Making APIs with MERN Stack

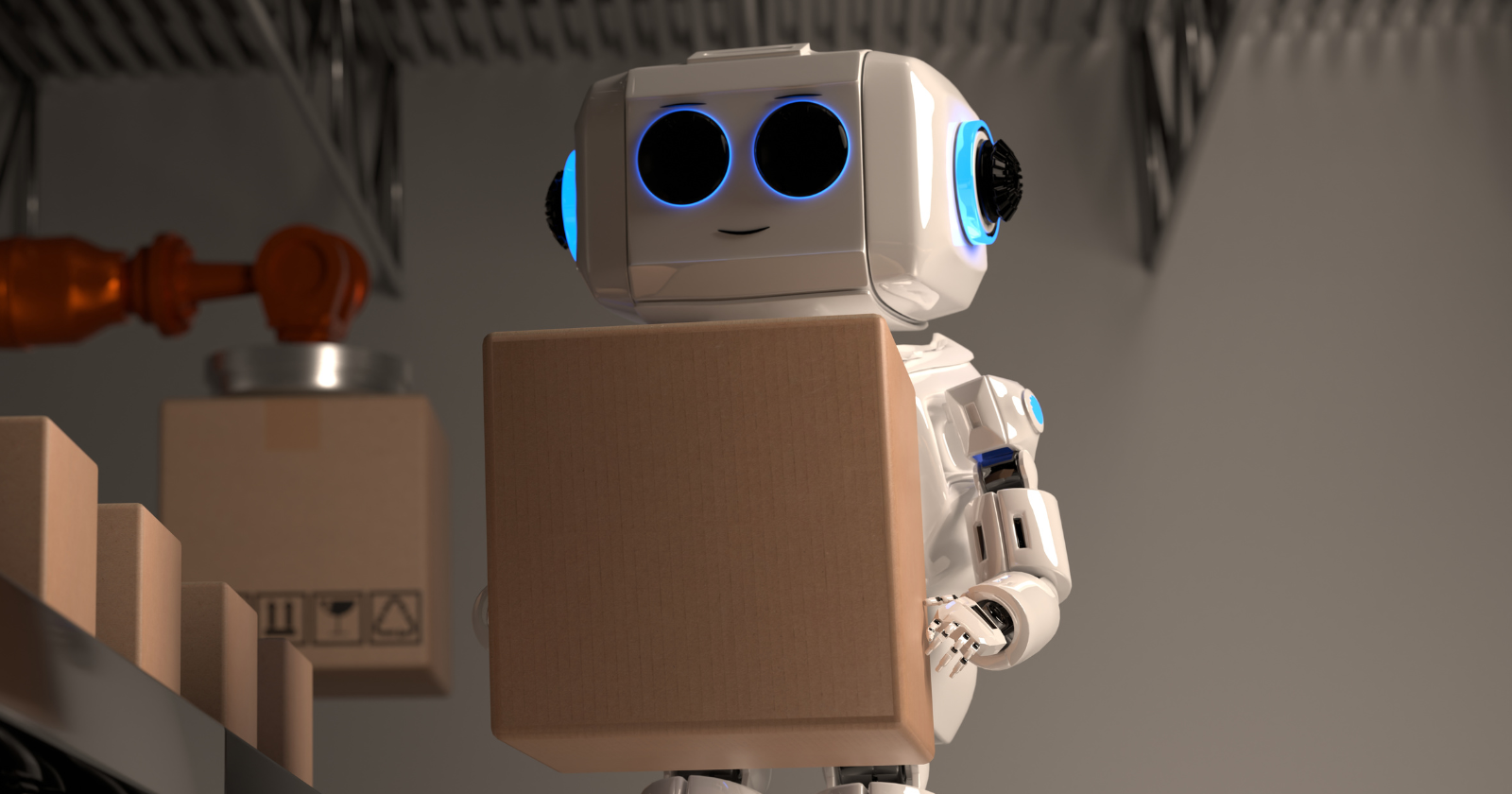
1. Basics of APIs
API = Application Programming Interface
Allows different software to talk to each other
We'll focus on web APIs that use HTTP
2. RESTful API Design
REST = Representational State Transfer
Design style for APIs
Uses standard HTTP methods:
GET: Fetch data
POST: Create new data
PUT/PATCH: Update existing data
DELETE: Remove data
3. MERN Stack Overview
M: MongoDB (database)
E: Express.js (backend framework)
R: React (frontend, not used for API itself)
N: Node.js (JavaScript runtime)
4. Setting Up the Project
Create a new folder for your project
Run
npm init
to start a new Node.js project
Install needed packages:
npm install express mongoose dotenv
5. Creating the Server
- Make a file called
server.js
Basic setup:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 5000;
app.use(express.json());
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
6. Connecting to MongoDB
Create a MongoDB Atlas account (free tier available)
Get your connection string
Create a
.env
file and add your connection string:
MONGO_URI=your_connection_string_here
- In
server.js
, add:
const mongoose = require('mongoose');
require('dotenv').config();
mongoose.connect(process.env.MONGO_URI)
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('MongoDB connection error:', err));
7. Creating a Model
- Make a new folder called
models
Create a file, e.g., Item.js
:
const mongoose = require('mongoose');
const ItemSchema = new mongoose.Schema({
name: { type: String, required: true },
description: String,
price: { type: Number, required: true }
});
module.exports = mongoose.model('Item', ItemSchema);
8. Making API Routes
Create a folder called
routes
Make a file, e.g.,
items.js
:
const express = require('express');
const router = express.Router();
const Item = require('../models/Item');
// GET all items
router.get('/', async (req, res) => {
try {
const items = await Item.find();
res.json(items);
} catch (err) {
res.status(500).json({ message: err.message });
}
});
// POST a new item
router.post('/', async (req, res) => {
const item = new Item({
name: req.body.name,
description: req.body.description,
price: req.body.price
});
try {
const newItem = await item.save();
res.status(201).json(newItem);
} catch (err) {
res.status(400).json({ message: err.message });
}
});
// Add more routes (GET by ID, PUT, DELETE) here
module.exports = router;
In server.js
add:
const itemsRouter = require('./routes/items');
app.use('/api/items', itemsRouter);
9. Testing Your API
Use Postman or any API testing tool
Send requests to
http://localhost:5000/api/items
10. Next Steps
Add authentication (e.g., using JSON Web Tokens)
Create more models and routes
Add input validation
Learn about error handling and best practices
Subscribe to my newsletter
Read articles from QzSeeker directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

QzSeeker
QzSeeker
Frontend Developer | Aspiring MERN Stack Engineer | Building responsive web applications | Open to opportunities