Mastering Test Data Management: Innovative Strategies for Effective Data Handling
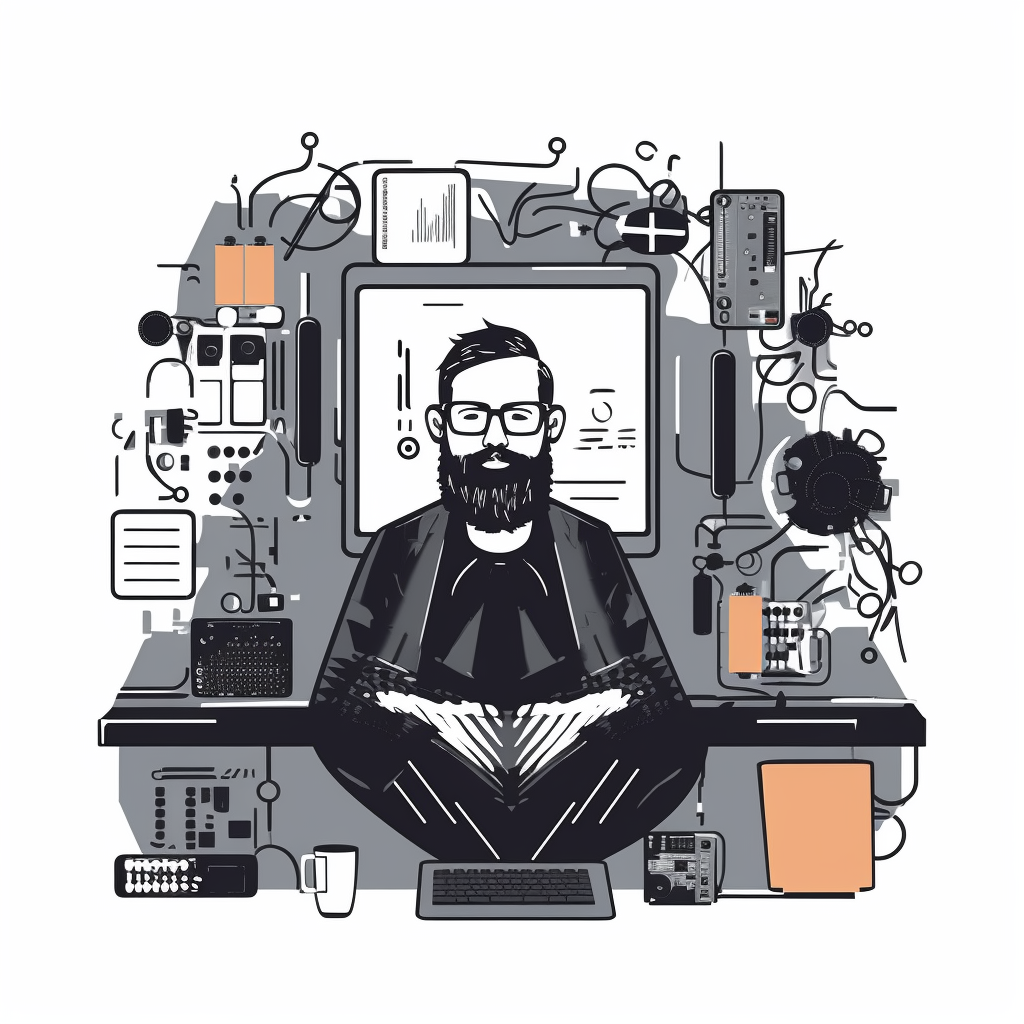
Table of contents
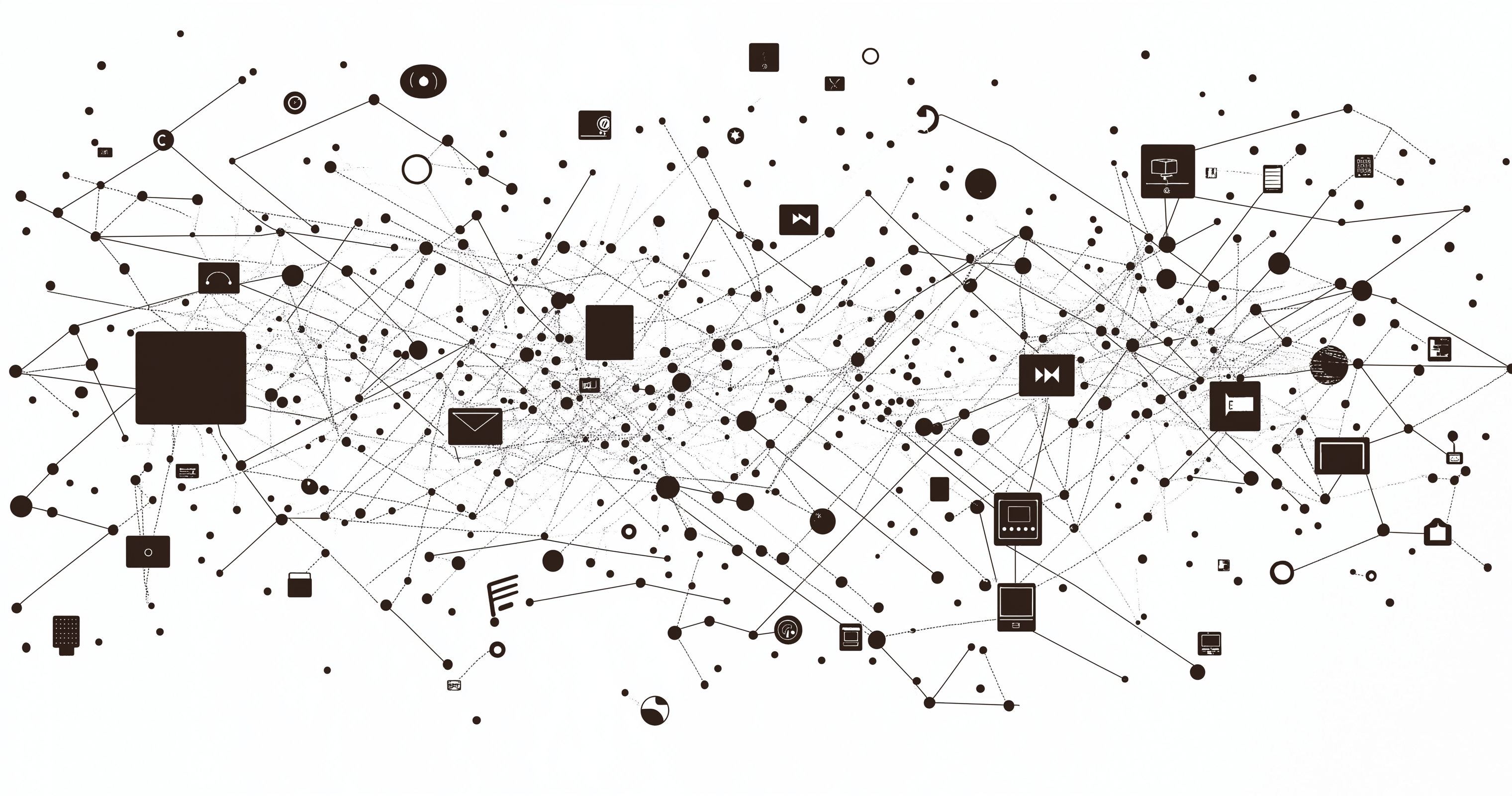
In the ever-evolving landscape of software development, effective test data management is crucial for ensuring the reliability and efficiency of your testing processes. This post explores innovative strategies for managing test data, including data generation, storage, and cleaning techniques. We'll dive into practical scenarios, provide code samples, and discuss the return on investment (ROI) for implementing robust test data management solutions.
The Challenge: Real-World Scenario
Imagine you're working on a large-scale e-commerce platform. Your team is tasked with testing various components, from user authentication to order processing and inventory management. As the project grows, you face several challenges:
Creating realistic test data that covers all possible scenarios
Maintaining data consistency across different environments
Ensuring data privacy and compliance with regulations
Managing large volumes of test data efficiently
Cleaning up test data after each test run to prevent interference
These challenges are common in many software development projects, especially as they scale. Without proper test data management, teams often struggle with unreliable tests, data inconsistencies, and inefficient testing processes. Let's explore how to tackle these challenges with innovative solutions and practical code examples.
Solution 1: Dynamic Test Data Generation
Approach and Reasoning
We chose to implement a dynamic data generation system using Python's Faker library combined with custom logic. This approach allows us to create realistic and diverse test data on-demand, ensuring that our tests cover a wide range of scenarios.
