Understanding the Virtual DOM, Fiber, and Reconciliation in React
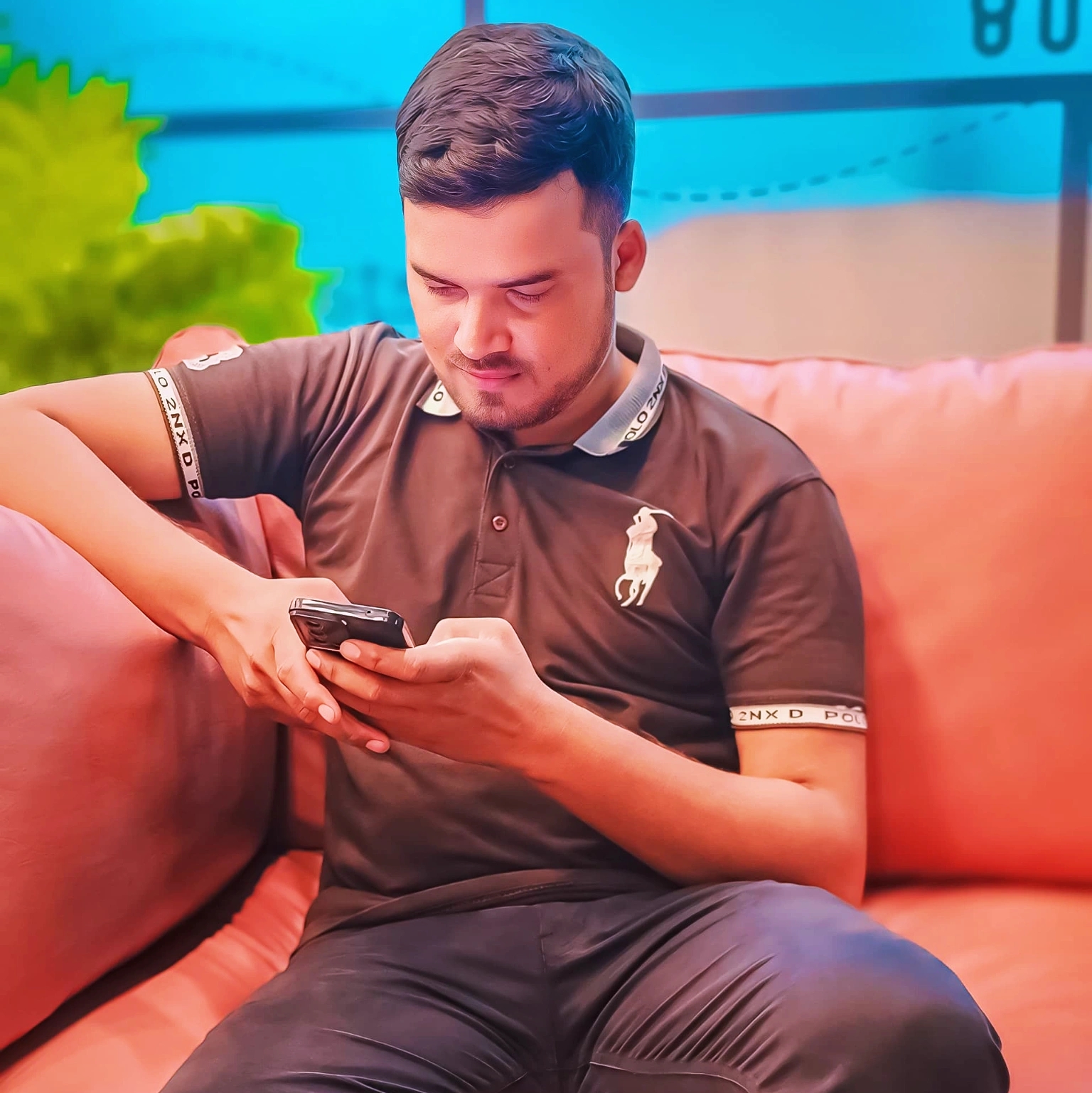
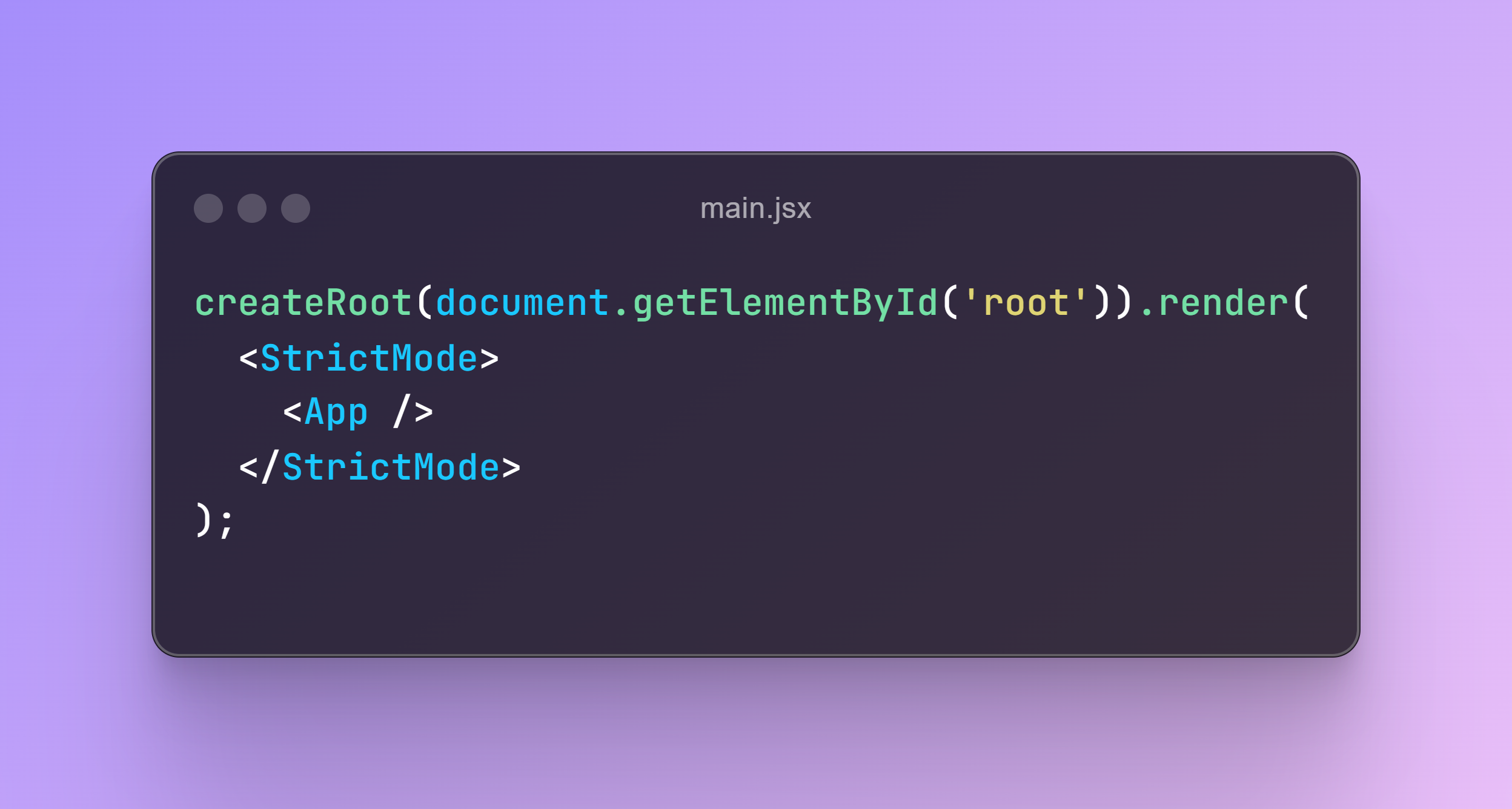
Today, I learned about one of the most important concepts in React: the Virtual DOM, Fiber, and Reconciliation. If you've worked with React, you've likely used the createRoot
function to render your app. But how does React manage to update the user interface so efficiently behind the scenes? Let's break down these concepts to understand what’s really happening!
