How to Use Scikit-learn for Classification Tasks: A Comprehensive Guide
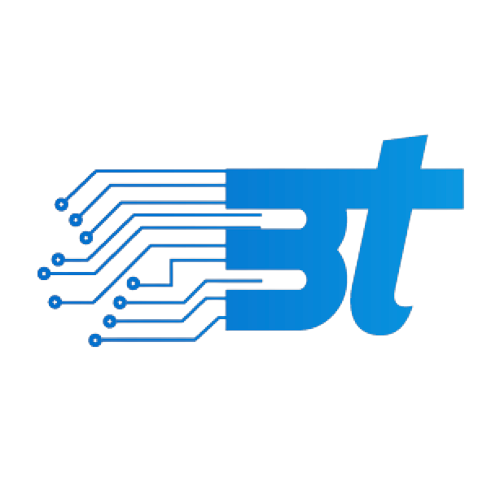
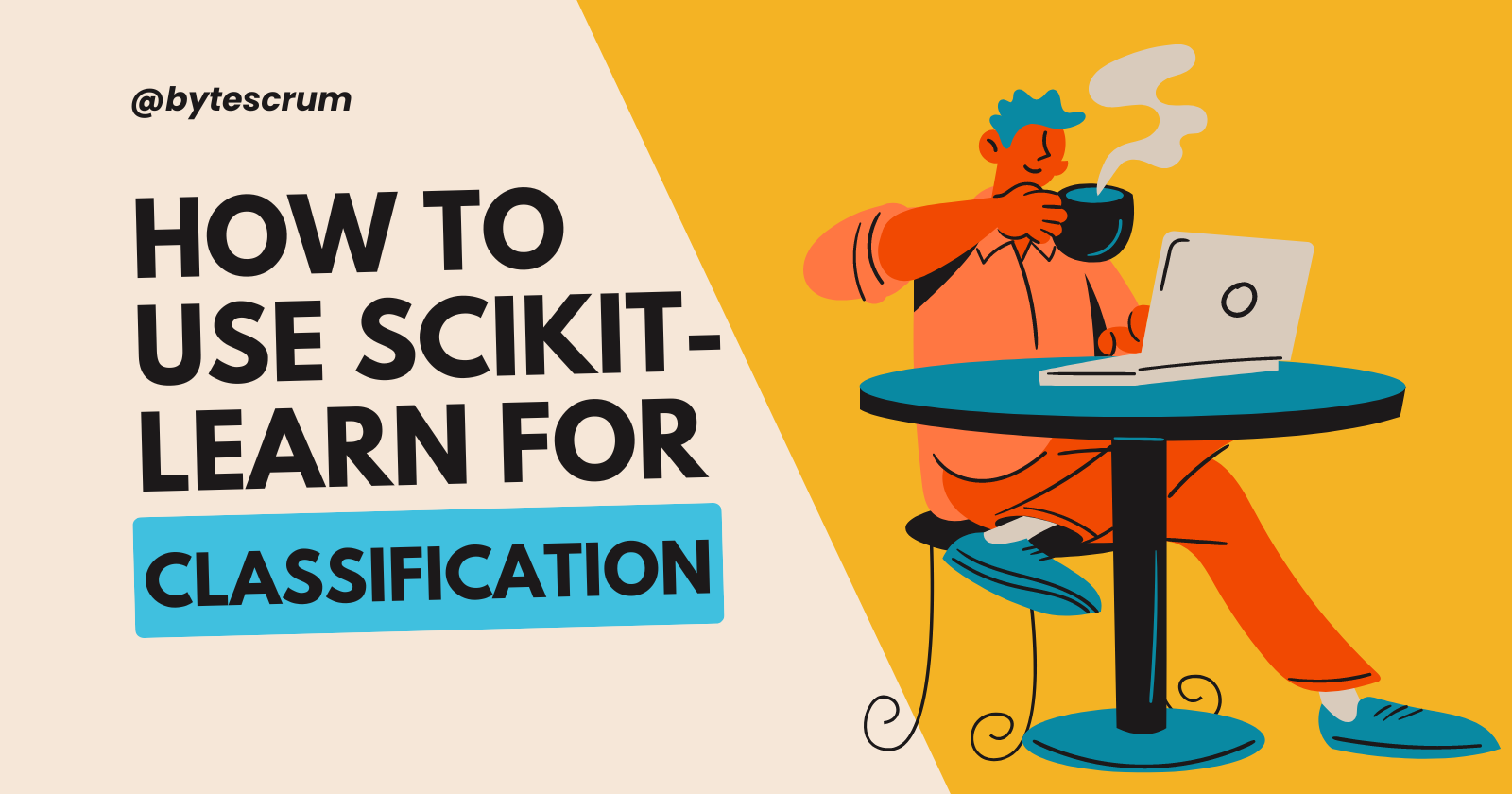
Classification is one of the most common tasks in machine learning, where the objective is to categorize data points into predefined labels or classes. Whether you're building a spam filter, diagnosing diseases, or identifying objects in an image, classification models play a crucial role in such tasks. Scikit-learn, one of the most popular machine learning libraries in Python, provides easy-to-use tools for implementing classification algorithms.
This blog will guide you through the process of performing classification using Scikit-learn, covering the essential steps: from loading data and preprocessing to building and evaluating classification models.
