Graph Theory Algorithms and Neural Networks: An In-Depth Exploration

Table of contents
- 1. Introduction to Graph Theory and Neural Networks
- 2. Graph Theory Algorithms: Overview
- 3. Neural Network Algorithms: Overview
- 4. The Relationship Between Graph Theory and Neural Networks
- 5. Real-World Examples of Graph Theory in Neural Networks
- 6. 6. Code Implementation: Graph Neural Networks (GNNs)
- 7. Recent Advancements in Graph Theory Impacting Deep Learning
- 8. Future Problems in Neural Networks That Graph Theory May Solve
- 9. Challenges and Limitations
- 10. Conclusion
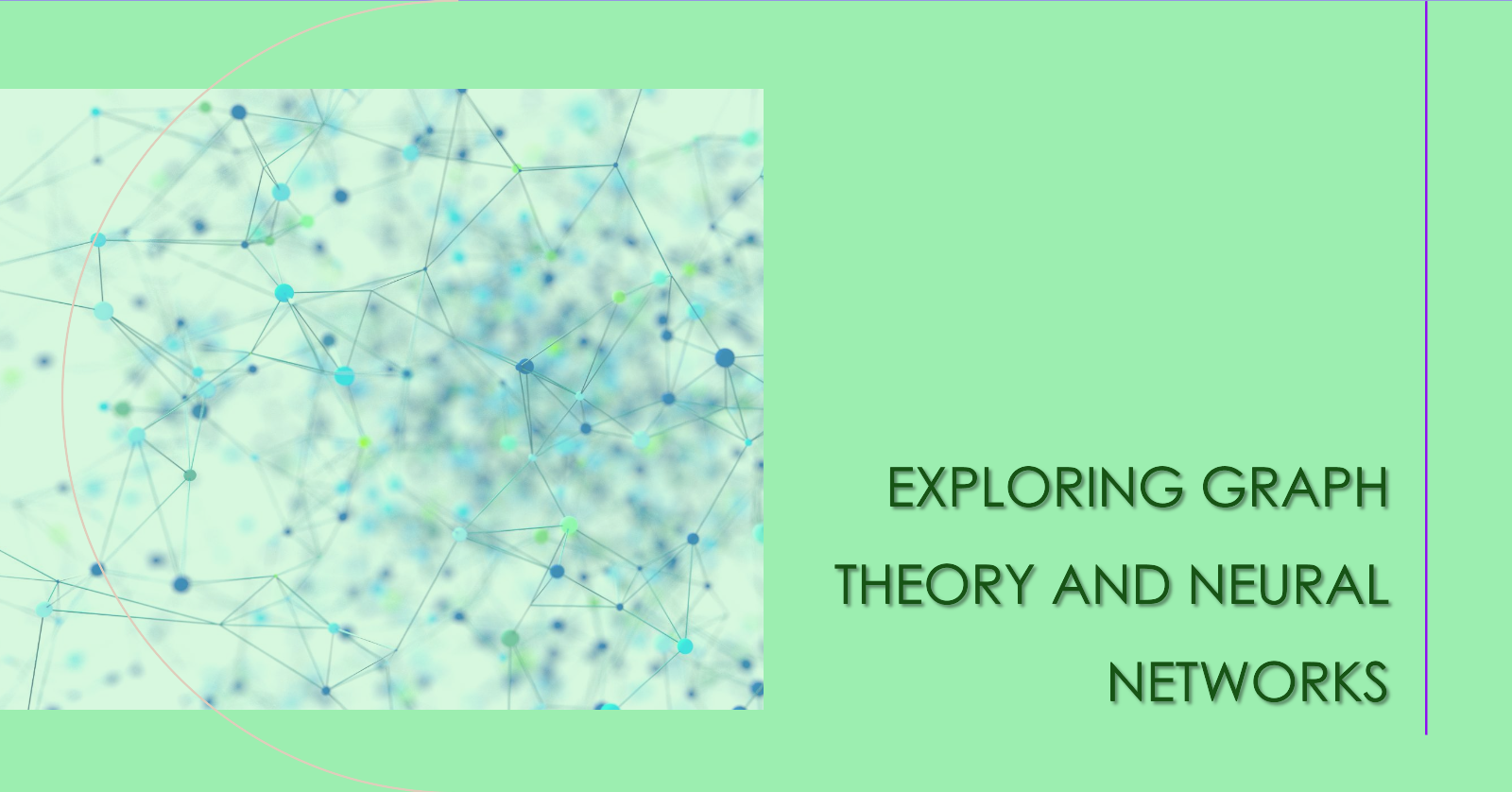
Graph theory and neural networks are two seemingly distinct fields in computer science and mathematics, but they have an intrinsic connection that has become increasingly significant as advancements in both areas have evolved. Graph theory, which deals with the study of graphs (a set of vertices connected by edges), has found numerous applications in machine learning, particularly in neural networks. From understanding complex relationships between data points to enhancing deep learning models, graph theory plays a crucial role in improving the efficiency and accuracy of neural networks.
