Efficient Database Lookups: Checking if Email Exists in Millions of Records
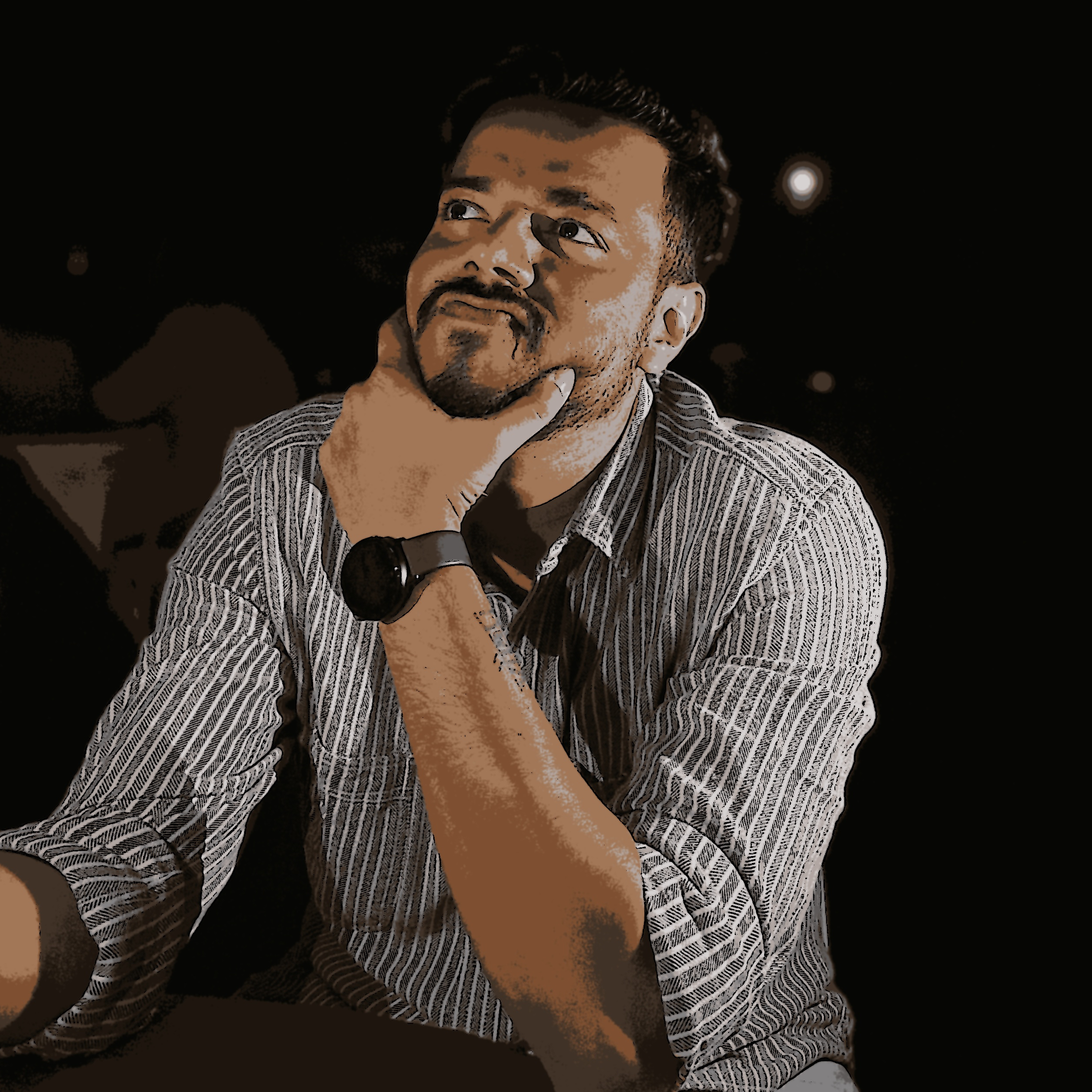
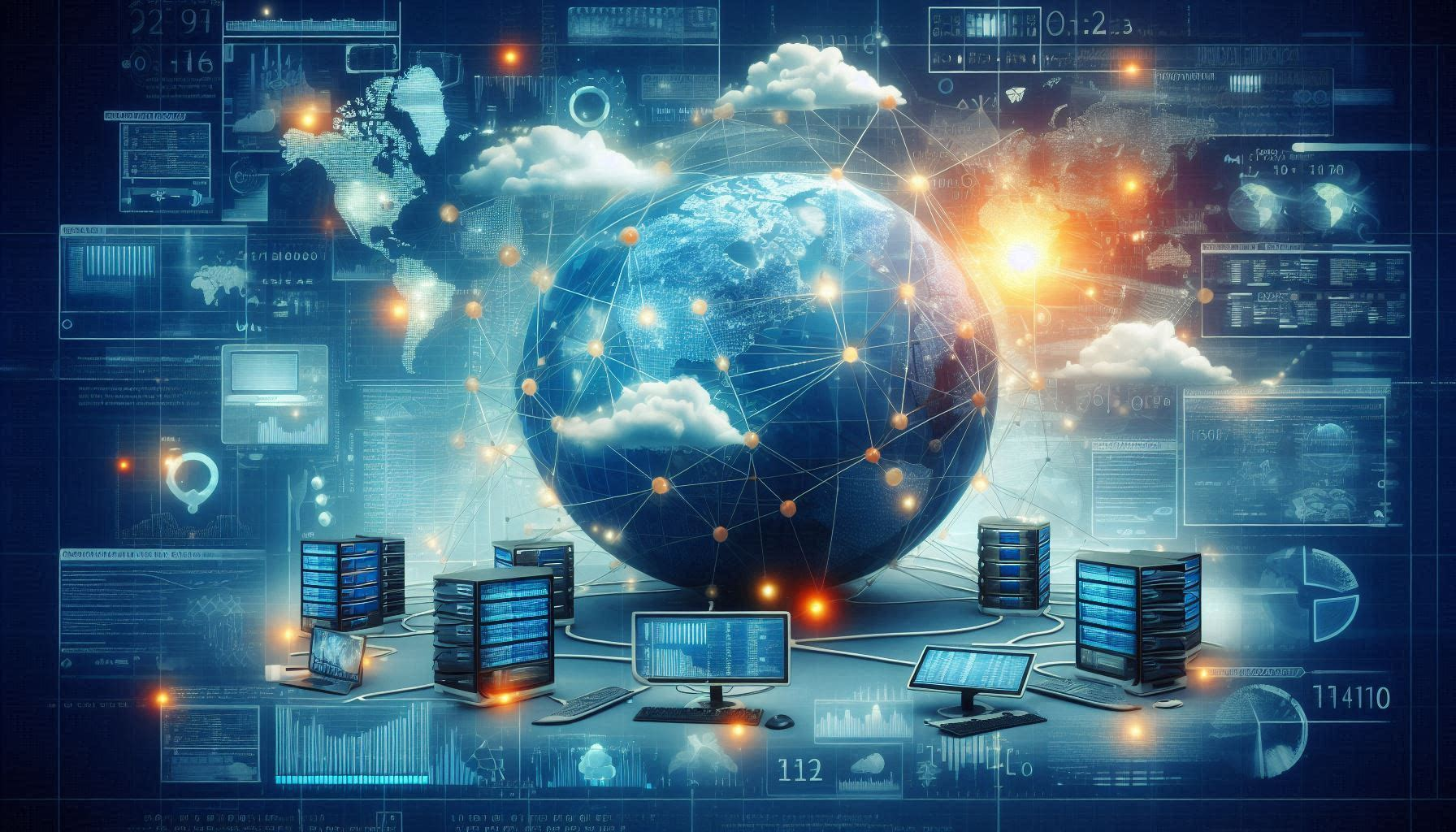
Introduction
Imagine this: Your apps user base has grown rapidly overnight. Suddenly, your fast user registration system is slowing down. The problem? A simple email check to see if a new user's email is already in the database. What used to take milliseconds now takes seconds, frustrating users and possibly losing signups.
