Exploring the Differences Between CommonJS and ES6 Modules in Node.js: A Personal Experience

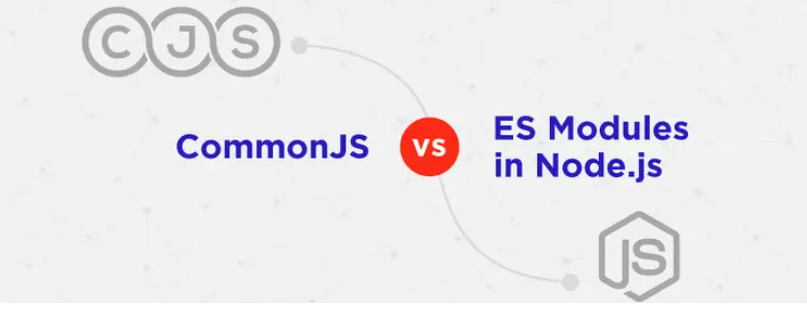
When I first started working with Node.js, I knew I had to get comfortable with its module systems. Like many developers, I began with CommonJS, using the familiar require
syntax to import modules. As I became more confident and started exploring the latest features in JavaScript, I transitioned to ES6 modules with import
. I expected the change to be straightforward, but I quickly learned that there are many differences that can significantly impact the behavior of my code, especially when dealing with asynchronous operations.
The Moment of Realization: Why Is My Output Different?
It all started when I was playing around with a simple Node.js script that used setTimeout
and setImmediate
. I wanted to see how these functions behaved in different scenarios. Here’s a simplified version of the code I was working with:
import { readFile } from "fs"; //const{readFile}=require("fs"); for CommonJS
const a = 100;
setImmediate(() => console.log("SetImmediate"));
readFile("./file.txt", "utf8", () => console.log("Read File CB"));
setTimeout(() => console.log("Time Expired"), 0);
function PrintA() {
console.log("a=", a);
}
PrintA();
console.log("Last Line of File");
When I ran this code using CommonJS (require
), the output was:
a= 100
Last Line of File
SetImmediate
Time Expired
Read File CB
However, when I switched to ES6 modules (import
), the output changed to:
a= 100
Last Line of File
Time Expired
SetImmediate
Read File CB
The Investigation: What's Going On Here?
At first, I was confused. Why was the order different? It’s the same code, just a different way of importing modules. That’s when I decided to dig deeper and understand what was happening under the hood.
Understanding the Event Loop and Task Scheduling
I already knew that both setTimeout
and setImmediate
are used to schedule tasks asynchronously. The key difference is in their timing:
setTimeout(callback, 0)
: Schedules a callback to run after at least 0 milliseconds. It’s placed in the timer queue, meaning it will run as soon as the current call stack is clear and any I/O operations are complete.setImmediate(callback)
: Schedules a callback to run immediately after the current event loop iteration, but before any timers set withsetTimeout
. It’s queued in the check phase of the event loop.
So why did these differences become apparent only when switching between require
and import
?
The Role of Module Systems: CommonJS vs. ES6 Modules
Here’s where it got interesting. It turns out that the way Node.js handles the module system can affect the order in which tasks are processed in the event loop:
CommonJS (
require
): This module system loads modules synchronously. When I userequire
, Node.js processes the script in a straightforward, synchronous manner, leading tosetImmediate
running beforesetTimeout
with a delay of0
. This is becausesetImmediate
is placed in the check phase, which follows the I/O operations.ES6 Modules (
import
): ES6 modules are loaded asynchronously. This subtle shift means that by the time Node.js processes the event loop, it might handlesetTimeout
beforesetImmediate
. The asynchronous nature ofimport
can alter the microtask queue, which in turn changes howsetTimeout
andsetImmediate
are scheduled.
The Takeaway: Predicting Asynchronous Behavior
What I realized from this experiment is that understanding the nuances of the module system you’re using in Node.js is crucial, especially when working with asynchronous code. Here’s what I learned:
Stick to One Module System: To avoid unexpected behaviors, it’s often best to stick to one module system throughout your project. If you’re using
require
, be consistent. The same goes forimport
.Test in the Right Environment: Always test your asynchronous code in the environment it will run. If you’re developing using ES6 modules, make sure to test in an environment that supports them natively, as the behavior might differ from a CommonJS-based environment.
Know Your Tools: Understanding how the event loop,
setTimeout
, andsetImmediate
work is essential for any Node.js developer. Even minor changes, like switching the module system, can have a big impact.
Wrapping Up: My Personal Growth
This experience taught me a lot about the intricacies of Node.js. It’s not just about writing code that works but understanding why it works and how different components interact. As I continue to delve deeper into JavaScript and Node.js, I’m constantly reminded that the smallest details can have the most significant impact.
Whether you’re a seasoned developer or just starting, I encourage you to experiment and question everything. The more you dig into the “why” and “how,” the better you’ll understand the tools you’re using, and the more effective you’ll be in building robust and reliable applications.
What’s Next?
I plan to keep exploring and experimenting with different aspects of Node.js and JavaScript. If you’re interested in this kind of content, stay tuned! I’ll be sharing more of my findings and experiences as I continue to learn and grow.
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SANKALP HARITASH
SANKALP HARITASH
Hey 👋🏻, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. 📬 Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 📧 Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter