Mastering Terraform Basics - Day 5
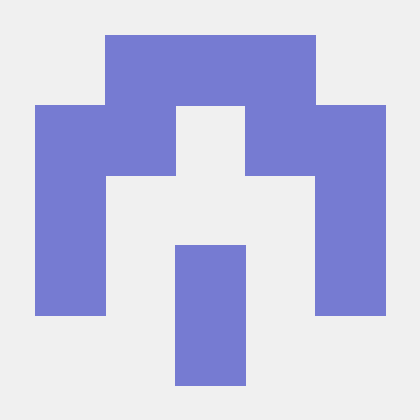
Table of contents
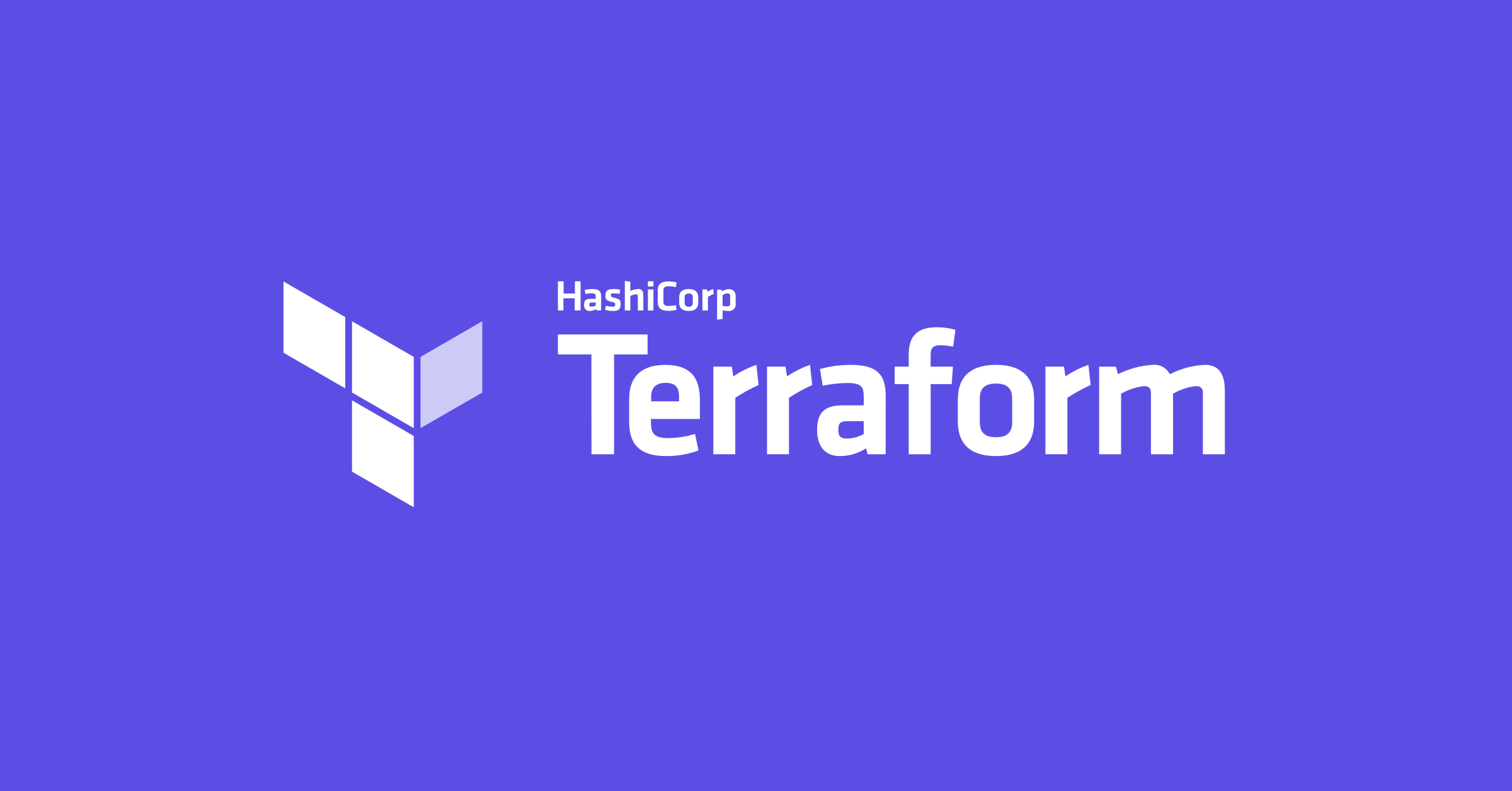
Understanding the Terraform State File: A Comprehensive Guide
The Terraform state file is a critical part of how Terraform operates. It keeps track of the resources Terraform manages and their current state. This guide covers what the state file is, its importance, how it works, best practices for managing it, and how to troubleshoot issues.