Code Sample
from faker import Faker
import random
fake = Faker()
def generate_user():
return {
"id": fake.uuid4(),
"name": fake.name(),
"email": fake.email(),
"age": random.randint(18, 80),
"address": fake.address()
}
def generate_order(user_id):
return {
"id": fake.uuid4(),
"user_id": user_id,
"products": [generate_product() for _ in range(random.randint(1, 5))],
"total_amount": round(random.uniform(10, 1000), 2),
"status": random.choice(["pending", "processing", "shipped", "delivered"])
}
def generate_product():
return {
"id": fake.uuid4(),
"name": fake.word() + " " + fake.word(),
"price": round(random.uniform(5, 500), 2),
"quantity": random.randint(1, 10)
}
# Generate a dataset
users = [generate_user() for _ in range(100)]
orders = [generate_order(user["id"]) for user in users for _ in range(random.randint(0, 5))]
Advantages
Flexibility: This approach allows easy customization of data generation to match specific business rules or edge cases.
Scalability: You can generate large volumes of data quickly and efficiently.
Realism: Faker provides realistic-looking data, improving the quality of your tests.
Reproducibility: By using seeded random number generators, you can reproduce the same dataset when needed.
Variety: The randomness introduces a wide range of scenarios, helping uncover edge cases.
Solution 2: Containerized Test Environments
Approach and Reasoning
To maintain data consistency across different environments, we've chosen to use Docker to create isolated, reproducible test environments. This approach ensures that every team member and CI/CD pipeline has access to the same test environment with consistent data.
Code Sample
version: '3'
services:
db:
image: postgres:13
environment:
POSTGRES_DB: testdb
POSTGRES_USER: testuser
POSTGRES_PASSWORD: testpass
volumes:
- ./init.sql:/docker-entrypoint-initdb.d/init.sql
test_app:
build: .
depends_on:
- db
environment:
DATABASE_URL: postgresql://testuser:testpass@db:5432/testdb
volumes:
- ./test_data:/app/test_data
Advantages
Consistency: Ensures all team members work with identical environments, eliminating "it works on my machine" issues.
Isolation: Containers provide isolated environments, preventing conflicts with other systems or tests.
Version Control: Docker configurations can be version-controlled, tracking changes to the test environment over time.
Portability: Containerized environments can be easily moved between development, staging, and CI/CD systems.
Scalability: Easy to scale up for parallel testing or to simulate production-like loads.
Solution 3: Data Masking for Privacy and Compliance
Approach and Reasoning
To address data privacy concerns and comply with regulations like GDPR, we've implemented a data masking technique. This approach allows us to work with realistic data structures while protecting sensitive information.
Code Sample
import re
from typing import Dict, Any
class DataMasker:
@staticmethod
def mask_email(email: str) -> str:
username, domain = email.split('@')
return f"{username[0]}{'*' * (len(username) - 2)}{username[-1]}@{domain}"
@staticmethod
def mask_credit_card(cc_number: str) -> str:
return f"{'*' * 12}{cc_number[-4:]}"
@staticmethod
def mask_phone(phone: str) -> str:
cleaned = re.sub(r'\D', '', phone)
return f"{'*' * (len(cleaned) - 4)}{cleaned[-4:]}"
@classmethod
def mask_data(cls, data: Dict[str, Any]) -> Dict[str, Any]:
masked = data.copy()
if 'email' in masked:
masked['email'] = cls.mask_email(masked['email'])
if 'credit_card' in masked:
masked['credit_card'] = cls.mask_credit_card(masked['credit_card'])
if 'phone' in masked:
masked['phone'] = cls.mask_phone(masked['phone'])
return masked
# Usage
original_data = {
"name": "John Doe",
"email": "john.doe@example.com",
"credit_card": "1234567890123456",
"phone": "+1 (555) 123-4567"
}
masker = DataMasker()
masked_data = masker.mask_data(original_data)
print(masked_data)
Advantages
Compliance: Helps meet data protection regulations by obscuring sensitive information.
Flexibility: Can be easily extended to mask different types of data as needed.
Realistic Testing: Maintains the structure and format of data, allowing for realistic testing scenarios.
Security: Reduces the risk of exposing sensitive information during testing or development.
Consistency: Ensures that all sensitive data is masked uniformly across the application.
Solution 4: Efficient Data Storage and Retrieval
Approach and Reasoning
For managing large volumes of test data efficiently, we've implemented a custom data store using SQLite for local development and testing. This approach provides a lightweight, file-based database solution that's easy to set up and use in various environments.