This situation is more common than one might think. As apps grow, tasks we once thought were easy can become big problems. Today, we're looking into one such task: email lookups. We'll discuss ways to make this important task faster, starting with single large databases and moving up to distributed sharded systems.
The Problem
Before we dive into solutions, let's understand why email lookups can become a performance bottleneck:
1. Uniqueness Constraint: Emails often serve as unique identifiers in user systems, requiring a check against all existing records for each new registration.
2. High-Frequency Operation: User registration and login are typically high-volume operations, especially for growing applications.
3. Full Table Scans: Without proper optimization, each email lookup might require scanning the entire user table.
4. Concurrent Requests: As user numbers grow, the likelihood of concurrent registration attempts increases, potentially leading to race conditions.
The impact of slow email lookups extends beyond just frustrated users. It can lead to increased server load, higher infrastructure costs, and even lost business if users abandon the registration process due to delays.
As systems scale from thousands to millions of users, the challenge evolves. What works for a modest user base might crumble under the weight of millions of records distributed across multiple database servers.
Optimising Performance in Monolithic Databases
Let's start with options for optimizations for a single, large monolithic database. These strategies can significantly improve performance before the need to consider distributed architectures.
Speed Up Lookups with Effective Indexing
Indexing is often the first and most impactful optimization for database lookups, creating a data structure that allows the database to find specific rows quickly without scanning the entire table. For email lookups, we typically use a B-tree or hash index.
Read More: Common Mistakes That Undermine Index Efficiency
Pros
Dramatically faster lookups, especially for exact matches
Works with existing database systems without additional infrastructure
Cons
Slightly slower insert and update operations
Requires additional storage space
Boost Performance with In-Memory Caching
Caching frequently accessed or recently checked email addresses can significantly reduce database load. Instead of querying the database first, check a fast, in-memory cache. If the email is found in the cache, we can return the result immediately without touching the database.
Pros
Extremely fast lookups for cached emails
Reduces database load for repeated checks
Cons
Requires additional infrastructure
Need to manage cache consistency and expiration
Designing Tables for Faster Email Lookups
Designing the database schema with email lookups in mind can yield significant performance improvements. By creating a separate, denormalised table specifically for email lookups. This table would contain just email addresses and minimal additional information.
CREATE TABLE email_lookup (
email VARCHAR(255) PRIMARY KEY,
user_id INT
);
CREATE UNIQUE INDEX idx_email_lookup ON email_lookup(email);
Pros
Can be highly optimised for read operations
Allows for different storage engines or optimizations without affecting the main user table
Cons
Requires maintaining consistency between two tables
Additional storage space required
Another approach on similar ground that can be used is a key-value storage system for email addresses in the application. Systems like Redis or DynamoDB can serve as the primary storage for email lookups. They are easy to implement, scale efficiently, and provide extremely fast searches. The downside is keeping it in sync with the source of truth.
Enhance Query Performance with Table Partitioning
For very large tables, partitioning can improve query performance by reducing the amount of data scanned. We can divide the user table into smaller, more manageable pieces based on a partition key (e.g., the first letter of the email or a hash of the email). This way, when looking up an email, only the relevant partition needs to be searched.
Read more: Guide to Sharding and Partitioning in Relational Databases
Pros
Improves query performance for large datasets
Can improve overall database performance by distributing I/O
Cons
Adds complexity to database design and maintenance
May require changes to application logic to leverage partitioning effectively
Using Bloom Filters for Efficient Email Checks
A Bloom filter is a space-efficient probabilistic data structure designed to test whether an element is a member of a set. The key characteristics of a Bloom filter are:
Space efficiency: It can represent a large set using very little memory.
Fast lookups: It can determine whether an element is in the set very quickly.
