Effective Error Handling in Flutter: Strategies for Building Robust Apps

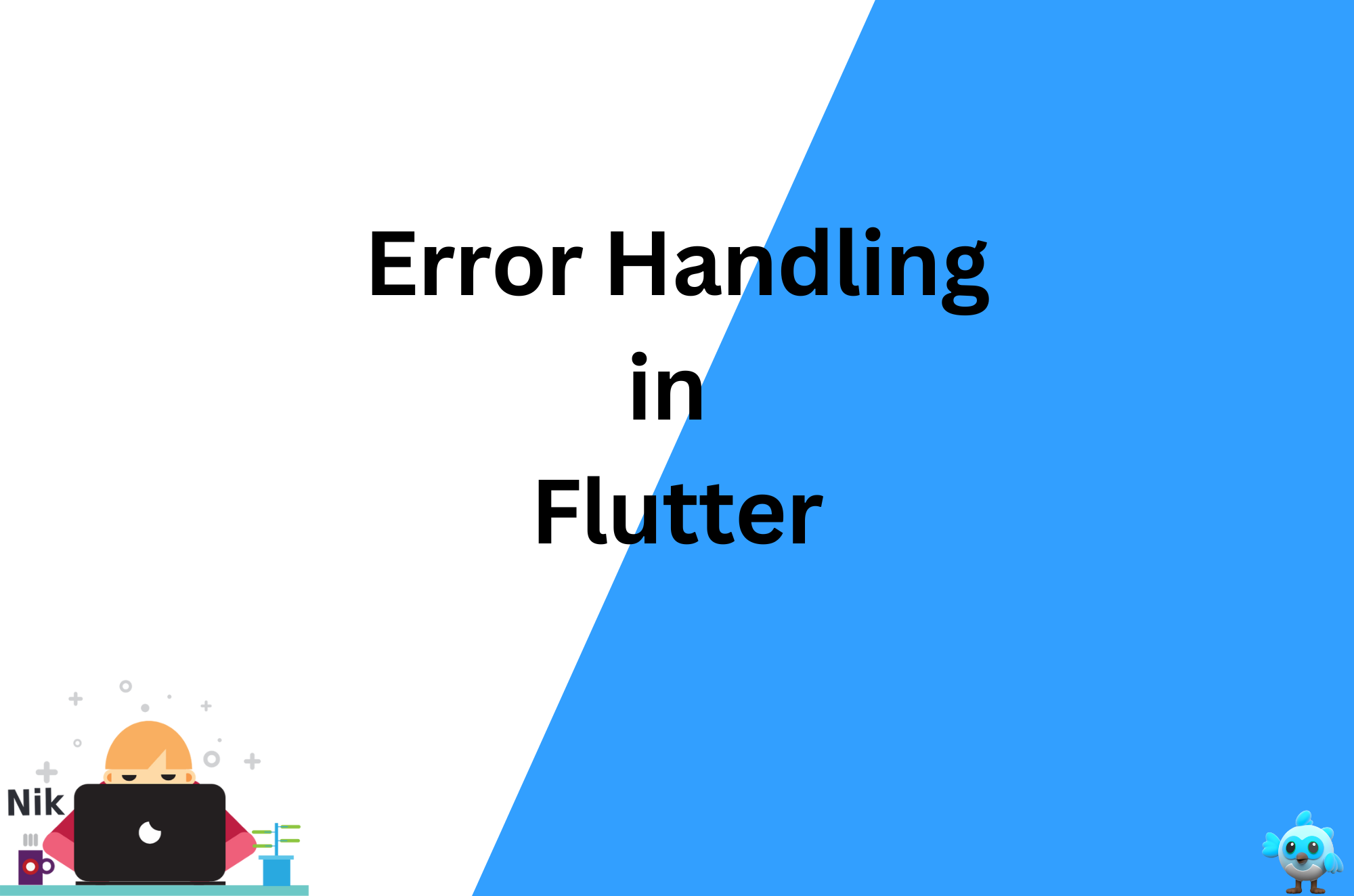
Error handling is a crucial aspect of app development that ensures a smooth user experience even when things go wrong. In Flutter, robust error handling helps you manage and respond to runtime errors effectively, improving your app’s reliability and user satisfaction. In this blog, we’ll explore various strategies for effective error handling in Flutter applications.