Code Sample
import sqlite3
import json
from typing import List, Dict, Any
class TestDataStore:
def __init__(self, db_path: str):
self.conn = sqlite3.connect(db_path)
self.create_tables()
def create_tables(self):
with self.conn:
self.conn.execute('''
CREATE TABLE IF NOT EXISTS test_data (
id INTEGER PRIMARY KEY,
category TEXT,
data JSON
)
''')
def insert_data(self, category: str, data: Dict[str, Any]):
with self.conn:
self.conn.execute(
"INSERT INTO test_data (category, data) VALUES (?, ?)",
(category, json.dumps(data))
)
def get_data(self, category: str) -> List[Dict[str, Any]]:
cursor = self.conn.execute(
"SELECT data FROM test_data WHERE category = ?",
(category,)
)
return [json.loads(row[0]) for row in cursor.fetchall()]
def clear_data(self, category: str = None):
with self.conn:
if category:
self.conn.execute("DELETE FROM test_data WHERE category = ?", (category,))
else:
self.conn.execute("DELETE FROM test_data")
# Usage
store = TestDataStore("test_data.db")
store.insert_data("users", {"name": "Alice", "email": "alice@example.com"})
store.insert_data("orders", {"id": "123", "total": 99.99})
users = store.get_data("users")
print(users)
store.clear_data("orders")
Advantages
Efficiency: SQLite provides fast read and write operations, suitable for managing large volumes of test data.
Portability: The file-based nature of SQLite makes it easy to share test data across team members or environments.
Flexibility: Storing data as JSON allows for flexible schema changes without needing to modify the database structure.
Categorization: The category-based approach allows for easy organization and retrieval of different types of test data.
Lightweight: SQLite requires no separate server process, making it ideal for local development and testing.
Solution 5: Automated Test Data Cleanup
Approach and Reasoning
To ensure that test data doesn't interfere between test runs, we've implemented an automated cleanup process using Python's unittest
framework. This approach guarantees a clean slate for each test, improving test reliability and consistency.
Code Sample
import unittest
from test_data_store import TestDataStore
class TestDataCleanup(unittest.TestCase):
@classmethod
def setUpClass(cls):
cls.data_store = TestDataStore("test_data.db")
def setUp(self):
# Insert test data before each test
self.data_store.insert_data("users", {"name": "Test User", "email": "test@example.com"})
def tearDown(self):
# Clean up test data after each test
self.data_store.clear_data()
def test_example(self):
# Your test code here
users = self.data_store.get_data("users")
self.assertEqual(len(users), 1)
self.assertEqual(users[0]["name"], "Test User")
if __name__ == '__main__':
unittest.main()
Advantages
Consistency: Ensures each test starts with a known, clean state, improving test reliability.
Isolation: Prevents data leakage between tests, making it easier to debug issues.
Automation: Integrates cleanly with existing test frameworks, requiring no manual intervention.
Flexibility: Can be easily customized to handle different types of test data or cleanup strategies.
Maintainability: Centralized cleanup logic makes it easier to update or modify data management practices.
Return on Investment (ROI)
Implementing robust test data management solutions offers significant benefits to organizations:
Improved Test Coverage: By generating diverse and realistic test data, you can uncover edge cases and bugs that might otherwise go unnoticed, leading to higher quality software.
Increased Efficiency: Automated data generation and cleanup processes save time and reduce manual effort, allowing testers to focus on more complex scenarios.
Reduced Environment-related Issues: Containerized test environments ensure consistency across different stages of development, minimizing the "it works on my machine" problem.
Enhanced Data Privacy and Compliance: Data masking techniques help organizations comply with data protection regulations, avoiding potential legal issues and fines.
Faster Time-to-Market: With more efficient testing processes, organizations can release software updates more frequently and with greater confidence.
Cost Savings: By catching bugs earlier in the development process, organizations can significantly reduce the cost of fixing issues in production.
To quantify the ROI, consider the following example:
Cost of implementing test data management solutions: $50,000
Annual savings in developer time: 500 hours @ $100/hour = $50,000
Reduction in production bugs: 20 fewer bugs @ $5,000 per bug = $100,000
Total annual savings: $150,000
ROI = (Annual Savings - Implementation Cost) / Implementation Cost ROI = ($150,000 - $50,000) / $50,000 = 200%
In this scenario, the organization would see a 200% return on investment in the first year alone, with ongoing benefits in subsequent years.
Additional ROI Considerations
Reduced Risk: Improved testing reduces the risk of costly data breaches or compliance violations.
Improved Team Morale: More reliable tests and fewer production issues lead to happier, more productive development teams.
