Day 55 - Setting up AdonisJs +Prisma (ORM) + MySQL

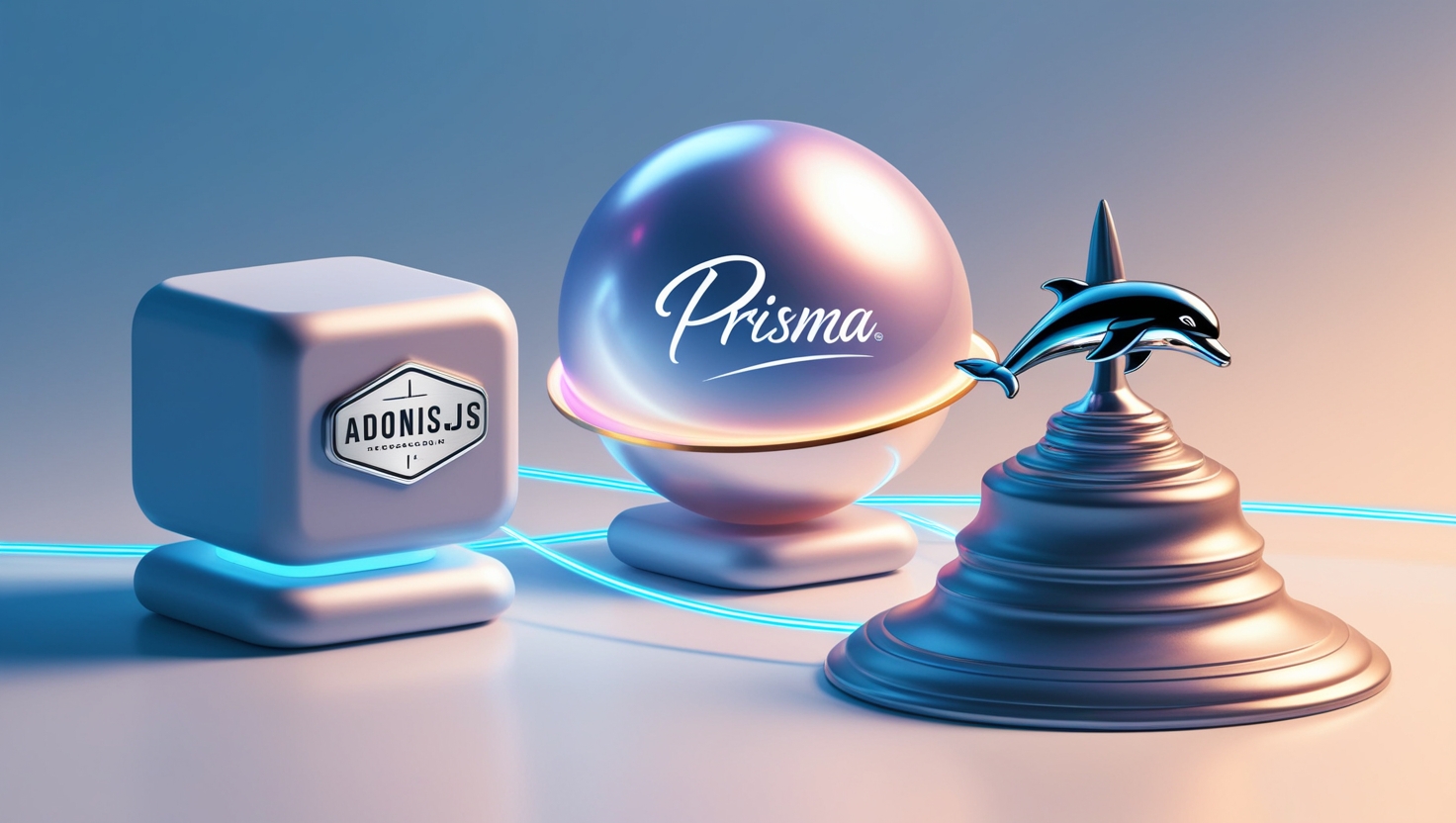
Today marks Day 55 of #100DaysOfCode. I set up an API using AdonisJS, connected it to a MySQL database with Prisma, and created a guide to help my colleagues contribute to the project.
In this article, I’ll walk through how I set up the API and connected it to MySQL using Prisma.
Why AdonisJs?
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Why did we choose AdonisJS? - According to Medium, AdonisJS has demonstrated better speed, performance, project structure, and more efficient memory and CPU usage compared to NestJS and TotalJS. Read the full article for details here.
AdonisJS offers numerous built-in features, including CORS, error handling, JSON parsing, testing capabilities, and more.
Setting up Adonisjs
- To create the new API, run the following command in your terminal
npm init adonisjs@latest <api-name> -- --kit=api
Replace <api-name>
with the desired name for your new API. You can also customize the installation to include your preferred database and other options. For more details, refer to the AdonisJS documentation.
To run the application, use the following command:
node ace serve --watch
The server runs locally at http://localhost:3333
and automatically watches for file changes, applying updates in real-time.
Link MySQL database using Prisma
To link a MySQL database to your AdonisJS project using Prisma, follow these steps:
Step 1: Install Prisma and Dependencies
- First, install the necessary packages for Prisma in your the project:
npm install prisma @prisma/client
Step 2: Initialize Prisma
Run the following command to initialize Prisma in the project. This will create a prisma/
folder and a schema.prisma
file:
npx prisma init
You will see a directory structure like this:
prisma/
└── schema.prisma
Step 3: Configure the MySQL Database
In your .env
file, add your MySQL database credentials. The .env
file might look like this:
DATABASE_URL="mysql://username:password@localhost:3306/database_name"
Replace username
, password
, localhost
, and database_name
with your actual MySQL database credentials.
Step 4: Define the Prisma Schema
In the prisma/schema.prisma
file, configure Prisma to use the MySQL provider by updating the datasource
block:
datasource db {
provider = "mysql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
Next, you can define your database models (tables) in the schema.prisma
file.
For example:
model User {
id Int @id @default(autoincrement())
email String @unique
name String
createdAt DateTime @default(now())
}
This defines a User
model with id
, email
, name
, and createdAt
fields.
Step 5: Migrate the Database
Run the following command to create the database tables according to your Prisma schema:
npx prisma migrate dev --name init
This will generate the migration files and apply them to your MySQL database.
Step 6: Generate the Prisma Client
Prisma uses a client to interact with the database. To generate the Prisma client, run:
npx prisma generate
This will create the client in node_modules/.prisma
.
5. Run the Application
Once the migration is applied and the Prisma client is regenerated, you can start or restart your application:
node ace serve --watch
By following these steps, you should now have your API set up with AdonisJS and successfully connected to your MySQL database.
Thanks for reading 😊
Feel free to reach out if you have any feedback or suggestions.
Subscribe to my newsletter
Read articles from Allahisrabb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Allahisrabb
Allahisrabb
Software Engineer | Freelancer