Setting Up a Modern JavaScript Development Environment: Tools and Best Practices
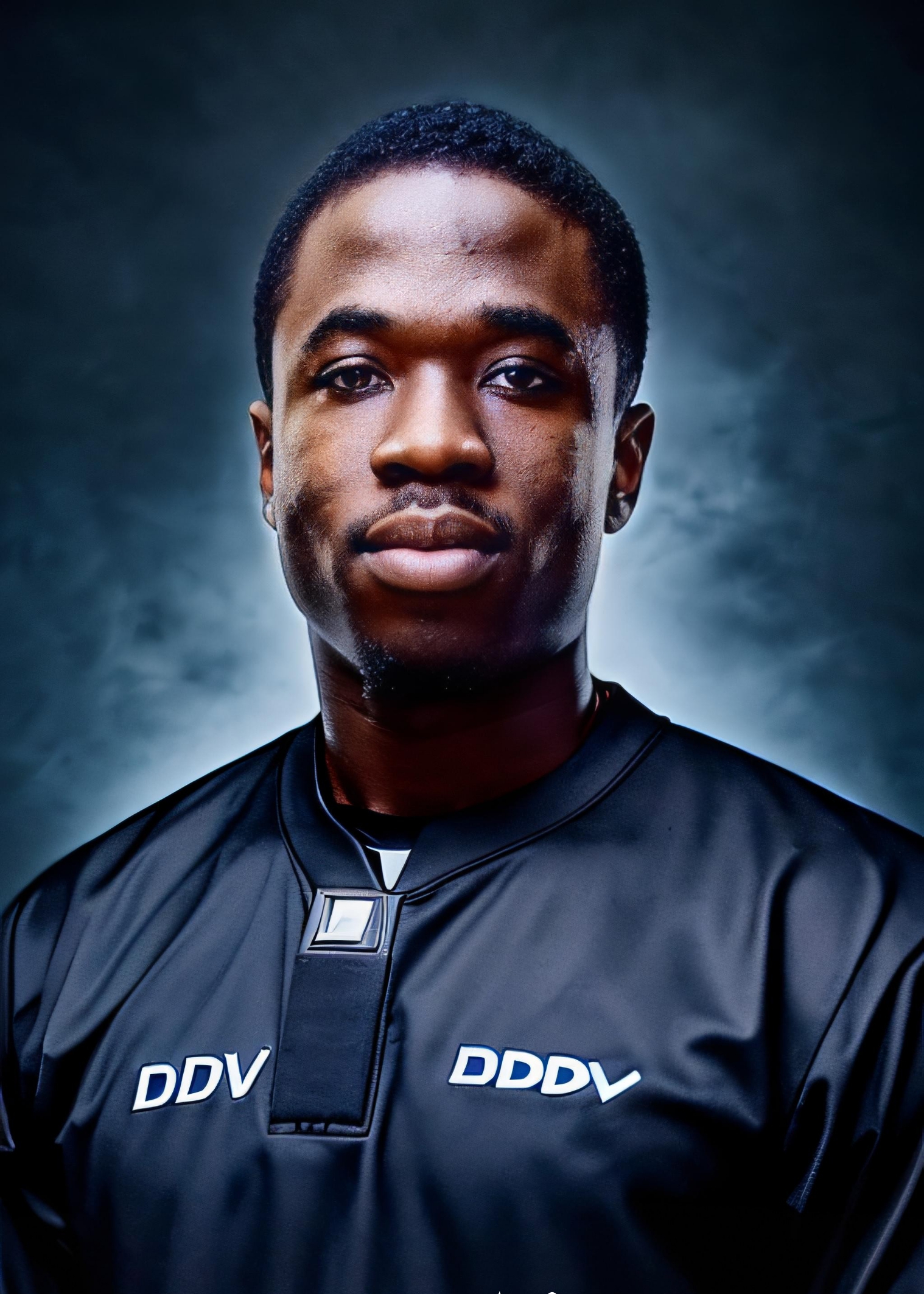
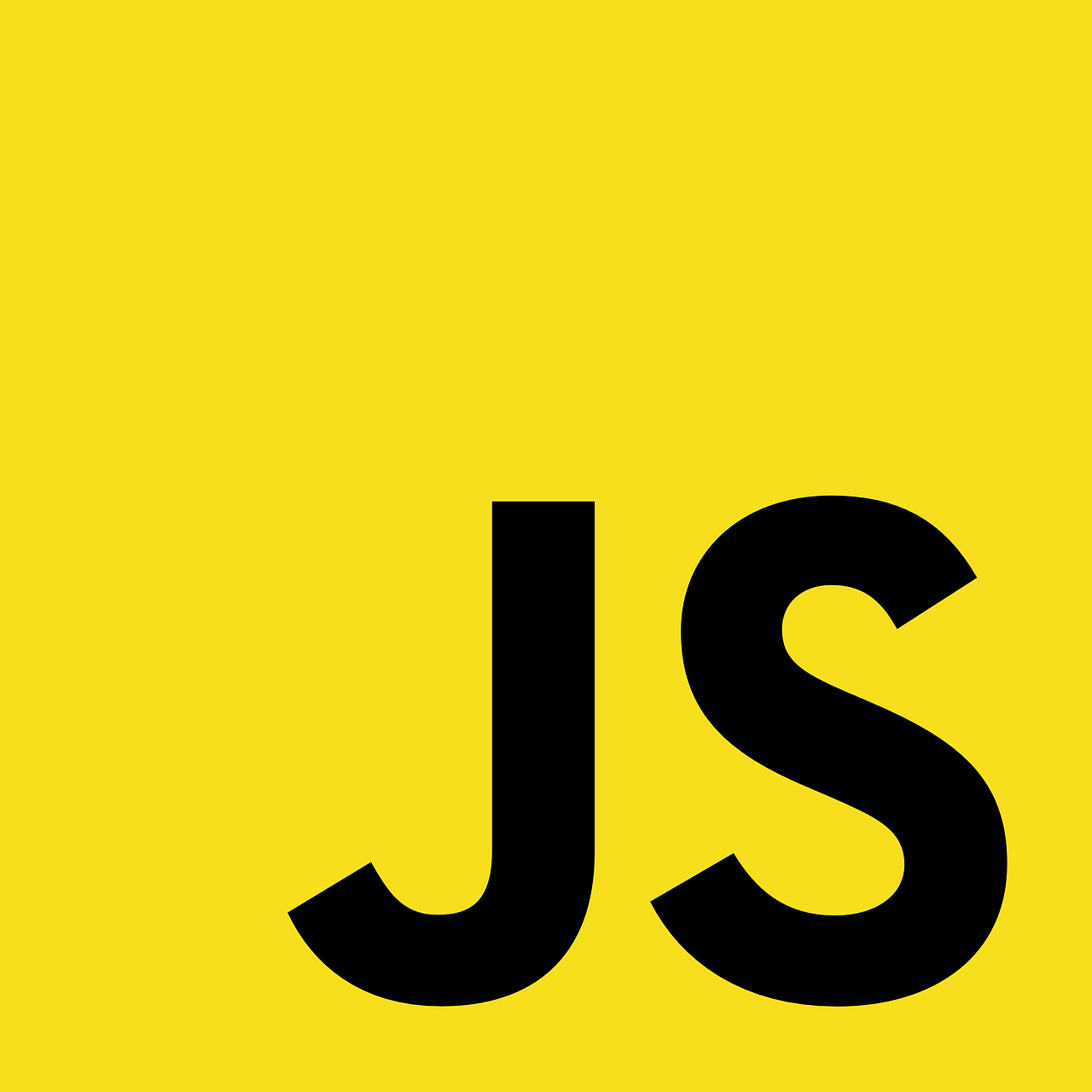
In today’s fast-paced web development world, having a well-structured and efficient JavaScript development environment is essential for productivity. Whether you’re working on a small project or a complex enterprise application, using the right tools can make a world of difference. In this guide, I’ll walk you through the essential steps to setting up a modern JavaScript development environment and share best practices to streamline your workflow.
Prerequisites
Before diving into setting up your environment, you’ll need a few prerequisites in place:
Basic Understanding of JavaScript: You should already be familiar with core JavaScript concepts like variables, functions, and control flow.
Command Line Basics: Basic knowledge of how to navigate the terminal or command prompt is necessary for running scripts and managing dependencies.
Node.js and npm: Ensure you have Node.js and npm (Node Package Manager) installed. Node.js allows you to run JavaScript on your machine, while npm is crucial for managing project dependencies.
- To install Node.js, download the appropriate installer for your operating system from the official website.
Text Editor or IDE: Familiarize yourself with modern text editors like Visual Studio Code, Sublime Text, or an IDE such as WebStorm.
