Essential JavaScript Skills for React Development - Part 1

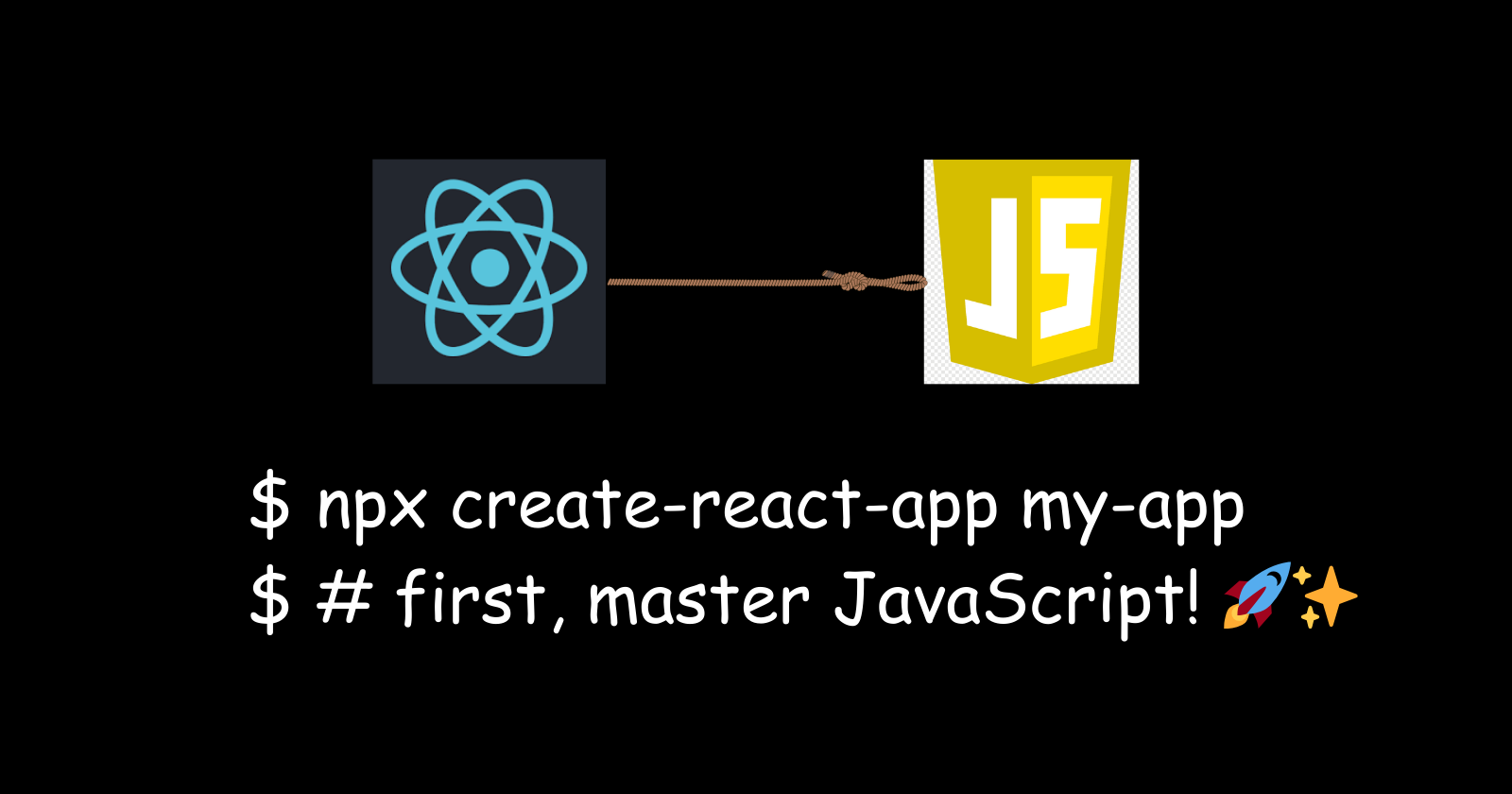
React, developed by Facebook (now Meta), is a popular JavaScript library for building user interfaces, particularly in single-page applications. The key benefit is the reusability of the UI elements due to its component-based structure. However, knowing the basic concepts of JavaScript is crucial to using React efficiently. This blog post explores further key aspects of JavaScript like variable declaration, functions, arrays, objects, conditional statements, array methods, and event listeners, etc. which any aspiring React developer should be familiarized with, to code better and faster.
