Radix Sort: A Beginner's Guide with C++ Implementation
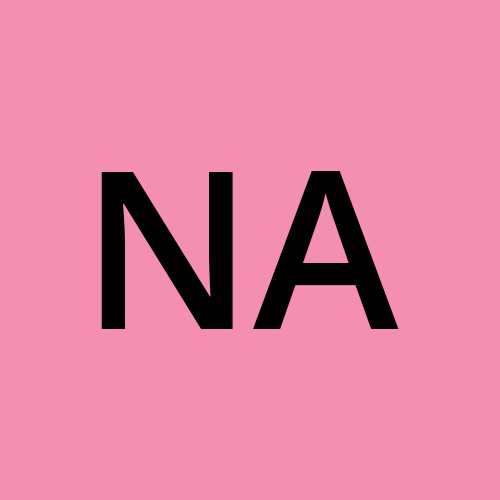
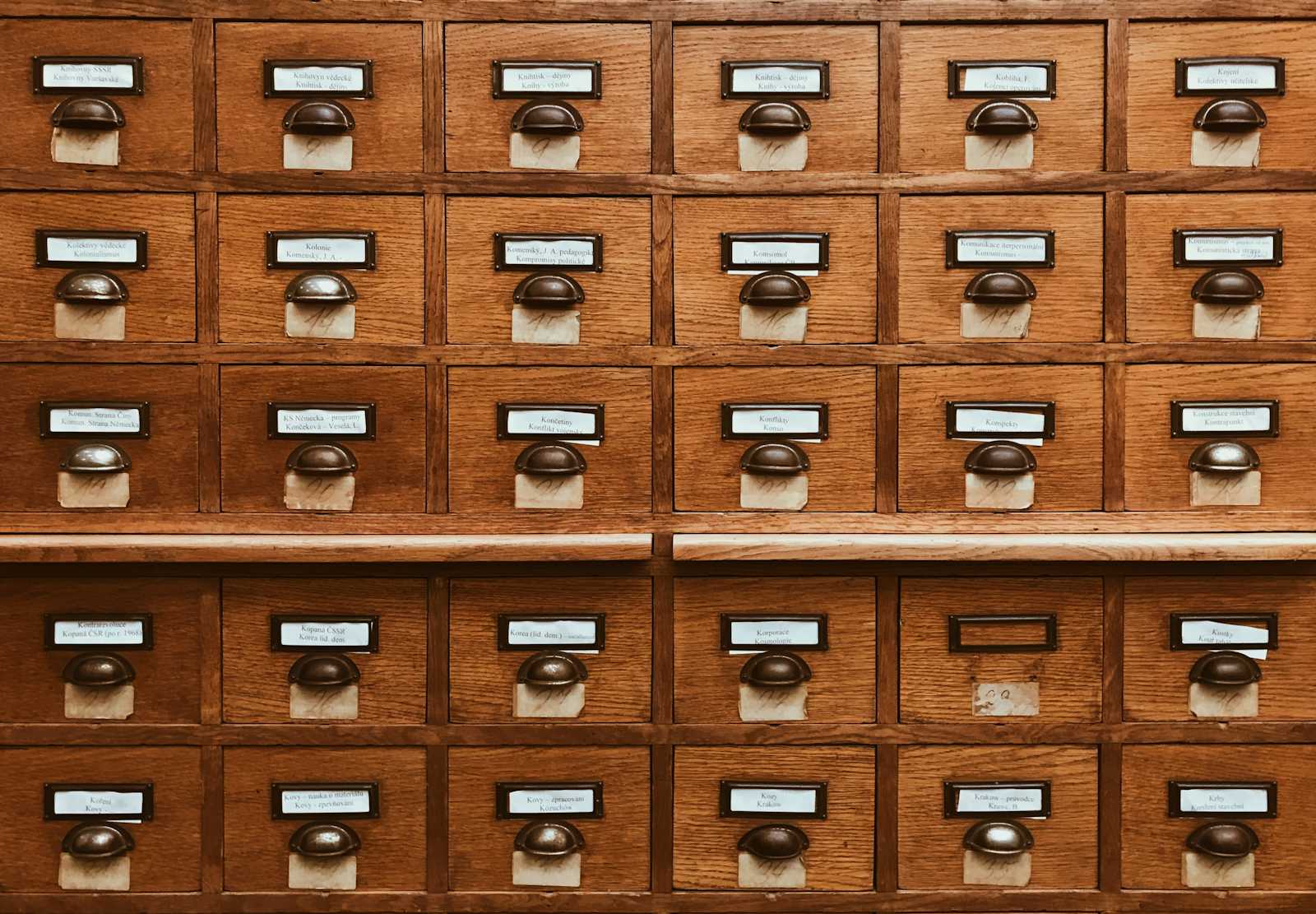
Radix Sort: A Beginner's Guide with C++ Implementation
Introduction
Sorting algorithms are a fundamental part of computer science and are used to arrange data in a specific order. One such powerful yet efficient sorting algorithm is Radix Sort. Unlike comparison-based sorting algorithms (like Quick Sort, Merge Sort, etc.), Radix Sort doesn’t compare elements directly. Instead, it sorts numbers digit by digit, making it an ideal choice for sorting large datasets of integers.