In this blog post, we will explore the relationship between graph theory algorithms and neural network algorithms, delve into recent advancements in graph theory that are impacting deep learning algorithms, and discuss the future problems that graph theory may solve. We will also use real-world examples, code snippets, and graphical representations to illustrate the concepts.
1. Introduction to Graph Theory and Neural Networks
Graph theory is a branch of discrete mathematics that focuses on the study of graphs—mathematical structures used to model pairwise relations between objects. A graph consists of vertices (also called nodes) and edges (which are lines connecting pairs of vertices). Some common real-world examples of graphs include social networks, transportation systems, biological networks, and communication systems.
Neural networks are a subset of machine learning, inspired by the structure and functioning of the human brain. They consist of layers of artificial neurons (nodes) interconnected through weighted edges. These networks are used to model complex functions and are the foundation of deep learning models, which excel in tasks such as image classification, natural language processing, and reinforcement learning.
At first glance, these two fields might seem unrelated, but recent advances in artificial intelligence (AI) and machine learning have increasingly blurred the lines between them. By combining the mathematical principles of graph theory with the flexibility and learning capabilities of neural networks, researchers have created more powerful and adaptable models.
2. Graph Theory Algorithms: Overview
Common Graph Theory Algorithms
Some of the most well-known algorithms in graph theory include:
Depth-First Search (DFS): DFS is an algorithm for traversing or searching tree or graph structures. It starts at the root node (or an arbitrary node in the graph) and explores as far as possible along each branch before backtracking.
Breadth-First Search (BFS): BFS is another traversal algorithm that explores all the neighbor nodes at the present depth prior to moving on to nodes at the next depth level.
Dijkstra’s Algorithm: Dijkstra's algorithm finds the shortest path between nodes in a graph, which may represent, for example, road networks.
Prim’s and Kruskal’s Algorithm: These algorithms find the minimum spanning tree (MST) in a weighted graph, where a minimum spanning tree connects all vertices with the least total edge weight.
PageRank Algorithm: Developed by Google, PageRank is used to rank websites in search engine results by representing the web as a directed graph, where websites are nodes and hyperlinks are edges.
Types of Graphs
There are several types of graphs in graph theory:
Un-directed Graphs: Edges have no direction, meaning the connection between two nodes goes both ways.
Directed Graphs (Digraphs): Edges have a specific direction.
Weighted Graphs: Edges have weights or costs associated with them.
Unweighted Graphs: All edges are treated equally, without any weights.
Graph theory algorithms operate on these graphs and are used for analyzing the structure, traversal, and optimization of various real-world networks.
3. Neural Network Algorithms: Overview
Types of Neural Networks
Feedforward Neural Networks (FNNs): These are the simplest type of neural networks where information moves in only one direction—from input to output. There are no cycles or loops in the network.
Convolutional Neural Networks (CNNs): Designed primarily for image data, CNNs utilize convolutional layers to capture spatial hierarchies in data, making them highly effective for computer vision tasks.
Recurrent Neural Networks (RNNs): RNNs are designed for sequential data, such as time series or natural language. They maintain a memory of previous inputs to influence current outputs.
Graph Neural Networks (GNNs): GNNs are a recent innovation that utilizes the power of graph theory to operate on data represented as graphs. They are highly effective for problems where relationships between data points are more important than the data points themselves (e.g., social networks, molecular structures).