1. What is Classification?
Classification is a type of supervised learning where a model is trained on labeled data and is used to predict the class labels for new, unseen data. In simple terms, classification is about categorizing or assigning labels to data points. For example, determining whether an email is "spam" or "not spam" is a binary classification task, while identifying the type of flower based on its features could be a multi-class classification task.
The most commonly used types of classification tasks include:
Binary classification: Involves two classes (e.g., email spam detection: spam or not spam).
Multi-class classification: Involves more than two classes (e.g., categorizing types of flowers).
Multi-label classification: Where each instance can belong to more than one class (e.g., text categorization with multiple tags).
2. Why Scikit-learn for Classification?
Scikit-learn is one of the most widely used Python libraries for machine learning. Its design is simple and efficient, making it suitable for beginners and professionals alike. It provides a rich set of algorithms for classification tasks, including decision trees, support vector machines (SVM), logistic regression, random forests, and more.
Some key advantages of Scikit-learn include:
Simple and consistent API: Easy to understand, with standardized syntax across different models.
Versatile: Supports various machine learning tasks beyond classification, such as clustering, regression, and dimensionality reduction.
Comprehensive tools: Offers tools for preprocessing, model selection, and evaluation.
3. Step-by-Step Guide: Classification Using Scikit-learn
In this section, we’ll walk through a practical example of using Scikit-learn for classification tasks.
Step 1: Importing Necessary Libraries
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.metrics import accuracy_score, classification_report
from sklearn.ensemble import RandomForestClassifier
We import the following:
NumPy and Pandas for data manipulation.
train_test_split to split the dataset into training and testing subsets.
StandardScaler for feature scaling.
RandomForestClassifier as our classification model.
accuracy_score and classification_report for evaluating model performance.
Step 2: Loading and Exploring the Dataset
For this example, we will use the Iris dataset, which is included in Scikit-learn.
from sklearn.datasets import load_iris
iris = load_iris()
X = iris.data # Features
y = iris.target # Labels (species)
The Iris dataset contains 150 instances of iris flowers, with four features for each flower (sepal length, sepal width, petal length, petal width), and three species labels (Setosa, Versicolour, Virginica).
Step 3: Splitting the Dataset into Training and Test Sets
We now split the data into a training set (used for training the model) and a test set (used for evaluating the model).
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Step 4: Data Preprocessing (Feature Scaling)
Many machine learning algorithms perform better when features are on the same scale. We use StandardScaler
to standardize the data.
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
The StandardScaler
adjusts the features such that they have zero mean and unit variance.
Step 5: Building a Classification Model
We’ll use a Random Forest classifier, a robust algorithm that fits a multitude of decision trees and averages their results.
classifier = RandomForestClassifier(n_estimators=100, random_state=42)
classifier.fit(X_train, y_train)
The n_estimators
parameter defines the number of decision trees in the forest.
Step 6: Model Evaluation
After training the model, we evaluate its performance using the test set.
y_pred = classifier.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
The accuracy_score
function computes the ratio of correctly predicted instances. Additionally, we can use classification_report
to assess other metrics like precision, recall, and F1-score.
print(classification_report(y_test, y_pred, target_names=iris.target_names))
4. Popular Classification Algorithms in Scikit-learn
Here are a few widely used classification algorithms supported by Scikit-learn:
Logistic Regression: Best for binary classification tasks.
from sklearn.linear_model import LogisticRegression model = LogisticRegression()
Support Vector Machines (SVM): Useful for separating classes using a hyperplane in higher dimensions.
from sklearn.svm import SVC model = SVC(kernel='linear')
K-Nearest Neighbors (KNN): Classifies based on the majority class among its neighbors.
from sklearn.neighbors import KNeighborsClassifier model = KNeighborsClassifier(n_neighbors=3)
Decision Trees: A tree-like model of decisions that supports both classification and regression tasks.
from sklearn.tree import DecisionTreeClassifier model = DecisionTreeClassifier()
Random Forests: An ensemble method combining multiple decision trees to improve accuracy.
from sklearn.ensemble import RandomForestClassifier model = RandomForestClassifier()
5. Challenges in Classification
Classification, while a powerful tool, poses several challenges:
Imbalanced Datasets: Some classes may dominate, leading to skewed model predictions. Techniques like oversampling the minority class or using specialized algorithms can help.
Overfitting: When the model fits the training data too well, it may perform poorly on unseen data. Cross-validation and regularization techniques are effective in combating overfitting.
Curse of Dimensionality: High-dimensional data may reduce model performance. Dimensionality reduction techniques like PCA (Principal Component Analysis) can be applied.
Conclusion
With Scikit-learn, you have access to a wide variety of classification algorithms, empowering you to experiment and iterate until you achieve the best results.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
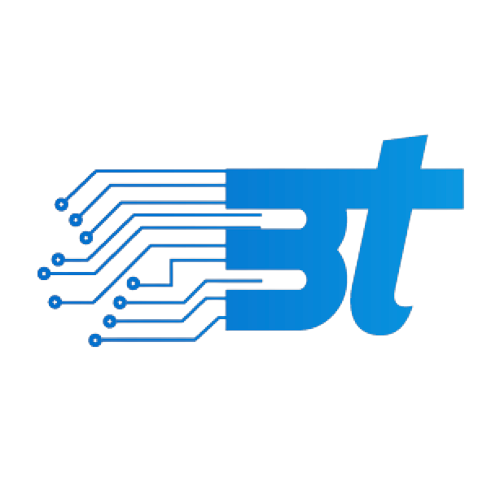
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.