What is the Terraform State File?
The Terraform state file (terraform.tfstate
) is a local or remote file that stores metadata about your infrastructure. It acts as a single source of truth for Terraform to manage infrastructure resources. Terraform uses this file to map configuration files to the real-world infrastructure it manages.
Why Does Terraform Need a State File?
Terraform needs to maintain state for the following reasons:
Tracking Resources: The state file tracks each resource (e.g., EC2 instance, S3 bucket) that Terraform manages. Without this file, Terraform wouldn’t know what already exists and what needs to be created or modified.
Performance: Storing state locally allows Terraform to quickly compare the desired state (defined in
.tf
files) with the current state of resources without having to query cloud APIs every time.Dependency Management: Terraform uses the state file to track dependencies between resources, ensuring that resources are created, updated, or destroyed in the correct order.
Handling Complex Infrastructure: For large infrastructures, querying cloud providers each time Terraform runs would be inefficient. The state file stores the data, making operations more efficient.
Structure of a Terraform State File
A Terraform state file is a JSON-formatted document that stores details about your infrastructure. Here’s a basic example of how the state file looks:
{
"version": 4,
"terraform_version": "1.6.0",
"serial": 1,
"lineage": "e41b29a2-486c-47a3-9b8d-b6a4d90562fe",
"outputs": {},
"resources": [
{
"mode": "managed",
"type": "aws_instance",
"name": "example",
"provider": "provider[\"registry.terraform.io/hashicorp/aws\"]",
"instances": [
{
"schema_version": 1,
"attributes": {
"id": "i-1234567890abcdef",
"ami": "ami-0c55b159cbfafe1f0",
"instance_type": "t2.micro",
"availability_zone": "us-west-2b",
...
}
}
]
}
]
}
Key Elements:
version
: The version of the Terraform state file format.terraform_version
: The version of Terraform that created the state file.serial
: A counter that increases each time the state file is modified.resources
: A list of resources managed by Terraform, including their current state, attributes, and IDs.outputs
: The values of output variables declared in the configuration.
How the State File Works
1. Tracking Resource Attributes
When you apply a Terraform configuration, the state file records the real-world attributes of each resource. For example, it stores the instance ID, public IP address, or security group rules for an AWS EC2 instance. This allows Terraform to track resource changes over time.
2. Managing Resource Dependencies
Terraform uses the state file to understand the relationships between resources. For example, if an EC2 instance depends on an Elastic IP, Terraform will ensure the EIP is created before the EC2 instance.
3. Comparing Desired vs. Actual State
During a terraform plan
or terraform apply
, Terraform compares the desired state (from your configuration files) with the current state (stored in the state file). It then calculates the required changes to make the current infrastructure match the desired state.
4. Refreshing State
Terraform can refresh the state by querying the cloud provider (or other infrastructure providers) to check if any manual changes were made outside of Terraform. This helps to reconcile the actual state with the stored state.
Types of Terraform State Files
Local State File:
By default, Terraform stores the state file locally in the working directory as
terraform.tfstate
.This is useful for small, single-user projects but poses challenges for collaboration.
Remote State File:
For team environments, Terraform supports storing state files remotely in a backend such as S3, Azure Blob Storage, Google Cloud Storage, or Terraform Cloud.
Remote state enables shared access and locking, preventing concurrent modifications that could corrupt the state.
Working with the Terraform State File
1. Remote State Backends
Terraform supports a variety of backends to store the state file remotely. Popular options include:
AWS S3: Commonly used for storing Terraform state for AWS infrastructures.
Azure Blob Storage: For storing Terraform state in Microsoft Azure.
Google Cloud Storage: Used in GCP environments.
Terraform Cloud/Enterprise: Terraform’s own managed service for state storage, collaboration, and automation.
Example: Configuring a Remote State Backend with AWS S3
Here’s an example of how to configure an S3 backend in Terraform:
terraform {
backend "s3" {
bucket = "my-terraform-state-bucket"
key = "path/to/my/key"
region = "us-west-2"
encrypt = true
dynamodb_table = "terraform-lock-table"
}
}
In this example:
bucket: The S3 bucket where the state file will be stored.
key: The path within the bucket.
region: The region where the bucket resides.
encrypt: Encrypts the state file in S3 using AWS server-side encryption.
dynamodb_table: Enables state locking by using DynamoDB to prevent concurrent modifications.
2. State Locking
State locking prevents multiple users or processes from modifying the state file at the same time, which could corrupt it. Remote state backends like S3 with DynamoDB or Terraform Cloud support state locking.