No false negatives: If the Bloom filter says an element is not in the set, it's definitely not in the set.
Possible false positives: The Bloom filter might say an element is in the set when it actually isn't.
Learn more: Bloom Filters by Arpit Bhayani
Steps involved to utilize the Bloom filters would be to
Maintain a Bloom filter containing all email addresses in the database.
Check the filter before querying the database.
If the Bloom filter says the email doesn't exist, it's guaranteed not to be in the database.
If the Bloom filter says it might exist, perform a database query to confirm.
Pros
Very fast initial check.
Space-efficient.
Can significantly reduce unnecessary database queries.
Cons
Requires periodic rebuilding to stay in sync with the database.
Possibility of false positives (but no false negatives).
Transition to Distributed Systems
As the user base grows from millions to tens or hundreds of millions, a single database server, no matter how optimized, may no longer suffice. At this point, we might need to consider a distributed architecture.
The problem statement will also evolve as we move from a single to sharded distributed systems. Now when a new user attempts to register, we need to ensure that their email address is not already in use across any of the shards.
Ensuring Email Uniqueness Across Shards
Implement a global unique index for email addresses across all shards. This involves maintaining a separate database or service that stores all email addresses as unique keys. Before adding a new user to any shard, this index needs to be checked to see if the email exists. If it doesn't exist, add it to the global index and proceed with user creation. If it does exist, reject the registration.
Pros
Guarantees uniqueness across all shards.
Relatively simple to implement and understand.
Cons
Creates a single point of failure.
Can become a bottleneck as the system scales.
Increases latency due to an additional lookup.
Distributing Load with Consistent Hashing
Using consistent hashing allows us to always route emails to the same shard. This is done by hashing the email address with a consistent hashing function. The hash determines which shard handles that email. When checking for uniqueness, only that specific shard needs to be queried.
Pros
Distributes the load across shards.
Reduces the number of nodes that need to be queried.
Scales well as new shards are added.
Cons
Can lead to uneven distribution if the hashing function isn't well-designed.
Rebalancing data when adding or removing shards can be complex.
Leveraging Distributed Caching for Scalability
Extending our caching strategy from the single database to a distributed environment can help maintain performance as we scale. A distributed cache (e.g., Redis Cluster) that all application servers can access and help in quickly identifying the records which already exists in the system.
Pros
Scales well with distributed architecture
Can significantly reduce database load
Cons
Adds complexity to the system
Requires careful management of cache consistency
Combining Consistent Hashing and Bloom Filters for Optimal Performance
For a system with millions of users, one of the best approach would be a combination of Consistent Hashing and Bloom Filters. Here's how it would work:
Use consistent hashing to determine which shard is responsible for a given email address.
Maintain a Bloom filter for each shard, containing all emails in that shard.
When a new registration comes in:
Use consistent hashing to identify the target shard.
Check the Bloom filter for that shard.
If the Bloom filter indicates the email might exist, perform a database lookup on that shard.
If the Bloom filter indicates the email doesn't exist, it's safe to proceed with registration.
This approach provides several benefits:
It distributes the load evenly across shards.
It minimizes the number of database lookups required.
It scales well as new shards are added.
It provides very fast lookups in the common case where an email is new.
Best Practices for Implementing the Recommended Combo
Regularly rebalance the Bloom filters to maintain their effectiveness.
Implement a background process to sync the Bloom filters with the actual data in the shards.
Consider using a hierarchical approach for the Bloom filters (e.g., one global Bloom filter and then shard-specific ones) to further optimize lookups.
Conclusion
Optimising database lookups is a journey that evolves with the system's scale. Start with basic indexing and caching in a single database. As its grows, consider denormalisation and partitioning. When it hit the limits of a single database, explore distributed architectures with sharding, Bloom filters, and distributed caching.
Remember, the best solution depends on the specific use case. Always measure performance, understand the bottlenecks, and iterate the approach.
What challenges have you faced? What strategies worked best for you? Do share your thoughts in the comments below!
