Understanding the Fundamentals of Go Programming

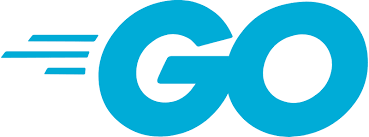
Welcome to the world of Go! This guide is designed to prepare you thoroughly for the basics of Go programming, covering all fundamental concepts. Let's dive in!
Table of Contents
Introduction to Go
Go, also known as Golang, is an open-source programming language developed by Google. It is designed for building fast, reliable, and efficient software. Key features include:
Static Typing and Efficiency: Go is statically typed and compiles to native machine code.
Concurrency Support: Built-in support for concurrent programming with goroutines and channels.
Simplicity and Readability: A clean syntax inspired by C, but with modern features.
Garbage Collection: Automatic memory management.
Setting Up the Go Environment
Download and Install Go
Download Go: Visit the official Go website and download the installer for your operating system.
Install Go: Run the installer and follow the prompts.
Verify Installation:
Open a terminal or command prompt and run:
go version
You should see the installed Go version.
Setting Up GOPATH and GOROOT
GOROOT
: The location where Go is installed. It's usually set automatically.GOPATH
: The workspace directory where your Go projects and dependencies reside.
Set GOPATH
environment variable to your workspace directory, e.g., ~/go
on Unix systems.
Hello World Example
Create a file named main.go
:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Run the program:
go run main.go
Basic Syntax and Structure
Packages: Every Go file belongs to a package.
Imports: Use
import
to include packages.Functions: The
main
function is the entry point.
Example:
package main
import "fmt"
func main() {
fmt.Println("Go Basics")
}
Variables and Data Types
Declaring Variables
Using
var
keyword:var a int = 10 var b = 20 // Type inferred as int var c string
Short Variable Declaration:
d := 30 // Type inferred as int
Data Types
Boolean:
bool
Numeric:
Integers:
int
,int8
,int16
,int32
,int64
,uint
(unsigned)Floating-point:
float32
,float64
Complex numbers:
complex64
,complex128
String:
string
Byte and Rune:
byte
(alias foruint8
)rune
(alias forint32
, represents Unicode code points)
Zero Values
Variables declared without an explicit initial value are given their zero value:
Numeric types:
0
Boolean:
false
String:
""
(empty string)Pointers, functions, interfaces, slices, channels, maps:
nil
Constants
Defined using the const
keyword.
const Pi = 3.14159
const Greeting = "Hello, World!"
Constants cannot be declared using the :=
syntax.
Operators
Arithmetic Operators:
+
,-
,*
,/
,%
Assignment Operators:
=
,+=
,-=
,*=
,/=
,%=
Comparison Operators:
==
,!=
,>
,<
,>=
,<=
Logical Operators:
&&
,||
,!
Bitwise Operators:
&
,|
,^
,&^
,<<
,>>
Control Structures
If-Else Statements
if x > 10 {
fmt.Println("x is greater than 10")
} else if x == 10 {
fmt.Println("x is equal to 10")
} else {
fmt.Println("x is less than 10")
}
Short Statement in If:
if err := doSomething(); err != nil {
fmt.Println("Error:", err)
}
Switch Statements
switch day {
case "Monday":
fmt.Println("Start of the work week.")
case "Friday":
fmt.Println("End of the work week.")
default:
fmt.Println("Midweek days.")
}
Switch with No Expression:
switch {
case x > 0:
fmt.Println("x is positive")
case x < 0:
fmt.Println("x is negative")
default:
fmt.Println("x is zero")
}
For Loops
Basic For Loop:
for i := 0; i < 5; i++ {
fmt.Println(i)
}
While Loop Equivalent:
i := 0
for i < 5 {
fmt.Println(i)
i++
}
Infinite Loop:
for {
// Do something
}
Range in For Loop:
nums := []int{2, 4, 6}
for index, value := range nums {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
Functions
Defining Functions
func functionName(parameterName parameterType) returnType {
// Function body
return value
}
Example:
func add(a int, b int) int {
return a + b
}
Multiple Return Values
func divide(a, b int) (int, int) {
quotient := a / b
remainder := a % b
return quotient, remainder
}
Named Return Values
func split(sum int) (x, y int) {
x = sum * 4 / 9
y = sum - x
return // Returns x, y
}
Variadic Functions
Functions that accept a variable number of arguments.
func sum(nums ...int) int {
total := 0
for _, num := range nums {
total += num
}
return total
}
Anonymous Functions
func() {
fmt.Println("Anonymous function")
}()
Closures
Functions that reference variables from outside their body.
func adder() func(int) int {
sum := 0
return func(x int) int {
sum += x
return sum
}
}
func main() {
pos, neg := adder(), adder()
fmt.Println(pos(1)) // 1
fmt.Println(pos(2)) // 3
fmt.Println(neg(-1)) // -1
}
Deferred Function Calls
Deferred functions are executed after the surrounding function returns.
func main() {
defer fmt.Println("World")
fmt.Println("Hello")
}
// Output:
// Hello
// World
Pointers
Pointers store the memory address of a value.
var x int = 10
var p *int = &x // p points to x
fmt.Println(*p) // Dereferencing p gives the value of x
*p = 20 // Modifies x through the pointer
fmt.Println(x) // x is now 20
- Nil Pointer: A pointer with zero value
nil
does not point to any address.
Arrays and Slices
Arrays
Fixed-size sequences of elements of a single type.
var arr [5]int // An array of 5 integers
arr := [3]string{"a", "b", "c"}
Slices
Dynamic-size, flexible view into arrays.
var s []int // A slice of integers
s = arr[1:3] // Slice from array
// Creating slices
s := []int{1, 2, 3}
// Append to slices
s = append(s, 4, 5)
// Slice capacity and length
fmt.Println(len(s), cap(s))
Slice Internals
Length: Number of elements in the slice.
