Creating a Headless Web Scraper with Playwright and Node.js
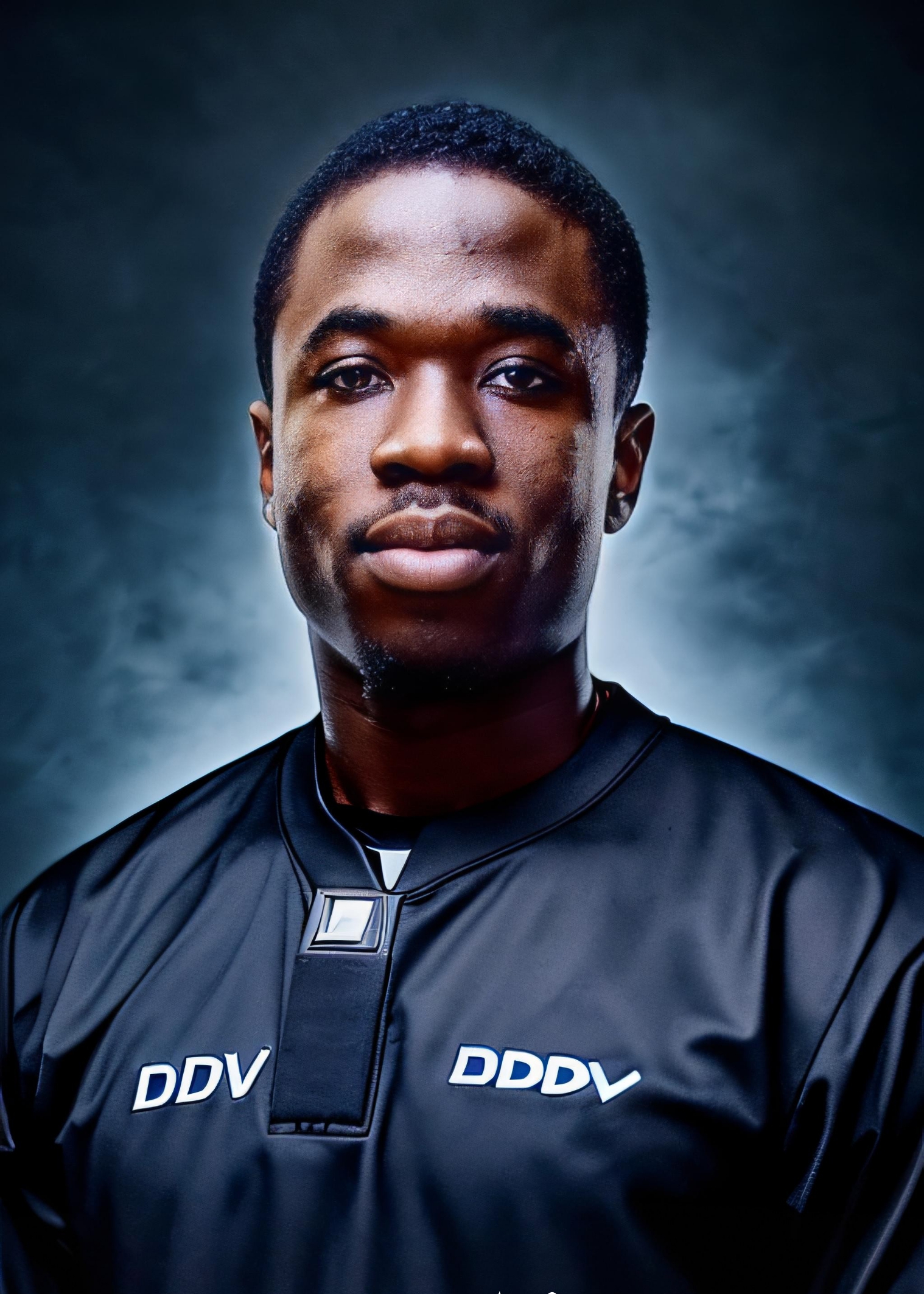
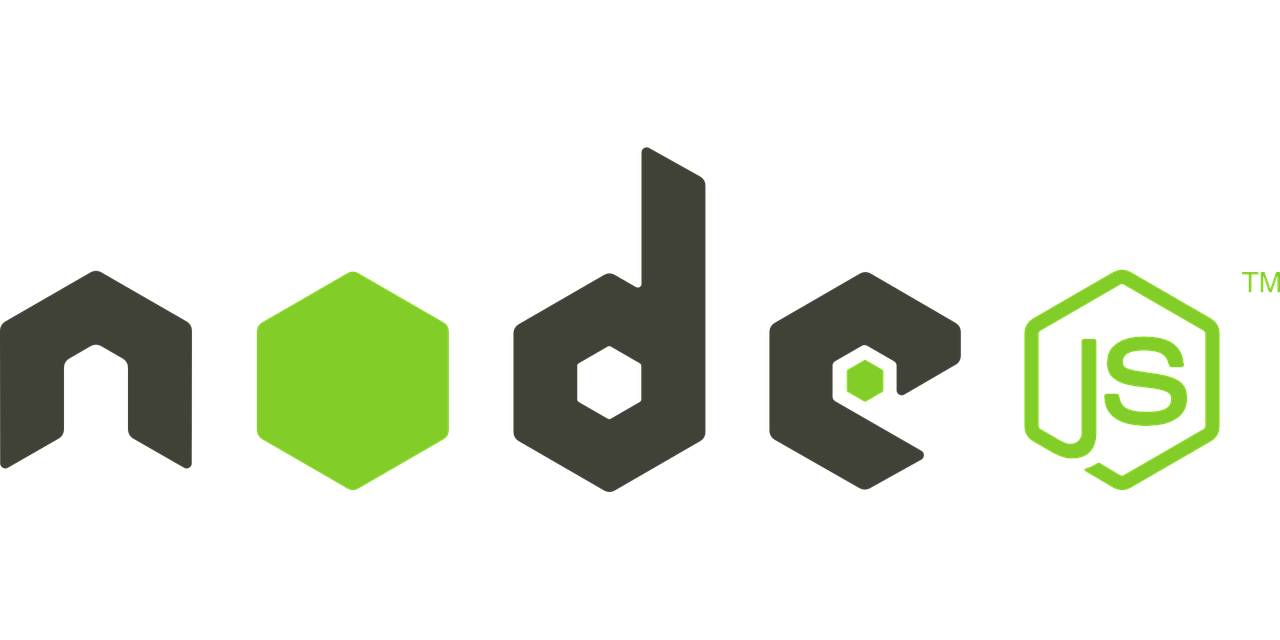
Introduction
Web scraping is an essential tool for gathering data from websites, automating processes, and interacting with dynamic content. By using web scraping, you can extract data such as prices, news, and social media trends, which can be analyzed or integrated into other applications.
A headless browser allows web scraping to run efficiently by executing tasks without rendering a graphical user interface, making it faster and more resource-efficient. In this article, we'll focus on using Playwright, a popular automation library, to build a headless web scraper with Node.js.
Playwright stands out for its ability to automate modern web applications that use advanced web technologies. Unlike other libraries like Puppeteer, Playwright can control multiple browser engines (Chromium, Firefox, and WebKit) and handle multi-page interactions seamlessly. This makes it ideal for complex scraping tasks where dynamic and JavaScript-driven content is involved.
By the end of this article, you’ll have a fully functional web scraper that can extract data from a target website using Playwright in Node.js.
Prerequisites
Before we dive into coding, let’s ensure you have the necessary prerequisites in place.
Basic Knowledge of JavaScript/Node.js: You should be comfortable with JavaScript syntax, promises, and asynchronous programming in Node.js.
Familiarity with Web Scraping Concepts: Understand the core ideas behind web scraping, such as HTML parsing and data extraction.
Node.js Installed: Ensure that Node.js is installed on your machine. You can download it from here.
Playwright Installed: We will install Playwright using npm (Node.js package manager).
Code Editor: A good code editor like Visual Studio Code (VSCode) will make the process easier.
Basic HTML and CSS Knowledge: You should know how to identify and select HTML elements, as this will be crucial when extracting content.
Setting Up the Environment
Let’s get started by setting up the Node.js project and installing Playwright.
