Mastering Order Processing Systems: A Comprehensive Guide to Building Efficient Business Solutions with Python
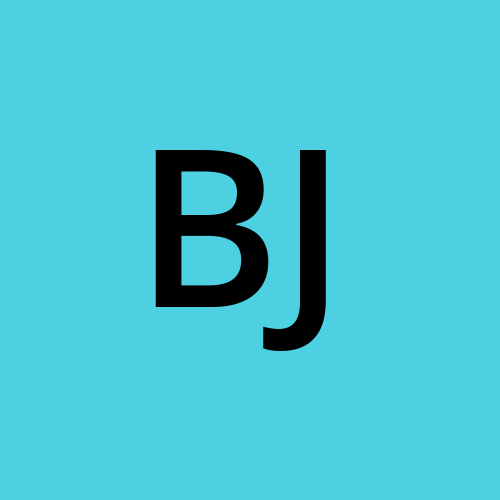
Part 2: Setting Up the Development Environment
Introduction
Before diving into building the order processing system, it’s essential to ensure that your development environment is set up correctly. This part of the blog will guide you through the installation of the necessary tools and libraries, the creation of a virtual environment, and the setup of your project structure. By the end of this post, you will have everything ready to start coding and building your system.
Step 1: Installing Python and Required Libraries
The foundation of this project is Python, a powerful yet easy-to-learn programming language. If you don't have Python installed on your machine, follow these steps:
Download Python:
Visit the official Python website and download the latest stable version (preferably Python 3.x).
Make sure to select the option to add Python to your system's PATH during installation.
Verify Python Installation:
Open your command prompt (Windows) or terminal (Mac/Linux).
Run the following command to check if Python is installed:
python --version
You should see something like:
Python 3.x.x
Install Required Libraries:
- For this project, you'll need several libraries such as
sqlite3
,tkinter
,matplotlib
,pandas
,seaborn
, and more. You can install these using Python’s package managerpip
.
- For this project, you'll need several libraries such as
Run the following command to install the necessary libraries:
pip install matplotlib pandas seaborn werkzeug
These libraries are essential for creating the GUI, managing data, and generating reports in your order processing system.
Verify Library Installation:
- After installing the libraries, you can verify the installation by importing the libraries in Python. Open your Python interpreter and run:
import matplotlib
import pandas
import seaborn
import sqlite3
import tkinter
If no errors occur, your environment is set up correctly.
Step 2: Setting Up a Virtual Environment
Using a virtual environment is a good practice for any Python project. It allows you to create an isolated environment for your project with its own set of dependencies, avoiding conflicts with other projects on your machine.
Create a Virtual Environment:
First, navigate to the folder where you want to create your project.
Run the following command to create a virtual environment named
venv
:
python -m venv venv
Activate the Virtual Environment:
- On Windows, use:
venv\Scripts\activate
- On Mac/Linux, use:
source venv/bin/activate
Install the Libraries in the Virtual Environment:
- With the virtual environment activated, install the necessary libraries again:
pip install matplotlib pandas seaborn werkzeug
Deactivating the Virtual Environment:
- Once you’re done working in the virtual environment, you can deactivate it by running:
deactivate
Step 3: Installing SQLite
SQLite is a lightweight, serverless database engine that we will use to manage the data for the order processing system. Python comes with built-in support for SQLite through the sqlite3
library, so you don’t need to install anything extra.
Verify SQLite Installation:
- You can check if SQLite is installed by running the following command in your terminal:
sqlite3 --version
This should return the installed version of SQLite. If SQLite is not installed, you can download it from here based on your operating system.
Step 4: Creating the Project Structure
Now that we have all the tools and libraries installed, it’s time to set up the project structure. This structure will help you organize your code effectively and ensure a smooth development process.
Create the Project Folder:
- In your desired location, create a new folder for the project. You can name it something like
order_processing_system
.
- In your desired location, create a new folder for the project. You can name it something like
Organize the Files: Inside the project folder, organize the following structure:
order_processing_system/ ├── venv/ # Virtual environment ├── database/ # Database files will be stored here │ └── order_system.db # SQLite database file ├── logs/ # Log files to record system events │ └── order_system.log # Log file for system operations ├── src/ # All Python code will be inside this folder │ ├── __init__.py # Initializes the Python package │ ├── main.py # Main file to run the application │ ├── db_setup.py # Database setup script │ └── order_processor.py # Order processing logic ├── README.md # Project documentation └── requirements.txt # List of all required libraries
Creating Initial Files:
db_
setup.py
: This script will contain the logic for initializing the database. As you saw in Part 1, the code for initializing the database could look like this:
def init_db():
conn = sqlite3.connect('database/order_system.db')
c = conn.cursor()
c.execute('''
CREATE TABLE IF NOT EXISTS users (
user_id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE,
password TEXT,
role TEXT
)
''')
# (Other table creation queries here...)
conn.commit()
conn.close()
main.py
: This will be the entry point of your application. Initially, it will import the required modules and call the necessary functions:
from db_setup import init_db
if __name__ == "__main__":
init_db()
print("Database initialized, ready to start the order processing system!")
requirements.txt
: This file will list all the libraries you need for this project. After installing the libraries usingpip
, you can generate this file by running:
pip freeze > requirements.txt
Your requirements.txt
will look something like this:
matplotlib==3.4.3
pandas==1.3.3
seaborn==0.11.2
werkzeug==2.0.1
Next Steps
With your development environment set up, you're now ready to start coding! In the next part of this series, we’ll dive into building the user interface using Tkinter, where users will be able to log in and begin interacting with the system.
By following the steps outlined above, you now have a solid foundation for your project. The virtual environment ensures that your project dependencies are isolated, and the organized project structure will make it easier to maintain and scale as we progress.
In the upcoming post, we’ll walk through nderstanding the Basics of Order Processing Systems. Stay tuned!
Link to My Source code : https://github.com/BryanSJamesDev/-Order-Processing-System-OPS-/tree/main
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
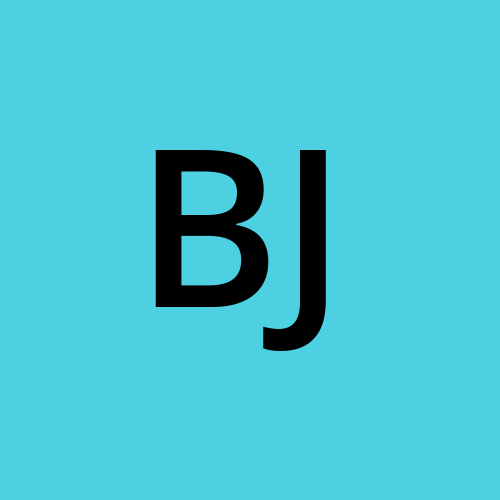