to create time-based one-time passwords (TOTPs) that the user will enter during the authentication process.
Step 1: Import Speakeasy
First, ensure that you have imported the Speakeasy library into your project. You should have already installed it using the command mentioned earlier. Now, include it in your code:
const speakeasy = require('speakeasy');
Step 2: Generate a Secret Key
Next, you need to generate a unique secret key for each user. This key will be stored securely in your database and used to generate OTPs. Here’s how you can generate a secret key using Speakeasy:
const secret = speakeasy.generateSecret({ length: 20 });
console.log(secret.base32); // This is the key you will store in your database
The generateSecret
method creates a secret key, and the base32
property provides a Base32-encoded string that you can store in your database. This string will be shared with the user to set up their authenticator app.
Step 3: Store the Secret Key
Once you have generated the secret key, you need to store it securely in your database. Here’s an example of how you might save it using Mongoose:
const User = require('./models/User'); // Assuming you have a User model
const userId = 'someUserId'; // Replace with the actual user ID
User.findByIdAndUpdate(userId, { twoFactorSecret: secret.base32 }, (err, user) => {
if (err) {
console.error('Error updating user with 2FA secret:', err);
} else {
console.log('2FA secret key stored successfully for user:', user.username);
}
});
Step 4: Share the Secret Key with the User
To complete the setup, you need to share the secret key with the user so they can configure their authenticator app. You can present this key as a QR code or a plain text string. Here’s an example of generating a QR code URL:
const qrcode = require('qrcode');
const otpauth_url = speakeasy.otpauthURL({
secret: secret.base32,
label: 'YourAppName',
issuer: 'YourCompanyName',
encoding: 'base32'
});
qrcode.toDataURL(otpauth_url, (err, data_url) => {
if (err) {
console.error('Error generating QR code:', err);
} else {
console.log('QR code URL:', data_url);
// You can now send this data_url to the user to scan with their authenticator app
}
});
By following these steps, you will have successfully generated and shared a secret key for 2FA with your users. The next phase involves verifying the OTPs generated by the user’s authenticator app during the login process.
Using the Speakeasy library
Storing Secrets Securely
Storing secrets securely is a crucial aspect of implementing Two-Factor Authentication (2FA). After generating the secret key for the user, it is essential to store this key in a secure manner to prevent unauthorized access. Here are some detailed steps and best practices for storing secrets securely:
Encryption: Always encrypt the secret key before storing it in your database. Use strong encryption algorithms such as AES-256 to ensure that even if the database is compromised, the secrets remain protected.
Environment Variables: Store encryption keys and other sensitive configuration details in environment variables rather than hardcoding them in your application. This practice helps in keeping sensitive information out of your source code.
Access Controls: Implement strict access controls to limit who and what can access the stored secrets. Use role-based access control (RBAC) to ensure that only authorized personnel and services can access the secret keys.
Audit Logs: Maintain detailed audit logs of all access and modifications to the stored secrets. This helps in tracking any unauthorized access attempts and understanding the history of changes made to the secrets.
Regular Rotation: Regularly rotate the secret keys and update the users' authenticator apps accordingly. This practice minimizes the risk of long-term exposure of a single secret key.
Secure Storage Solutions: Utilize secure storage solutions such as HashiCorp Vault, AWS Secrets Manager, or Azure Key Vault to manage and store secrets. These solutions provide additional layers of security and management features.
Here’s an example of securely storing a 2FA secret key in a database using encryption:
const crypto = require('crypto');
const algorithm = 'aes-256-cbc';
const key = process.env.ENCRYPTION_KEY; // Ensure this is 32 bytes
const iv = crypto.randomBytes(16);
function encrypt(text) {
let cipher = crypto.createCipheriv(algorithm, Buffer.from(key), iv);
let encrypted = cipher.update(text);
encrypted = Buffer.concat([encrypted, cipher.final()]);
return iv.toString('hex') + ':' + encrypted.toString('hex');
}
const secretKey = secret.base32;
const encryptedSecretKey = encrypt(secretKey);
User.update({ _id: userId }, { $set: { '2fa.secret': encryptedSecretKey } }, (err, user) => {
if (err) {
console.error('Error updating user with encrypted 2FA secret:', err);
} else {
console.log('Encrypted 2FA secret key stored successfully for user:', user.username);
}
});
By following these detailed steps and best practices, you can ensure that the secret keys used for 2FA are stored securely, thereby enhancing the overall security of your application.
Displaying the QR Code for User Setup
Once you have securely stored the 2FA secret key in your database, the next step is to display a QR code to the user. This QR code allows users to easily set up 2FA on their authentication app, such as Google Authenticator or Authy.
Here’s a detailed example of how you can generate and display a QR code for user setup:
Generate a 2FA Secret Key: First, generate a new 2FA secret key for the user. This key will be used to generate the QR code.
const speakeasy = require('speakeasy'); const secret = speakeasy.generateSecret({ length: 20 });
Store the Secret Key: Encrypt and store the secret key in your database as shown in the previous example.