Basics of JavaScript
One of the fundamental concepts is how variables are declared and managed within different scopes.
Variable Declaration in JavaScript
There are three primary ways to declare variables in JavaScript: var
, let
, and const
. Each has unique characteristics that influence how variables behave in your code.
In case you don’t know, hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope during the compilation phase. This means you can use variables and functions before they are declared in your code.
Key Differences:
Keyword | Scope | Hoisting Behavior | Redeclaration Allowed | Use Case |
var | Function or global | Hoisted, initialized to undefined | Yes | Legacy code or global variables |
let | Block-scoped | Hoisted but in a "temporal dead zone" | No | Variables that change within a block |
const | Block-scoped | Hoisted but in a "temporal dead zone" | No | Constants or non-reassigned variables |
Example: var
Scope: Function or global.
Hoisting: Can be accessed before declaration (value is
undefined
).
console.log(myVar); // undefined
var myVar = 5;
console.log(myVar); // 5
Example: let
Scope: Block-scoped.
Hoisting: Cannot be accessed before declaration (throws
ReferenceError
).
if (true) {
let blockScopedVar = 'Inside block';
console.log(blockScopedVar); // 'Inside block'
}
// console.log(blockScopedVar); // ReferenceError
Example: const
Scope: Block-scoped.
Hoisting: Cannot be accessed before declaration (throws
ReferenceError
).Immutability: This cannot be reassigned, but object properties can still be modified.
const constantVar = 10;
// constantVar = 20; // TypeError
const obj = { name: 'Jay' };
obj.name = 'John'; // Works since object properties are mutable
Functions in JavaScript
Functions are at the heart of JavaScript, serving as essential building blocks for structuring code. Understanding how to define functions, including arrow functions and higher-order functions (HOF), is crucial for effective programming, especially in frameworks like React.
Defining Functions
In JavaScript, functions can be defined in several ways, each with its own syntax and use cases:
1. Function Declaration
A function declaration is a traditional way to define a function. It is hoisted, meaning it can be called before its definition in the code.
function calculateTotalPrice(itemPrice, taxRate) {
const taxAmount = itemPrice * taxRate;
return itemPrice + taxAmount;
}
2. Function Expression
A function expression defines a function as part of a larger expression, often assigning it to a variable. Function expressions are not hoisted, so they cannot be called before their definition.
const calculateDiscountedPrice = function(originalPrice, discountRate) {
const discountAmount = originalPrice * discountRate;
return originalPrice - discountAmount;
};
3. Arrow Functions
Arrow functions provide a more concise syntax for writing function expressions. They are especially useful for shorter functions and can be defined as follows:
const calculateFinalPrice = (basePrice, shippingCost) => {
return basePrice + shippingCost;
};
Arrow functions allow for implicit returns, meaning you can omit the return
keyword for single-expression functions, which enhances readability:
const addTax = (price, taxRate) => price + (price * taxRate); // Implicit return
This syntax is beneficial for improving code readability and reducing boilerplate code.
Higher-Order Functions (HOF)
Higher-order functions are functions that can take other functions as arguments or return functions as their results. This concept may be new to developers coming from other programming languages, where functions are typically standalone. In JavaScript, HOFs are commonly used for operations on arrays, such as map
, filter
, and reduce
(discussed below in array functions).
Custom Higher-Order Function:
A function that takes another function as an argument and applies it to a number.function applyFunction(num, func) { return func(num); } const square = x => x * x; console.log(applyFunction(5, square)); // 25
Function Returning Another Function:
A function that generates a multiplier function based on a given factor.function createMultiplier(factor) { return function(x) { return x * factor; }; } const double = createMultiplier(2); const triple = createMultiplier(3); console.log(double(5)); // 10 console.log(triple(5)); // 15
Event Handling:
A higher-order function that takes a callback to handle an event.
function onEvent(eventName, callback) {
console.log(`Event: ${eventName} occurred.`);
callback();
}
onEvent('click', () => console.log('Button clicked!'));
Arrays and Objects
Arrays and objects are the primary data structures in JavaScript, and mastering them is crucial for effective programming.
