🚀Flutter Performance Hacks: How to Build Faster and More Efficient Apps

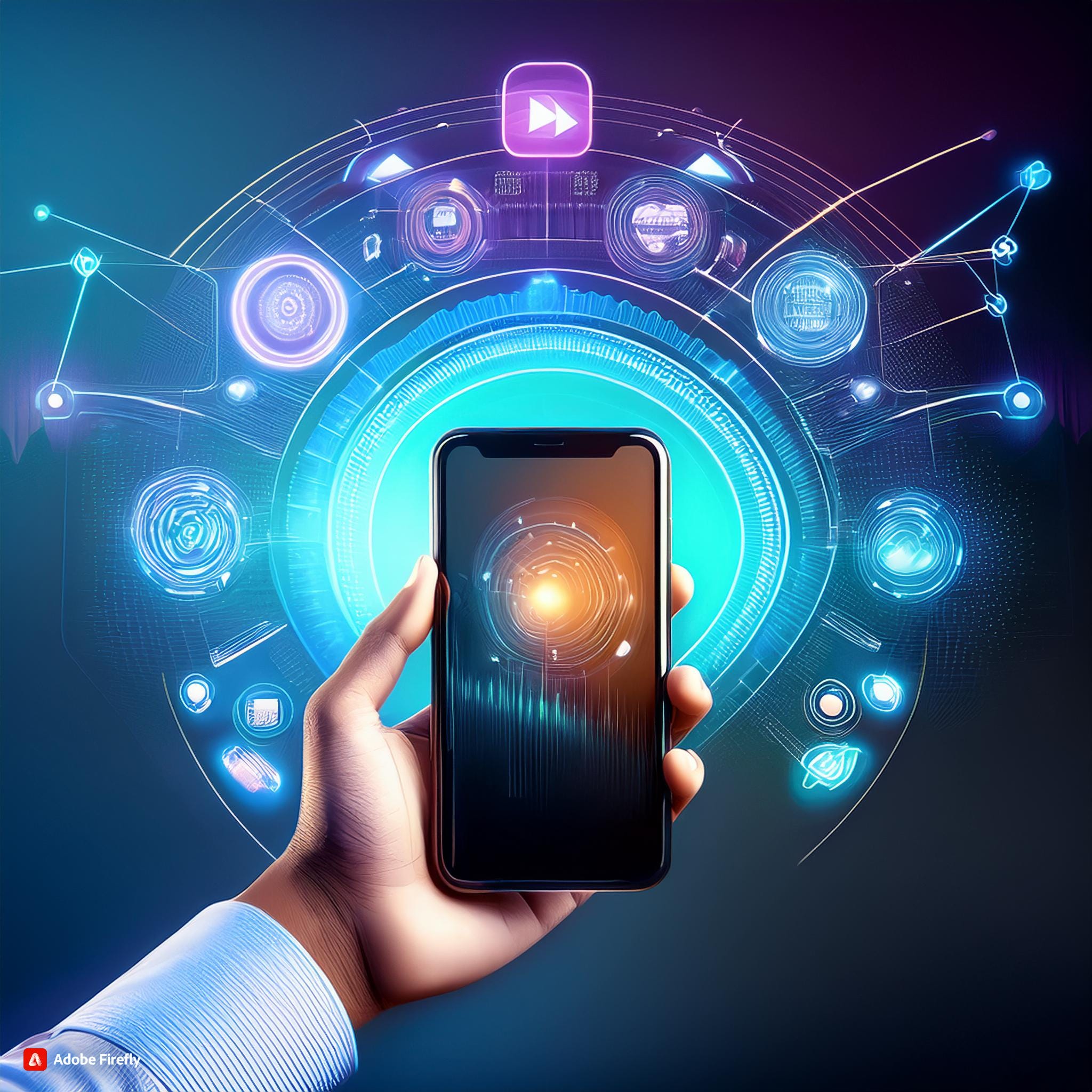
Improving Flutter app performance is crucial to ensuring a smooth user experience, particularly on mobile devices with limited resources. Below are strategies to help you boost the performance of your Flutter app:
1. Minimize Rebuilds
Use
const
Widgets: Wherever possible, declare widgets asconst
. This ensures they are not rebuilt unnecessarily, improving performance.Optimize
setState
Calls: Only update the specific part of the widget tree that needs to change, rather than triggering a rebuild of the entire widget tree.
// Instead of: setState(() { _counter++; });
// Use: setState(() { _counter = newValue; });
- Avoid Unnecessary Builds: Use widgets like
Builder
,Consumer
, orSelector
to avoid rebuilding large parts of the UI unnecessarily.
2. Use Efficient Layouts
Avoid Nested Widgets: Deeply nested widgets can slow down your app. Instead, use lightweight layouts like
Stack
andAlign
to position elements efficiently.Use
ListView.builder
: For long lists, useListView.builder
instead ofListView
, as it only builds the widgets that are visible on the screen.
