Code Smart, Not Fast: Avoiding Premature Optimization
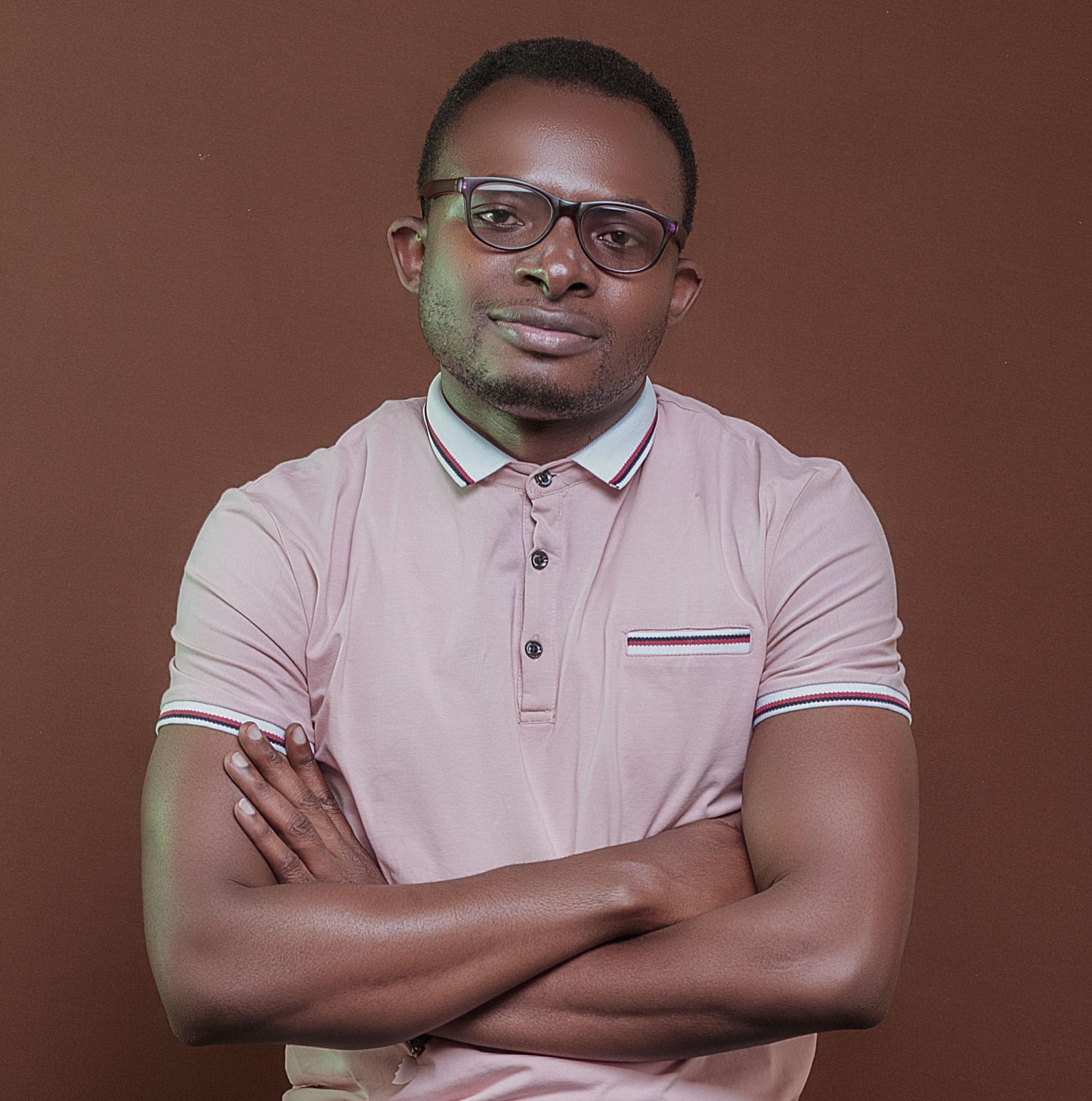
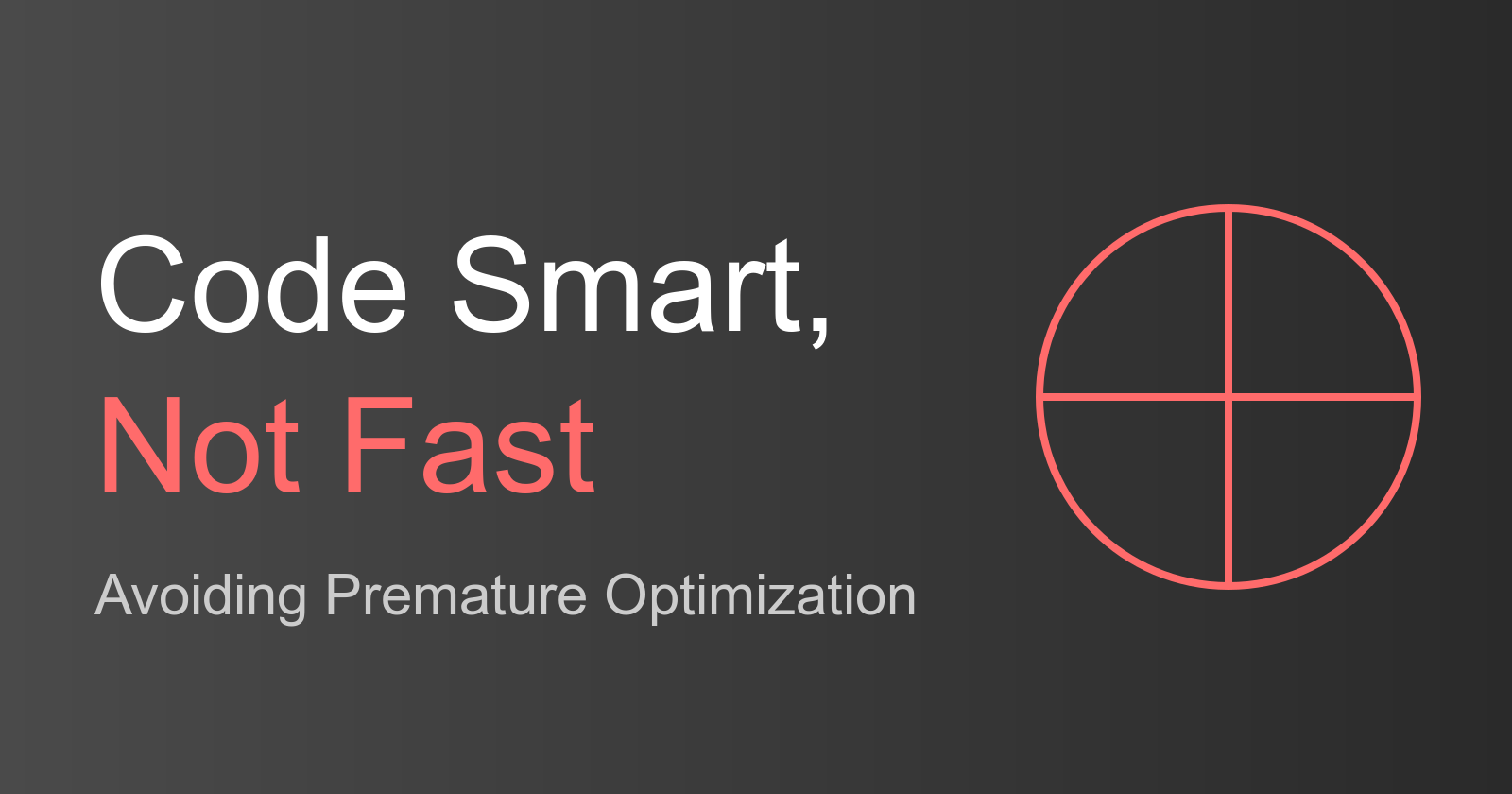
As a software engineer with several years of experience, I've seen my fair share of projects go off the rails. But there's one mistake that keeps popping up, no matter how many Medium articles warn against it: premature optimization. It's like that one friend who starts planning their retirement before they've even landed their first job. Sounds silly, right? Well, in the world of code, it's just as misguided. Let me break it down for you.
The Optimization Obsession: A Cautionary Tale
Picture this: It's 2 AM, I'm on my third cup of coffee, staring at a function that calculates user engagement metrics. "This loop could be 0.001% faster if I just..." I mutter to myself, eyes bloodshot. Fast forward two days, and I've turned a simple 10-line function into a Lovecraftian horror of bit-shifting and dark magic that nobody (including future me) can understand.
Sound familiar? Welcome to the world of premature optimization.
Why We Fall for It
The Performance Mirage: We convince ourselves that every microsecond counts. Spoiler alert: it usually doesn't.
Imposter Syndrome's Evil Twin: Sometimes, we optimize to prove we're "real" engineers. News flash: clear, working code is what real engineers write.