Core Tools for a Modern JavaScript Development Environment
1. Version Control with Git
Version control is critical in modern software development. Git allows developers to track changes in their code, collaborate with others, and revert to previous versions if needed.
Installation: Download and install Git from the official website.
Setting up a Repository: Once Git is installed, initialize a repository in your project folder using:
git init
Creating a Remote Repository: For collaboration, you can push your code to platforms like GitHub or GitLab. This ensures that your code is safely stored and accessible for other team members.
2. Node.js and npm/yarn
Node.js is a runtime that allows you to run JavaScript on the server or your local machine, while npm or yarn handles package management.
npm: Installed automatically with Node.js, npm allows you to manage external libraries or tools.
yarn: An alternative to npm, yarn offers some advantages in speed and consistency. You can install yarn globally via npm:
npm install --global yarn
3. Modern Text Editors or IDEs
Choosing the right text editor can greatly enhance your development experience.
Visual Studio Code: Widely used due to its flexibility and rich extension ecosystem. Extensions like ESLint, Prettier, and Debugger for Chrome make VS Code an excellent choice for JavaScript developers.
WebStorm: This IDE offers advanced features tailored to JavaScript development, such as integrated debugging, code analysis, and version control support.
Development Tools and Best Practices
1. Package Management
Managing third-party libraries is a fundamental part of modern JavaScript development.
npm and yarn are used to manage dependencies. Use a
package.json
file to track these dependencies, specify version ranges, and set up scripts to automate tasks:npm init -y # Creates a default package.json
To install a package:
npm install <package-name>
2. Module Bundlers
Bundlers help you compile your JavaScript code and its dependencies into a single file or multiple optimized files.
Webpack: A highly customizable bundler that can manage your project’s assets such as JavaScript, CSS, and images.
Parcel: A simpler bundler with zero-config setup.
Rollup: Focused on ES modules and is commonly used for library bundling.
For example, setting up Webpack in your project involves:
npm install webpack webpack-cli --save-dev
Create a webpack.config.js
file for your build settings:
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: __dirname + '/dist'
},
mode: 'development'
};
3. Transpilers
Modern JavaScript features (ES6+) often need to be transpiled into backward-compatible versions for older browsers. Babel is the go-to tool for this.
Install Babel and configure it with Webpack:
npm install --save-dev @babel/core @babel/preset-env babel-loader
Add Babel to your Webpack configuration:
module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'] } } } ] }
4. Linters and Formatters
Maintaining code quality and consistency is essential.
ESLint: A popular linter that enforces coding standards. Install it globally or locally in your project:
npm install eslint --save-dev npx eslint --init # Initialize ESLint configuration
Prettier: A code formatter that ensures consistent style:
npm install prettier --save-dev
Setting Up a Local Development Server
Running a local server during development helps with hot-reloading and testing your application in a live environment.
Live Server Extensions: In VS Code, you can install the "Live Server" extension, which provides an easy way to view your code in the browser with automatic reloading.
Node.js and Express: For more control, you can set up a local server using Express.
npm install express --save
Basic Express setup:
const express = require('express'); const app = express(); app.use(express.static('public')); app.listen(3000, () => console.log('Server running on port 3000'));
Version Control Best Practices
Setting up
.gitignore
: Avoid committing unnecessary files (e.g.,node_modules
) by creating a.gitignore
file:node_modules/ dist/ .env
Commit Guidelines: Follow conventional commit messages, like:
git commit -m "feat: add new login feature"
Branching Strategies: Use feature branches (
feature/my-new-feature
) to keep the main branch clean.
Testing Tools
Testing ensures the reliability of your code.
Jest: A popular testing framework for JavaScript, especially suited for React projects.
npm install jest --save-dev
Create a test file and run:
npx jest
Continuous Integration (CI) Setup
Automating your testing and deployment process ensures that your code is always production-ready.
GitHub Actions: You can automate tasks like running tests or deploying your project:
name: Node.js CI on: [push] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Setup Node.js uses: actions/setup-node@v1 - run: npm install - run: npm test
Best Practices for a Modern Development Workflow
Automated Code Formatting and Linting: Use tools like Husky to automate linting and formatting before committing code.
npm install husky lint-staged --save-dev
Configure
package.json
:"husky": { "hooks": { "pre-commit": "lint-staged" } }, "lint-staged": { "*.js": [ "eslint --fix", "git add" ] }
Performance Optimization: Use tree-shaking in Webpack to remove unused code and reduce bundle size.
