A Beginner's Guide to Using FlatList in React Native
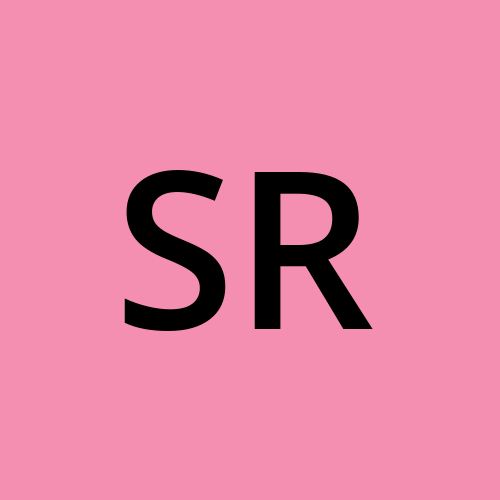
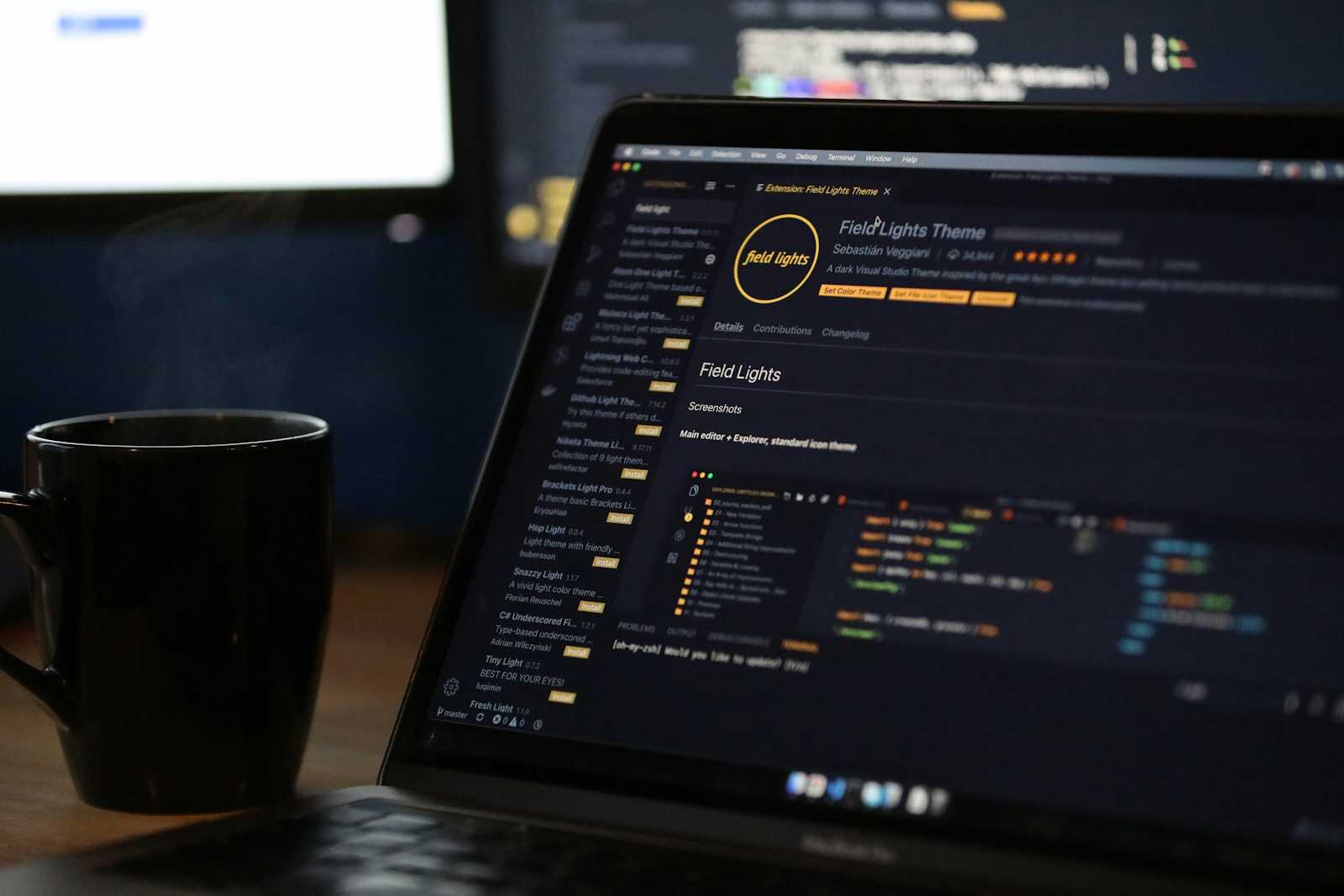
If you're building a mobile app using React Native and need to render a list of items efficiently, the FlatList
component is your go-to solution. It’s optimized for handling large datasets and supports a range of features like infinite scrolling, item separators, headers, and more.