ListView.builder( itemCount: items.length, itemBuilder: (context, index) { return ListTile(title: Text(items[index])); }, );
3. Reduce Overdraw
Minimize Layers: Avoid placing unnecessary widgets on top of each other. For example, avoid using too many layers of
Container
for styling when a single widget could achieve the same effect.Use Offscreen Layers Sparingly: Widgets like
Opacity
andClipRRect
can create offscreen layers, which may slow down rendering. Only use them when necessary.
4. Optimize Images
Compress Images: Use optimized image formats and compress images to reduce their size. Tools like
Flutter_image_compress
can help.Use
CachedNetworkImage
: For images loaded from the network, use theCachedNetworkImage
package to cache images and reduce load times.
CachedNetworkImage(
imageUrl: "https://example.com/image.jpg",
placeholder: (context, url) => CircularProgressIndicator(),
errorWidget: (context, url, error) => Icon(Icons.error),
);
- Resize Images: Load images at the resolution you need, rather than scaling down large images in the app. Use
Image.asset()
orImage.network()
with a specified size.
5. Leverage Asynchronous Code
Use
FutureBuilder
andStreamBuilder
: Load data asynchronously usingFutureBuilder
andStreamBuilder
, ensuring that UI rendering is not blocked by heavy tasks.Isolate Heavy Computations: For CPU-intensive tasks, use
Isolate
to run the task in a separate thread, keeping the UI thread responsive.
6. Optimize Animations
Use
AnimatedBuilder
: For complex animations, useAnimatedBuilder
to only rebuild the necessary parts of the widget tree.Reduce Animation Overhead: Simplify animations to reduce their impact on performance. Use tools like the
performance overlay
to measure and optimize animation smoothness.
7. Reduce App Size
Tree Shaking: Flutter automatically removes unused code during compilation. To optimize further, ensure you are not importing unnecessary packages or dependencies.
Split the APK/IPA: For Android, you can split your APK by architecture to reduce the download size. For iOS, use
bitcode
and app thinning to achieve a similar result.
flutter build apk --split-per-abi
8. Use Profiling Tools
Flutter DevTools: Use Flutter DevTools to profile your app and identify performance bottlenecks. Analyze CPU, memory, and GPU usage to find areas for improvement.
Performance Overlay: Enable the performance overlay to visualize your app’s frame rendering. Look for jank (frame drops) and optimize accordingly.
// Enable performance overlay in development mode MaterialApp( debugShowCheckedModeBanner: false, showPerformanceOverlay: true, home: MyHomePage(), );
9. Manage App State Efficiently
- Use State Management: Choose a state management solution that fits your app’s complexity. Popular choices include Provider, Riverpod, Bloc, and GetX. Efficient state management reduces unnecessary widget rebuilds and optimizes resource usage.
10. Optimize for Specific Platforms
Use Platform Channels Wisely: When interacting with native code via platform channels, ensure that the communication is as efficient as possible. Minimize the number of calls and batch operations where possible.
Platform-Specific Optimizations: For iOS, ensure that you are using Metal for rendering, as it provides better performance. On Android, optimize for different hardware configurations.
11. Lazy Load Data
- Load Data on Demand: Instead of loading all data at once, fetch it as needed. For instance, use pagination when loading a long list of items from a server.
12. Optimize Text Rendering
Use
TextPainter
: For complex text layouts, consider usingTextPainter
to measure and render text more efficiently.Reduce Custom Fonts: While custom fonts can enhance UI design, they can also increase app size and rendering time. Use system fonts where possible and only load the weights and styles you need.
13. Optimize Network Requests
Batch API Requests: Where possible, batch API requests to reduce the number of network calls.
Use Efficient Caching: Implement caching mechanisms for network responses to avoid fetching the same data repeatedly.
Conclusion
Boosting the performance of your Flutter app involves a combination of code optimization, efficient state management, and mindful use of resources. By implementing these strategies, you’ll be able to create smoother, faster apps that provide a better experience for your users. Always profile your app regularly during development to identify bottlenecks and address them before they become bigger issues.
Happy coding! 🚀
Let’s Connect!
Thanks for reading! If you found this article helpful and want to stay in touch, feel free to connect with me on LinkedIn. I’m always open to discussing tech trends, career advice, or just networking with like-minded professionals. Let’s grow together in this ever-evolving tech landscape!
Subscribe to my newsletter
Read articles from Vineet Nigam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vineet Nigam
Vineet Nigam
"Hey there! I'm Vineet Nigam, your friendly neighborhood Flutter & Android wizard 🧙‍♂️ with 8+ years of brewing mobile magic. From crafting smooth-as-butter user experiences to slaying app performance issues, I’ve been in the trenches building apps like Tata 1mg Consumer and a B2B Rider’s App. I’m all about clean code, smart architecture, and making tech fun. Stick around for some dev tips, tricks, and techy goodness—served with a dash of humor and a whole lot of passion for all things mobile!"