What is the Virtual DOM?
The Virtual DOM is a key feature of React that makes UI updates fast and efficient. But how does it work?
In simple terms, the Virtual DOM is a lightweight copy of the real DOM (Document Object Model). The real DOM is the structure that represents everything on a webpage. When something changes in the UI, like the user typing into a form or clicking a button, the real DOM has to update. However, updating the real DOM is slow and can cause performance issues, especially in large applications.
React uses the Virtual DOM to solve this problem. Instead of directly updating the real DOM, React first updates the Virtual DOM. After that, it compares the new Virtual DOM with the previous version and only changes the parts of the real DOM that have been updated. This process is called diffing and helps React update the UI much faster than if it were updating the entire DOM every time something changes.
How Does React Render the App? Let's take a look at the following code:
createRoot(document.getElementById('root')).render( );
Here’s a breakdown of how this works:
createRoot: This function creates the root where your React app will live. The root is connected to an element in the actual DOM (in this case, the element with the id
root
).render: The
render
function takes the React components (in this case,<App />
) and renders them into the real DOM. But it doesn't just do this directly—it uses the Virtual DOM to figure out what needs to be updated.
So, whenever you run this code, React checks for changes in the Virtual DOM and then updates the real DOM as efficiently as possible.
What is Fiber?
Fiber is a key concept introduced in React 16 to make the rendering process even faster and smoother.
Earlier versions of React would try to update the entire app in one go. This was fine for small apps, but as apps became more complex, this approach started to cause performance issues. With Fiber, React breaks down the rendering process into smaller units of work. This allows React to pause and resume rendering, making the app more responsive even when complex updates are happening.
Reconciliation: How React Updates the UI
When React updates the UI, it goes through a process called Reconciliation. Here’s how it works:
React first compares the new Virtual DOM with the previous version. This process is called diffing.
React looks for differences between the two versions and decides which parts of the real DOM need to be updated.
Only the parts that have changed are updated in the real DOM.
This process ensures that React updates the UI as efficiently as possible, without unnecessary re-rendering of elements that haven’t changed.
Real-Time Working of Virtual DOM
I watched a fantastic YouTube video by Chai aur Code that explained the real-time working of the Virtual DOM. One interesting thing I learned is how React performs this process so quickly using diffing and reconciliation. It’s fascinating to see how React updates only the necessary parts of the real DOM without rebuilding the entire UI.
I also explored the React main.js file to see how the createRoot
function works behind the scenes. It was amazing to understand the inner workings of React and how it manages the DOM so efficiently.
Conclusion
Understanding the Virtual DOM, Fiber, and Reconciliation is crucial for any React developer. These concepts are what make React such a powerful and efficient framework for building user interfaces. By learning how React works behind the scenes, we can write more efficient code and build better-performing applications.
Learning Resources
Chai aur Code (YouTube Video): The video really helped me understand the real-time working of the Virtual DOM and Fiber.
React Documentation: Always a great place to dive deeper into how React works.
