My EJS Learning Experience: From Basics to Fun Projects
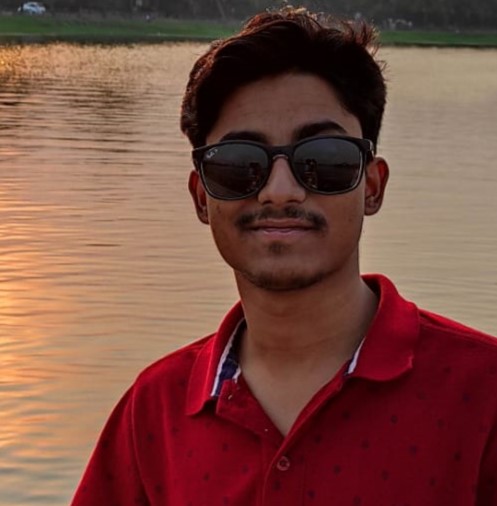
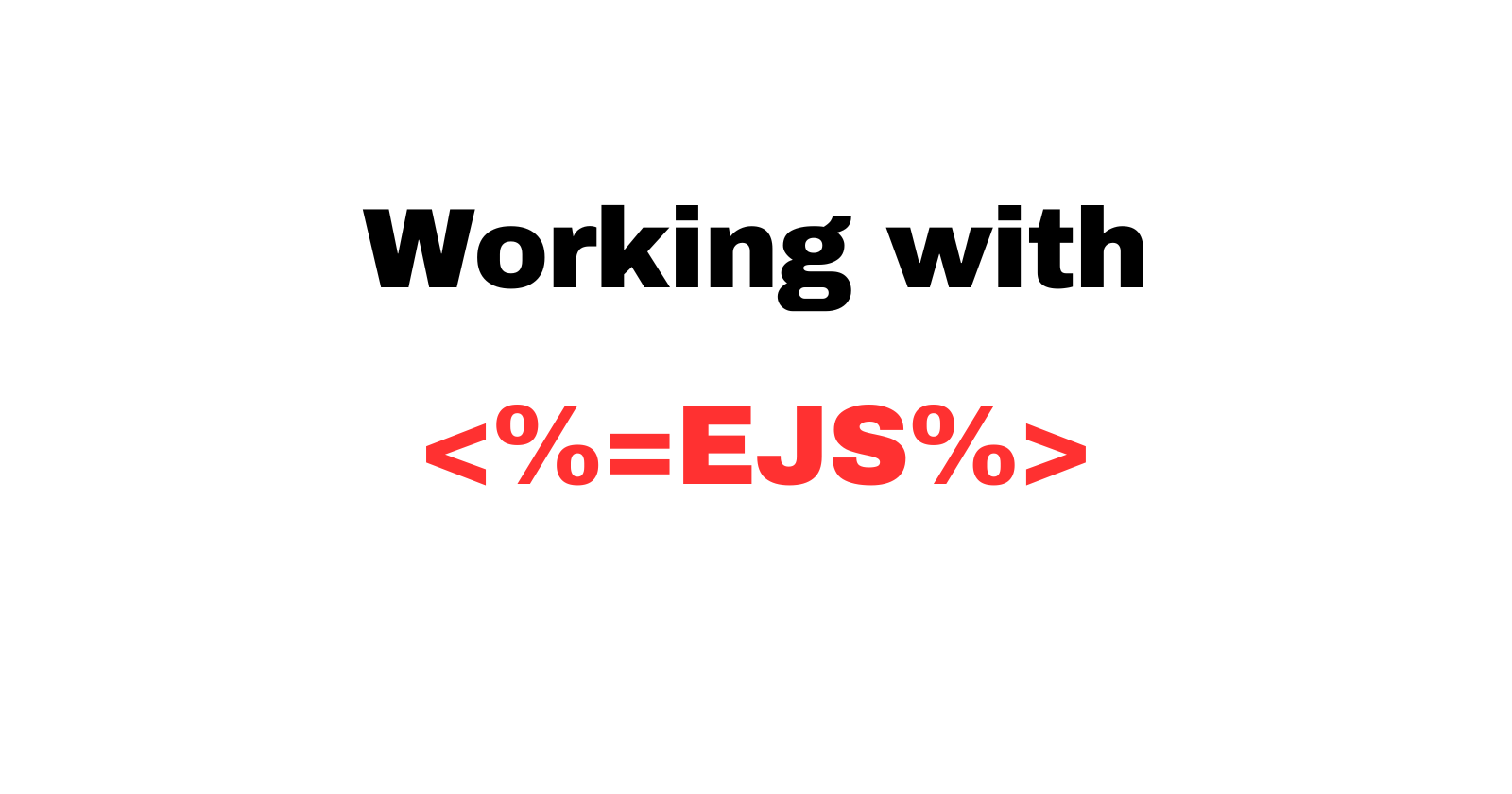
Over the past few days, I’ve been exploring EJS (Embedded JavaScript), a simple but powerful templating language for Node.js. EJS makes it really easy to run JavaScript from HTML. I wanted to share what I’ve learned and the projects I’ve worked on as I get to know this new tool. Let’s discuss the key concepts I learned, the challenges I faced and how I solved them, and some fun projects I built while learning!
What is EJS?
