Understanding Angular: Components, Routing, and Directives Explained
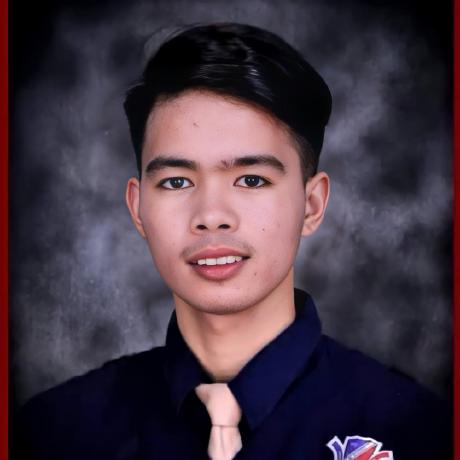
Components
Components are the building blocks of Angular apps. Each component has:
Template: The HTML part of the component.
Class: Contains the logic (written in TypeScript).
Styles: Defines the look of the component
@Injectable({
providedIn: 'root'
})
export class ExampleService {
getData() {
return 'Some data';
}
}
Routing:
Angular uses the Router module to navigate between different pages (or views).
Example of setting up routes:
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: '', redirectTo: '/home', pathMatch: 'full' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
Directives:
Directives add behavior to your HTML elements. Common directives:
*ngIf
: Conditionally display an element.*ngFor
: Loop through an array and display elements.
Example:
<div *ngIf="isVisible">This is visible</div>
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
Subscribe to my newsletter
Read articles from Thirdy Gayares directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
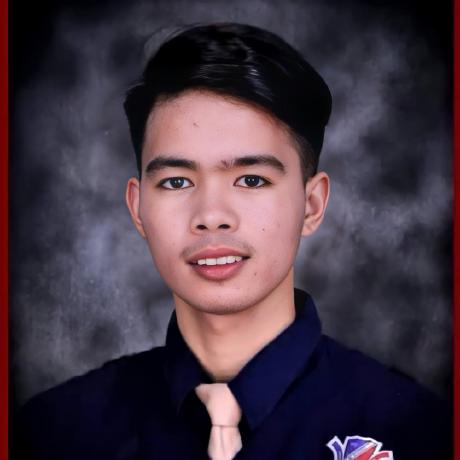
Thirdy Gayares
Thirdy Gayares
I am a dedicated and skilled Software Engineer specializing in mobile app development, backend systems, and creating secure APIs. With extensive experience in both SQL and NoSQL databases, I have a proven track record of delivering robust and scalable solutions. Key Expertise: Mobile App Development: I make high-quality apps for Android and iOS, ensuring they are easy to use and work well. Backend Development: Skilled in designing and implementing backend systems using various frameworks and languages to support web and mobile applications. Secure API Creation: Expertise in creating secure APIs, ensuring data integrity and protection across platforms. Database Management: Experienced with SQL databases such as MySQL, and NoSQL databases like Firebase, managing data effectively and efficiently. Technical Skills: Programming Languages: Java, Dart, Python, JavaScript, Kotlin, PHP Frameworks: Angular, CodeIgniter, Flutter, Flask, Django Database Systems: MySQL, Firebase Cloud Platforms: AWS, Google Cloud Console I love learning new things and taking on new challenges. I am always eager to work on projects that make a difference.