Neural Network Algorithms
Neural network algorithms work by optimizing a loss function (a measure of how far off the model is from the actual values) using techniques like backpropagation and gradient descent. Popular optimization algorithms include:
Stochastic Gradient Descent (SGD)
Adam Optimizer
RMSprop
Neural networks excel at finding patterns in large amounts of data but struggle when the data has complex relationships that cannot be easily captured by simple layers of neurons. This is where graph theory steps in.
4. The Relationship Between Graph Theory and Neural Networks
Graph theory and neural networks are connected through the representation of relationships. In many real-world applications, data is not independent and identically distributed (i.i.d.), but instead involves complex interactions between entities. Graph theory provides a way to model these interactions, and neural networks provide a way to learn from them.
Some key areas where graph theory is used in neural networks include:
Graph Representation of Data
Many types of data, such as social networks, transportation systems, or biological interactions, are naturally represented as graphs. Neural networks, which traditionally operate on vector data, can be enhanced with graph theory to better model these structures. For example, in a social network, the relationships between users can be modeled as edges in a graph, and user attributes (age, gender, interests) can be represented as node features.
Graph Neural Networks (GNNs)
Graph Neural Networks (GNNs) are a powerful way to combine graph theory and neural networks. GNNs operate directly on the graph structure and can capture complex relationships in the data. In GNNs, the nodes represent entities, and the edges represent relationships between these entities. The GNN learns to aggregate information from neighboring nodes, allowing it to make predictions based on both the node features and the structure of the graph.
A popular application of GNNs is in recommendation systems, where users are represented as nodes and their interactions with items (movies, products) are represented as edges. The GNN can learn to predict what items a user might like based on their interactions with other items and the interactions of similar users.
5. Real-World Examples of Graph Theory in Neural Networks
Example 1: Social Network Analysis
In social networks, users are represented as nodes, and connections between them (friendships, follows) are edges. Traditional neural networks may struggle to make sense of the relationships between users, but GNNs can take advantage of the graph structure. A GNN could be used to predict user behavior, recommend friends, or detect communities within the network.
Example 2: Drug Discovery
In drug discovery, molecules are often represented as graphs, where atoms are nodes, and bonds are edges. GNNs can be used to predict the properties of new molecules by learning from the graph structure of known molecules. This has the potential to revolutionize drug discovery by reducing the need for expensive and time-consuming lab experiments.
Example 3: Transportation Networks
Transportation networks, such as airline routes or road systems, are naturally represented as graphs. Nodes represent airports or intersections, and edges represent routes or roads. GNNs can be used to predict traffic patterns, optimize routes, or even design new transportation systems.
6. 6. Code Implementation: Graph Neural Networks (GNNs)
Let's dive into a code example to understand how GNNs work in practice. We'll use the popular Python library PyTorch
along with the PyTorch Geometric
library, which is designed for working with graph-structured data.This code implements a Graph Convolutional Network (GCN) for node classification on the CORA citation network.
Building a Simple GNN using CORA Dataset
import torch
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
from torch_geometric.datasets import Planetoid
from torch_geometric.data import DataLoader
# Load the Cora dataset (a citation network)
dataset = Planetoid(root='/tmp/Cora', name='Cora')
class GCN(torch.nn.Module):
def __init__(self):
super(GCN, self).__init__()
self.conv1 = GCNConv(dataset.num_features, 16)
self.conv2 = GCNConv(16, dataset.num_classes)
def forward(self, data):
x, edge_index = data.x, data.edge_index
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, training=self.training)
x = self.conv2(x, edge_index)
return F.log_softmax(x, dim=1)
# Create the model, define the optimizer and loss function
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = GCN().to(device)
data = dataset[0].to(device)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01, weight_decay=5e-4)
# Training the GNN
model.train()
for epoch in range(200):
optimizer.zero_grad()
out = model(data)
loss = F.nll_loss(out[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
# Testing the GNN
model.eval()
_, pred = model(data).max(dim=1)
correct = int(pred[data.test_mask].eq(data.y[data.test_mask]).sum().item())
accuracy = correct / int(data.test_mask.sum())
print(f'Accuracy: {accuracy:.4f}')
After running the code the Accuracy came out to be 0.8110.