Capacity: Number of elements in the underlying array starting from the slice's first element.
Maps
Maps are unordered collections of key-value pairs.
m := make(map[string]int)
m["one"] = 1
m["two"] = 2
// Accessing values
value := m["one"]
// Checking for key existence
value, exists := m["three"]
if !exists {
fmt.Println("Key not found")
}
// Deleting keys
delete(m, "two")
Structs
Custom data types that group together fields.
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "Alice", Age: 30}
fmt.Println(p.Name)
}
Methods
Functions associated with a type.
func (p Person) Greet() {
fmt.Printf("Hello, my name is %s.\n", p.Name)
}
func main() {
p := Person{Name: "Bob"}
p.Greet()
}
Pointer Receivers
Methods can have pointer receivers to modify the struct.
func (p *Person) HaveBirthday() {
p.Age++
}
Embedding (Composition)
One struct can embed another, promoting code reuse.
type Employee struct {
Person
EmployeeID string
}
func main() {
e := Employee{
Person: Person{Name: "Carol", Age: 28},
EmployeeID: "E123",
}
e.Greet() // Inherits Greet from Person
}
Interfaces
Interfaces define a set of method signatures.
type Shape interface {
Area() float64
}
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return math.Pi * c.Radius * c.Radius
}
type Rectangle struct {
Width, Height float64
}
func (r Rectangle) Area() float64 {
return r.Width * r.Height
}
func main() {
var s Shape
s = Circle{Radius: 5}
fmt.Println("Circle Area:", s.Area())
s = Rectangle{Width: 4, Height: 6}
fmt.Println("Rectangle Area:", s.Area())
}
- Empty Interface:
interface{}
can hold any type.
Type Assertions
To extract the underlying value from an interface.
var i interface{} = "Hello"
s := i.(string)
fmt.Println(s)
- Type Switches:
switch v := i.(type) {
case string:
fmt.Println("String:", v)
case int:
fmt.Println("Integer:", v)
default:
fmt.Println("Unknown type")
}
Error Handling
In Go, errors are values.
func doSomething() error {
// ...
return errors.New("Something went wrong")
}
func main() {
if err := doSomething(); err != nil {
fmt.Println("Error:", err)
}
}
Custom Error Types
Implement the error
interface.
type MyError struct {
Code int
Message string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error %d: %s", e.Code, e.Message)
}
Concurrency
Goroutines
Lightweight threads managed by Go runtime.
func say(s string) {
fmt.Println(s)
}
func main() {
go say("Hello")
go say("World")
// Wait for goroutines to finish
time.Sleep(time.Second)
}
Channels
Channels are used for communication between goroutines.
c := make(chan int)
// Sending and receiving
go func() {
c <- 42 // Send 42 to channel c
}()
value := <-c // Receive from channel c
fmt.Println(value) // Outputs: 42
- Buffered Channels:
c := make(chan int, 2)
c <- 1
c <- 2
- Channel Direction:
func send(c chan<- int, value int) {
c <- value
}
func receive(c <-chan int) {
value := <-c
fmt.Println(value)
}
Select Statement
Waits on multiple channel operations.
func fibonacci(c, quit chan int) {
x, y := 0, 1
for {
select {
case c <- x:
x, y = y, x+y
case <-quit:
fmt.Println("Quit")
return
}
}
}
func main() {
c := make(chan int)
quit := make(chan int)
go func() {
for i := 0; i < 10; i++ {
fmt.Println(<-c)
}
quit <- 0
}()
fibonacci(c, quit)
}
Packages and Modules
Packages
Code organization in Go is done using packages.
Every Go file starts with
package
declaration.
Importing Packages
import (
"fmt"
"math"
)
Modules
Modules are collections of packages.
Initialize a module:
go mod init module-name
Add dependencies:
go get package-path
Standard Library Overview
Go's standard library provides a rich set of packages:
fmt: Formatting I/O
os: Operating system functionality
io: Basic interfaces to I/O primitives
bufio: Buffered I/O
net/http: HTTP client and server implementations
strconv: String conversions
encoding/json: JSON encoding and decoding
time: Time functionality
math: Basic math functions
sync: Synchronization primitives like mutexes
context: Context propagation for cancellation, timeouts
Testing
Go has built-in support for testing.
Writing Tests
Create a file ending with _test.go
.
package math
import "testing"
func TestAdd(t *testing.T) {
total := Add(2, 3)
if total != 5 {
t.Errorf("Add(2, 3) = %d; want 5", total)
}
}
Run tests:
go test
Benchmarking
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(2, 3)
}
}
Best Practices
Code Formatting: Use
gofmt
orgo fmt
to format code.Naming Conventions: Use CamelCase for functions and variable names.
Error Handling: Check errors explicitly.
Avoid Global Variables: Use local variables or pass variables as parameters.
Write Tests: Ensure code reliability.
Documentation: Comment your code and use
godoc
for documentation.
Conclusion
You've now covered the fundamental concepts of Go programming, from basic syntax to advanced topics like concurrency and interfaces. Go's simplicity and powerful features make it an excellent choice for building efficient and scalable software.
Next Steps:
Practice: Build small projects to apply what you've learned.
Explore Packages: Delve deeper into the standard library.
Concurrency Patterns: Study concurrency patterns for efficient goroutine usage.
Join the Community: Participate in Go forums and contribute to open-source projects.
Additional Resources:
Happy Coding with Go!
Subscribe to my newsletter
Read articles from Sundaram Kumar Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sundaram Kumar Jha
Sundaram Kumar Jha
I Like Building Cloud Native Stuff , the microservices, backends, distributed systems and cloud native tools using Golang