Scraping data from a website
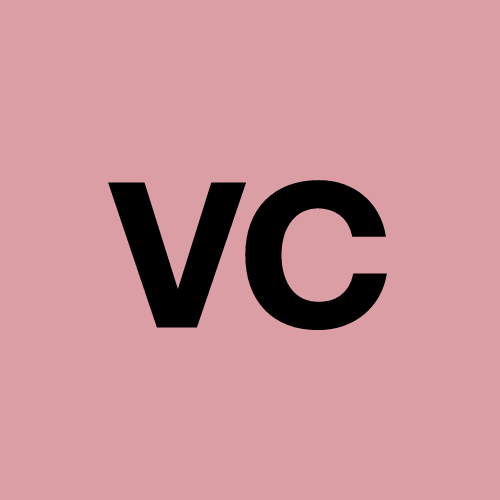
To help you get started with web scraping in Python, I’ll guide you through creating a simple script that scrapes data from a website using the requests
and BeautifulSoup
libraries.
Here’s a basic Python script for scraping a website:
Steps:
Install required libraries.
- You can install them using pip:
bashCopy codepip install requests beautifulsoup4
- Create the Python script to scrape data from a website.
Example Script:
pythonCopy codeimport requests
from bs4 import BeautifulSoup
# Step 1: Define the URL of the website you want to scrape
url = "https://example.com" # Replace with the target URL
# Step 2: Send a request to the website
response = requests.get(url)
# Step 3: Parse the website content using BeautifulSoup
soup = BeautifulSoup(response.content, "html.parser")
# Step 4: Extract the specific data you are interested in
# For example, extracting all the headings (h1, h2, etc.)
headings = soup.find_all(["h1", "h2", "h3"])
# Step 5: Display the scraped data
for heading in headings:
print(heading.get_text())
# Step 6: (Optional) Save the data to a file
with open("scraped_data.txt", "w") as file:
for heading in headings:
file.write(heading.get_text() + "\n")
Explanation:
requests.get(url)
fetches the HTML content of the webpage.BeautifulSoup(response.content, "html.parser")
parses the HTML content.find_all(["h1", "h2", "h3"])
searches for all heading tags (you can adjust this to other tags likep
,div
, etc.).The script prints the headings and saves them to a text file (
scraped_data.txt
).
Modifying the Script:
You can replace
"
https://example.com
"
with the website you want to scrape.To extract different kinds of data (like links, images, or specific sections), modify the
soup.find_all()
part.
If you have a particular website or data structure you'd like to scrape, feel free to share the details, and I can adjust the script accordingly!
Subscribe to my newsletter
Read articles from Vero Chan II directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
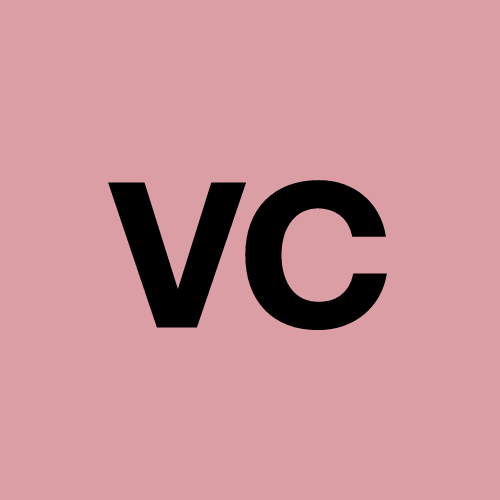