Why Error Handling Matters
Proper error handling:
Enhances User Experience: Prevents crashes and unexpected behaviors, providing a seamless experience.
Improves Debugging: Helps identify and fix issues more efficiently during development.
Maintains Application Stability: Ensures your app can handle errors gracefully without disrupting functionality.
Strategies for Error Handling in Flutter
1. Using Try-Catch for Synchronous Code
For synchronous code, use try-catch
blocks to handle exceptions and errors. This approach allows you to catch exceptions thrown during execution and handle them accordingly.
Example:
dartCopy codeclass TryCatchExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Try-Catch Example')),
body: Center(
child: ElevatedButton(
onPressed: () {
try {
int result = 10 ~/ 0; // This will throw a DivideByZeroException
} catch (e) {
print('Error: $e');
}
},
child: Text('Divide by Zero'),
),
),
);
}
}
2. Handling Errors in Asynchronous Code
For asynchronous code, use try-catch
within async
functions or handle errors with the Future
API. This ensures you catch and manage errors occurring in asynchronous operations.
Example:
dartCopy codeclass AsyncErrorHandling extends StatelessWidget {
Future<void> fetchData() async {
try {
// Simulate a network request
await Future.delayed(Duration(seconds: 2));
throw Exception('Failed to fetch data');
} catch (e) {
print('Error: $e');
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Async Error Handling')),
body: Center(
child: ElevatedButton(
onPressed: fetchData,
child: Text('Fetch Data'),
),
),
);
}
}
3. Using Flutter’s Error Widgets
Flutter provides built-in error widgets for displaying errors in the UI. Use ErrorWidget
to show user-friendly error messages when an error occurs during widget build.
Example:
dartCopy codeclass CustomErrorWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
builder: (context, child) {
ErrorWidget.builder = (errorDetails) {
return Scaffold(
body: Center(
child: Text(
'Something went wrong: ${errorDetails.exception}',
style: TextStyle(color: Colors.red),
),
),
);
};
return child!;
},
home: Scaffold(
appBar: AppBar(title: Text('Custom Error Widget')),
body: Center(
child: Text('No errors yet'),
),
),
);
}
}
4. Global Error Handling
Use FlutterError.onError
to catch and handle errors globally. This approach helps in logging and managing errors that occur outside the scope of individual widgets.
Example:
dartCopy codevoid main() {
FlutterError.onError = (FlutterErrorDetails details) {
// Log error details or send them to a monitoring service
print('Global Error: ${details.exception}');
};
runApp(MyApp());
}
5. Handling API Errors
When working with APIs, handle errors based on HTTP response statuses. Ensure you manage scenarios like network failures, server errors, and invalid responses.
Example:
dartCopy codeFuture<void> fetchApiData() async {
final response = await http.get(Uri.parse('https://api.example.com/data'));
if (response.statusCode == 200) {
// Process the response
} else {
throw Exception('Failed to load data: ${response.statusCode}');
}
}
Best Practices for Error Handling
Provide User-Friendly Messages: Display clear and helpful error messages to users.
Log Errors for Debugging: Use logging to capture error details and improve debugging.
Test Error Scenarios: Ensure your app handles various error scenarios effectively.
Implement Retry Logic: For network errors, consider implementing retry mechanisms.
Conclusion
Effective error handling is essential for building robust Flutter applications. By using try-catch
blocks, handling asynchronous errors, utilizing Flutter’s error widgets, and implementing global error handling, you can create applications that manage errors gracefully and provide a seamless user experience. Embrace these strategies to enhance your app’s stability and reliability.
Happy coding!
Subscribe to my newsletter
Read articles from Ravi Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ravi Patel
Ravi Patel
"📱 Passionate Flutter Developer with 6+ years of experience, dedicated to crafting exceptional mobile applications. 🚀 Adept at turning ideas into polished, user-friendly experiences using Flutter's magic. 🎨 Design enthusiast who believes in the power of aesthetics and functionality. 🛠️ Expertise in translating complex requirements into clean, efficient code. 🌟 Committed to staying updated with the latest trends and continuously pushing boundaries. Let's create something extraordinary together!"