Subscribe to my newsletter
Read articles from Rajat Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
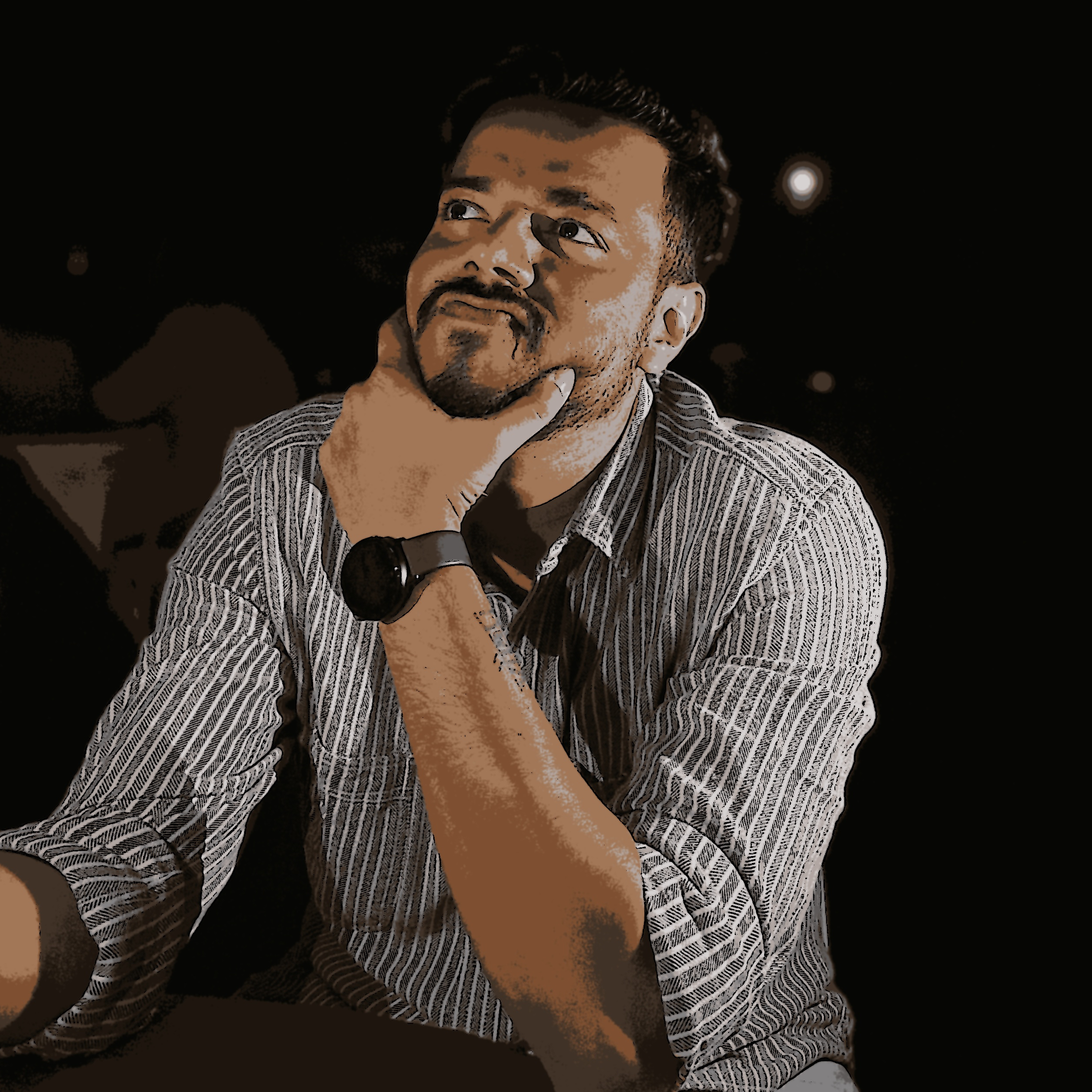