Building a Multi-Currency Crypto HD Wallet for Solana and Ethereum

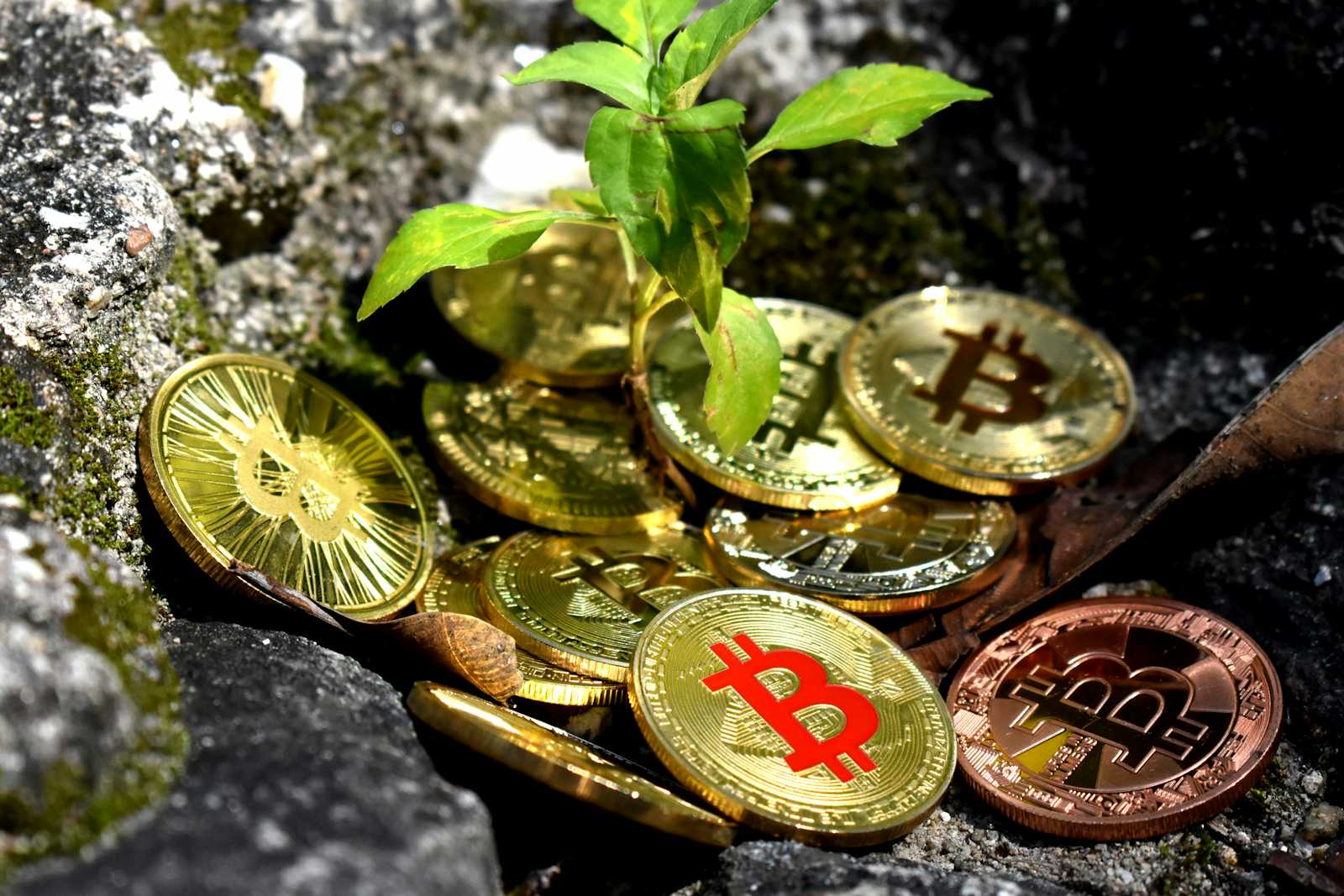
Introduction: What is a Crypto HD Wallet?
- A Crypto HD (Hierarchical Deterministic) Wallet is a type of wallet that allows users to generate multiple addresses from a single seed or mnemonic phrase. These wallets enhance privacy and security by ensuring that each transaction can use a new address while all funds are still accessible with the same seed.
Tech Stack Used
For this project, I used the following technologies:
React.js for building the front-end interface
Crypto libraries such as
ethers.js
(for Ethereum) and@solana/web3.js
(for Solana) to handle wallet generation and transactionsSolana and Ethereum SDKs for integrating both blockchains
Development Process
1. Generating Wallets
For Solana, I used the @solana/web3.js
library to create a new wallet from a randomly generated private key:
import { Keypair } from '@solana/web3.js';
// Generate a new wallet
const wallet = Keypair.generate();
console.log('Public Key:', wallet.publicKey.toBase58());
For Ethereum, I utilized the ethers.js
library to create a wallet from a mnemonic phrase:
import { ethers } from 'ethers';
// Generate a new Ethereum wallet
const wallet = ethers.Wallet.createRandom();
console.log('Ethereum Address:', wallet.address);
This enables users to easily create wallets and view their public addresses for transactions.
2. Dropdown for Currency Selection
One challenge was managing multiple blockchains within the same app. To handle this, I created a dropdown menu that allows users to toggle between their Solana and Ethereum wallets. Based on the selection, the app dynamically renders the respective wallet details.
Here’s the implementation for the dropdown:
const [selectedBlockchain, setSelectedBlockchain] = useState('Solana');
const handleChange = (e) => {
setSelectedBlockchain(e.target.value);
};
return (
<select onChange={handleChange}>
<option value="Solana">Solana</option>
<option value="Ethereum">Ethereum</option>
</select>
);
The selected blockchain is then used to show either the Solana or Ethereum wallet based on user preference.
code: github
Subscribe to my newsletter
Read articles from Nikhil Wagh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