In this guide, I’ll walk you through everything you need to know to use FlatList
like a pro, even if you’re just getting started with React Native.
Table of Contents:
What is
FlatList
?Why Use
FlatList
?Setting Up Your React Native Project
Basic Usage of
FlatList
Rendering Custom Items
KeyExtractor: Why It's Important
Handling Larger Lists
Advanced Features of
FlatList
Horizontal Scroll
Adding Headers and Footers
Handling Infinite Scrolling
Conclusion
1. What is FlatList
?
FlatList
is a core component of React Native that provides an efficient way to display lists of data. It automatically renders only the visible items on the screen, which improves performance, especially when dealing with large lists.
The key features of FlatList
include:
Lazy loading of items (it only renders what's visible).
Built-in support for scrollable lists.
Support for rendering custom components.
Easy to integrate with infinite scrolling.
2. Why Use FlatList
?
React Native has multiple options for rendering lists, but FlatList
is highly recommended when you’re working with larger datasets because:
Performance: It’s optimized for performance by rendering only the visible items.
Customization: You can easily customize how items are displayed by passing a custom component for rendering each item.
Features: It supports key features like scroll loading, pull-to-refresh, and item separators.
3. Setting Up Your React Native Project
Before we dive into using FlatList
, make sure you have your React Native environment set up. If not, follow the official React Native setup guide to get started.
Once your environment is ready, you can create a new project:
bashCopy codenpx react-native init FlatListExample
After the project is created, navigate into your project folder:
bashCopy codecd FlatListExample
4. Basic Usage of FlatList
The simplest way to use FlatList
is by passing an array of data and a function to render each item.
Basic Example
Here’s a simple example of how to use FlatList
to display a list of items:
jsxCopy codeimport React from 'react';
import { FlatList, Text, View, StyleSheet } from 'react-native';
const App = () => {
const data = [
{ id: '1', name: 'Item 1' },
{ id: '2', name: 'Item 2' },
{ id: '3', name: 'Item 3' },
];
return (
<FlatList
data={data}
renderItem={({ item }) => <Text>{item.name}</Text>}
keyExtractor={item => item.id}
/>
);
};
export default App;
Breakdown:
data
: This is an array of objects, each representing an item in the list.renderItem
: A function that takes anitem
as input and returns a component to display it.keyExtractor
: A function that returns a unique key for each item in the list, which is crucial for React’s reconciliation process.
5. Rendering Custom Items
In most cases, you won’t want to render just plain text. You’ll probably want to create a more complex layout for each list item.
