JavaScript Looping Techniques: for...of, for...in, and forEach

3 min read
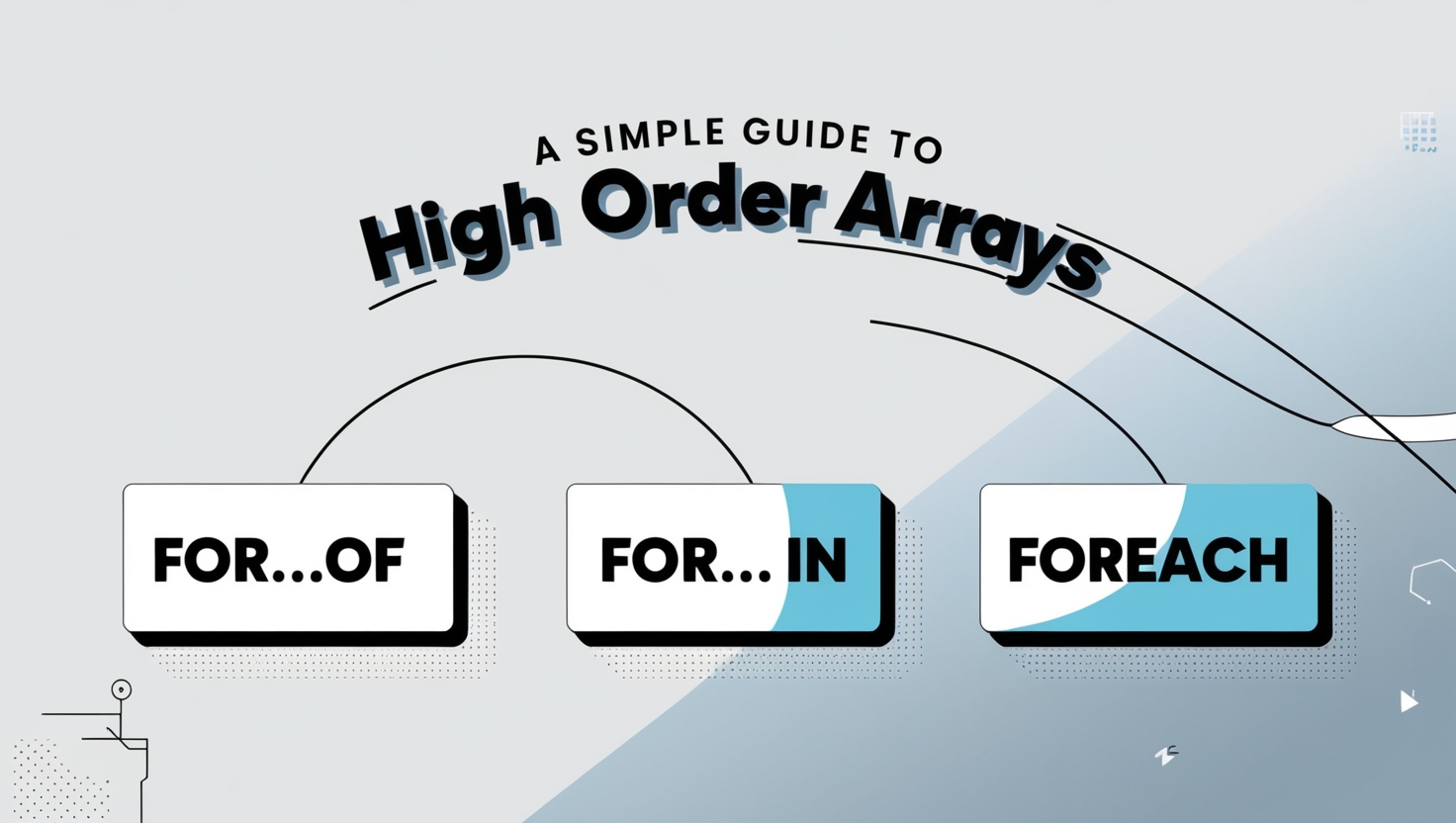
for...of from MDN
The for...of
statement runs a loop that works on a sequence of values from an iterable object. Iterable objects include instances of built-ins such as Array, String, Type Array, Map, Set, NodeList, etc
syntax for…of
for (let variable of iterable)
statement
Array
let arr = [1,2,3,4];
for(let num of arr){
num +=1
console.log(num);
}
2
3
4
5
String
let greeting = "Hello World !"
for(let greet of greeting){
console.log(`charter is ${greet}`);
}
charter is H
charter is e
charter is l
charter is l
charter is o
charter is
charter is W
charter is o
charter is r
charter is l
charter is d
charter is
charter is !
Map
const map = new Map()
map.set('In','India')
map.set('Rus','Russia')
map.set('Rus','Russia') //doublicate is not allowed
for(const [code,country] of map){
console.log(`${country} code is ${code}`);
}
//[code,country] is called array destructure
India code is In
Russia code is Rus
for (const code of map ){
console.log(code);
}
[ 'In', 'India' ]
[ 'Rus', 'Russia' ]
Set
const num = new Set([1,2,3,4,4,5,5])
for(const value of num){
console.log(value);
}
1
2
3
4
5
for...in from MDN
The for...in
statement loops over all enumerable strings.
const myObject = {
js:"Javascript",
cpp:"c++",
rb:"ruby"
}
for(const key in myObject){
console.log(key); //for key
console.log(myObject[key]); //for value
}
js
Javascript
cpp
c++
rb
ruby
for in on Array
const programming = [ "js","cpp","java","python"] for(const key in programming){ console.log(programming[key]); } js cpp java python
why are we able you use for…in on array because at the end array is also an object i.e key-value pair.
const programming = [0: "js",1: "cpp",2:"java",3:"python"]
forEach form MDN
The forEach()
method of Array
instances executes a provided function once for each array element.
const coding = [ "js", "cpp", "java", "python"]
//normal way
coding.forEach(function (value){
console.log(value);
})
//pro way
coding.forEach((value)=>{
console.log(value);
})
//legend way
coding.forEach((value)=> console.log(value))
//---------------------------------
js
cpp
java
python
forEach with function
const coding = [ "js","cpp","java","python"]
function printMe(item){
console.log(item);
}
coding.forEach(printMe)
//need to give only reference not to execute
//-----------------
js
cpp
java
python
forEach with function advance
const coding = [ "js","cpp","java","python"]
function primeMeAll(item,index,arr){
console.log(item,index,arr);
}
coding.forEach(primeMeAll)
//----------------------------
js 0 [ 'js', 'cpp', 'java', 'python' ]
cpp 1 [ 'js', 'cpp', 'java', 'python' ]
java 2 [ 'js', 'cpp', 'java', 'python' ]
python 3 [ 'js', 'cpp', 'java', 'python' ]
💡
use for…of on Array
💡
use for…in on Object
Thank you for reading 😊
Don't copy and paste; try it on your ownRakesh Kumar
0
Subscribe to my newsletter
Read articles from Rakesh kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