The Bikeshedding Effect: It's easier to obsess over minor performance tweaks than to tackle the big, scary architectural problems.
The Real-World Costs
Time Vampire: Remember that user authentication system you were supposed to build? Yeah, it's still not done because you spent three days optimizing a sorting algorithm that runs once a week.
Readability Nightmare:
// Before: Clear and simple foreach (var user in users) { SendEmail(user); } // After: "Optimized" and obscure ๐๐คฃ users?.Where(u => u != null).ToList().ForEach(u => SendEmail(u));
Congratulations, you saved 0.00001 seconds and confused every developer who'll ever read this code.
Flexibility? What Flexibility?: Nothing says "I don't trust future me" like hardcoding assumptions about data size and usage patterns.
Chasing Phantoms: You optimized the hell out of that database query, only to realize the real bottleneck was a badly configured Nginx server. Oops.
The Voice of Reason: Donald Knuth
As the sage Donald Knuth said, "Premature optimization is the root of all evil." And let's be real, if you're arguing with Donald Knuth about computer science, you've probably taken a wrong turn somewhere.
A Better Way: The Lazy Developer's Guide to Optimization
Make it Work: First, write code that correctly solves the problem. Revolutionary, I know.
Make it Right: Refactor for clarity and maintainability. Your future self will thank you.
Make it Fast (If Needed): Use profiling tools to identify actual bottlenecks. I'm a fan of dotTrace for .NET and Chrome DevTools for JavaScript.
Measure, Don't Guess: Always benchmark before and after optimization. You might be surprised (or disappointed) by the results.
Real-Life Example: The Great LINQ Query Massacre of 2023
Last year, I was working on a C# application that was processing customer orders. The system was slower than a snail racing through peanut butter. My first instinct? Optimize all the LINQ queries! Rewrite everything with loops! Pre-compute all the things!
Here's what the original code looked like:
public List<OrderSummary> GetOrderSummaries(int customerId)
{
return _context.Orders
.Where(o => o.CustomerId == customerId)
.Select(o => new OrderSummary
{
OrderId = o.Id,
TotalAmount = o.OrderItems.Sum(i => i.Price * i.Quantity),
ItemCount = o.OrderItems.Count,
OrderDate = o.OrderDate
})
.ToList();
}
In my optimization frenzy, I turned it into this monstrosity:
public List<OrderSummary> GetOrderSummaries(int customerId)
{
var orderIds = _context.Orders
.Where(o => o.CustomerId == customerId)
.Select(o => o.Id)
.ToList();
var orderItems = _context.OrderItems
.Where(i => orderIds.Contains(i.OrderId))
.ToList();
var groupedItems = orderItems
.GroupBy(i => i.OrderId)
.ToDictionary(g => g.Key, g => g.ToList());
return _context.Orders
.Where(o => orderIds.Contains(o.Id))
.AsEnumerable()
.Select(o => new OrderSummary
{
OrderId = o.Id,
TotalAmount = groupedItems[o.Id].Sum(i => i.Price * i.Quantity),
ItemCount = groupedItems[o.Id].Count,
OrderDate = o.OrderDate
})
.ToList();
}
I spent three days refactoring every query in the system like this, convinced I was going to make our app blazing fast. The result? The app was now 20% slower and the code was about as readable as ancient Sumerian.
After a week of premature optimization hell, I finally pulled out the profiler. Turns out, we had a simple N+1 query problem in our ORM configuration, and we were making hundreds of unnecessary database calls on every request.
The fix? Adding a simple Include statement:
public List<OrderSummary> GetOrderSummaries(int customerId)
{
return _context.Orders
.Include(o => o.OrderItems) // This was the real performance boost!
.Where(o => o.CustomerId == customerId)
.Select(o => new OrderSummary
{
OrderId = o.Id,
TotalAmount = o.OrderItems.Sum(i => i.Price * i.Quantity),
ItemCount = o.OrderItems.Count,
OrderDate = o.OrderDate
})
.ToList();
}
This single change made the app 10x faster, no fancy optimizations required. The database queries dropped from hundreds to just one, and the code remained clean and readable.
Lesson learned: Measure first, optimize later. And sometimes, the simplest solution is the best one.
Wrapping Up
Look, I get it. Optimization is sexy ๐. It makes us feel like coding wizards. But unless you're working at NASA or optimizing the next big cryptocurrency algorithm (please don't), chances are your time is better spent elsewhere.
So the next time you feel the urge to prematurely optimize, take a deep breath, step away from the keyboard, and ask yourself: "Is this really the best use of my time right now?" Your future self, your team, and your project deadlines will thank you.
Remember, in the wise words of Kent Beck: "Make it work, make it right, make it fast" โ and always in that order.
Now, if you'll excuse me, I have a perfectly functional, slightly inefficient algorithm to not optimize. ๐๐คฃ
Subscribe to my newsletter
Read articles from Sudi David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
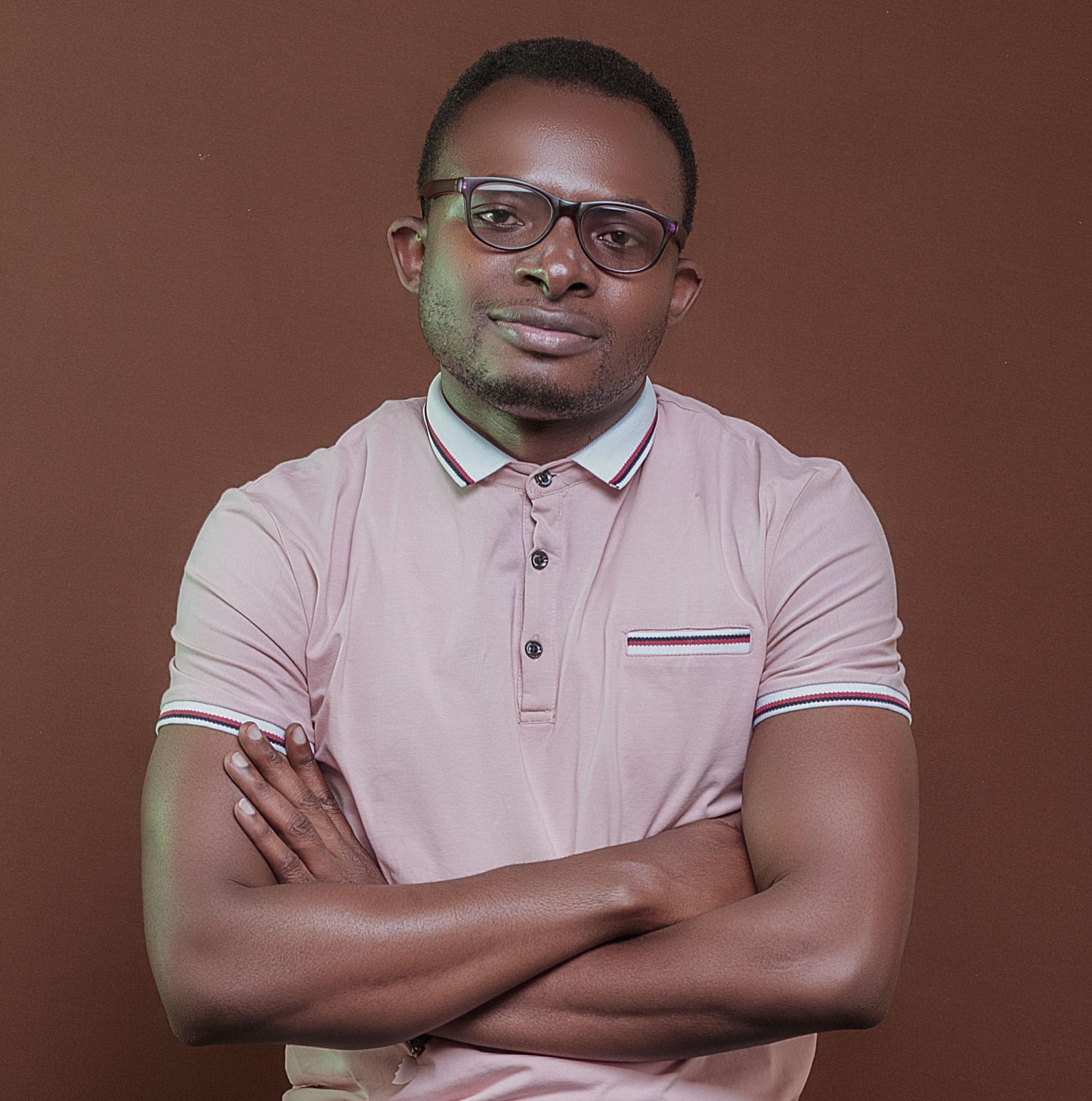
Sudi David
Sudi David
Hello There, I am Sudi David, a Software Developer who enjoys solving problems. I am on a quest to discover the world of frontend development specially with React.