Enhanced Reputation: Higher quality software and faster release cycles can improve customer satisfaction and market position.
Scalability: These solutions often become more valuable as the project scales, providing long-term benefits.
Conclusion
Effective test data management is crucial for modern software development. By implementing innovative solutions for data generation, storage, privacy, and cleanup, organizations can significantly improve their testing processes, leading to higher quality software and substantial cost savings.
The approaches outlined in this post - dynamic data generation, containerized environments, data masking, efficient storage, and automated cleanup - work together to create a comprehensive test data management strategy. Each solution addresses specific challenges in the testing process, from creating realistic data to ensuring test isolation and compliance.
The initial investment in robust test data management solutions pays off quickly, making it a wise choice for organizations of all sizes. As software projects grow in complexity and scale, the benefits of these approaches become even more pronounced, providing a strong foundation for efficient, reliable, and compliant testing practices.
By adopting these strategies, development teams can focus more on creating value and less on managing test data, ultimately leading to better software and more successful projects.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
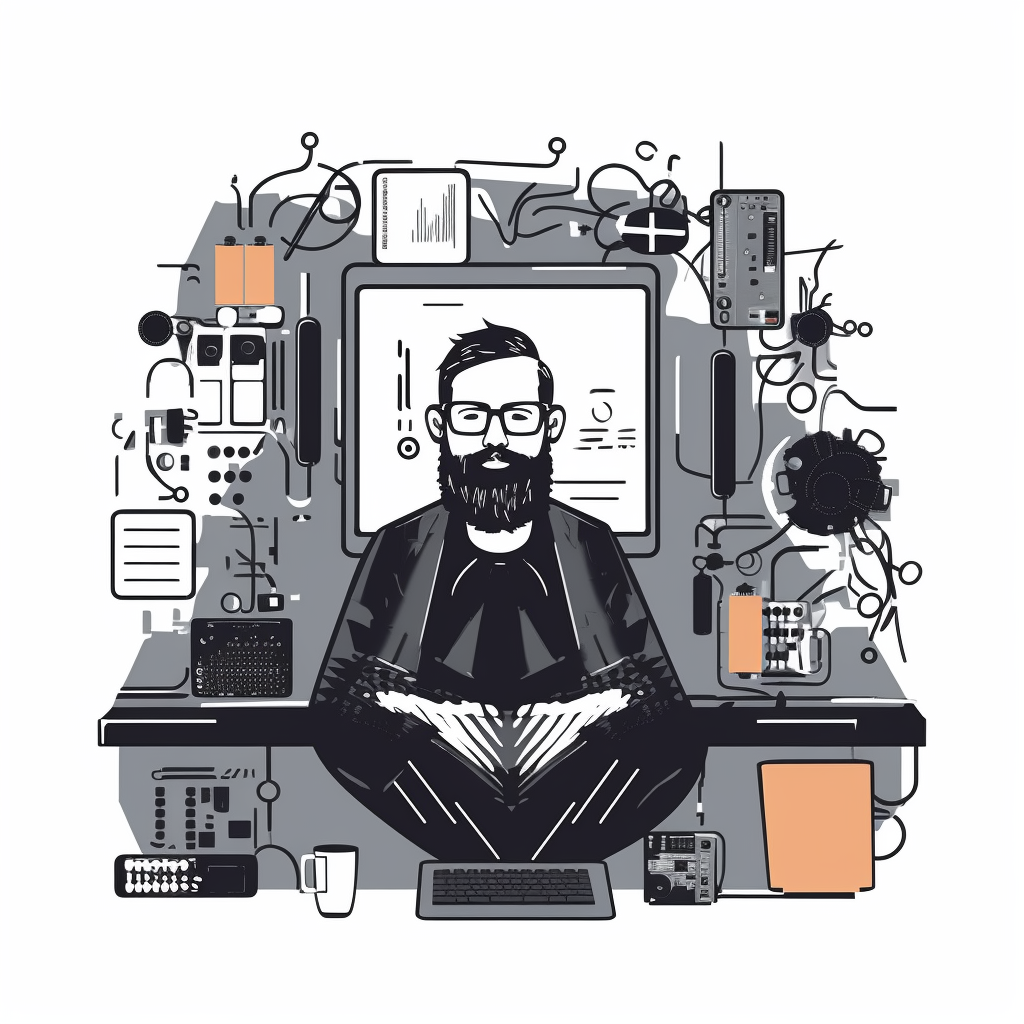
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.