Create a QR Code URL: Use the secret key to create a URL that can be used to generate a QR code. This URL follows the
otpauth://
protocol.const otpauthURL = speakeasy.otpauthURL({ secret: secret.ascii, label: encodeURIComponent(user.email), issuer: 'YourAppName', encoding: 'ascii' });
Generate the QR Code: Use a library like
qrcode
to generate the QR code image from the URL.const QRCode = require('qrcode'); QRCode.toDataURL(otpauthURL, (err, dataURL) => { if (err) { console.error('Error generating QR code:', err); return; } // Send the dataURL to the client to display the QR code res.send(`<img src="${dataURL}" alt="Scan this QR code with your 2FA app">`); });
Display the QR Code to the User: Finally, send the generated QR code to the client side and display it to the user. Users can then scan this QR code with their 2FA app to complete the setup.
By following these detailed steps, you ensure that users can easily and securely set up 2FA on their accounts. This process not only enhances the security of your application but also provides a seamless user experience.
Generating a QR code with Google Authenticator
Verifying 2FA Tokens
Setting up the verification route
Setting up the verification route is a crucial step in ensuring the security of your application. This route will handle the verification of the 2FA tokens submitted by the users. Here’s how you can set it up in detail:
First, you need to create a new route in your server that will handle the POST requests for token verification. This route will receive the token entered by the user and verify it against the secret key stored in your database.
const express = require('express');
const speakeasy = require('speakeasy');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/verify-2fa', (req, res) => {
const { token, userId } = req.body;
// Fetch the user's secret from the database
const user = getUserFromDatabase(userId); // Replace with your database call
const secret = user.twoFactorSecret;
// Verify the token
const verified = speakeasy.totp.verify({
secret: secret,
encoding: 'ascii',
token: token
});
if (verified) {
res.send('2FA token is valid!');
} else {
res.status(401).send('Invalid 2FA token.');
}
});
function getUserFromDatabase(userId) {
// This function should fetch the user from your database
// Here, we are just simulating a user object for demonstration purposes
return {
id: userId,
twoFactorSecret: 'your-secret-key-here'
};
}
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, the /verify-2fa
route accepts a POST request with the token
and userId
in the request body. It then fetches the user's secret key from the database and uses the speakeasy.totp.verify
method to check if the provided token is valid.
If the token is valid, the server responds with a success message. If the token is invalid, the server responds with a 401 status code and an error message.
By setting up this verification route, you ensure that only users with the correct 2FA token can access protected parts of your application. This adds an extra layer of security, making it much harder for unauthorized users to gain access.
Remember to replace the getUserFromDatabase
function with your actual database call to fetch the user's secret key. This example is simplified for demonstration purposes.
Integrating 2FA with the Login Process
Modifying the Authentication Flow to Include 2FA
To integrate Two-Factor Authentication (2FA) into your existing authentication flow, you need to make several modifications. First, after a user successfully logs in with their username and password, you should prompt them to enter a 2FA token. This token can be generated using an app like Google Authenticator or Authy, which the user has previously set up with your application.
Here's a step-by-step outline of the process:
User Login: The user enters their username and password.
Initial Verification: The server verifies the username and password.
2FA Prompt: If the credentials are correct, the server sends a prompt to the user to enter their 2FA token.
Token Verification: The user submits the 2FA token, which the server then verifies using the user's secret key stored in the database.
Access Granted: If the token is valid, the user is granted access to the application. If not, an error message is displayed, and the user is asked to try again.
Ensuring Seamless User Experience
To ensure a seamless user experience, it's crucial to make the 2FA process as smooth as possible. Here are some tips:
User-Friendly Prompts: Clearly instruct users on what they need to do when prompted for a 2FA token. Provide examples and links to help resources if necessary.
Remember Device Option: Offer an option to remember the user's device for a set period, so they don't have to enter a 2FA token every time they log in from the same device.
Timeouts and Retries: Implement reasonable timeouts and allow users to retry entering their 2FA token if they make a mistake.
Handling Backup Codes or Recovery Options
Backup codes and recovery options are essential for users who may lose access to their 2FA device. Here’s how you can handle these scenarios:
Backup Codes: Provide users with a set of backup codes when they first set up 2FA. These codes should be stored securely and used only if the user cannot access their 2FA device.
Recovery Options: Implement alternative recovery options, such as email or SMS-based recovery, to help users regain access to their accounts. Ensure these methods are secure and require additional verification steps to prevent unauthorized access.
Support: Offer robust customer support to assist users who encounter issues with 2FA. Provide clear instructions on how to contact support and what information they need to provide.
By carefully integrating 2FA into your authentication flow, ensuring a seamless user experience, and handling backup codes and recovery options effectively, you can significantly enhance the security of your application while maintaining user satisfaction.
Security Best Practices
Securing the 2FA data
Protecting sensitive routes
Regularly updating dependencies
Conclusion and Further Improvements
Summary of the implementation steps
This article covers the importance of Two-Factor Authentication (2FA) and how to implement it in a Node.js application. It provides an overview of various 2FA methods, such as SMS codes, authenticator apps, hardware tokens, and biometric verification. The guide walks through setting up a Node.js project, installing necessary packages like Speakeasy, generating and storing 2FA secrets securely, and displaying QR codes for user setup. It also details verifying 2FA tokens and integrating 2FA into the login process, while emphasizing security best practices. Potential improvements like biometric 2FA and third-party services are also discussed.
Subscribe to my newsletter
Read articles from Vishad Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
