Mastering Authentication in Next.js with Auth.js
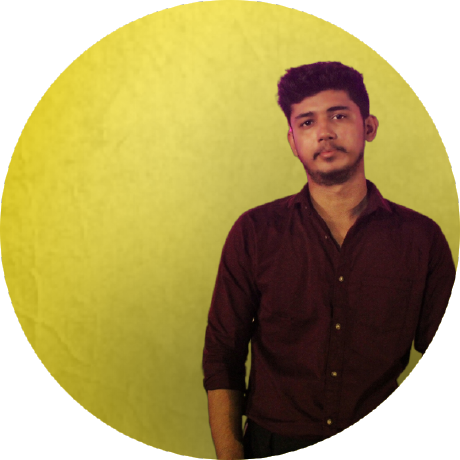
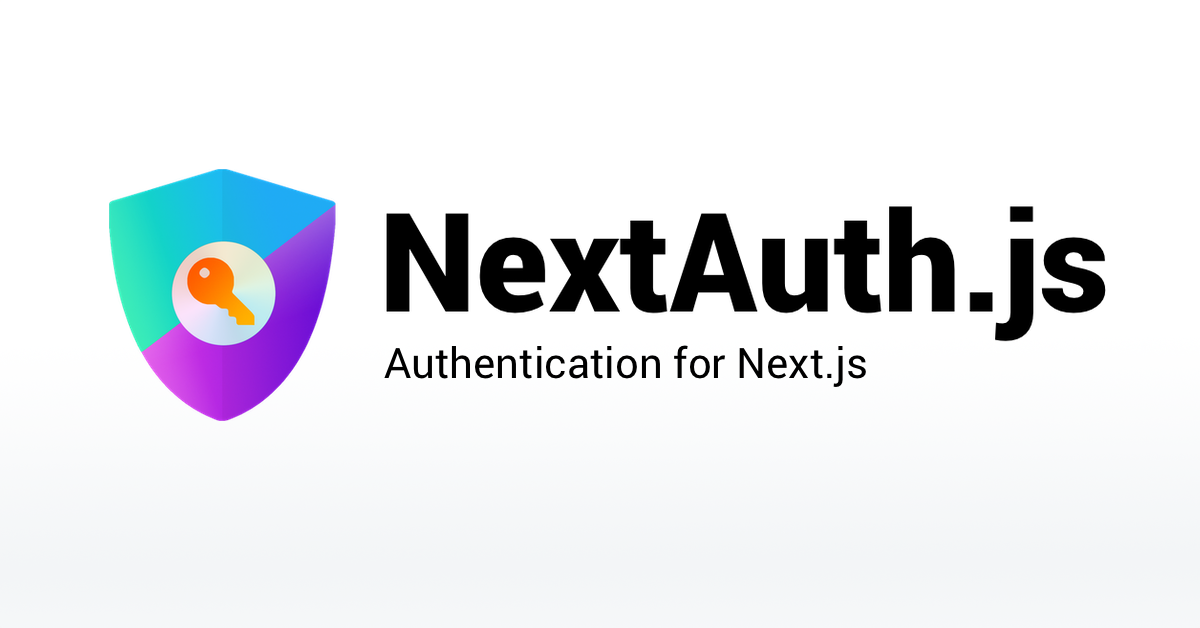
Authentication is a fundamental aspect of modern web applications, ensuring users can securely access their accounts and sensitive data. Integrating authentication in Next.js can be straightforward with the help of Auth.js (formerly known as NextAuth.js). This powerful library simplifies adding various authentication methods to your Next.js application, making it easier to manage user sessions and secure your app.
In this guide, we'll walk through the steps of integrating Auth.js into a Next.js application, covering everything from setup to customization.
Why Use Auth.js with Next.js?
Auth.js provides a comprehensive solution for handling authentication in Next.js. Here’s why it’s a great choice:
Multiple Providers: Support for OAuth providers like Google, GitHub, and Facebook, as well as custom credential-based authentication.
Session Management: Built-in session handling with secure cookies.
Flexibility: Customizable and extensible to fit various authentication needs.
Ease of Use: Simplifies the implementation of authentication flows with minimal configuration.
Setting Up Your Next.js Application
Let's start by creating a new Next.js project and integrating Auth.js.
Step 1: Create a New Next.js Project
First, create a new Next.js application if you don’t have one already:
npx create-next-app
cd my-auth-app
Step 2: Install Auth.js and Dependencies
npm install next-auth
Step 3: Configure Auth.js
Create a configuration file for Auth.js to set up authentication providers and session management. Create a file named
[...nextauth].js
inside thepages/api/auth/
directory:// pages/api/auth/[...nextauth].js import NextAuth from "next-auth"; import GoogleProvider from "next-auth/providers/google"; export default NextAuth({ providers: [ GoogleProvider({ clientId: process.env.GOOGLE_CLIENT_ID, clientSecret: process.env.GOOGLE_CLIENT_SECRET, }), ], secret: process.env.NEXTAUTH_SECRET, });
In this configuration:
We use GoogleProvider for authentication with Google. Replace
GOOGLE_CLIENT_ID
andGOOGLE_CLIENT_SECRET
with your credentials obtained from the Google Developer Console.The
NEXTAUTH_SECRET
is used to sign and encrypt session cookies. Set this in your.env.local
file.Step 4: Set Up Environment Variables
Create a .env.local
file in the root directory of your project and add the following environment variables:
GOOGLE_CLIENT_ID=your-google-client-id
GOOGLE_CLIENT_SECRET=your-google-client-secret
NEXTAUTH_SECRET=your-next-auth-secret
Step 5: Create Authentication UI
Now, let’s create a basic authentication UI to handle sign-in and sign-out. Open pages/index.js
and add the following code:
Step 6: Protecting Routes
To protect specific routes from unauthorized access, use middleware. Create a file named middleware.js
in the root directory:
import { withAuth } from "next-auth/middleware";
export default withAuth({
pages: {
signIn: "/auth/signin",
},
});
This middleware ensures that users are redirected to the sign-in page if they are not authenticated.
Step 7: Customizing the Sign-In Page
You can create a custom sign-in page to match your application's design. Create pages/auth/signin.js
with the following content:
Conclusion
Integrating Auth.js into your Next.js application allows you to handle authentication with ease. You can quickly set up various authentication providers, manage user sessions, and protect routes using the configuration provided by Auth.js. With a few steps, you’ve transformed your Next.js app into a secure and user-friendly environment.
Feel free to customize the authentication flow and UI according to your needs. With Auth.js, building robust authentication systems has never been easier!
Resources
Next.js Documentation
Feel free to reach out with any questions or feedback. Happy coding!
Subscribe to my newsletter
Read articles from Shivam barthwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
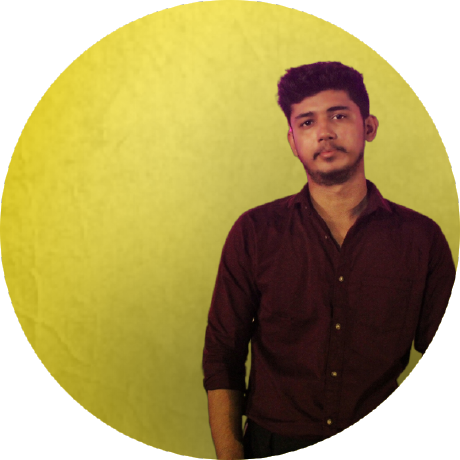