Here’s a new framework to build multi-host distributed systems in Python

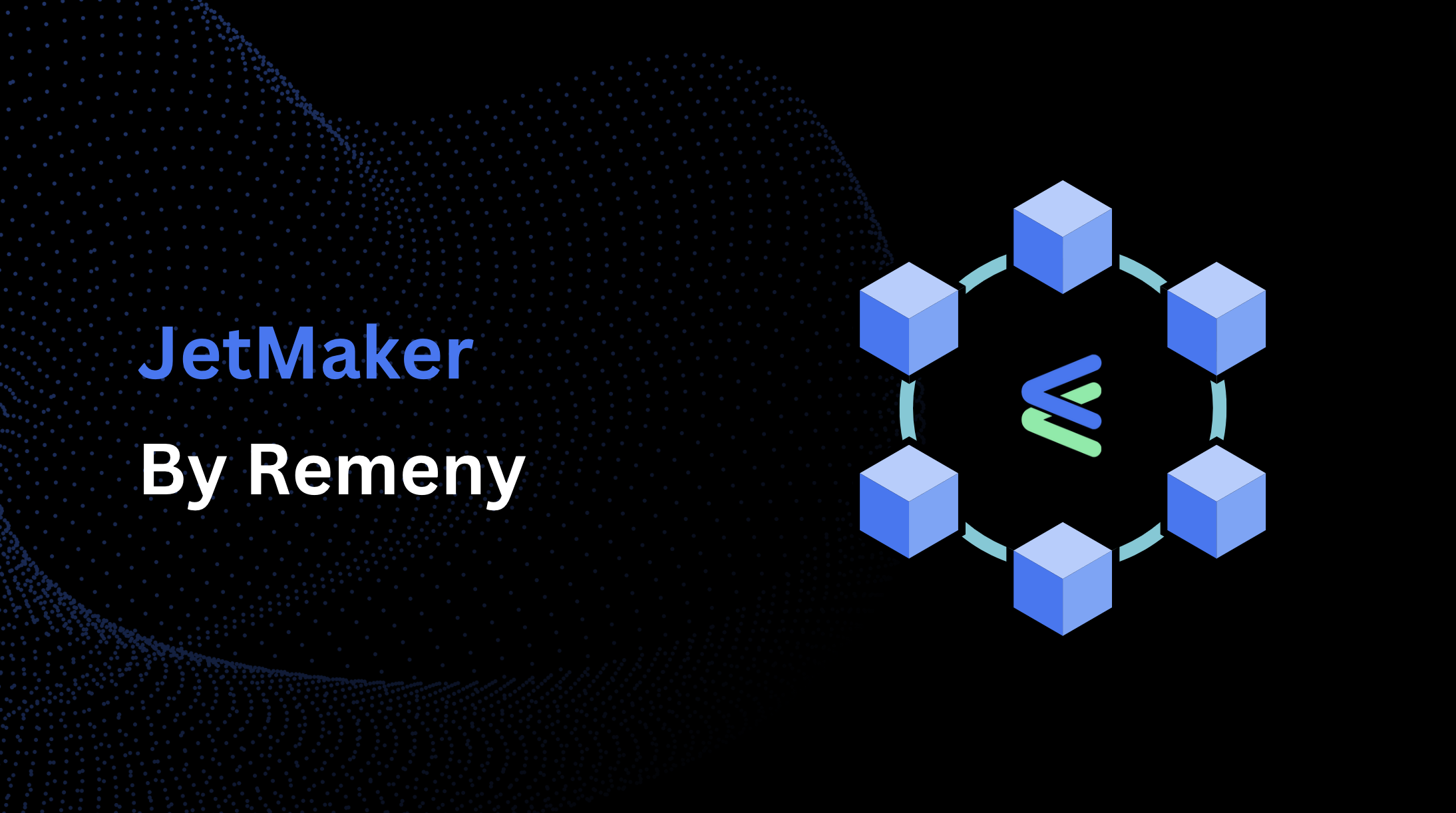
In an interconnected group of distributed nodes in a system, where different nodes want to access each other’s services and data. Such cases can happen in any heterogeneous distributed systems, for example, in simulation environment for AI, rendering nodes and training nodes might not be the same (a very simple example), or you just want different micro-services to access each other.
Using APIs to connect them might be a solution, but it involves more steps like data transformation and configuration to setup.
So we built a simple framework Jetmaker, a new type of networking framework for Python developers to easily let distributed nodes communicate with each other as if they are in the same namespace, we tools to synchronize operations on different nodes are also provided.
So let’s say how to use Jetmaker with simple examples.
Example: Access services
Let’s assume we have two remote nodes
Let install Jetmaker first
pip install jetmaker
Node 1
Use the IP address and port of Node 1 to start this Jetmaker app, the node calling create_app will act as the coordinator node.
from jetmaker import create_app
host = '<you ip address>:<your port>'
pwd = '<your password>'
app = create_app(host=host, password=pwd, join_as='main_node')
create a simple function and a simple stateful object for testing
def recv_string(string):
return f'{string} is received'
class Instance:
def __init__(self) -> None:
self.val = 0
def set_value(self, val):
self.val = val
def get_value(self):
return self.val
instance = Instance()
link it to the app for the network of applications to access
app.share(recv_string, 'recv_str')
app.share(instance, 'ins')
Let this persist if you it always available for access
app.persist()
Node 2
On another remote node, you can join the network of distributed applications and share services of Node 2 or access services of other nodes . Join the app
from jetmaker import join_app
host = '<address of Node 1>:<port of Node 1>'
pwd = '<your password>'
app = join_app(host=host, password=pwd, join_as='other_node')
Access the function shared by Node1
result = app.call('recv_str').run('this string')
-> this string is received
Access the object shared by Node1
instance = app.Object('ins')
print(instance.get_value())
instance.set_value(100)
print(instance.get_value())
-> 0
-> 100
Hope everyone enjoy this ^_^
if you would like more example of using Jetmaker, you can access the link below
Subscribe to my newsletter
Read articles from Gavin Wei directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