So, what exactly does the "E" in EJS stand for? "Embedded" JavaScript? Sure. But I'd also say it stands for "Easy," "Effective," and "Elegant"! 🤩
EJS is a lightweight templating language that allows you to write JavaScript directly inside your HTML. It’s like merging HTML and JavaScript into one seamless tool. The best part? You don’t need to learn anything fancy or weird, because EJS just uses plain JavaScript! 🧑💻
Unlike other templating engines (like Jinja for Python or Twig for PHP), EJS feels natural and simple. Here's how you can embed JavaScript into HTML using EJS:
<% // JavaScript logic goes here %>
<%= // Outputs a value into the HTML %>
No <script>
tag is needed, and that’s the magic of EJS. It's simple but super flexible. If you’ve used other templating languages before, you’ll find EJS quite intuitive!
Passing Data to EJS Templates
One of the core things I learned early on was how to send data from the server to the EJS template. It's super easy! Here's the basic syntax:
res.render("template_name", { key: value });
This sends the data (key: value
) from the server to your EJS file. Inside your EJS template, you can then access and display the data like this:
<%= key %>
Challenge: Weekday or Weekend Project
As a challenge, I built a project that tells you whether today is a weekday or a weekend. This project gave me a chance to use EJS to display dynamic content.
Here’s the server-side code that figures out the day and sends data to the EJS template:
const today = new Date();
const day = today.getDay();
let type = "a weekday";
let advice = "work hard";
if (day === 0 || day === 6) {
type = "a weekend";
advice = "have fun!";
}
res.render("index.ejs", { daytype: type, advice: advice });
The EJS template will display whether it's a weekday or weekend, and give you advice based on the day!
Getting Deeper into EJS Syntax
EJS offers various tags that let you control how you inject or manipulate data in your templates. Here's a quick breakdown of some important tags:
<%
: Scriptlet tag for logic (control-flow) with no output.<%=
: Outputs the value into the HTML (escaped for security).<%-
: Outputs unescaped HTML (be careful with this!).<%#
: Comment tag, which doesn’t output anything.<%%
: Outputs a literal<%
.
Project: Display a List Based on Odd or Even Seconds
In this fun project, I used EJS to display a list of fruits based on whether the current second is odd or even. If the second is even, it displays a list; otherwise, it shows a message saying, "No items to display."
Here’s how it worked:
<h1><%= title %></h1>
<p>Current second: <%= seconds %></p>
<% if (seconds % 2 === 0) { %>
<ul>
<% items.forEach((item) => { %>
<li><%= item %></li>
<% }) %>
</ul>
<% } else { %>
<p>No items to display</p>
<% } %>
Based on the current second, the template either renders the list or a message. It's a simple yet powerful example of EJS logic in action.
Handling Data in EJS Templates (Safely!)
One thing that caught me off guard was that EJS doesn’t automatically check if the data you’re trying to use exists. If the data is missing, your app might crash! 😅
Here’s how to handle it safely:
<% if (locals.data) { %>
<%= data %>
<% } else { %>
<p>Data is not available</p>
<% } %>
This way, you can prevent your app from breaking by checking if the data is available before trying to use it. Super helpful, right?
Creating Partials with EJS
Now let’s talk about partials — one of the coolest features of EJS. They allow you to reuse HTML code across multiple views, which is especially handy for headers, footers, and other repetitive parts of your site.
For example, you wouldn’t want to rewrite the same header and footer on every page, right? So, instead of duplicating code, you can create a partial for the header and footer, and include them like this:
<%- include("header") %>
<!-- View-specific content goes here -->
<%- include("footer") %>
This way, your header and footer will be included in every view without the hassle of rewriting the same code.
Challenge: Creating a Portfolio with Partials
To practice partials, I built a mini-portfolio site with multiple views (Home, About, Contact). I used the same header and footer across all views using partials. Here’s the process I followed:
Rendered the home page (
index.ejs
).Linked the static files (CSS, JS).
Created routes for About and Contact pages.
Used partials for the header and footer across all views.
You can check out the full code here: Portfolio with Partials Project.
Final Wrap-Up Project: Band Name Generator
Finally, I wanted to combine everything I learned into a fun project — a Band Name Generator! 🎸 This project generates random band names and displays them on a stylized webpage.
Here’s a snippet of the code:
app.get("/", (req, res) => {
res.render("index.ejs", { bandName: generateBandName() });
});
I ran into an error while working on this project — turns out I forgot to provide the full path for my EJS file, which led to a SyntaxError. Lesson learned! 😅
You can check out the full project here: Band Name Generator Project.
In a nutshell 🥜
EJS makes it easy to inject JavaScript into HTML.
You can pass data between your server and templates seamlessly.
Partials help you reuse code and make your templates DRY (Don’t Repeat Yourself).
Always check if data exists before using it in EJS to prevent crashes.
I’ve loved learning EJS and building fun projects along the way. If you’re just getting started, I hope this post helps you understand EJS and motivates you to try it out for your next project! 🚀
If you’ve got any questions or want to share your own experience with EJS, feel free to comment below! Let’s keep learning together! 💻
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
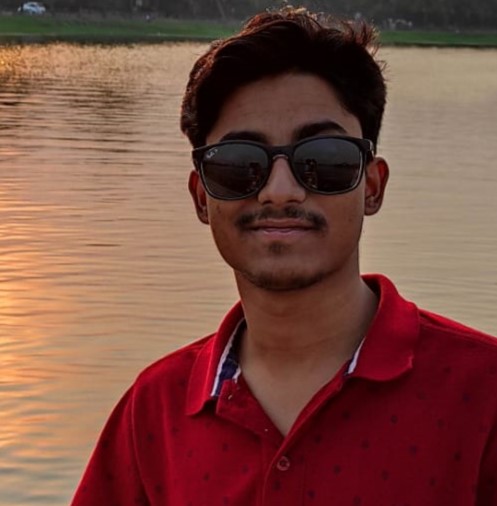
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.