Open your terminal and create a new directory for your project:
mkdir playwright-web-scraper cd playwright-web-scraper
Initialize a Node.js project:
npm init -y
Install Playwright:
npm install playwright
Now that you have Playwright installed, you're ready to start building the scraper.
Understanding Headless Browsers
A headless browser operates without a graphical user interface (GUI). It behaves just like a regular browser but doesn't display the content, making it faster for tasks like web scraping. This is ideal for automating data extraction from websites without unnecessary overhead from rendering visual elements.
You can still choose to run the browser in a non-headless mode during development to visualize and debug interactions, but for production scraping, headless mode is more efficient.
Creating Your First Web Scraper with Playwright
Let’s write a simple web scraper that extracts data from a sample website.
Create a JavaScript file: Create a new file called
scraper.js
in your project directory.Write the basic Playwright script:
const { chromium } = require('playwright'); (async () => { // Launch a headless browser const browser = await chromium.launch({ headless: true }); const page = await browser.newPage(); // Navigate to the target website await page.goto('https://example.com'); // Extract data const pageTitle = await page.title(); const pageText = await page.textContent('h1'); console.log('Page Title:', pageTitle); console.log('Heading Text:', pageText); // Close the browser await browser.close(); })();
Run the script: Execute the script using Node.js:
node scraper.js
You should see output that displays the page title and the text of the <h1>
element from https://example.com
.
Handling Dynamic Content
Many modern websites load content dynamically through JavaScript. Playwright excels at handling such dynamic content by waiting for elements to load before interacting with them.
To scrape a site that loads content dynamically, you can use Playwright’s waiting functions:
await page.waitForSelector('h1');
This ensures that Playwright waits for the h1
element to appear before extracting its text content. You can also use waitForResponse
to wait for network requests to complete.
Dealing with Pagination
Scraping paginated content is common when working with search engines, e-commerce sites, and other platforms displaying large datasets. Playwright allows you to easily automate interactions like clicking on a "Next" button to load new pages.
Example of handling pagination:
const nextButton = await page.$('text=Next'); // Find the 'Next' button by its text
if (nextButton) {
await nextButton.click();
await page.waitForTimeout(2000); // Wait for the next page to load
}
This approach can be placed in a loop to scrape data from multiple pages.
Saving Scraped Data
After scraping the desired data, you can save it to a file in formats like JSON or CSV.
Here’s how to save the data to a JSON file:
const fs = require('fs');
const scrapedData = {
title: pageTitle,
heading: pageText
};
// Write to a JSON file
fs.writeFileSync('data.json', JSON.stringify(scrapedData, null, 2));
This will create a data.json
file in your project directory with the scraped content.
Error Handling and Debugging
Web scraping can encounter various issues like network errors, timeouts, or unexpected content changes. To make your scraper robust, implement proper error handling and retries.
Example using try-catch for error handling:
try {
const pageText = await page.textContent('h1');
} catch (error) {
console.error('Error fetching text:', error);
}
For debugging, run the browser in non-headless mode to see exactly what’s happening:
const browser = await chromium.launch({ headless: false });
This will open the browser so you can watch it perform the scraping tasks in real time.
Respecting Website Policies
When scraping websites, it's important to respect their policies to avoid legal issues or getting banned. Check the site’s robots.txt
file to see if scraping is allowed, and be sure to limit the rate of requests to avoid overloading the server.
Example of setting a delay between requests:
await page.waitForTimeout(3000); // Wait for 3 seconds before making the next request
Additionally, avoid scraping user-protected data and take care when bypassing CAPTCHA systems, as doing so could lead to a violation of terms of service.
Extending the Scraper
To make your scraper more versatile, consider adding more features, such as:
Handling Forms: Use Playwright’s
fill
andclick
methods to fill out and submit forms.Authentication: Use Playwright’s ability to handle cookies and sessions for scraping behind login-protected areas.
Example of filling out a search form:
await page.fill('input[name="q"]', 'Playwright web scraping');
await page.click('button[type="submit"]');
Conclusion
In this article, we covered how to create a headless web scraper using Playwright and Node.js. You learned how to set up the environment, interact with dynamic content, handle pagination, and save the scraped data. By following these steps, you can now build robust scrapers that efficiently gather data from websites.