Here’s an example of rendering a custom item layout using a View
and Text
elements:
jsxCopy codeconst App = () => {
const data = [
{ id: '1', name: 'Apple', price: '$1.00' },
{ id: '2', name: 'Banana', price: '$0.50' },
{ id: '3', name: 'Orange', price: '$0.80' },
];
return (
<FlatList
data={data}
renderItem={({ item }) => (
<View style={styles.itemContainer}>
<Text style={styles.itemName}>{item.name}</Text>
<Text style={styles.itemPrice}>{item.price}</Text>
</View>
)}
keyExtractor={item => item.id}
/>
);
};
const styles = StyleSheet.create({
itemContainer: {
padding: 10,
borderBottomWidth: 1,
borderBottomColor: '#ccc',
},
itemName: {
fontSize: 18,
},
itemPrice: {
fontSize: 14,
color: 'gray',
},
});
Here, each item is displayed with both a name and a price in a styled layout.
6. KeyExtractor: Why It's Important
keyExtractor
is a critical part of using FlatList
. It ensures that each item in the list has a unique key, which is important for React’s performance. Without unique keys, React will have trouble efficiently updating the list when changes occur.
In most cases, you can use the id
from your data as the key:
javascriptCopy codekeyExtractor={item => item.id}
If your data doesn’t have an id
, you can generate one using the item’s index:
javascriptCopy codekeyExtractor={(item, index) => index.toString()}
7. Handling Larger Lists
If your dataset is large, FlatList
shines by only rendering items that are visible on the screen. It does this by keeping a limited number of items in memory. This is especially useful when you’re working with hundreds or thousands of items.
8. Advanced Features of FlatList
Horizontal Scroll
By default, FlatList
scrolls vertically. However, you can make it scroll horizontally by setting the horizontal
prop to true
:
jsxCopy code<FlatList
data={data}
renderItem={({ item }) => <Text>{item.name}</Text>}
horizontal
keyExtractor={item => item.id}
/>
Adding Headers and Footers
You can add a header or footer to your list by using the ListHeaderComponent
and ListFooterComponent
props:
jsxCopy code<FlatList
data={data}
renderItem={({ item }) => <Text>{item.name}</Text>}
ListHeaderComponent={() => <Text style={styles.header}>My List Header</Text>}
ListFooterComponent={() => <Text style={styles.footer}>End of List</Text>}
keyExtractor={item => item.id}
/>
Handling Infinite Scrolling
Infinite scrolling is essential when you have a large dataset and want to load more items as the user scrolls.
Use the onEndReached
prop to detect when the user reaches the end of the list:
jsxCopy code<FlatList
data={data}
renderItem={({ item }) => <Text>{item.name}</Text>}
onEndReached={() => {
// Fetch more data here
}}
onEndReachedThreshold={0.5} // Trigger when 50% of the list is reached
keyExtractor={item => item.id}
/>
9. Conclusion
The FlatList
component is powerful yet simple to use, making it an essential tool for any React Native developer. Whether you're building a small app with just a few items or a large app with infinite scrolling, FlatList
will help you render your lists efficiently and with flexibility.
By mastering FlatList
, you'll be able to display data in a way that feels smooth and responsive to users, even when working with large datasets.
Feel free to experiment with the features discussed here and customize them according to your app’s needs. Happy coding! 🚀
Subscribe to my newsletter
Read articles from Subham Rawat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
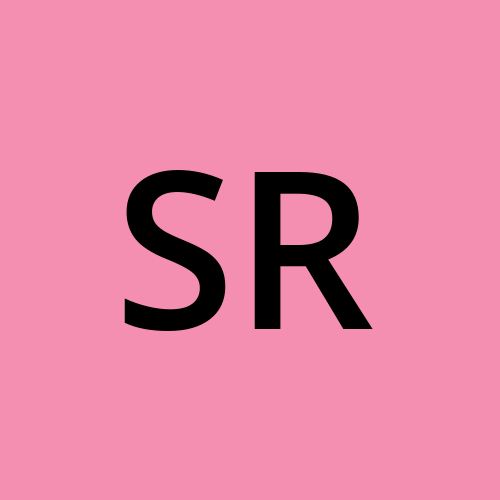