Collections Framework in Java
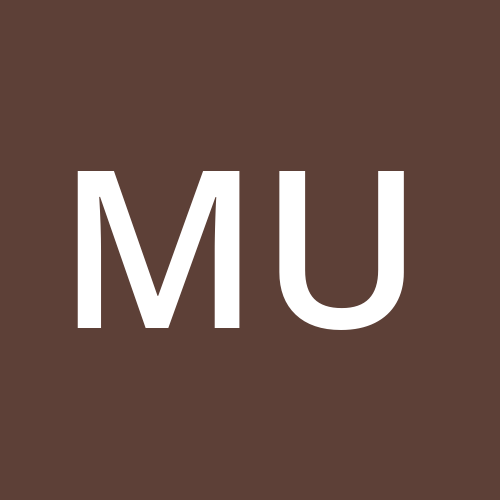
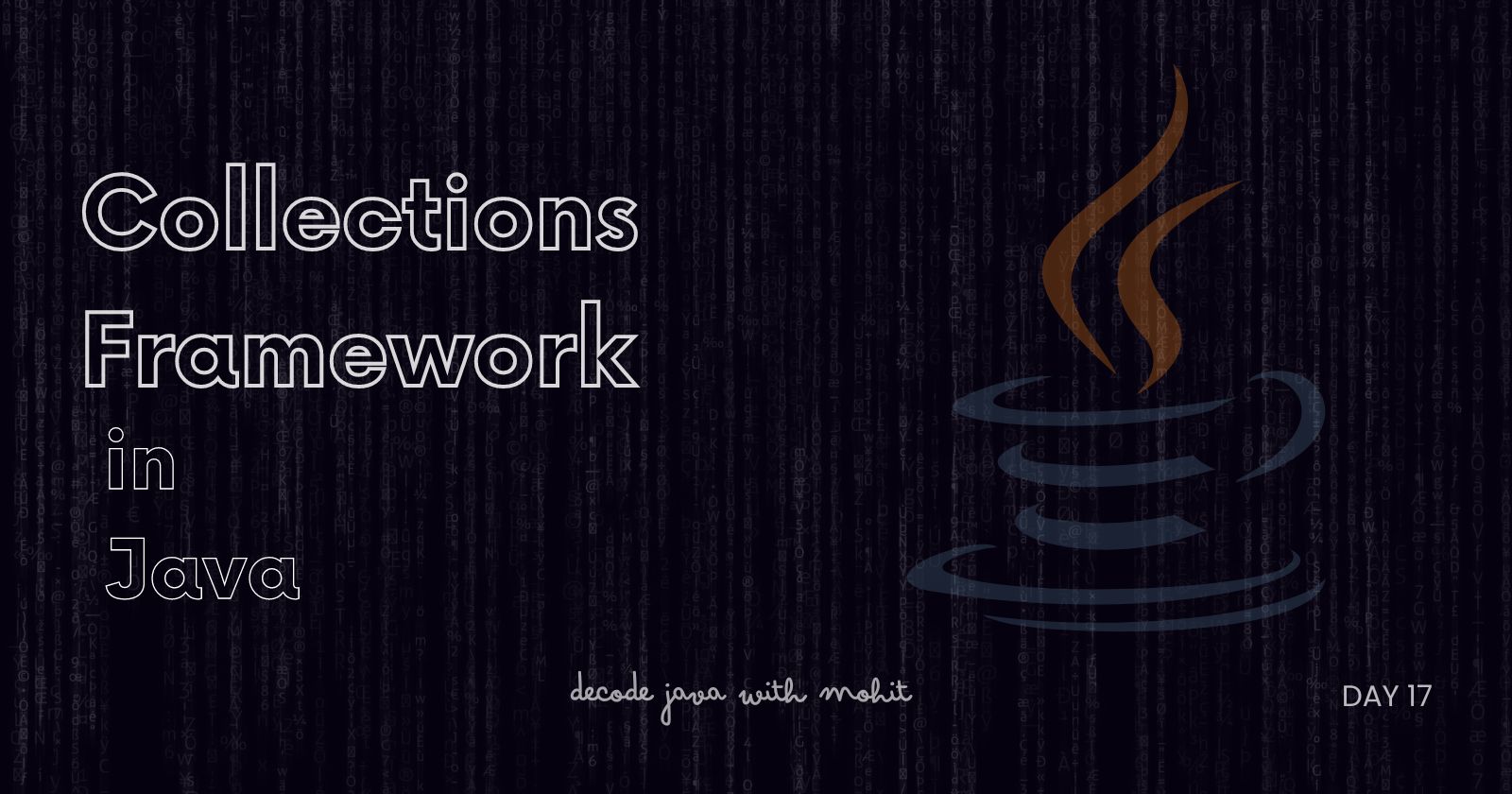
On Day 17, we dive into the Collections Framework in Java, a vital part of Java programming that allows developers to store, manage, and manipulate groups of objects efficiently.