Google Colab Link - Simple_GNN_using_CORA_Dataset.ipynb
7. Recent Advancements in Graph Theory Impacting Deep Learning
In recent years, there have been several notable advancements in graph theory that have significantly impacted deep learning:
7.1 Graph Neural Networks (GNNs)
The most significant advancement is the development of Graph Neural Networks (GNNs). GNNs extend traditional neural networks to graph-structured data, allowing deep learning models to work with complex relationships between data points. GNNs have shown great success in areas such as social network analysis, recommendation systems, and molecular biology.
7.2 Graph Attention Networks (GATs)
Graph Attention Networks (GATs) are a type of GNN that use attention mechanisms to weigh the importance of different neighbors when aggregating information from the graph. This allows the model to focus on the most relevant neighbors, improving performance on tasks where some relationships are more important than others.
7.3 Dynamic Graph Neural Networks
Traditional GNNs operate on static graphs, but many real-world graphs are dynamic, meaning they change over time. Dynamic Graph Neural Networks (DGNNs) can handle this by updating the graph structure and node features as the graph evolves.
7.4 Higher-Order Graph Convolutions
In traditional GCNs, each layer aggregates information from neighboring nodes, but higher-order graph convolutions allow the model to capture more complex patterns by aggregating information from nodes that are further away in the graph. This improves the model’s ability to capture long-range dependencies.
8. Future Problems in Neural Networks That Graph Theory May Solve
8.1 Handling Non-Euclidean Data
Traditional neural networks are designed for Euclidean data (e.g., images or time series), but much of the data in the real world is non-Euclidean, such as social networks or biological interactions. Graph theory provides a framework for representing and processing non-Euclidean data, and future advances in graph theory could lead to even more powerful neural network models capable of handling this data.
8.2 Improving Interpretability
One of the biggest challenges in neural networks is their lack of interpretability. Graph theory could help by providing more interpretable models that can explicitly represent the relationships between different entities in the data.
8.3 Scalability
As graphs grow larger, processing them efficiently becomes increasingly challenging. Advances in graph theory, such as scalable algorithms for large graphs, could help make GNNs and other graph-based models more efficient and scalable.
8.4 Improving Robustness
Neural networks are often vulnerable to adversarial attacks, where small perturbations in the input data can cause the model to make incorrect predictions. Graph theory could help improve the robustness of neural networks by providing models that are more resistant to these types of attacks.
9. Challenges and Limitations
9.1 Computational Complexity
Graph algorithms can be computationally expensive, especially for large graphs. This makes it challenging to scale GNNs to very large datasets, such as social networks with millions of users.
9.2 Graph Representation
One of the challenges in applying graph theory to neural networks is finding an appropriate representation of the graph. Real-world data often comes in different forms, and converting it into a graph can be non-trivial.
9.3 Overfitting
GNNs are prone to overfitting, especially when the graph structure is highly complex or when there is limited training data. Regularization techniques and careful tuning of the model are necessary to avoid overfitting.
10. Conclusion
Graph theory and neural networks are two fields that are increasingly converging, with graph theory providing a powerful framework for modeling complex relationships in data, and neural networks offering the flexibility and learning capabilities to make sense of these relationships. Recent advancements in graph theory, such as GNNs, GATs, and dynamic graphs, have opened up new possibilities for deep learning, allowing it to handle more complex data and tasks.
Looking ahead, we can expect even more exciting developments at the intersection of graph theory and deep learning. Future research may focus on improving the scalability and interpretability of GNNs, developing new graph-based models for non-Euclidean data, and using graph theory to solve some of the biggest challenges in neural networks, such as robustness and overfitting.
With the potential to revolutionize fields ranging from drug discovery to transportation systems, the combination of graph theory and neural networks is set to have a lasting impact on both artificial intelligence and real-world applications.
References:
Kipf, T. N., & Welling, M. (2017). Semi-Supervised Classification with Graph Convolutional Networks.
Veličković, P., et al. (2018). Graph Attention Networks.
Subscribe to my newsletter
Read articles from Pronod Bharatiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pronod Bharatiya
Pronod Bharatiya
As a passionate Machine Learning and Deep Learning enthusiast, I document my learning journey on Hashnode. My experience encompasses various projects, from exploring foundational algorithms to implementing advanced neural networks. I enjoy breaking down complex concepts into digestible insights, making them accessible for all. Join me as I share my thoughts, tutorials, and tips to navigate the exciting world of ML and DL. Connect with me on LinkedIn to explore collaboration opportunities!