What is JAVA and why DSA
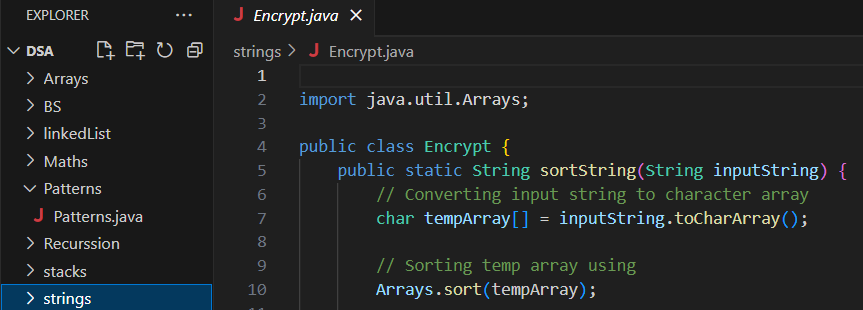
Have you ever thought about why we solve certain questions a particular way? And why, when we submit some questions using simple loops on LeetCode, we get the response "Time Limit Exceeded"? The word you're looking for is time complexity. You've probably heard of it before, but before we dive into this, let me answer something else first.
There’s a huge debate over which language is the best for DSA. As someone who has used C, C++, Java, JavaScript, and Python, let me tell you – it doesn’t matter. If, for you, the language matters, then you're probably focusing on the wrong thing. Personally, I use Java because, in languages like Python and JavaScript, there are built-in functions for many things. But if you want to build your basics and fully grasp DSA, C++ or Java are great choices. I chose Java because I wanted to learn Kotlin too.
So, the language doesn’t matter.
Now, for the introduction: I’m starting a Java + DSA series where I’ll explain everything I’ve learned. Honestly, the reason is simple – I want to improve my learning, and if I can help anyone along the way, it will be an achievement for me.
From the basics to logic building, and all the way through arrays, stacks, queues, dynamic programming, and graphs, let’s start this wild journey together!
Now introduction to java
Java is a powerful, high-level programming language that is widely used in everything from web applications to mobile development. Created by Sun Microsystems in 1995, it’s known for its portability, meaning code written in Java can run on any platform that supports it, thanks to the Java Virtual Machine (JVM). This "write once, run anywhere" principle is one of Java’s strongest features, making it an ideal language for developing cross-platform applications.
Java is also an object-oriented language, meaning it focuses on creating objects that contain both data and methods, which makes code reusable and easier to manage. Concepts like inheritance, polymorphism, encapsulation, and abstraction are key pillars of Java’s design, allowing developers to write modular, organized code.
Despite its simplicity, Java is incredibly versatile. You can use it for:
Web Development: Backend development using frameworks like Spring. Android Android Development: Android apps are typically written in Java (or Kotlin). Enterprise Applications: Java powers many large-scale systems in industries like banking and insurance.
And many more…
Introduction to DSA (Data Structures and Algorithms)
Data Structures and Algorithms (DSA) form the backbone of computer science. While coding languages allow us to write programs, DSA helps us understand how to solve problems efficiently. Whether you're working on a simple program or a complex application, understanding DSA is critical for writing optimized, scalable, and maintainable code.
What Are Data Structures?
A data structure is a way of organizing and storing data in a computer so that it can be accessed and modified efficiently. Some of the most commonly used data structures include:
Arrays: A collection of items stored at contiguous memory locations.
Linked Lists: A sequence of elements, where each element points to the next.
Stacks: Follows a Last-In-First-Out (LIFO) principle.
Queues: Follows a First-In-First-Out (FIFO) principle.
Trees: A hierarchical data structure used for quick searching and sorting.
Graphs: A collection of nodes connected by edges, useful for modeling networks.
Each data structure has its own strengths and weaknesses depending on the type of operations you want to perform, such as insertion, deletion, or searching.
What Are Algorithms?
An algorithm is a step-by-step procedure to solve a problem. It’s not just about getting the correct output but doing so in an optimal way. The efficiency of an algorithm is often measured by its time complexity (how fast it runs) and space complexity (how much memory it uses).
Common algorithms include:
Sorting Algorithms: Such as Bubble Sort, Quick Sort, and Merge Sort.
Searching Algorithms: Like Binary Search, Depth-First Search (DFS), and Breadth-First Search (BFS).
Dynamic Programming: Techniques for solving problems by breaking them into simpler subproblems (e.g., the Fibonacci sequence).
Greedy Algorithms: Making locally optimal choices in the hope of finding the global optimum (e.g., Dijkstra’s algorithm for finding the shortest path).
Why Is DSA Important?
Efficiency: DSA allows you to write programs that perform tasks quickly, saving both time and computing resources. In competitive programming and coding interviews, efficiency often matters as much as correctness.
Problem Solving: Understanding DSA helps you break down complex problems into smaller, more manageable parts, making it easier to develop solutions.
Real-World Applications: DSA is used in various domains, including:
Search engines and databases to efficiently handle large amounts of data.
Networking and routing systems to find optimal paths.
Graphics rendering in games or simulations.
Mastering DSA isn't just about acing interviews – it’s about becoming a more capable and resourceful programmer. As we dive into DSA in this series, we'll explore various data structures, algorithms, and problem-solving techniques that will help you tackle any coding challenge with confidence!
In this series, we won’t get bogged down by installation or setup. You can find plenty of tutorials on YouTube for that. Instead, we’ll focus on core Java concepts, from syntax and OOP to mastering data structures and algorithms. Whether you’re new to Java or just looking to solidify your understanding, this series will guide you through the journey of learning Java and solving complex problems with it.
Installation link - https://www.youtube.com/watch?v=WRISYpKhIrc
Before we dive in big thanks to @Striver he is the real motivation and teacher for me . For me he is the best!!!
Let’s dive in and start coding!
Subscribe to my newsletter
Read articles from Vaishnavi Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by