In this blog post, we'll explore what Radix Sort is, how it works, and provide an implementation in C++.
What is Radix Sort?
Radix Sort is a non-comparative sorting algorithm. It works by sorting numbers based on their individual digits, starting from the least significant digit (LSD) to the most significant digit (MSD). This makes Radix Sort a stable sorting algorithm, meaning it maintains the relative order of items with equal keys.
Radix Sort relies on another stable sorting algorithm (usually Counting Sort) as a subroutine to sort the digits of the numbers.
How Radix Sort Works?
Determine the maximum number in the dataset to figure out how many digits the largest number has.
Sort the numbers digit by digit, starting from the least significant digit (rightmost) to the most significant digit (leftmost).
The sorting for each digit is done using a stable sorting algorithm like Counting Sort.
For example, if you have the following array of numbers:[170, 45, 75, 90, 802, 24, 2, 66]
First, sort by the least significant digit (ones place).
Then, sort by the tens place.
Continue until all digits are sorted.
Time Complexity of Radix Sort
Radix Sort runs in O(d * (n + k)) time where:
n
is the number of elements in the input array,d
is the number of digits in the largest number, andk
is the range of the digits (usually 0-9 for decimal numbers).
For large datasets, the time complexity of Radix Sort can outperform other comparison-based sorting algorithms, especially when the range of numbers is small compared to the size of the dataset.
C++ Implementation of Radix Sort
Here’s a step-by-step C++ implementation of Radix Sort:
#include <iostream>
using namespace std;
// Function to get the maximum value in the array
int getMax(int arr[], int n) {
int max = arr[0];
for (int i = 1; i < n; i++)
if (arr[i] > max)
max = arr[i];
return max;
}
// Counting sort based on the digit represented by exp (1 for units, 10 for tens, etc.)
void countingSort(int arr[], int n, int exp) {
int output[n]; // Output array to store sorted numbers
int count[10] = {0}; // Count array to store the occurrences of each digit (0-9)
// Store the count of occurrences of digits
for (int i = 0; i < n; i++)
count[(arr[i] / exp) % 10]++;
// Change count[i] so that it contains the actual position of this digit in output[]
for (int i = 1; i < 10; i++)
count[i] += count[i - 1];
// Build the output array
for (int i = n - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
// Copy the output array to arr[], so that arr[] now contains sorted numbers
for (int i = 0; i < n; i++)
arr[i] = output[i];
}
// Radix sort function
void radixSort(int arr[], int n) {
// Find the maximum number to know the number of digits
int max = getMax(arr, n);
// Apply counting sort to each digit, starting from the least significant digit
for (int exp = 1; max / exp > 0; exp *= 10)
countingSort(arr, n, exp);
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
}
int main() {
int arr[] = {170, 45, 75, 90, 802, 24, 2, 66};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Original array: ";
printArray(arr, n);
radixSort(arr, n);
cout << "Sorted array: ";
printArray(arr, n);
return 0;
}
Explanation of the Code:
getMax Function:
This function returns the maximum element from the array to determine how many digits we need to process.countingSort Function:
Counting Sort is applied based on each digit (units, tens, hundreds, etc.). It sorts the array based on the current digit, which is extracted using(arr[i] / exp) % 10
, whereexp
is the current place value (1 for units, 10 for tens, and so on).radixSort Function:
This is the main function where we loop through each digit place (units, tens, hundreds) and apply the counting sort for each place.printArray Function:
A utility function to print the elements of the array.
Example Run:
Input:[170, 45, 75, 90, 802, 24, 2, 66]
Output:[2, 24, 45, 66, 75, 90, 170, 802]
When to Use Radix Sort?
Radix Sort is most useful when:
You need to sort large numbers of integers.
The number of digits is relatively small compared to the dataset size.
You want an algorithm with guaranteed linear time complexity in such cases.
Conclusion
Radix Sort is an efficient and stable sorting algorithm for datasets where the number of digits is manageable. It outperforms comparison-based algorithms like Quick Sort or Merge Sort when sorting integers in specific cases. The key to its performance is how it sorts numbers by processing individual digits, making it an excellent tool for specific sorting needs.
By understanding how Radix Sort works and implementing it in C++, you can leverage this algorithm in your projects where sorting efficiency is critical.
Subscribe to my newsletter
Read articles from Namra Ajmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
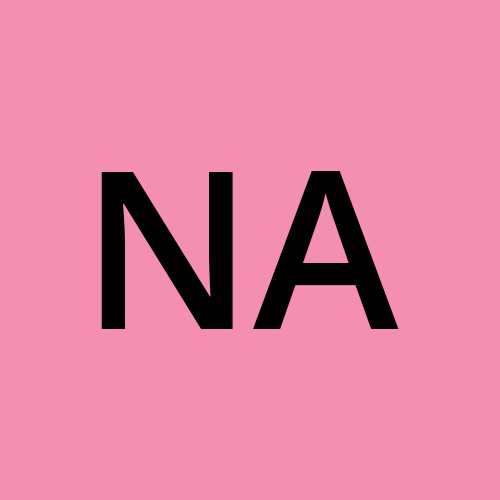