The Collections Framework provides several interfaces, classes, and algorithms for working with data structures like lists, sets, maps, and more. Understanding this framework is crucial for handling large datasets and optimizing performance in Java applications.
1. What is the Collections Framework?
The Collections Framework in Java is a unified architecture for representing and manipulating collections of objects. It includes:
Interfaces: Define abstract data types for collections (e.g., List, Set, Map).
Implementations (Classes): Provide concrete implementations of these interfaces (e.g., ArrayList, HashSet, HashMap).
Algorithms: Provide methods to perform common tasks like sorting, searching, and shuffling (e.g.,
Collections.sort()
).
The framework is located in the java.util
package and helps in storing, retrieving, and managing groups of objects in a consistent and efficient manner.
2. Key Interfaces of the Collections Framework
There are several core interfaces in the Collections Framework, each representing a different type of collection:
A. List Interface
A List is an ordered collection that allows duplicate elements. Lists maintain the insertion order of elements and provide indexed access to them.
Common Implementations:
ArrayList: A resizable array that allows random access.
LinkedList: A doubly linked list that is more efficient for frequent insertions or deletions.
Vector: A synchronized version of ArrayList (less commonly used today).
Example:
import java.util.ArrayList;
import java.util.List;
public class ListExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.add("JavaScript");
System.out.println("List: " + list);
System.out.println("Element at index 1: " + list.get(1));
list.remove("Python");
System.out.println("After removal: " + list);
}
}
- In this example, an ArrayList is used to store and manipulate a list of programming languages.
B. Set Interface
A Set is an unordered collection that does not allow duplicate elements. It is useful when you need to store unique elements and do not care about the order of elements.
Common Implementations:
HashSet: A set backed by a hash table, provides constant time for basic operations like adding, removing, and checking for an element.
LinkedHashSet: Maintains the insertion order of elements.
TreeSet: A sorted set backed by a tree, provides log time operations and maintains a sorted order.
Example:
import java.util.HashSet;
import java.util.Set;
public class SetExample {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("Apple");
set.add("Banana");
set.add("Apple"); // Duplicate element
System.out.println("Set: " + set); // "Apple" will only appear once
}
}
- The HashSet in this example ensures that no duplicate values are added.
C. Map Interface
A Map is a collection that maps keys to values. Each key can map to only one value, and maps do not allow duplicate keys but can have duplicate values.
Common Implementations:
HashMap: Provides constant time for basic operations and does not maintain any order.
LinkedHashMap: Maintains the insertion order.
TreeMap: A sorted map that stores keys in ascending order.
Example:
import java.util.HashMap;
import java.util.Map;
public class MapExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Java", 100);
map.put("Python", 90);
map.put("C++", 80);
System.out.println("Map: " + map);
System.out.println("Score of Python: " + map.get("Python"));
map.remove("C++");
System.out.println("After removal: " + map);
}
}
- In this example, a HashMap is used to store programming languages and their respective scores.