Subscribe to my newsletter
Read articles from Muhammad Waqar Rajput directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
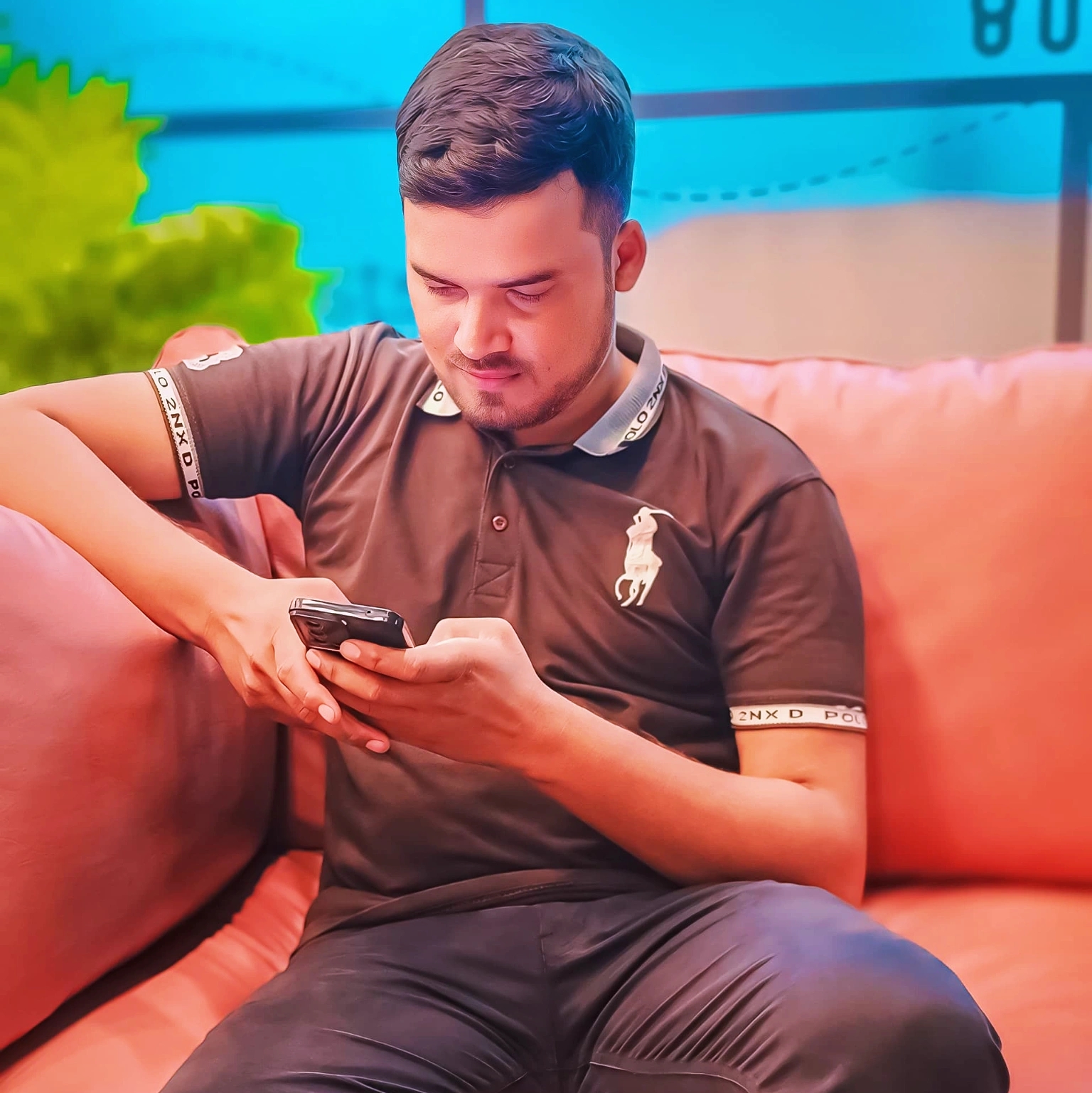
Muhammad Waqar Rajput
Muhammad Waqar Rajput
I’m Muhammad Waqar, a passionate Full-Stack Developer with 3.5 years of experience specializing in the MERN stack. I love building modern web applications using React.js, Node.js, and MongoDB, while continuously learning and exploring new technologies like TypeScript, Next.js, and 3D web development. I'm also delving into cybersecurity and cloud technologies. Join me on my journey as I share insights, tutorials, and projects focused on full-stack development and beyond!