Arrays
An array is a collection of elements accessed by numerical indices. You can store various data types in an array, including numbers, strings, and even other objects or arrays. Here’s an example of creating and accessing an array:
const fruitBasket = ['apple', 'banana', 'cherry', 'date'];
console.log(fruitBasket[1]); // Output: banana
Objects
Objects are collections of key-value pairs, where keys are strings and values can be of any data type. Objects are created using curly braces. Here’s how to define an object and access its properties:
const car = {
make: 'Toyota',
model: 'Camry',
year: 2022,
features: ['Air Conditioning', 'Navigation', 'Bluetooth']
};
console.log(car.model); // Output: Camry
Destructuring
Destructuring is a powerful feature that allows you to unpack values from arrays and objects into distinct variables, simplifying your code. For example, you can easily extract values from an array:
const colors = ['red', 'green', 'blue'];
const [primaryColor, secondaryColor] = colors;
console.log(primaryColor); // Output: red
You can also destructure objects:
const user = {
username: 'jay',
email: 'jay@hashnode.dev',
age: 30
};
const { username, email } = user;
console.log(username); // Output: jay
Conditional Statements
Conditional statements are essential for controlling the flow of your application. In JavaScript, theif-else
statement allows you to execute different code blocks based on certain conditions. Here’s a simple example:
const temperature = 75;
if (temperature > 70) {
console.log('It’s a warm day!');
} else {
console.log('It’s a cool day!');
}
The ternary operator is a shorthand forif-else
statements and is particularly useful for inline conditions:
const isRaining = false;
const weatherMessage = isRaining ? 'Take an umbrella!' : 'Enjoy the sunshine!';
console.log(weatherMessage); // Output: Enjoy the sunshine!
Array Methods
Familiarity with array methods is vital for manipulating data in JavaScript, especially in React applications. Here are some commonly used array methods:
map()
: This method creates a new array by applying a function to each element of the original array.const numbers = [1, 2, 3, 4]; const doubled = numbers.map(num => num * 2); console.log(doubled); // Output: [2, 4, 6, 8]
filter()
: This method creates a new array with all elements that pass the test implemented by the provided function.const ages = [15, 22, 18, 30]; const adults = ages.filter(age => age >= 18); console.log(adults); // Output: [22, 18, 30]
reduce()
: This method executes a reducer function on each element of the array, resulting in a single output value.const prices = [10, 20, 30]; const total = prices.reduce((accumulator, currentValue) => accumulator + currentValue, 0); console.log(total); // Output: 60
sort()
: This method sorts the elements of an array in place and returns the sorted array.const names = ['John', 'Alice', 'Bob']; names.sort(); console.log(names); // Output: ['Alice', 'Bob', 'John']
Event Listeners
Understanding how to handle events is critical for creating interactive web applications. Events, such as clicks and key presses, trigger actions in your application. Here’s how to add an event listener in JavaScript:
const button = document.getElementById('myButton');
button.addEventListener('click', () => {
alert('Button was clicked!');
});
Event Bubbling and Capturing
JavaScript events can propagate in two phases: bubbling and capturing. In bubbling, the event starts from the target element and propagates up to the document root. In capturing, the event starts from the document root and propagates down to the target element. You can specify the phase when adding an event listener:
button.addEventListener('click', () => {
console.log('Button clicked during bubbling phase');
}, false); // Bubbling phase
document.addEventListener('click', () => {
console.log('Document clicked during capturing phase');
}, true); // Capturing phase
This blog post covers just the beginning of what you need to know to become proficient in JavaScript. There's much more to explore, and we'll continue our journey in the next part of this series. Stay tuned for more advanced topics and examples that will take your JavaScript skills to the next level!
Subscribe to my newsletter
Read articles from Jay Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jay Patel
Jay Patel
I'm a software engineer who loves bringing ideas to life through code. When I'm not coding, I'm busy dreaming up my next big idea! When I finally take a break, you can find me having long conversations with my friends.