For more advanced use cases, explore Playwright’s features like multi-page scraping, session management, and parallel scraping to further optimize your web scraping projects.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
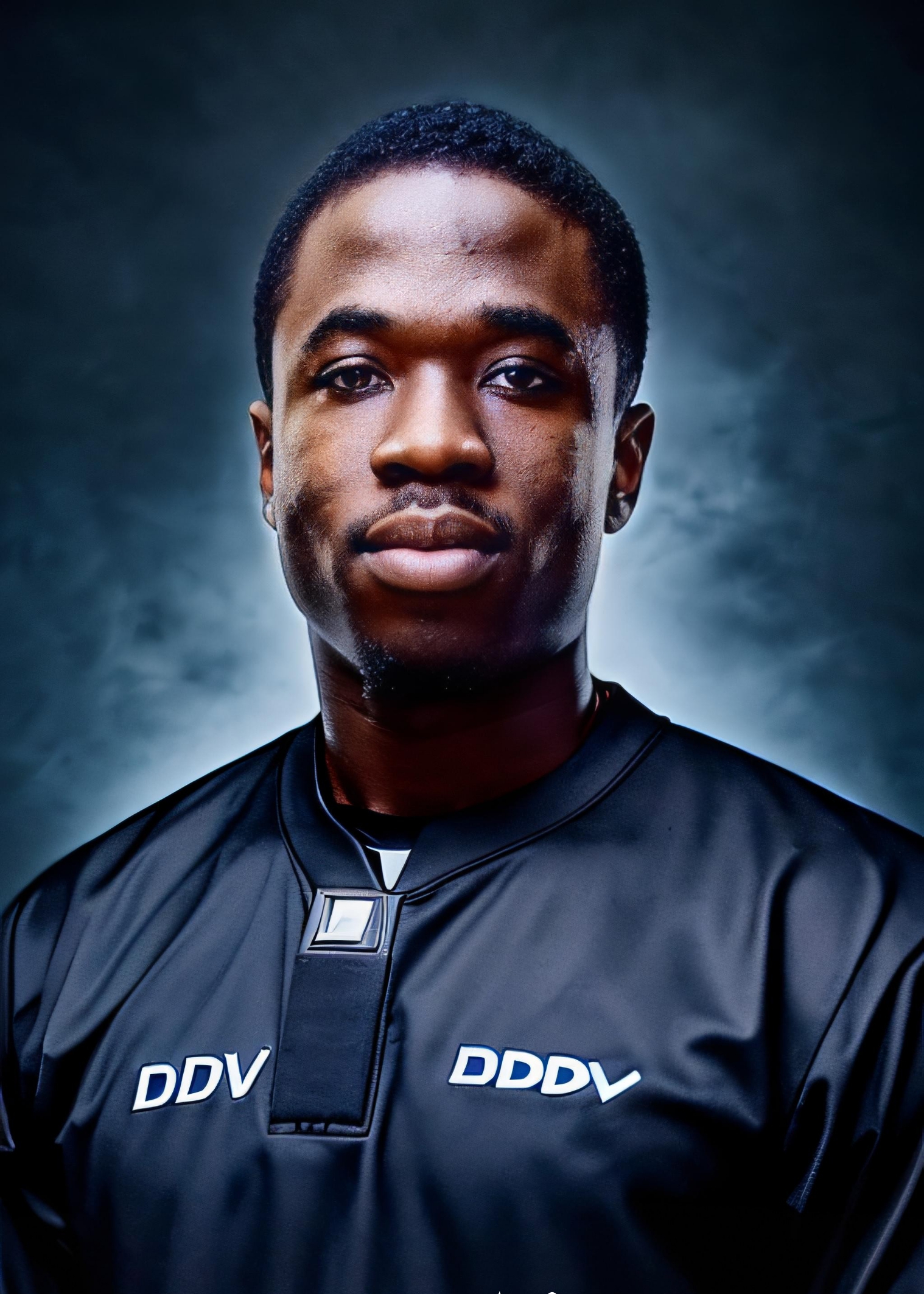
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.