Managing Configuration: Use
.env
files to store environment variables like API keys and secrets.
Conclusion
Setting up a modern JavaScript development environment involves integrating various tools like Git, Node.js, Webpack, ESLint, and testing frameworks into your workflow. By following the best practices and leveraging automation, you can optimize your development process, ensuring code quality and efficiency. Now, it's time to explore and experiment with these tools to find the setup that best fits your project's needs!
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
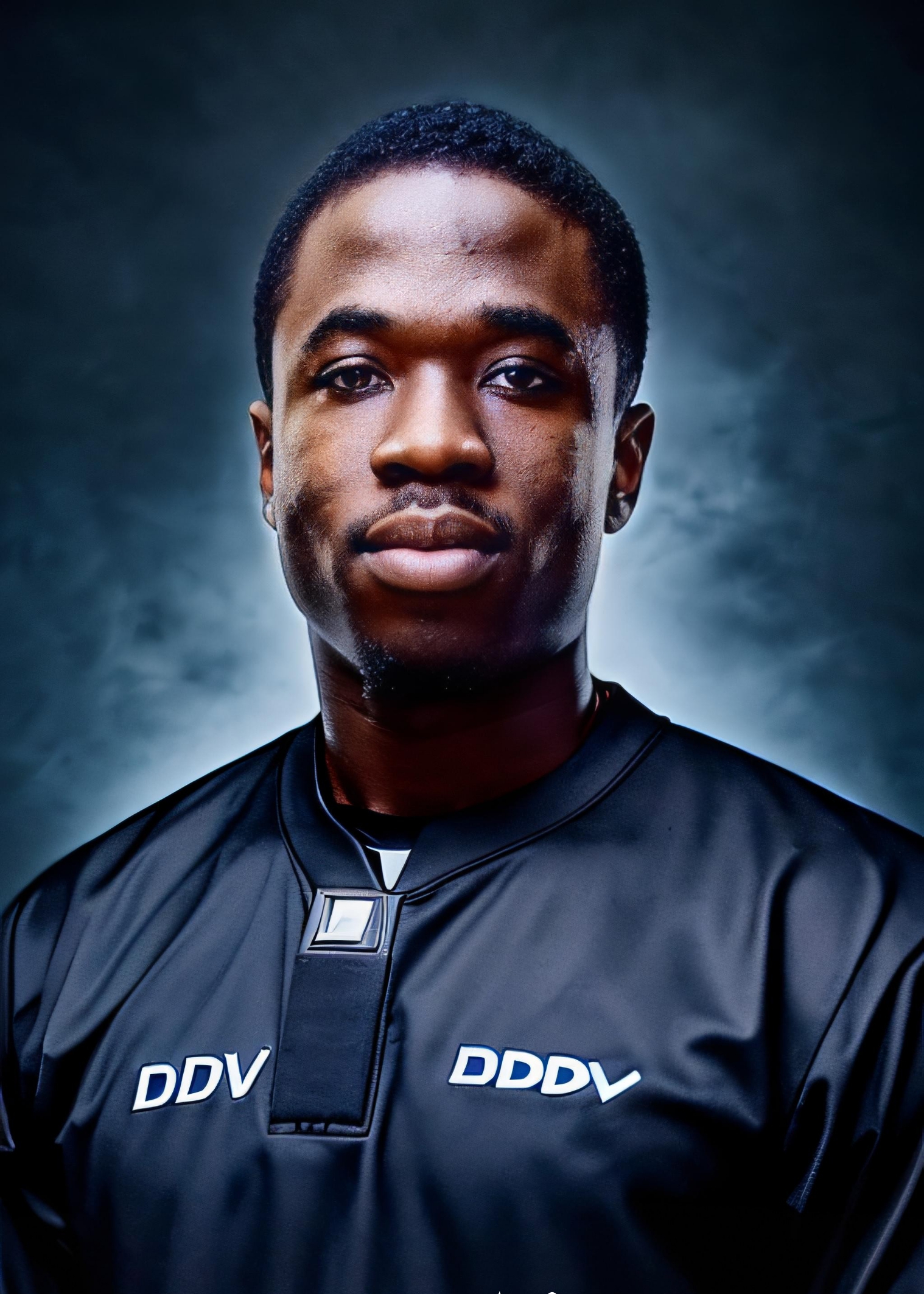
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.