3. Terraform State Commands
Terraform provides several commands to manage the state file directly:
a) terraform state list
Lists all the resources managed in the current state file.
terraform state list
b) terraform state show
Shows detailed information about a specific resource in the state file.
terraform state show aws_instance.example
c) terraform state mv
Moves a resource from one location to another in the state file. This is useful for renaming resources or refactoring configurations.
terraform state mv aws_instance.example aws_instance.new_example
d) terraform state rm
Removes a resource from the state file without destroying the actual resource. This is useful if you want to stop managing a resource with Terraform but keep it in your infrastructure.
terraform state rm aws_instance.example
Best Practices for Managing Terraform State
Use Remote Backends for Collaboration:
- If multiple people are working on a Terraform project, use a remote backend to store the state file. This prevents conflicts and allows locking to prevent concurrent state modifications.
Secure the State File:
- The state file may contain sensitive data (like access keys, passwords, or resource details), so it’s important to secure it. Encrypt the state file if you’re using a remote backend and restrict access to it.
Use State Locking:
- Enable state locking when using remote backends to avoid corruption from concurrent operations.
Avoid Manual Edits:
- Don’t manually modify the state file. Terraform provides commands for interacting with the state, and manually changing it can lead to inconsistencies.
Version Control Configuration, Not State:
- Don’t store the state file in version control (e.g., Git). Only track the Terraform configuration files (
.tf
files) in version control, as the state file should be dynamic and environment-specific.
- Don’t store the state file in version control (e.g., Git). Only track the Terraform configuration files (
Back Up the State File:
- Ensure regular backups of the state file, especially if using local storage. For remote backends, rely on the backend provider’s backup mechanisms (e.g., AWS S3 versioning).
Common Issues and Troubleshooting
1. State File Corruption
If the state file is corrupted (e.g., due to manual edits or concurrent access without locking), Terraform will fail to apply changes. The best solution is to restore the state file from a backup.
2. Out-of-Sync State
If resources are created or modified manually outside of Terraform, the state file may become out of sync with the actual infrastructure. To resolve this, run:
terraform refresh
This will update the state file to match the real-world infrastructure.
3. State File Missing
If the state file is deleted or lost, Terraform will attempt to create new resources even if they already exist. This can result in duplicate infrastructure. Ensure that the state file is backed up and stored securely.
terraform output
- Viewing Outputs
terraform output
is used to extract and display the values of output variables defined in your configuration files.
Purpose:
- View the values of output variables after applying the configuration.
Syntax:
terraform output [options] [NAME]
Example:
terraform output
Key Options:
-json
: Produces output in JSON format.
Explanation:
After running terraform apply
, you can use terraform output
to view the output values you defined in your configuration, such as instance IPs or resource IDs.
Tips:
- Useful for retrieving dynamically generated data (e.g., an EC2 instance’s public IP).
terraform taint
- Marking Resources for Recreation (Deprecated in Terraform v0.15)
terraform taint
was used to mark a resource as "tainted," which meant Terraform would destroy and recreate the resource during the next apply
. This command is deprecated in favor of terraform apply -replace
.
terraform graph
- Visualizing Dependencies
terraform graph
generates a DOT format graph of the resources in your configuration. You can use this graph to visualize dependencies between resources.
Purpose:
- Visualize resource dependencies in a configuration.
Syntax:
terraform graph [options]
Example:
terraform graph | dot -Tpng > graph.png
Explanation:
This command generates a graph in DOT format. You can use visualization tools like Graphviz to convert it into a graphical image.
Conclusion
The Terraform state file is a crucial component that enables Terraform to manage infrastructure efficiently. By understanding how the state file works, implementing best practices for state management, and using remote backends, you can ensure smooth, reliable infrastructure as code workflows with Terraform.
Whether you’re working alone or as part of a team, taking care of your Terraform state file will help prevent issues and keep your infrastructure in sync with your desired configurations.
We will be covering the coding scenarios once we get basic understanding on all topics of Terraform.
Subscribe to my newsletter
Read articles from Vikram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
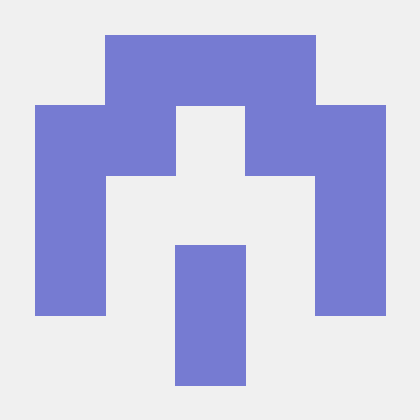