Getting Started with GitHub Actions for Your Node.js App: A Step-by-Step Guide
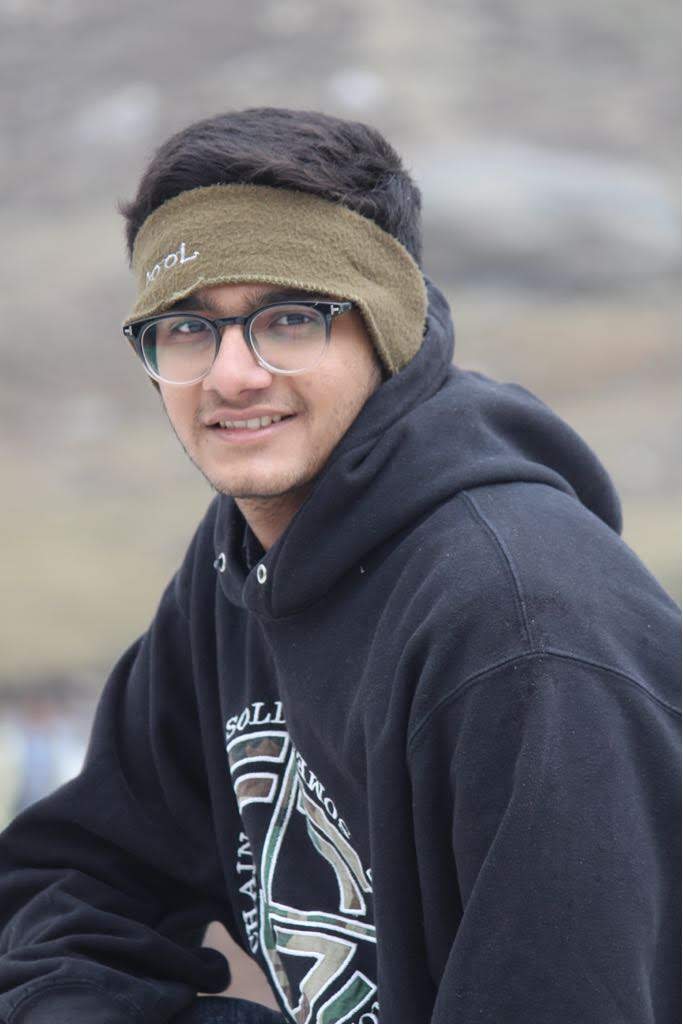
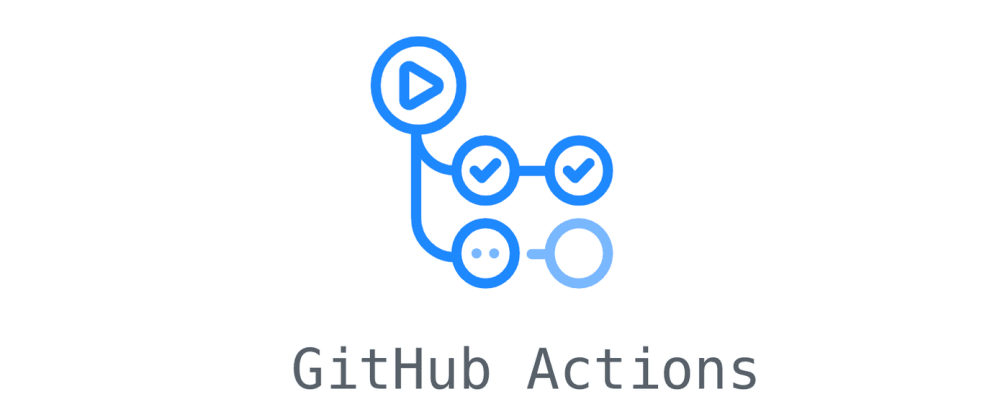
Hello fellow developers! 🎉 In this post, we’re diving into GitHub Actions—one of the best tools for automating workflows. Whether you’re new to CI/CD or looking to optimize your process, we’ll walk through setting up a simple GitHub Actions workflow for a Node.js app, complete with automated testing.
Let’s get rolling!
👓 What is GitHub Actions?
GitHub Actions enables us to automate, customize, and execute our software development workflows right from our GitHub repository. We can define actions that respond to events like push, pull request, and issue creation.
🌟 Why GitHub Actions?
Imagine this: every time we push code to GitHub, a magic script automatically runs tests, checks the code, and gives us the green light before deploying. That’s what GitHub Actions brings to the table!
By the end of this post, we’ll have:
A workflow that tests our Node.js app automatically.
Knowledge of how to extend the workflow for deployment and more.
Sound good? Let’s get started! 🚀
🚀 Step 1: Create Your Node.js App
If you already have a Node.js app, great! If not, let’s build a simple one real quick. We’ll use Express to create a basic server with a single endpoint.
Create the Server File
// server.js
const express = require('express');
const app = express();
app.get('/api/hello', (req, res) => {
res.json({ message: 'Hello, world!' });
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
module.exports = app;
This just returns a JSON message at /api/hello
. We can run this with node server.js
to check it out. Pretty simple, right? Let’s make sure it works by writing a test!
🧪 Step 2: Write a Unit Test
We’re going to use Jest and Supertest to verify that the /api/hello
endpoint works. Tests are our safety net, especially when deploying automatically.