3. Important Classes in the Collections Framework
A. ArrayList
An ArrayList is a resizable array implementation of the List interface. It provides fast random access to elements but is slower for adding or removing elements in the middle of the list.
Key Features:
Fast random access (
get()
method).Resizable, grows dynamically.
Allows duplicate elements.
Example:
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
ArrayList<String> arrayList = new ArrayList<>();
arrayList.add("Java");
arrayList.add("C++");
arrayList.add("Python");
System.out.println("ArrayList: " + arrayList);
}
}
B. HashSet
A HashSet is a collection that does not allow duplicates and does not maintain any order. It is best used when you need to ensure the uniqueness of elements.
Key Features:
Constant time for adding, removing, and checking elements.
No duplicate elements.
Example:
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
HashSet<String> hashSet = new HashSet<>();
hashSet.add("Apple");
hashSet.add("Orange");
hashSet.add("Banana");
System.out.println("HashSet: " + hashSet);
}
}
C. HashMap
A HashMap provides a way to store key-value pairs. It is one of the most widely used data structures for mapping data, especially when you need quick lookups based on a key.
Key Features:
Constant time for basic operations (add, remove, check).
No duplicate keys allowed.
Example:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
hashMap.put("Java", 100);
hashMap.put("Python", 90);
System.out.println("HashMap: " + hashMap);
}
}
4. Utility Methods in the Collections Class
The Collections
class provides various utility methods for manipulating collections such as sorting, searching, and shuffling. Some common methods include:
Collections.sort(List)
: Sorts a list.Collections.reverse(List)
: Reverses the order of elements in a list.Collections.shuffle(List)
: Shuffles the elements randomly.Collections.max(Collection)
: Returns the maximum element of the collection.Collections.min(Collection)
: Returns the minimum element of the collection.
Example:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class CollectionsUtilityExample {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(5);
numbers.add(30);
// Sort the list
Collections.sort(numbers);
System.out.println("Sorted List: " + numbers);
// Reverse the list
Collections.reverse(numbers);
System.out.println("Reversed List: " + numbers);
}
}
5. Best Practices with Collections Framework
Choose the Right Data Structure: Use the appropriate collection (e.g., ArrayList for fast access, LinkedList for frequent insertions/removals).
Use Generics: Always specify the type of elements the collection can hold to avoid
ClassCastException
.Avoid Using Raw Types: Always use parameterized types (e.g.,
List<String>
,Set<Integer>
) to enforce type safety.Prefer Interfaces: Use interfaces (e.g.,
List
,Set
,Map
) for defining collection types rather than specific implementations.Handle Null Values: Be cautious of
null
values, especially in sets and maps.
6. Summary
By the end of Day 17, readers will have a strong understanding of:
The structure of the Collections Framework in Java.
The core interfaces like List, Set, and Map.
Common classes such as ArrayList, HashSet, and HashMap.
Useful utility methods provided by the Collections class.
Best practices when working with collections.
Mastering the Collections Framework enables developers to work with complex data structures in Java efficiently, making it a key topic in advanced Java programming. Stay tuned! for further Advanced topics in Java.
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
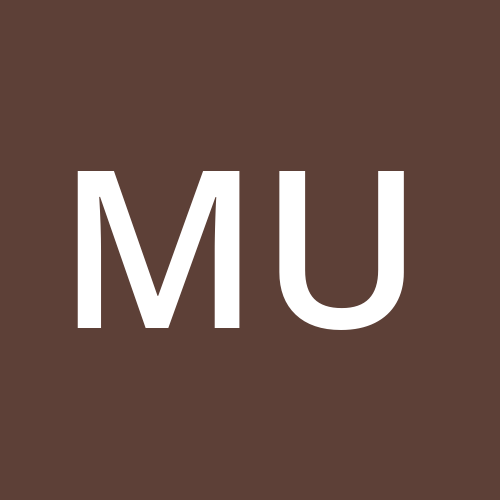