Create the Test File for Node project
// server.test.js
const request = require('supertest');
const app = require('./server');
describe('GET /api/hello', () => {
it('should return Hello, world! message', async () => {
const res = await request(app).get('/api/hello');
expect(res.statusCode).toEqual(200);
expect(res.body.message).toBe('Hello, world!');
});
});
🛠️ Step 3: Setting Up GitHub Actions
Now for the exciting part—let’s automate! 💻
We’re going to create a GitHub Actions workflow that runs this test automatically every time we push to the main
branch or open a pull request.
Create the Workflow File
In the project root, create a
.github/workflows
directory (if it doesn’t exist).Inside that folder, create a file named
nodejs.yml
.
# .github/workflows/nodejs.yml
name: Node.js CI
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [16.x]
steps:
- name: Checkout repository
uses: actions/checkout@v3
- name: Setup Node.js
uses: actions/setup-node@v3
with:
node-version: ${{ matrix.node-version }}
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
🧐 Step 4: What’s Going On Here?
Let’s break it down together:
name: The name of the workflow—"Node.js CI" in this case.
on: We trigger this workflow whenever there’s a
push
orpull_request
to themain
branch.jobs: Here, we define the job that will run. It checks out the repository, sets up Node.js, installs dependencies, and runs the tests.
matrix: This allows us to run the job on different Node.js versions (like testing on
14.x
or16.x
).
📋 Quick check! Before moving forward, do a quick push and see if everything works. GitHub Actions will kick off automatically, running our workflow!
👀 Step 5: Watch Your Workflow in Action
Head over to the Actions tab in our GitHub repo. We’ll see the workflow running. If all goes well, it should pass! 🎉
If there’s an error, don’t worry—this is part of the learning process! Double-check the code and the workflow file to ensure everything matches up.
🛠️Step 6: Customize Your Workflow
Want to go a step further? Here are a few ideas:
Multiple Node.js versions: Add more versions to the
matrix
to test across14.x
and16.x
.Linting: Add a step for running ESLint to keep the code clean.
Deploying: Integrate the deployment process using GitHub Actions, so our app gets deployed once tests pass.
🌈 Wrapping Up
And there we have it, a simple but powerful GitHub Actions setup for our Node.js app! By automating testing, we ensure our code is always in good shape before merging or deploying. Feel free to extend this setup to cover more advanced workflows, like deployments or integrations with third-party services.
What else would we add to our CI/CD pipeline? Drop your thoughts in the comments below! 💬
That’s it for this guide! I hope you found it helpful. Don’t hesitate to reach out if you have any questions. Let us know how your GitHub Actions setup goes!
Happy coding! 👨💻👩💻
This post explores GitHub Actions, a powerful tool for automating development workflows directly from your GitHub repository. We'll guide you through setting up a simple CI/CD pipeline for a Node.js app, complete with automated testing using Jest and Supertest. By the end, you'll have a working workflow that runs tests on every push or pull request to the main branch, and ideas on how to extend it for deployment and other enhancements. Let's dive in! 🚀
Subscribe to my newsletter
Read articles from Dhruvang Dave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
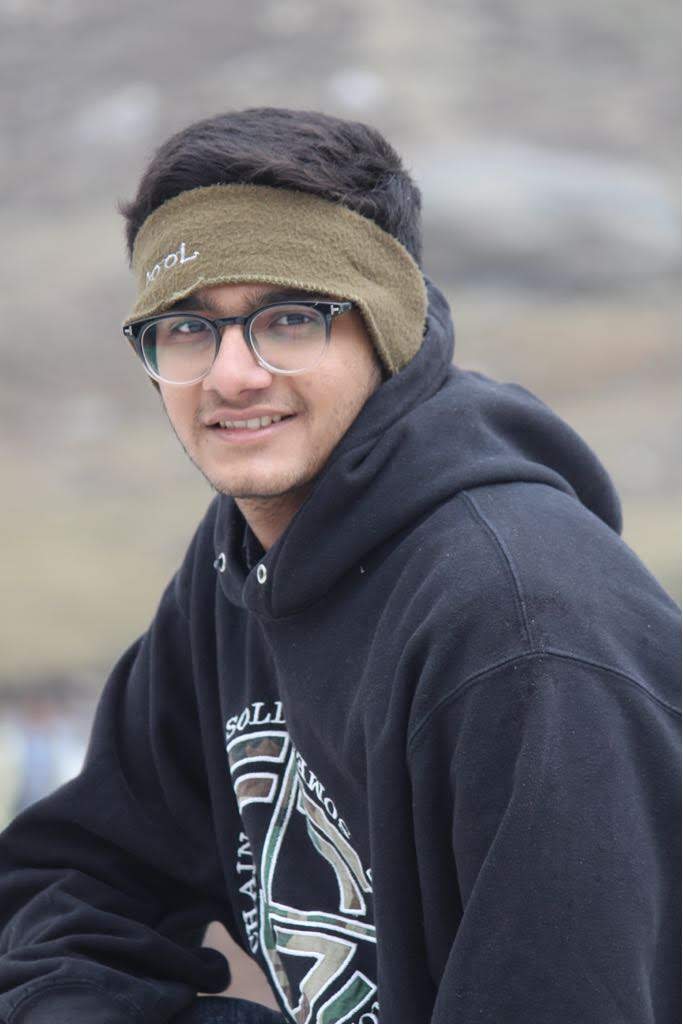